Spring Boot+BootStrap fileInput 多圖片上傳
阿新 • • 發佈:2018-07-28
lang log pty void 轉存 input 目標 一個 src 一、依賴文件
![技術分享圖片]()
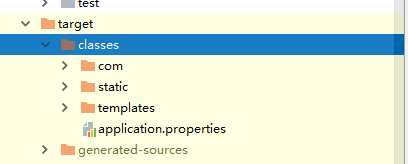
ResourceUtils.getURL("classpath: /static/images/upload/").getPath(); 測試失敗 File uploadFile = new File(path.getAbsolutePath(), "static/images/upload/"); 在開發環境下獲取到的是/target/class/images/upload/ 在將項目打包位war包部署在tomcat之後,/target/class/ --> /WEB-INF/classes/,同理static/images/upload/ --> /WEB-INF/classes/static/images/upload/ 2、fileinput需要有返回參數 參數格式隨意(不要太隨意哈) 3、一下子想不起來了,如果有會繼續更 六、如果有錯的地方,還請指出,謝謝了!
<link rel="stylesheet" type="text/css" th:href="@{/js/bootstrap/css/bootstrap.css}"> <link rel="stylesheet" type="text/css" th:href="@{/js/bootstrap/fileinput/css/fileinput.css}"> <!-- 加入FileInput --> <script th:src="@{/js/jquery-3.3.1.min.js}"></script> <script th:src="@{/js/bootstrap/js/bootstrap.js}"></script> <script th:src="@{/js/bootstrap/fileinput/js/fileinput.js}"></script> <script th:src="@{/js/bootstrap/fileinput/js/zh.js}"></script>/*語言環境*/
二、表單
<form class="form" action="#" method="post" enctype="multipart/form-data" id="pollutionForm"> <!-- 註意事項:Input type類型為file class為樣式 id隨意 name隨意 multiple(如果是要多圖上傳一定要加上,不加的話每次只能選中一張圖)--> 圖片:<input type="file" class="file" id="img" multiple name="images"><br> </form>
三、JavaScript代碼
<script>/**/ var imageData = []; //多圖上傳返回的圖片屬性接受數組 這裏是因為我在做表單其他屬性提交的時候使用,在這裏我沒有將別的input寫出來 $("#img").fileinput({ language : ‘zh‘, uploadUrl : "/image/save-test", showUpload:true, //是否顯示上傳按鈕 showRemove : true, //顯示移除按鈕 showPreview : true, //是否顯示預覽 showCaption: false,//是否顯示標題 autoReplace : true, minFileCount: 0, uploadAsync: true, maxFileCount: 10,//最大上傳數量 browseOnZoneClick: true, msgFilesTooMany: "選擇上傳的文件數量 超過允許的最大數值!", enctype: ‘multipart/form-data‘, // overwriteInitial: false,//不覆蓋已上傳的圖片 allowedFileExtensions : [ "jpg", "png", "gif" ], browseClass : "btn btn-primary", //按鈕樣式 previewFileIcon : "<i class=‘glyphicon glyphicon-king‘></i>" }).on("fileuploaded", function(e, data) {//文件上傳成功的回調函數,還有其他的一些回調函數,比如上傳之前... var res = data.response; console.log(res) imageData.push({ "path": res.data.path, "date":res.data.date }); console.log(imageData); }); </script>
四、後臺代碼
//圖片類 import java.util.Date; /** * 圖片類 */ public class Img { private String name; private String path; private Date date; public Img() { } public Img(String path, Date date) { this.path = path; this.date = date; } public Img(String name, String path, Date date) { this.name = name; this.path = path; this.date = date; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getPath() { return path; } public void setPath(String path) { this.path = path; } public Date getDate() { return date; } public void setDate(Date date) { this.date = date; } @Override public String toString() { return "Img{" + "name=‘" + name + ‘\‘‘ + ", path=‘" + path + ‘\‘‘ + ", date=" + date + ‘}‘; } } //圖片上傳Controller public class UploadController { //fileinput 其實每次只會上傳一個文件 多圖上傳也是分多次請求,每次上傳一個文件 所以不需要循環 @RestController //@RequestParam("images") 這裏的images對應表單中name 然後MultipartFile 參數名就可以任意了 @RequestMapping(value = "/image/save-test",method = RequestMethod.POST) public UploadResponseData saveImg(@RequestParam("images") MultipartFile file) throws IOException { String pathname = ""; String returnPath = ""; if (!file.isEmpty()){ String fileName = file.getOriginalFilename(); File path = new File(ResourceUtils.getURL("classpath:").getPath());//獲取Spring boot項目的根路徑,在開發時獲取到的是/target/classes/ System.out.println(path.getPath());//如果你的圖片存儲路徑在static下,可以參考下面的寫法 File uploadFile = new File(path.getAbsolutePath(), "static/images/upload/");//開發測試模式中 獲取到的是/target/classes/static/images/upload/ if (!uploadFile.exists()){ uploadFile.mkdirs(); } //獲取文件後綴名 String end = FilenameUtils.getExtension(file.getOriginalFilename()); DateFormat df = new SimpleDateFormat("yyyyMMddHHmmssSSS"); //圖片名稱 采取時間拼接隨機數 String name = df.format(new Date()); Random r = new Random(); for(int i = 0 ;i < 3 ;i++){ name += r.nextInt(10); } String diskFileName = name + "." +end; //目標文件的文件名 pathname = uploadFile.getPath()+ "/" + diskFileName; System.out.println(pathname); returnPath = "images/upload/" + diskFileName;//這裏是我自己做返回的字符串 file.transferTo(new File(pathname));//文件轉存 }//UploadResponseData 自定義返回數據類型實體{int code, String msg, Object data} return new UploadResponseData(CodeEnum.SUCCESS.getCode(),MsgEnum.SUCCESS.getMsg(),new Img(returnPath,new Date())); } }
五、總結吧
最後在這裏想說一些問題
1、spring boot路徑獲取問題:
ResourceUtils.getURL("classpath:").getPath(); 在開發環境下獲取到的是項目根路徑/target/class/
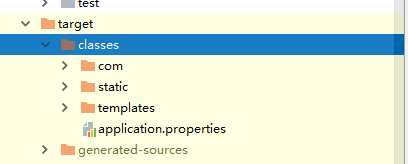
ResourceUtils.getURL("classpath: /static/images/upload/").getPath(); 測試失敗 File uploadFile = new File(path.getAbsolutePath(), "static/images/upload/"); 在開發環境下獲取到的是/target/class/images/upload/ 在將項目打包位war包部署在tomcat之後,/target/class/ --> /WEB-INF/classes/,同理static/images/upload/ --> /WEB-INF/classes/static/images/upload/ 2、fileinput需要有返回參數 參數格式隨意(不要太隨意哈) 3、一下子想不起來了,如果有會繼續更 六、如果有錯的地方,還請指出,謝謝了!
Spring Boot+BootStrap fileInput 多圖片上傳