【LeetCode & 劍指offer刷題】樹題9:34 二叉樹中和為某一值的路徑(112. Path Sum)
阿新 • • 發佈:2019-01-05
【LeetCode & 劍指offer 刷題筆記】目錄(持續更新中...)
112. Path Sum
Given a binary tree and a sum, determine if the tree has a root-to-leaf path such that adding up all the values along the path equals the given sum. Note: A leaf is a node with no children. Example: Given the below binary tree and
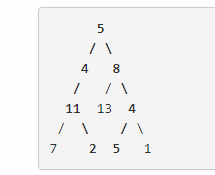
Return: [ [5,4,11,2], [5,8,4,5] ] /** * Definition for a binary tree node. * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right; * TreeNode(int x) : val(x), left(NULL), right(NULL) {} * }; */ /* 求所有和等於某數的路徑 */ #include <numeric> //算容器類元素和可用accumulate函式 class Solution { public : vector < vector < int >> pathSum ( TreeNode * root , int sum ) { vector < vector < int >> result ; vector < int > path ; path_sum ( root , path , result , sum ); return result ; } private : void path_sum ( TreeNode * root , vector < int >& path , vector < vector < int >>& result , int gap ) { if ( root == nullptr ) return ; //遞迴出口 else path . push_back ( root -> val ); // 儲存結點元素到path if ( root -> left == nullptr && root -> right == nullptr ) //葉子結點時push path到結果向量中 { if ( gap == root -> val ) result . push_back ( path ); //如果該path和為sum則push到結果向量中(這裡用sum累減路徑上的元素,得到gap與路徑上最後一個元素比較,節省時間,如果得到path再accumulate,則會造成不同路徑間的重複計算) // return; //遞迴出口,到葉結點後退出,(不能寫這句,還需執行到結尾進行pop) } path_sum ( root -> left , path , result , gap - root -> val ); //沿深度方向遍歷 path_sum ( root -> right , path , result , gap - root -> val ); path . pop_back (); // 刪除最後一個元素 ,騰出空間(本函式中只push了一次,故只需pop一次) } };