SpringBoot整合Mybatis實現增刪改查的功能
阿新 • • 發佈:2018-05-16
ger 開始 pan ble img 映射 講師 -name date 
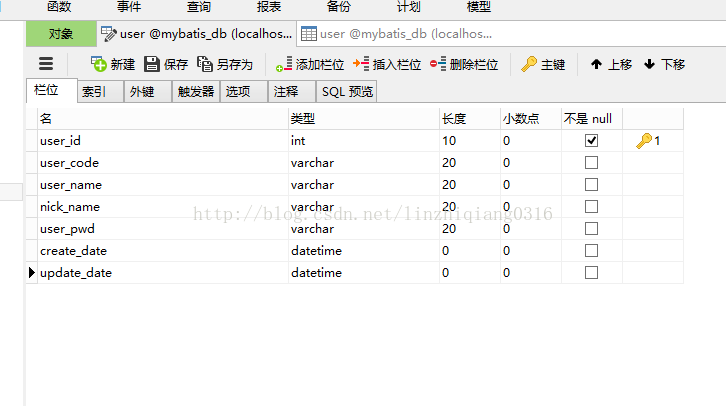
我們可以利用navicat來手動的新建這張表並加相應的數據,也可以通過sql命令來實現,sql命令如下所示: [sql] view plain copy
[java] view plain copy

8.如果想要將執行的sql打印在控制臺上面,可以在application.properties添加如下的配置信息。
logging.level.com.joy=DEBUG
這裏需要特別註意的是:level後面是你項目包的地址不要寫的和我一樣。
到這裏關於SpringBoot整合Mybatis項目就介紹完畢,如果大家想要源代碼的話可以去我的GitHub地址下載。
GitHub地址:https://github.com/1913045515/MyBatis.git 如果按照博客上面寫的步驟操作還有問題,可以觀看我為大家錄制的視頻講解。因為本人不是專業的講師,所以可能講的不是特別細致。有什麽建議都可以提出來,謝謝大家。視頻地址:https://pan.baidu.com/s/1qYcFDow點擊打開鏈接 如果大家對文章有什麽問題或者疑意之類的,可以加我訂閱號在上面留言,訂閱號上面我會定期更新最新博客。如果嫌麻煩可以直接加我wechat:lzqcode
SpringBoot框架作為現在主流框架之一,好多框架都漸漸的移植到SpringBoot中來。前面我給大家介紹過redis,jpa等等的的整合,今天在這裏給大家介紹一下Mybatis的整合過程。
SpringBoot+Mybatis一般有兩種形式。一種是采用原生的xml模式,還有一種就是采用註解模式。今天我給大家介紹的就是後者,也就是註解模式。 1.首選需要在SpringBoot的啟動類裏面增加用來掃描Mapper接口的註解,用來掃描Mapper包下面的接口。 [java] view plain copy
- package com.joy;
- import org.mybatis.spring.annotation.MapperScan;
- import org.springframework.boot.SpringApplication;
- import org.springframework.boot.autoconfigure.SpringBootApplication;
- @SpringBootApplication
- @MapperScan("com.joy.*")
- public class App {
- public static void main(String[] args) {
- SpringApplication.run(App.class, args);
- }
- }
- #DB Configuration:
- spring.datasource.driverClassName = com.mysql.jdbc.Driver
- spring.datasource.url = jdbc:mysql://localhost:3306/mybatis_db?useUnicode=true&characterEncoding=utf-8&useSSL=false
- spring.datasource.username = root
- spring.datasource.password = 220316
- <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
- <modelVersion>4.0.0</modelVersion>
- <groupId>springboot-mybatis</groupId>
- <artifactId>com.joy</artifactId>
- <packaging>war</packaging>
- <version>0.0.1-SNAPSHOT</version>
- <name>com.joy Maven Webapp</name>
- <url>http://maven.apache.org</url>
- <parent>
- <groupId>org.springframework.boot</groupId>
- <artifactId>spring-boot-starter-parent</artifactId>
- <version>1.5.7.RELEASE</version>
- </parent>
- <properties>
- <java.version>1.8</java.version>
- </properties>
- <dependencies>
- <dependency>
- <groupId>org.springframework.boot</groupId>
- <artifactId>spring-boot-starter-web</artifactId>
- </dependency>
- <dependency>
- <groupId>javax.servlet</groupId>
- <artifactId>javax.servlet-api</artifactId>
- </dependency>
- <!-- https://mvnrepository.com/artifact/mysql/mysql-connector-java -->
- <dependency>
- <groupId>mysql</groupId>
- <artifactId>mysql-connector-java</artifactId>
- </dependency>
- <!-- https://mvnrepository.com/artifact/com.github.pagehelper/pagehelper-spring-boot-starter -->
- <dependency>
- <groupId>com.github.pagehelper</groupId>
- <artifactId>pagehelper</artifactId>
- <version>4.1.0</version>
- </dependency>
- <!-- https://mvnrepository.com/artifact/org.mybatis.spring.boot/mybatis-spring-boot-starter -->
- <dependency>
- <groupId>org.mybatis.spring.boot</groupId>
- <artifactId>mybatis-spring-boot-starter</artifactId>
- <version>1.2.2</version>
- </dependency>
- <dependency>
- <groupId>junit</groupId>
- <artifactId>junit</artifactId>
- <scope>test</scope>
- </dependency>
- </dependencies>
- <build>
- <finalName>springboot-mybatis</finalName>
- </build>
- </project>
- package com.joy.entity;
- import java.util.Date;
- public class UserEntity {
- private long userId;
- private String userCode;
- private String userName;
- private String nickName;
- private String userPwd;
- private Date createDate;
- private Date updateDate;
- public long getUserId() {
- return userId;
- }
- public void setUserId(long userId) {
- this.userId = userId;
- }
- public String getUserCode() {
- return userCode;
- }
- public void setUserCode(String userCode) {
- this.userCode = userCode;
- }
- public String getUserName() {
- return userName;
- }
- public void setUserName(String userName) {
- this.userName = userName;
- }
- public String getNickName() {
- return nickName;
- }
- public void setNickName(String nickName) {
- this.nickName = nickName;
- }
- public String getUserPwd() {
- return userPwd;
- }
- public void setUserPwd(String userPwd) {
- this.userPwd = userPwd;
- }
- public Date getCreateDate() {
- return createDate;
- }
- public void setCreateDate(Date createDate) {
- this.createDate = createDate;
- }
- public Date getUpdateDate() {
- return updateDate;
- }
- public void setUpdateDate(Date updateDate) {
- this.updateDate = updateDate;
- }
- }
我們可以利用navicat來手動的新建這張表並加相應的數據,也可以通過sql命令來實現,sql命令如下所示: [sql] view plain copy
- SET FOREIGN_KEY_CHECKS=0;
- -- ----------------------------
- -- Table structure for user
- -- ----------------------------
- DROP TABLE IF EXISTS `user`;
- CREATE TABLE `user` (
- `user_id` int(10) NOT NULL AUTO_INCREMENT,
- `user_code` varchar(20) DEFAULT NULL,
- `user_name` varchar(20) DEFAULT NULL,
- `nick_name` varchar(20) DEFAULT NULL,
- `user_pwd` varchar(20) DEFAULT NULL,
- `create_date` datetime DEFAULT NULL,
- `update_date` datetime DEFAULT NULL,
- PRIMARY KEY (`user_id`)
- ) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=utf8;
- -- ----------------------------
- -- Records of user
- -- ----------------------------
- INSERT INTO `user` VALUES (‘1‘, ‘10001‘, ‘user10001‘, ‘no1‘, ‘123456‘, ‘2017-10-22 15:23:32‘, ‘2017-10-22 15:23:35‘);
- INSERT INTO `user` VALUES (‘2‘, ‘10002‘, ‘user10002‘, ‘no2‘, ‘123456‘, ‘2017-10-22 15:23:32‘, ‘2017-10-22 15:23:35‘);
- @Select("select * from user")
- @Results({
- @Result(property = "userId", column = "user_id"),
- @Result(property = "nickName", column = "nick_name"),
- @Result(property = "userCode", column = "user_code"),
- @Result(property = "userName", column = "user_name"),
- @Result(property = "userPwd", column = "user_pwd"),
- @Result(property = "createDate", column = "create_date"),
- @Result(property = "updateDate", column = "update_date") })
- public List<UserEntity> queryList();
[java] view plain copy
- @Select("SELECT * FROM USER WHERE user_id = #{userId}")
- @Results({
- @Result(property = "userId", column = "user_id"),
- @Result(property = "nickName", column = "nick_name"),
- @Result(property = "userCode", column = "user_code"),
- @Result(property = "userName", column = "user_name"),
- @Result(property = "userPwd", column = "user_pwd"),
- @Result(property = "createDate", column = "create_date"),
- @Result(property = "updateDate", column = "update_date") })
- UserEntity findById(long userId);
- package com.joy.dao;
- import java.util.List;
- import java.util.Map;
- import org.apache.ibatis.annotations.*;
- import com.joy.entity.UserEntity;
- public interface UserMapper {
- @Select("select * from user")
- @Results({
- @Result(property = "userId", column = "user_id"),
- @Result(property = "nickName", column = "nick_name"),
- @Result(property = "userCode", column = "user_code"),
- @Result(property = "userName", column = "user_name"),
- @Result(property = "userPwd", column = "user_pwd"),
- @Result(property = "createDate", column = "create_date"),
- @Result(property = "updateDate", column = "update_date") })
- public List<UserEntity> queryList();
- @Select("SELECT * FROM USER WHERE user_id = #{userId}")
- @Results({
- @Result(property = "userId", column = "user_id"),
- @Result(property = "nickName", column = "nick_name"),
- @Result(property = "userCode", column = "user_code"),
- @Result(property = "userName", column = "user_name"),
- @Result(property = "userPwd", column = "user_pwd"),
- @Result(property = "createDate", column = "create_date"),
- @Result(property = "updateDate", column = "update_date") })
- UserEntity findById(long userId);
- @Insert("INSERT INTO USER(nick_name, user_code) VALUES(#{nickName}, #{userCode})")
- int insertParam(@Param("nickName") String nickName, @Param("userCode") String userCode);
- @Insert("INSERT INTO USER(nick_name, user_code) VALUES(#{nickName,jdbcType=VARCHAR}, #{userCode,jdbcType=INTEGER})")
- int insertByMap(Map<String, Object> map);
- @Insert("insert into user(nick_name,user_code,user_name,user_pwd,create_date,update_date) values(#{nickName},#{userCode},#{userName},#{userPwd},#{createDate},#{updateDate})")
- public int insertEntity(UserEntity entity);
- @Update("UPDATE user SET nick_name=#{nickName} WHERE user_id=#{userId}")
- int updateEntity(UserEntity user);
- @Delete("DELETE FROM user WHERE user_id =#{userId}")
- int delete(Long userId);
- @Delete("DELETE FROM user WHERE user_id =#{userId}")
- int deleteEntity(UserEntity entity);
- }
- package com.joy.service;
- import java.util.Date;
- import java.util.HashMap;
- import java.util.List;
- import java.util.Map;
- import org.springframework.beans.factory.annotation.Autowired;
- import org.springframework.stereotype.Service;
- import com.joy.dao.UserMapper;
- import com.joy.entity.UserEntity;
- @Service
- public class UserService {
- @Autowired(required = false)
- private UserMapper mapper;
- public List<UserEntity> queryList(){
- List<UserEntity> userList=mapper.queryList();
- return userList;
- }
- public UserEntity findById(long userId){
- System.out.println("userId:"+userId);
- return mapper.findById(userId);
- }
- public int insertEntity() {
- UserEntity entity=new UserEntity();
- entity.setUserName("lisi");
- entity.setUserCode("lisi"+new Date());
- entity.setNickName("郭靖");
- entity.setUserPwd("123");
- entity.setCreateDate(new Date());
- entity.setUpdateDate(new Date());
- return mapper.insertEntity(entity);
- }
- public int insertParam() {
- return mapper.insertParam("linzhiqiang","lzq");
- }
- public int insertByMap() {
- Map<String, Object> map=new HashMap<String, Object>();
- map.put("nickName","zhaotong");
- map.put("userCode","zt");
- return mapper.insertByMap(map);
- }
- public int updateEntity() {
- UserEntity entity=new UserEntity();
- entity.setUserId(1);
- entity.setNickName("郭靖");
- return mapper.updateEntity(entity);
- }
- public int deleteEntity() {
- UserEntity entity=new UserEntity();
- entity.setUserId(11);
- return mapper.deleteEntity(entity);
- }
- }
- package com.joy.controller;
- import java.util.List;
- import org.springframework.beans.factory.annotation.Autowired;
- import org.springframework.web.bind.annotation.RequestMapping;
- import org.springframework.web.bind.annotation.RestController;
- import com.github.pagehelper.PageHelper;
- import com.joy.entity.UserEntity;
- import com.joy.service.UserService;
- @RestController
- public class UserController {
- @Autowired
- private UserService userService;
- @RequestMapping("/userlist")
- public List<UserEntity> queryList(){
- PageHelper.startPage(1, 2);
- return userService.queryList();
- }
- @RequestMapping("/queryUser")
- public UserEntity queryUserEntity(long userId){
- UserEntity userEntity=userService.findById(userId);
- return userEntity;
- }
- @RequestMapping("/insert")
- public int insertEntity() {
- return userService.insertEntity();
- }
- @RequestMapping("/insertParam")
- public int insertParam() {
- return userService.insertParam();
- }
- @RequestMapping("/insertByMap")
- public int insertByMap() {
- return userService.insertByMap();
- }
- @RequestMapping("/updateEntity")
- public int updateEntity() {
- return userService.updateEntity();
- }
- @RequestMapping("/deleteEntity")
- public int deleteEntity() {
- return userService.deleteEntity();
- }
- }
GitHub地址:https://github.com/1913045515/MyBatis.git 如果按照博客上面寫的步驟操作還有問題,可以觀看我為大家錄制的視頻講解。因為本人不是專業的講師,所以可能講的不是特別細致。有什麽建議都可以提出來,謝謝大家。視頻地址:https://pan.baidu.com/s/1qYcFDow點擊打開鏈接 如果大家對文章有什麽問題或者疑意之類的,可以加我訂閱號在上面留言,訂閱號上面我會定期更新最新博客。如果嫌麻煩可以直接加我wechat:lzqcode
SpringBoot整合Mybatis實現增刪改查的功能