2018今日頭條春招的一道筆試題 —— 通過改變枚舉的變量進行枚舉優化
阿新 • • 發佈:2018-06-11
圖片 strong uno class stl BE lose ostream erase
代碼如下:
unordered_set::find、unordered_set::erase.....
題目如下:
這道題我們最先想到的做法,應該就是2重循環枚舉數對,然後把數對放在set裏去重,最後輸出set的大小,即輸出set.size( )。
代碼如下:

1 #include<iostream> 2 #include<set> 3 using namespace std; 4 5 int n, k, a[100000]; 6 set<pair<int, int>> mypairs; 7 8 int main() 9 { 10 cin >> n >> k; 11 for(intView Codei = 0; i < n; i ++) 12 cin >> a[i]; 13 for(int i = 0; i < n; i ++) 14 { 15 for(int j = 0; j < n; j ++) 16 { 17 if(a[i] + k == a[j]) 18 mypairs.insert(make_pair(a[i], a[j])); 19 } 20 } 21 cout << mypairs.size() << endl;22 return 0; 23 }
通過使用哈希表進行枚舉優化
優化後的思路:
如果我們只枚舉a[i],比如a[i]=3,那麽如果存在數對的話,這個數對肯定是(a[i], a[i]+k)。比如樣例裏,假設我枚舉數對裏較小的值是3,那根據差是2,較大的肯定是5。所以,問題就變成,查找a[i]+k在不在輸入的數組裏。 該代碼(支持C++11標準): 針對這樣的問題,哈希表是一個非常好用的工具。而且更方便的是,C++的STL已經幫我們把這些工具都實現好了,提供了非常方便的接口,我們直接用行了。下面介紹一下unordered_set,unordered_set可以把它想象成一個集合,它提供了幾個函數讓我們可以增刪查:unordered_set::insert、1 #include<iostream> 2 #include<unordered_set> 3 using namespace std; 4 5 int n, k, x, ans = 0; 6 unordered_set<int> myset; 7 8 int main() 9 { 10 cin >> n >> k; 11 12 for(int i = 0; i < n; i ++) 13 { 14 cin >> x; 15 myset.insert(x); 16 } 17 for(int x : myset) 18 { 19 if(myset.find(x+k) != myset.end()) 20 ans ++; 21 } 22 cout << ans << endl; 23 return 0; 24 }
或者:(編譯器不支持C++11標準的代碼)

1 #include<iostream> 2 #include<set> 3 using namespace std; 4 5 int n, k, x, ans = 0; 6 set<int> myset; 7 8 int main() 9 { 10 cin >> n >> k; 11 12 for(int i = 0; i < n; i ++) 13 { 14 cin >> x; 15 myset.insert(x); 16 } 17 for(set<int> ::iterator i = myset.begin(); i != myset.end(); i ++) 18 { 19 if(myset.find((*i)+k) != myset.end()) 20 ans ++; 21 } 22 cout << ans << endl; 23 return 0; 24 }View Code
運行結果圖如下:
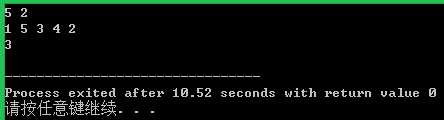
2018今日頭條春招的一道筆試題 —— 通過改變枚舉的變量進行枚舉優化