【達內課程】Android中的動畫(下)
阿新 • • 發佈:2018-12-26
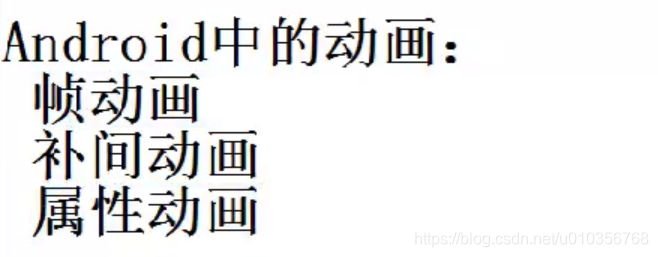

幀動畫
在drawable下新建一個fragme.xml檔案
<?xml version="1.0" encoding="utf-8"?> <animation-list xmlns:android="http://schemas.android.com/apk/res/android"> <item android:drawable="@mipmap/wifi0" android:duration="200"/> <item android:drawable="@mipmap/wifi1" android:duration="200"/> <item android:drawable="@mipmap/wifi2" android:duration="200"/> <item android:drawable="@mipmap/wifi3" android:duration="200"/> <item android:drawable="@mipmap/wifi4" android:duration="200"/> <item android:drawable="@mipmap/wifi5" android:duration="200"/> </animation-list>
activity_main.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" > <Button android:id="@+id/btn" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="動起來"/> <TextView android:id="@+id/tv" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="@drawable/frame" /> </LinearLayout>
MainActivity
public class MainActivity extends Activity implements View.OnClickListener{ private Button button; private TextView tv; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); button = findViewById(R.id.btn); tv = findViewById(R.id.tv); button.setOnClickListener(this); } @Override public void onClick(View view) { //使用配置檔案定義幀動畫的執行序列 //載入配置檔案,執行幀動畫 AnimationDrawable animationDrawable = (AnimationDrawable) tv.getBackground(); animationDrawable.start(); } }
只執行一次
animationDrawable.setOneShot(true);
動態新增幀
AnimationDrawable animationDrawable = (AnimationDrawable) tv.getBackground();
animationDrawable.addFrame(getResources().getDrawable(R.mipmap.wifi5),200);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap.wifi4),200);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap.wifi3),200);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap.wifi2),200);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap.wifi1),200);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap.wifi0),200);
animationDrawable.start();
純程式碼新增幀動畫
如果事先不給TextView新增background,現在用純程式碼新增
AnimationDrawable animationDrawable = new AnimationDrawable();
animationDrawable.addFrame(getResources().getDrawable(R.mipmap.wifi5),200);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap.wifi4),200);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap.wifi3),200);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap.wifi2),200);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap.wifi1),200);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap.wifi0),200);
tv.setBackground(animationDrawable);
animationDrawable.start();
補間動畫
TranslateAnimation animation = new TranslateAnimation(0,0,0,150);
animation.setDuration(1000);
view.startAnimation(animation);
TranslateAnimation animation = new TranslateAnimation(0,0,0,150);
animation.setDuration(1000);
animation.setFillAfter(true);
view.startAnimation(animation);
補間動畫只是在視覺上的一個動畫展示,控制元件的屬性並沒有變化
例如這個button,起始座標是(0,0),經過這個平移動畫,屬性依舊是(0,0),只是視覺上移動到了(0,150)
如果我們再點選button原來的位置,它還會再執行一遍動畫
屬性動畫
ObjectAnimator animator = ObjectAnimator.ofFloat(img,"alpha",1,0);
animator.setDuration(1000);
animator.start();
ObjectAnimator animator = ObjectAnimator.ofFloat(img, "alpha", 1, 0);
animator.setDuration(1000);
//常見屬性設定
//設定迴圈次數,設定為INFINITE表示無限迴圈
animator.setRepeatCount(3);
//設定迴圈模式
//value取值有RESTART,REVERSE,
animator.setRepeatMode(ObjectAnimator.RESTART);
animator.start();
其中alph是屬性,小寫,程式會自動大寫首字母,並且執行setAlph方法
讓我們盡情搖擺的程式碼:
ObjectAnimator animator = ObjectAnimator.ofFloat(img, "x", 0, 100);
animator.setDuration(1000);
animator.setRepeatCount(ObjectAnimator.INFINITE);
animator.setRepeatMode(ObjectAnimator.REVERSE);
animator.start();
ObjectAnimator animator = ObjectAnimator.ofFloat(img, "abc", 1, 0);
animator.setDuration(1000);
animator.setRepeatCount(ObjectAnimator.INFINITE);
animator.setRepeatMode(ObjectAnimator.REVERSE);
//給ObjectAnimator設定屬性更新
//每時每刻改view的屬性
animator.addUpdateListener(new ValueAnimator.AnimatorUpdateListener(){
@Override
public void onAnimationUpdate(ValueAnimator valueAnimator) {
//獲取更新時,ObjectAnimator計算出來的引數值
Float value = (Float) valueAnimator.getAnimatedValue();
img.setScaleX(value);
img.setScaleY(value);
}
});
animator.start();
其實預設情況下動畫是由慢變快再變慢的過程,下一個動畫效果是勻速變化的
......
//勻速變化
animator.setInterpolator(new LinearInterpolator());
animator.start();
ObjectAnimator animator = ObjectAnimator.ofFloat(view,"x",0,300);
animator.setDuration(3000);
animator.setInterpolator(new BounceInterpolator());
animator.start();