1、模板引擎
用於渲染頁面
介紹jade或ejs
jade:侵入式,與原生html/css不共存,使用縮排代表層級
模板字尾.jade
ejs:則非侵入式的
2、jade
1)簡單使用:
//程式碼
const jade = require('jade') let str=jade.renderFile('./template/a.jade') console.log(str) //模板
//模板部分
html
head
style
script
body
div
ul
li
li
li
div
2)語法:根據縮排,確定層級
在jade模板內書寫屬性,例如為script標籤新增src屬性
script(src="a.js")
div(style="width:200px;height:150px")
上述內容也可以使用json表示,但只有style標籤允許
同理,class可以使用陣列進行表示
div(class=['a','b'])
div(style={"width:200px;height:150px"})
若有多個屬性需要設定,則使用逗號分隔:
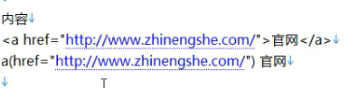
const jade = require('jade')
const fs=require('fs')
let str=jade.renderFile('./template/a.jade')
fs.writeFile('./result.html',str,function(err){
if(err){
console.log('failed')
}else{
console.log('ok')
}
})
console.log(str)
若要書寫內容,則在標籤後空一格寫內容即可,但是後面的內容不可換行
簡寫:
div.app
div#app
如果一定要以物件的方式為元素書寫屬性的話,可以:
div&attributes({各屬性})
使用attribute顯式說明
jade自動區分單雙標籤,所有的自定義標籤均識別為雙標籤
但是有時候會出現我們的內容被jade識別為自定義標籤的情況
我們在內容之前加|,即可按內容輸出,如:
|abc
也可以解決上面大段js程式碼換行後的內容報錯問題
或者使用.
表示.後的所有次及內容都原樣輸出
或者通過外鏈引入,使用Include即可:
在jade中使用變數:
//使用
const jade = require('jade') console.log(jade.renderFile('./template/a.jade',{pretty:true,name:'小智'}))//進行自動格式化 //模板
html
head
body
div 我的名字是:#{name} #{variable}:使用變數的格式,裡面同樣可以放表示式
同時,我們隊class和style屬性有靈活的書寫方式:
const jade = require('jade') console.log(jade.renderFile('./template/a.jade', {
pretty: true,
json:{width:'200px',height:'400px',display:'block'},
arr:['aaa','bbb']
}))
與html不同的是,jade允許你設定多個class標籤,編譯時它將自動為你合併
在jade內定義和使用變數,使用-
html
head
body
-var a=12
-var b=5 //不會輸出到模板上
div 結果是:#{a+b}
html
head
body
-var a=12
-var b=5
span #{a}
span=b 上下兩個寫法等價
jade的迴圈語法:
html
head
body
-for(var i=0;i<arr.length;i++)
div=arr[i]
jade解析輸出html標籤,為了防止使用者在輸入時進行注入式攻擊,它將不會編譯html標籤
const jade = require('jade') console.log(jade.renderFile('./template/a.jade', {
pretty: true,
content:'<h2>你好呀金合歡花<h2>'
}))
jade分支語法:
if:
html
head
body
-var a=12
-if(a>10)
div 偶數
-else
div 奇數
switch:使用case-when代替switch關鍵字
html
head
body
-var a=12
case a
when 0
div a
when 1
div b
when 12
div c
default
div d
注意,前面的給了-字首註明這是程式碼時,不中斷的前提下
後面的js程式碼不用加-
一個比較完整的例項:
const jade = require('jade')
const fs=require('fs') let str=jade.renderFile('./template/a.jade',{
pretty:true
})
fs.writeFile('./result.html',str,function(err){
if(err){
console.log('failed!')
}else{
console.log('ok')
}
})
doctype
html
head
meta(charset="utf-8")
title 測試頁面
style.
div{
width:100px;
height:400px;
border:1px solid red
}
body
-var a=0
while a++<12
if a%4==0&&a!=0
h1 #{a}
else
h2 #{a}