Lua中使用table實現的其它5種數據結構
阿新 • • 發佈:2017-10-27
但是 else func 簡單 value 允許 pan 維數 push
Lua中使用table實現的其它5種數據結構
lua中的table不是一種簡單的數據結構,它可以作為其他數據結構的基礎,如:數組,記錄,鏈表,隊列等都可以用它來表示。
1、數組
在lua中,table的索引可以有很多種表示方式。如果用整數來表示table的索引,即可用table來實現數組,在lua中索引通常都會從1開始。
代碼如下:--二維數組 n=10 m=10 arr={} for i=1,n do arr[i]={} for j=1,m do arr[i][j]=i*n+j end end for i=1, n do forj=1, m do if(j~=m) then io.write(arr[i][j].." ") -- io.write 不會自動換行 else print(arr[i][j]) -- print 自動換行 end end end
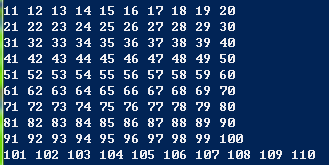
2、鏈表
在lua中,由於table是動態的實體,所以用來表示鏈表是很方便的,其中每個節點都用table來表示。
代碼如下:list = nil for i = 0, 10 do list = {next = list, value = i} end local ls = listwhile ls do print(ls.value) ls = ls.next end
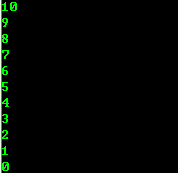
3、隊列與雙端隊列
在lua中實現隊列的簡單方法是調用table中insert和remove函數,但是如果數據量較大的話,效率還是很慢的,下面是手動實現,效率快許多。
代碼如下:List={} function List.new() return {first=0, last=-1} end function List.pushFront(list,value) list.first=list.first-1 list[ list.first ]=value end function List.pushBack(list,value) list.last=list.last+1 list[ list.last ]=value end function List.popFront(list) local first=list.first if first>list.last then error("List is empty!") end local value =list[first] list[first]=nil list.first=first+1 return value end function List.popBack(list) local last=list.last if last<list.first then error("List is empty!") end local value =list[last] list[last]=nil list.last=last-1 return value end lp=List.new() List.pushFront(lp,1) List.pushFront(lp,2) List.pushBack(lp,-1) List.pushBack(lp,-2) x=List.popFront(lp) print(x) x=List.popBack(lp) print(x) x=List.popFront(lp) print(x) x=List.popBack(lp) print(x) x=List.popBack(lp) print(x)
4、集合和包
在Lua中用table實現集合是非常簡單的,見如下代碼:
代碼如下:reserved = { ["while"] = true, ["end"] = true, ["function"] = true, } if not reserved["while"] then --do something end
在Lua中我們可以將包(Bag)看成MultiSet,與普通集合不同的是該容器中允許key相同的元素在容器中多次出現。下面的代碼通過為table中的元素添加計數器的方式來模擬實現該數據結構,如:
代碼如下:
function insert(Bag,element) Bag[element]=(Bag[element] or 0)+1 end function remove(Bag,element) local count=Bag[element] if count >0 then Bag[element]=count-1 else Bag[element]=nil end end
5、StringBuild
如果在lua中將一系列字符串連接成大字符串的話,有下面的方法:
低效率:
代碼如下:local buff="" for line in io.lines() do buff=buff..line.."\n" end
高效率:
代碼如下:
local t={} for line in io.lines() do if(line==nil) then break end t[#t+1]=line end local s=table.concat(t,"\n") --將table t 中的字符串連接起來
Lua中使用table實現的其它5種數據結構