Spring boot使用 hello world
阿新 • • 發佈:2019-01-08
建立完成後,修改pom.xml檔案內容如下: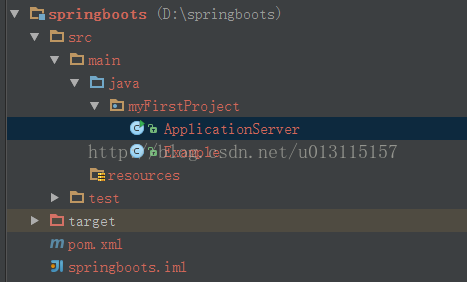
ApplicationServer.java內容: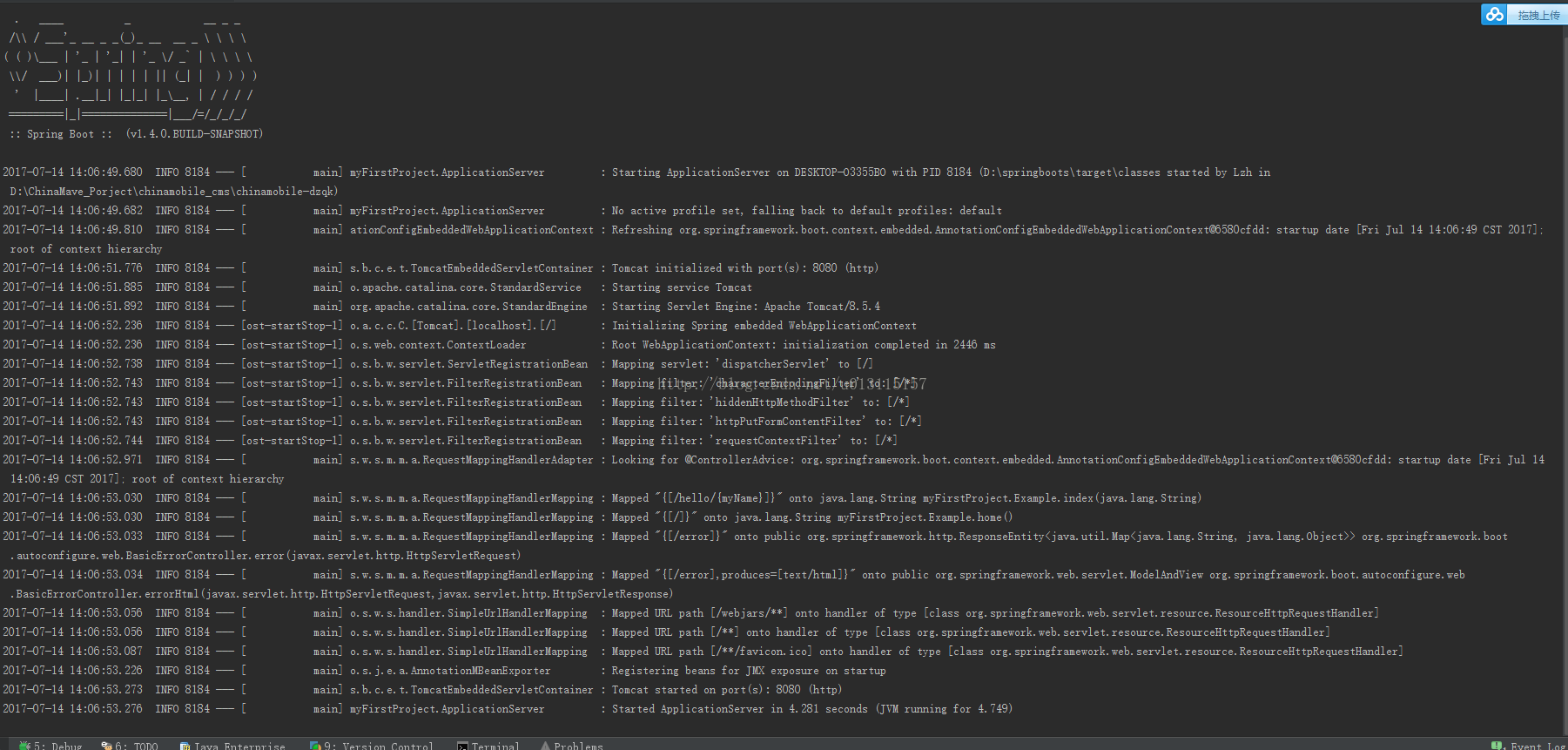
然後在瀏覽器中訪問地址localhost:8080localhost:8080/hello/God頁面會輸出: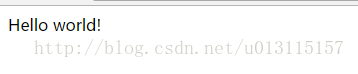
建立ApplicationServer與Example兩個類<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>springboots</groupId> <artifactId>com.springboot</artifactId> <version>1.0-SNAPSHOT</version> <!-- 繼承Spring boot 預設值 --> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.4.0.BUILD-SNAPSHOT</version> </parent> <!--新增典型的依賴關係到應用程式中 --> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> </dependencies> <!-- 打包一個可執行的jar --> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> <!--新增Spring儲存庫 --> <!-- (如果您使用的是.RELEASE版本,則不需要此功能) --> <repositories> <repository> <id>spring-snapshots</id> <url>http://repo.spring.io/snapshot</url> <snapshots><enabled>true</enabled></snapshots> </repository> <repository> <id>spring-milestones</id> <url>http://repo.spring.io/milestone</url> </repository> </repositories> <pluginRepositories> <pluginRepository> <id>spring-snapshots</id> <url>http://repo.spring.io/snapshot</url> </pluginRepository> <pluginRepository> <id>spring-milestones</id> <url>http://repo.spring.io/milestone</url> </pluginRepository> </pluginRepositories> </project>
ApplicationServer.java內容:
我們的應用程式最後部分是main方法。這只是一個標準的方法,它遵循Java對於一個應用程式入口點的約定。我們的main方法通過呼叫run,將業務委託給了Spring Boot的SpringApplication類。SpringApplication將引導我們的應用,啟動Spring,相應地啟動被自動配置的Tomcat web伺服器。我們需要將 Example.class 作為引數傳遞給run方法來告訴SpringApplication誰是主要的Spring元件。為了暴露任何的命令列引數,args陣列也會被傳遞過去Example.java內容:package myFirstProject; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; /** * Created by Lzh on 2017/7/14. */ @SpringBootApplication public class ApplicationServer { public static void main(String[] args) { SpringApplication.run(ApplicationServer.class, args); } }
我們的Example類上使用的第一個註解是 @RestController 。這被稱為一個構造型(stereotype)註解。它為閱讀程式碼的人們提供建議。對於Spring,該類扮演了一個特殊角色。在本示例中,我們的類是一個web @Controller ,所以當處理進來的web請求時,Spring會詢問它。@RequestMapping 註解提供路由資訊。它告訴Spring任何來自"/"路徑的HTTP請求都應該被對映到 home方法。@RestController 註解告訴Spring以字串的形式渲染結果,並直接返回給呼叫者。注: @RestController 和 @RequestMapping 註解是Spring MVC註解(它們不是Spring Boot的特定部分)。具體檢視Spring參考文件的MVC章節@EnableAutoConfiguration註解 第二個類級別的註解是 @EnableAutoConfiguration 。這個註解告訴Spring Boot根據新增的jar依賴猜測你想如何配置Spring。由於 spring-boot-starter-web 添加了Tomcat和Spring MVC,所以auto-configuration將假定你正在開發一個web應用並相應地對Spring進行設定啟動ApplicationServer中的主函式。會在控制輸出如下圖所示的資訊package myFirstProject; import org.springframework.boot.autoconfigure.EnableAutoConfiguration; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; /** * Created by Lzh on 2017/7/14. */ @RestController @EnableAutoConfiguration public class Example { @RequestMapping("/") String home() { return "Hello World!"; } @RequestMapping("/hello/{myName}") String index(@PathVariable String myName) { return "Hello "+myName+"!!!"; } }
然後在瀏覽器中訪問地址localhost:8080localhost:8080/hello/God頁面會輸出: