基於ORM實現用戶登錄
阿新 • • 發佈:2017-09-14
詳細信息 lte value 效果 input cmd abs 列表 htm
1. 與數據庫中的數據進行比較,檢驗用戶名和密碼是否正確。
2. 拿到的是QuerySet類型,類似於一個列表。驗證成功/失敗,返回到不同的頁面。
u = request.POST.get(‘user‘)
p = request.POST.get(‘pwd‘)
obj=models.UserInfo.objects.filter(username=u,password=p)
obj=models.UserInfo.objects.filter(username=u,password=p).first() #只拿第一個匹配的。
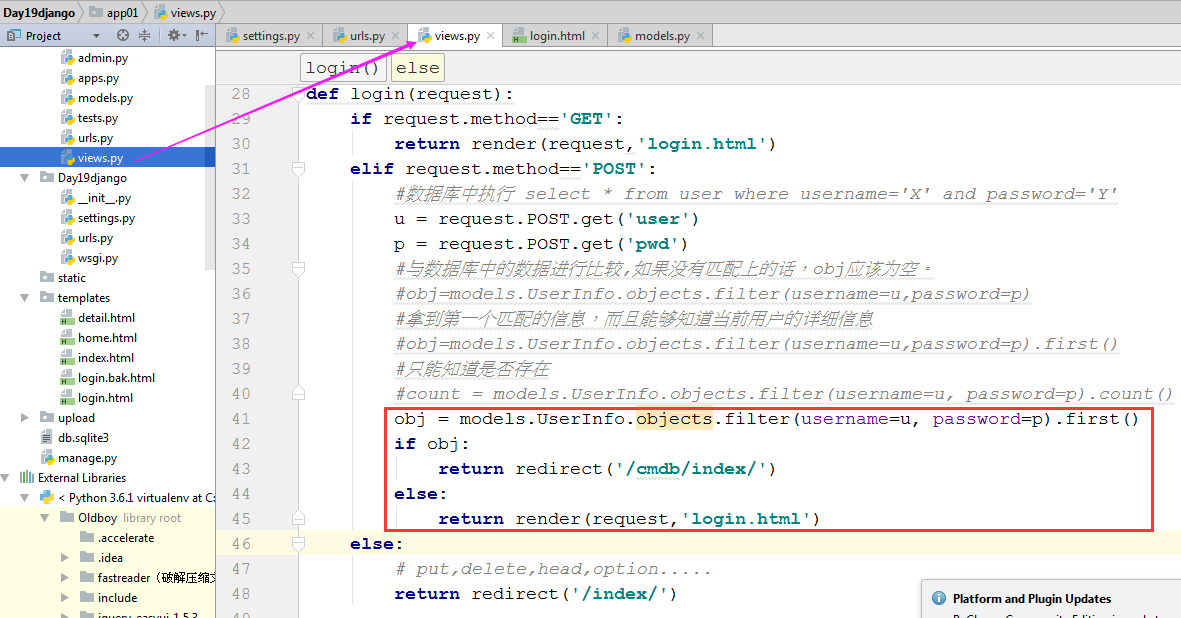
3. 如果驗證成功,則登陸後臺管理頁面
3.1寫路由
3.2 寫程序
3.3 寫index模板
3.4測試效果
4. 查看用戶信息
最終的呈現效果:
4.1 在urls.py中寫對應關系
4.2 在views.py中寫主體函數
views.py的全部函數如下,裏面包含了驗證用戶名和密碼的部分
from django.shortcuts import render,HttpResponse,redirect def login(request): if request.method==‘GET‘: return render(request,‘login.html‘) elif request.method==‘POST‘: #數據庫中執行 select * from user where username=‘X‘ and password=‘Y‘ u = request.POST.get(‘user‘) p = request.POST.get(‘pwd‘) obj = models.UserInfo.objects.filter(username=u, password=p).first() if obj: return redirect(‘/cmdb/index/‘) else: return render(request,‘login.html‘) else: return redirect(‘/index/‘) def index(request): return render(request,‘index.html‘) def user_info(request): user_list=models.UserInfo.objects.all() #QuerySet列表類型[obj,obj,obj] return render(request, ‘user_info.html‘,{‘user_list‘:user_list}) def user_detail(request,nid): obj=models.UserInfo.objects.filter(id=nid).first() #取單條數據,如果不存在,直接報錯。 #models.UserInfo.objects.get(id=nid) return render(request,‘user_detail.html‘,{‘obj‘:obj}) from app01 import models def orm(request): #增,創建方法1 #models.UserInfo.objects.create(username=‘root‘,password=‘123‘) # 增,創建方法2 #dic={‘username‘:‘eric‘,‘password‘:‘666‘} #models.UserInfo.objects.create(**dic) # 增,創建方法3 #obj=models.UserInfo(username=‘alex‘,password=‘123‘) #obj.save() #查 #result1=models.UserInfo.objects.all() #result2 = models.UserInfo.objects.filter(username=‘root‘) #all表示把這個表中的所有數據都拿到。 # 返回的result是QuerySet類型的,這個類型是Django提供的。可以把QuerySet理解成是個列表[]。 #[obj(id,username,password),obj(id,username,password),obj(id,username,password)] #刪除-所有數據 #models.UserInfo.objects.all().delete() #刪除-某些數據 #models.UserInfo.objects.filter(id=4).delete() #更新 models.UserInfo.objects.all().update(password=6669) for row in result1: print(row.id,row.username,row.password) print(result1) return HttpResponse(‘orm‘)
4.3在templates下寫html模板
login.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <form action="/cmdb/login/" method="POST" enctype="multipart/form-data"> <p> <input type="text" name="user" placeholder="用戶名"/> </p> <p> <input type="text" name="pwd" placeholder="密碼"/> </p> <input type="submit" value="提交"/> </form> </body> </html>
index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <style> body{ margin:0; } .menu{ display:block; padding:5px; } </style> </head> <body> <div style="height:48px;background-color:black;color:white"> 歡迎登陸教育系統 </div> <div> <div style="position:absolute;top:48px;bottom:0;left:0;width:200px;background-color:brown;"> <a class="menu" href="/cmdb/user_info/">用戶管理</a> <a class="menu" href="/cmdb/user_group/">用戶組管理</a> </div> </div> <div style="position:absolute;top:48px;left:210px;bottom:0;right:0;overflow:auto"> </div> </body> </html>
user_info.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <style> body{ margin:0; } .menu{ display:block; padding:5px; } </style> </head> <body> <div style="height:48px;background-color:black;color:white"> 歡迎登陸教育系統 </div> <div> <div style="position:absolute;top:48px;bottom:0;left:0;width:200px;background-color:brown;"> <a class="menu" href="/cmdb/user_info/">用戶管理</a> <a class="menu" href="/cmdb/user_group/">用戶組管理</a> </div> <div style="position:absolute;top:48px;left:210px;bottom:0;right:0;overflow:auto"> <h3>用戶列表</h3> <ul> {% for row in user_list %} <li><a href="/cmdb/userdetail-{{row.id}}/">{{row.username}}</a></li> {% endfor %} </ul> </div> </div> </body> </html>
user_detail.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <style> body{ margin:0; } .menu{ display:block; padding:5px; } </style> </head> <body> <div style="height:48px;background-color:black;color:white"> 歡迎登陸教育系統 </div> <div> <div style="position:absolute;top:48px;bottom:0;left:0;width:200px;background-color:brown;"> <a class="menu" href="/cmdb/user_info/">用戶管理</a> <a class="menu" href="/cmdb/user_group/">用戶組管理</a> </div> <div style="position:absolute;top:48px;left:210px;bottom:0;right:0;overflow:auto"> <h1>用戶詳細信息</h1> <h5>{{obj.id}}</h5> <h5>{{obj.name}}</h5> <h5>{{obj.password}}</h5> </div> </div> </body> </html>
5.增加信息
5.1 對應關系不用修改,修改函數如下:
為了避免重復代碼,也可以用redirect函數
5.2 修改user_info.html 模板
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <style> body{ margin:0; } .menu{ display:block; padding:5px; } </style> </head> <body> <div style="height:48px;background-color:black;color:white"> 歡迎登陸教育系統 </div> <div> <div style="position:absolute;top:48px;bottom:0;left:0;width:200px;background-color:brown;"> <a class="menu" href="/cmdb/user_info/">用戶管理</a> <a class="menu" href="/cmdb/user_group/">用戶組管理</a> </div> <div style="position:absolute;top:48px;left:210px;bottom:0;right:0;overflow:auto"> <h3>添加用戶</h3> <form method="POST" action="/cmdb/user_info/"> <input type="text" name="user"/> <input type="text" name="pwd"/> <input type="submit" name="添加"/> </form> <h3>用戶列表</h3> <ul> {% for row in user_list %} <li><a href="/cmdb/userdetail-{{row.id}}/">{{row.username}}</a></li> {% endfor %} </ul> </div> </div> </body> </html>
5.3 效果:
6. 增加刪除的功能。
6.1增加對應關系
6.2修改程序
6.3 更新模板
6.4測試效果
基於ORM實現用戶登錄