SpringBoot開發案例之整合mongoDB
一、說明
- Spring boot是對Spring的進一步封裝,旨在簡化Spring的安裝和配置過程。我們知道使用Spring搭建專案環境,往往需要引用很多jar包,並隨著業務的逐漸複雜,創建出很多的xml檔案。Spring boot封裝了Spring整合的很多服務元件,並自動建立這些物件的例項,你只用將所需使用的服務元件的jar包引入即可快速構建開發環境。
- Spring boot所整合的服務元件,可在官網找到,你可以勾選所使用的服務元件,並把相應maven 專案下載到本地。
- Spring boot同樣集成了對MongoDB等Nosql的支援,下面介紹通過Spring boot連線和操作MongoDB。
二、開發環境
- JDK1.8、Maven、iDEA、SpringBoot2.0.6.RELEASE、mongodb3.4,Robomongo(視覺化工具)
三、建立Spring boot專案
本文使用的IDE是idea,其Spring initializr是建立Spring Boot專案的快速視覺化元件,當然你也可以構建maven專案,然後在Spring boot官網將相關pom引入。
3.1 建立一個工程或Module,選擇Spring Initializr,選擇jdk版本
3.2 填寫工程或模板資訊
3.3 選擇nosql中的mongodb
3.4 建立
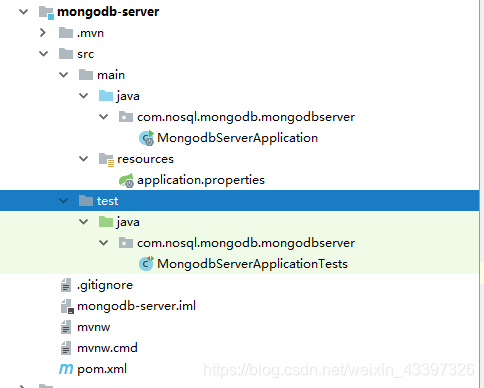
四、案例實戰
4.1 目錄結構
4.2 pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</ modelVersion>
<groupId>com.nosql.mongodb</groupId>
<artifactId>mongodb-server</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>mongodb-server</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.6.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
4.3 實體類 Users.java
package com.nosql.mongodb.mongodbserver.model;
import java.io.Serializable;
import org.springframework.data.annotation.Transient;
import org.springframework.data.mongodb.core.index.CompoundIndex;
import org.springframework.data.mongodb.core.index.CompoundIndexes;
import org.springframework.data.mongodb.core.index.Indexed;
import org.springframework.data.mongodb.core.mapping.Document;
@Document(collection="users")
@CompoundIndexes({
@CompoundIndex(name = "age_idx", def = "{'name': 1, 'age': -1}")
})
public class Users implements Serializable{
private static final long serialVersionUID = 1L;
@Indexed
private String uid;
private String name;
private int age;
@Transient
private String address;
public Users(String uid, String name, int age) {
super();
this.uid = uid;
this.name = name;
this.age = age;
}
public String getUid() {
return uid;
}
public void setUid(String uid) {
this.uid = uid;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
@Override
public String toString() {
return String.format(
"Customer[name='%s', age='%s']",
name, age);
}
}
4.4 業務介面 IUserService.java
package com.nosql.mongodb.mongodbserver.service;
import com.nosql.mongodb.mongodbserver.model.Users;
import java.util.List;
/**
* mongodb 案例
*
*/
public interface IUserService {
public void saveUser(Users users);
public Users findUserByName(String name);
public void removeUser(String name);
public void updateUser(String name, String key, String value);
public List<Users> listUser();
}
4.5 業務實現UserServiceImpl.java
package com.nosql.mongodb.mongodbserver.service.impl;
import java.util.List;
import com.nosql.mongodb.mongodbserver.model.Users;
import com.nosql.mongodb.mongodbserver.service.IUserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.mongodb.core.MongoOperations;
import org.springframework.data.mongodb.core.query.Criteria;
import org.springframework.data.mongodb.core.query.Query;
import org.springframework.data.mongodb.core.query.Update;
import org.springframework.stereotype.Component;
@Component("userService")
public class UserServiceImpl implements IUserService {
@Autowired
MongoOperations mongoTemplate;
public void saveUser(Users users) {
mongoTemplate.save(users);
}
public Users findUserByName(String name) {
return mongoTemplate.findOne(
new Query(Criteria.where("name").is(name)), Users.class);
}
public void removeUser(String name) {
mongoTemplate.remove(new Query(Criteria.where("name").is(name)),
Users.class);
}
public void updateUser(String name, String key, String value) {
mongoTemplate.updateFirst(new Query(Criteria.where("name").is(name)),
Update.update(key, value), Users.class);
}
public List<Users> listUser() {
return mongoTemplate.findAll(Users.class);
}
}
4.6 啟動類Application.java
import org.apache.log4j.Logger;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.context.annotation.ComponentScan;
@EnableAutoConfiguration
@ComponentScan(basePackages={"com.itstyle.mongodb"})
public class Application {
private static final Logger logger = Logger.getLogger(Application.class);
public static void main(String[] args) throws InterruptedException {
SpringApplication.run(Application.class, args);
logger.info("專案啟動 ");
}
}
4.7 基礎配置application.properties
# 專案contextPath,一般在正式釋出版本中
server.context-path=/mongodb
# 服務埠
server.port=8080
# session最大超時時間(分鐘),預設為30
server.session-timeout=60
# 該服務繫結IP地址,啟動伺服器時如本機不是該IP地址則丟擲異常啟動失敗,只有特殊需求的情況下才配置
# server.address=192.168.16.11
# tomcat最大執行緒數,預設為200
server.tomcat.max-threads=800
# tomcat的URI編碼
server.tomcat.uri-encoding=UTF-8
#mongo2.x支援以上兩種配置方式 mongo3.x僅支援uri方式
#mongodb note:mongo3.x will not use host and port,only use uri
#spring.data.mongodb.host=192.168.1.180
#spring.data.mongodb.port=27017
#spring.data.mongodb.database=itstyle
#沒有設定密碼
spring.data.mongodb.uri=mongodb://127.0.0.1:27017/My_Mongodb
#設定了密碼
#spring.data.mongodb.uri=mongodb://itstyle:[email protected]:27017/My_Mongodb
4.8測試類SpringbootMongodbApplication.java
package com.nosql.mongodb.mongodbserver;
import java.util.List;
import com.nosql.mongodb.mongodbserver.model.Users;
import com.nosql.mongodb.mongodbserver.service.IUserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.ComponentScan;
@SpringBootApplication
@ComponentScan(basePackages={"com.nosql.mongodb.mongodbserver"})
public class SpringbootMongodbApplication implements CommandLineRunner {
@Autowired
private IUserService userService;
public static void main(String[] args) {
SpringApplication.run(SpringbootMongodbApplication.class, args);
}
@Override
public void run(String... args) throws Exception {
try {
Users users = new Users("1", "小明", 10);
users.setAddress("青島市");
userService.saveUser(users);
List<Users> list = userService.listUser();
System.out.println("一共這麼多人:"+list.size());
} catch (Exception e) {
e.printStackTrace();
}
}
}
4.9 執行測試類
4.10 Robomongo檢視
五、註解說明
@Document 標註在實體類上,與hibernate異曲同工。
@Document(collection="users")
public class Users implements Serializable{
private static final long serialVersionUID = 1L;
...省略程式碼
@CompoundIndex 複合索引,加複合索引後通過複合索引欄位查詢將大大提高速度。
@Document(collection="users")
@CompoundIndexes({
@CompoundIndex(name = "age_idx", def = "{'name': 1, 'age': -1}")
})
public class Users implements Serializable{
private static final long serialVersionUID = 1L;
...省略程式碼
@Id MongoDB預設會為每個document生成一個 _id 屬性,作為預設主鍵,且預設值為ObjectId,可以更改 _id 的值(可為空字串),但每個document必須擁有 _id 屬性。 當然,也可以自己設定@Id主鍵,不過官方建議使用MongoDB自動生成。 @Indexed 宣告該欄位需要加索引,加索引後以該欄位為條件檢索將大大提高速度。 唯一索引的話是@Indexed(unique = true)。 也可以對陣列進行索引,如果被索引的列是陣列時,mongodb會索引這個陣列中的每一個元素。
@Indexed
private String uid;
@Transient 被該註解標註的,將不會被錄入到資料庫中。只作為普通的javaBean屬性。
@Transient
private String address;
@Field 代表一個欄位,可以不加,不加的話預設以引數名為列名。
@Field("firstName")
private String name;