轉載-iOS SDK開發
最近幫兄弟公司的做支付業務sdk,積累了 sdk 封裝的經驗!下面我會從零開始把我的 sdk 封裝和調試經歷分享給大家,希望能給看到這篇文章的人有所幫助!
本文我會從以下幾個方面來講述:
-
Framework生成配置以及集成在主項目裏調試
-
xib 文件和圖片的存放和引用
-
Pods以及第三方庫的使用
-
Framework導出與文檔
Framework生成配置以及集成在主項目裏調試
1.新建主項目,主項目的 ProjectName 是 HelloFramework(也就是我們要使用 sdk 業務的主項目)
2.接下來在主項目裏創建Framework,就叫MyFramework好了
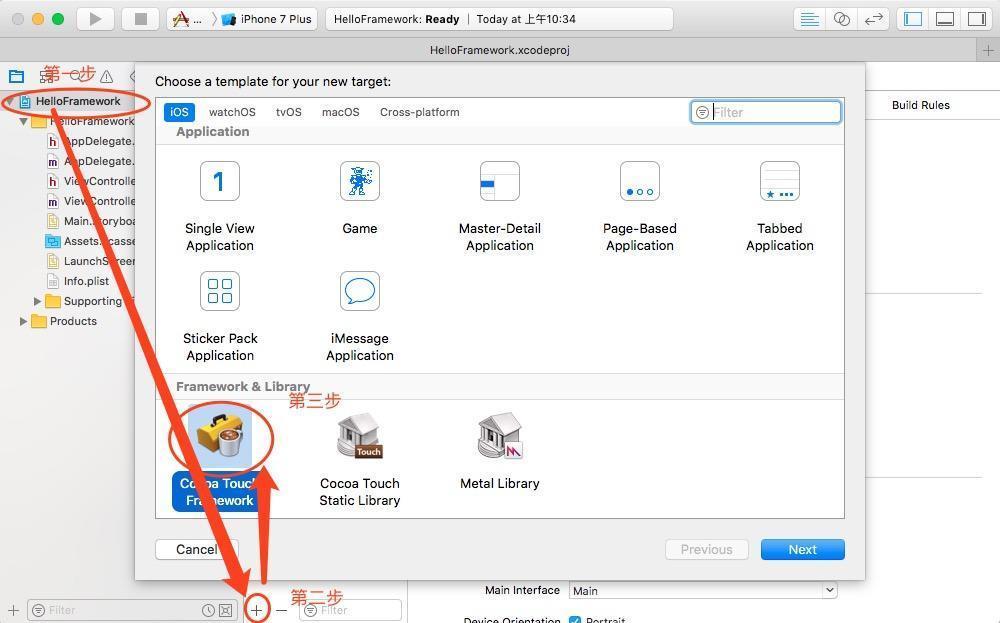
3.然後我們點開 Project 文件,在Target 列表裏選中 MyFramework,Build Settings
a、Architectures→Architectures 配置支持的指令集,這裏系統已經默認配置了 arm64和 armv7,所以只要增加一個 armv7s 就可以了
以下是指令集對應的設備
armv6 iPhone、iPhone 3G iPod 1G、iPod 2G armv7 iPhone 3GS、iPhone 4 iPod 3G、iPod 4G、iPod 5G iPad、iPad 2、iPad 3、iPad Mini armv7s iPhone 5、iPhone 5C iPad 4 arm64 iPhone 5s iPhone 6iPhone 6P iPhone 6s iPhone 6sP iPhone 7 iPhone 7P iPad Air, Retina iPad Mini
b、Linking→Mach-O Type 將Dynimic Library 改成 Static Library
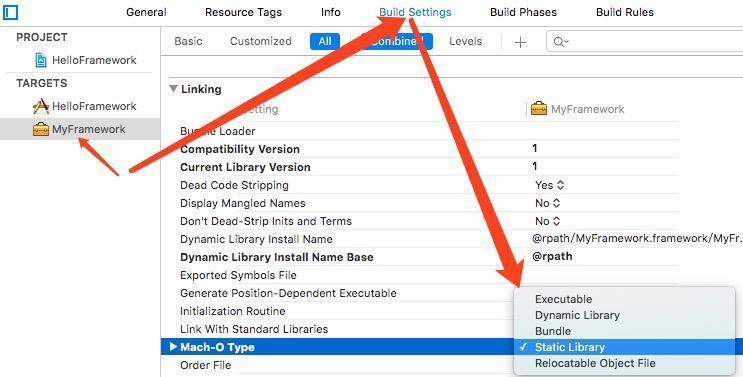
配置 Library 類型
c、Deployment→iOS Deployment Target 選擇你想兼容的最低 iOS 版本
d、新建一個 FrameworkManager,然後再創建好我們要使用的內容,這裏創建一個用 Xib 來顯示的 UIViewController,以及在裏面顯示圖片(用來示例 Xib 和圖片文件的引用)

新建 Framework 文件
我在FrameworkManager類裏實現了一個方法
//.h文件聲明
#import <UIKit/UIKit.h>
#import <Foundation/Foundation.h>
@interface MyFrameworkManager : NSObject
+ (NSString *)showFileVCWithSourceVC:(UIViewController *)VC;
@end
//.m文件實現
#import "MyFrameworkManager.h"
@implementation MyFrameworkManager
+ (NSString *)showFileVCWithSourceVC:(UIViewController *)VC {
return @"Call framework success!!!";
}
@end
e、在Build phase→Headers 裏面將要暴露出去的.h文件拖放到 Public 目錄下(這裏我們開發 MyFrameworkManager)
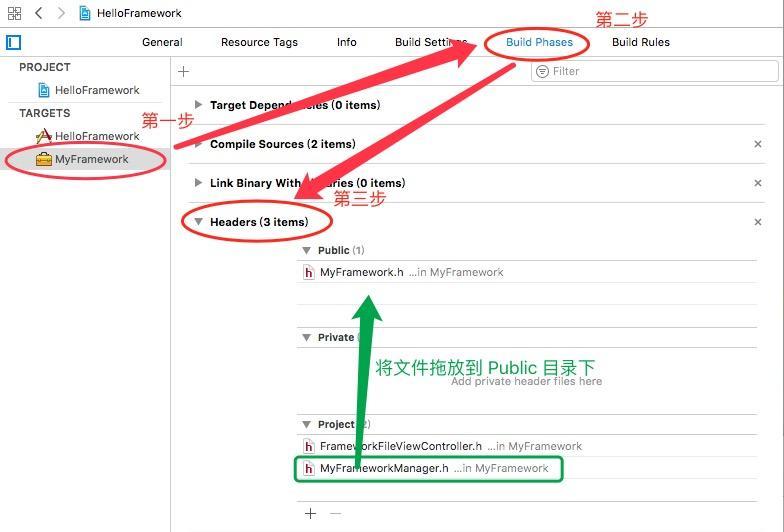
配置開放頭文件
f、現在我們可以在主項目裏引用 MyFrameworkManager,跑跑試試了!我加了一個按鈕和標簽用來演示調用效果!
//主項目 ViewController.m文件
#import "ViewController.h"
@interface ViewController ()
@property (weak, nonatomic) IBOutlet UILabel *callResultLbl;
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
}
- (IBAction)callFramework:(UIButton *)sender {
NSString *results = [MyFrameworkManager showFileVCWithSourceVC:self];
self.callResultLbl.text = results;
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
@end
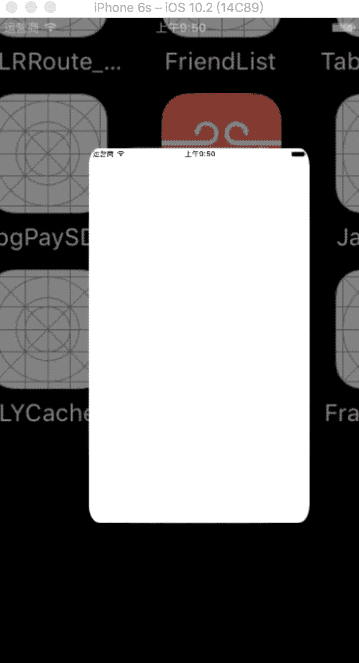
調用 Framework,We did it!
xib 文件和圖片的存放和引用
1.創建bundle,放置資源文件(xib 文件,圖片)
a、新建一個空文件
b、把要用圖片放置進文件
c、編譯 framework
d、在 finder 裏,會找到一個FrameworkFileViewController.nib文件,copy 出來也放到剛新建的文件夾裏(註意:如果 xib 文件有改動,就得重新編譯和替換)
e、然後把文件名改成任何你想要的名字(一般都會與你的 Framework 名字匹配),但是註意,記得更改文件名後綴為 .bundle
f、添加完文件,將該 bundle引用到主工程裏,接下來就可以將 MyFramework 裏的代碼改改,試下 xib 文件和圖片的引用效果了
//MyFrameworkManager.m文件實現
#import "MyFrameworkManager.h"
#import "FrameworkFileViewController.h"
#define MYFRAMEWORK_BUNDLE_IMG(imageName) [@"MyFramework.bundle" stringByAppendingPathComponent:imageName]
#define MYFRAMEWORK_BUNDLE [NSBundle bundleWithPath: [[NSBundle mainBundle] pathForResource:@"MyFramework" ofType: @"bundle"]]
@implementation MyFrameworkManager
+ (NSString *)showFileVCWithSourceVC:(UIViewController *)VC {
FrameworkFileViewController *fileVC = [[FrameworkFileViewController alloc] initWithNibName:@"FrameworkFileViewController" bundle:MYFRAMEWORK_BUNDLE];
[VC presentViewController:fileVC animated:YES completion:^{
}];
return @"Call framework success!!!";
}
@end
//FrameworkFileViewController.m 文件實現
#import "FrameworkFileViewController.h"
@interface FrameworkFileViewController ()
@property (weak, nonatomic) IBOutlet UIImageView *testImage;
@end
@implementation FrameworkFileViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view from its nib.
self.testImage.highlightedImage = [UIImage imageNamed:MYFRAMEWORK_BUNDLE_IMG(@"生日快樂")];
self.testImage.image = [UIImage imageNamed:MYFRAMEWORK_BUNDLE_IMG(@"七夕")];
}
- (IBAction)dismiss:(UIButton *)sender {
[self dismissViewControllerAnimated:YES completion:^{
}];
}
- (IBAction)changImage:(id)sender {
self.testImage.highlighted = !self.testImage.highlighted;
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
@end
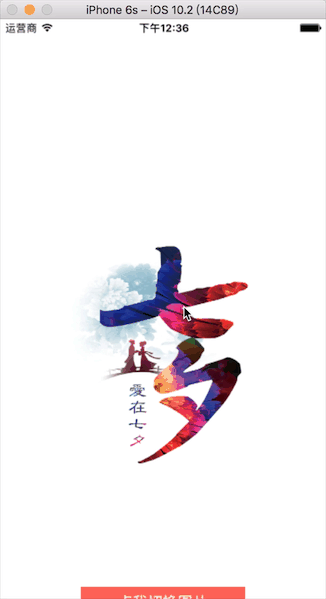
Xib和圖片文件的引用
Pods以及第三方庫的使用
1.pods 庫的引用和調試,同普通的 pods 庫應用,直接 pod init ,在 podfile 裏添加自己要用的庫,pod install 就可以直接在 Framework 裏直接引用使用 pods 庫的東西了
2.第三方庫的使用,這裏以引入支付寶為例
a、在主工程引入 AlipaySDK.framework 和 AlipaySDK.bundle
b、在主工程的 link binary 添加支付寶 sdk 依賴的庫
c、MyFramework 下link binary 添加 AlipaySDK.framework
d、在 FrameworkManager 裏調用 AlipaySDK API,編譯通過,正常使用
Framework 的導出與文檔
首先需要切換到 Release 模式:Product→Edit Scheme→Build Configuration
1.手動導出 Framework
a、在 Target 為 MyFramework 下,選擇模擬器和Generic iOS Device各自 Build 一次
b、在工程目錄 Products 下→右擊 framework→show in finder,會看到有兩個文件夾,一個是真機包,一個是模擬器包。我們要在終端通過 lipo -create將這兩個文件的 MyFramework 文件合成,命令如下:
lipo -create 模擬器包路徑/MyFramework.framework/MyFramework 真機包路徑/MyFramework.framework/MyFramework -output 導出路徑/MyFramework
c、將合成的MyFramework 包替換其中的一個,然後這個 MyFramework.framework就是我們需要的了
2.用 shell 腳本自動導出 Framework
新建一個 Aggregate,添加腳本
a、如果是單 Project
# Sets the target folders and the final framework product.
# 如果工程名稱和Framework的Target名稱不一樣的話,要自定義FMKNAME
FMK_NAME="MyFramework"
#FMK_NAME=${PROJECT_NAME}
# Install dir will be the final output to the framework.
# The following line create it in the root folder of the current project.
INSTALL_DIR=${SRCROOT}/Products/${FMK_NAME}.framework
# Working dir will be deleted after the framework creation.
WRK_DIR=build
DEVICE_DIR=${WRK_DIR}/Release-iphoneos/${FMK_NAME}.framework
SIMULATOR_DIR=${WRK_DIR}/Release-iphonesimulator/${FMK_NAME}.framework
# -configuration ${CONFIGURATION}
# Clean and Building both architectures.
xcodebuild -configuration "Release" -target "${FMK_NAME}" -sdk iphoneos clean build
xcodebuild -configuration "Release" -target "${FMK_NAME}" -sdk iphonesimulator clean build
# Cleaning the oldest.
if [ -d "${INSTALL_DIR}" ]
then
rm -rf "${INSTALL_DIR}"
fi
mkdir -p "${INSTALL_DIR}"
cp -R "${DEVICE_DIR}/" "${INSTALL_DIR}/"
# Uses the Lipo Tool to merge both binary files (i386 + armv6/armv7) into one Universal final product.
lipo -create "${DEVICE_DIR}/${FMK_NAME}" "${SIMULATOR_DIR}/${FMK_NAME}" -output "${INSTALL_DIR}/${FMK_NAME}"
rm -r "${WRK_DIR}"
open "${INSTALL_DIR}"
b、 如果你添加了 Pods 庫或者其他,用的workspace 的話,腳本編譯的命令會有些區別:
(1)編譯的文件路徑WRK_DIR=build這個獲取的路徑就不對了,需要根據自己的路徑靈活配置
(2)xcodebuild不是配置 Target 而是配置workspace 和 scheme,這兩個參數的值可以在終端 cd 當前項目目錄下通過 xcodebuild -list查看,以下是我根據 例子配置了一個
xcodebuild -configuration "Release" -workspace "HelloFramework.xcworkspace" -scheme "${FMK_NAME}" -sdk iphoneos -arch armv7 -arch armv7s -arch arm64 clean build
xcodebuild -configuration "Release" -workspace "HelloFramework.xcworkspace" -scheme "${FMK_NAME}" -sdk iphonesimulator -arch x86_64 -arch i386 clean build
c、配置好了Run Script,就可以選擇 FrameworkMerge 直接 Run 了,然後就會自動打開已經合成好的 MyFramework.framework文件,怎麽樣,是不是特別方便!
d、導出了合成的 framework 文件,就導到其它項目裏試試吧,這裏我就不多加贅述了!
3.最後一步就是編寫文檔了,作為 sdk 的導出,文檔裏你得寫清楚自己 Release 版本和依賴的庫,有 Pods 配置的話也得註明,系統配置,編譯配置等等~More detailed the better!
至此,整個Framework 調試和封裝流程就分享完了!希望路過的大牛不要笑話,也熱烈歡迎大家指正錯誤和不足,或者有更好的方式方法我們一起討論!
行文前我參閱過的文章:
http://www.jianshu.com/p/038dab7accbc
https://gist.github.com/leonardvandriel/3775603
https://developer.apple.com/legacy/library/documentation/Darwin/Reference/ManPages/man1/xcodebuild.1.html
轉載請註明出處,謝謝!
作者:Timmy_Yang
鏈接:http://www.jianshu.com/p/38ed8811c17f
來源:簡書
著作權歸作者所有。商業轉載請聯系作者獲得授權,非商業轉載請註明出處。
轉載-iOS SDK開發