Unleash the power of Slack App
1. Create NodeJS server
Create a new NodeJS project by following these steps:
Update package.json with the following content. This step is important for the app to work well after it is deployed in Heroku cloud.
Create src/index.js
:
Configure firebase & Slack client
Create src/firebase.js
— a utility module to help read and write to firebase real-time database.
And update src/config.js


And take slack configuration from “Basic Information” tab of your application’s settings.
2. Configure Slack OAuth
Slack uses OAuth 2.0 protocol to let your app request authorization to private details in a user’s Slack account without getting their password. This authorization is mandatory for the app to post messages and receive interaction requests from the users. The authorization happens when Slack apps are installed on a team.


If the user authorizes your app, Slack will redirect the user back to your specified redirect_uri
with a temporary code in a code
GET parameter. Exchange the authorization code for an access token using the API method (method documentation) and securely store this information for future purpose.
You can then use this token to call
Start building the API for your Slack app by adding an end point to handle OAuth request. Create src/slack-api.js
and add the following content:
3. Publish app to Heroku
You may choose to publish your app to any public cloud offering. I am going to use Heroku. Start this by adding files to git. Don’t forget to exclude node_modules
from git by adding it to .gitignore
. Commit changes.
Now create an application using the account you created at the beginning. Following the instructions at https://dashboard.heroku.com/new-app
Important Note: The name of your app may change based on availability of name in Heroku. Make sure to replace idea-slack-app
everywhere with a name you choose here.
Run the following commands to deploy the app to Heroku
Once the app is successfully deployed, you must be able to access:https://idea-slack-app.herokuapp.com
Slack needs a callback URL to redirect users after a successful login and this is configured in your app’s settings under > your app > OAuth & Permission
. The callback URL is going to point to the app we just deployed in Heroku.

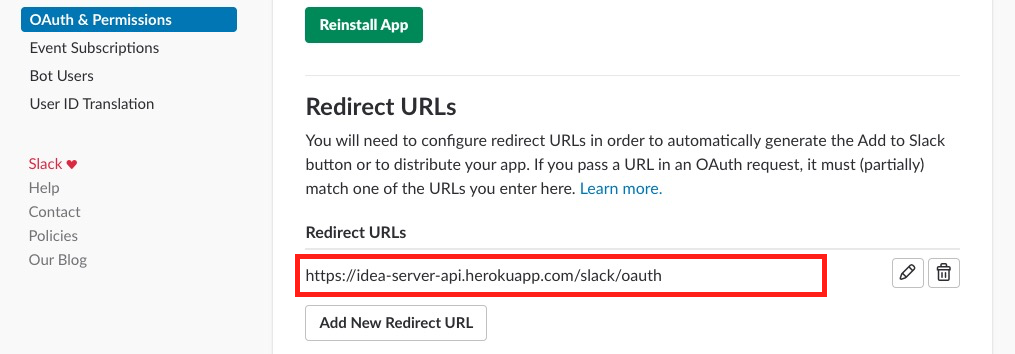
4. Create slash command /share
Slash Commands let users trigger an interaction with your app directly from the message box in Slack. A submitted Slash Command will cause a payload of data to be sent from Slack to an app, allowing the app to respond in whatever way it wants. These commands are the starting point for complex workflows.


Add /share command:


First, head to your app’s settings page, and then click the Slash Commands feature in the navigation menu.
You’ll be presented with a button marked Create New Command, and when you click on it, you’ll see a screen where you’ll be asked to define your new Slash Command:
- Command — the name of command, the actual string that users will type to trigger a world of magic. Bear in mind the naming advice below when you pick this.
- Request URL — the URL we’ll send the data payload to, when the command is invoked by a user. You’ll want to use a URL that you can setup to receive these payloads.
Configure Request URL for the slash commands as:


When user enter /share command, Slack will send a POST request to this URL. Your app should respond with an instruction to show a dialog like this.


Add following function to slack-api.js
to do this.
The form elements are defined in lines between 12 and 31 in the above snippet. Once the message object is created, send it to /dialog.open
API. Provide access_code
, that you saved in step 2, as the token
parameter to the API. Use slack
— a small utility function to send this instruction to Slack.
Did you notice callback_id
? We will use this in the next step.
5. Handle “Share” Button
When user submits the form, Slack will send another POST request to . Use callback_id
from previous step to identify the request — in this case it is /submit_idea
.
Once the input is validated, app gets the preview of the Article URL and this is saved to Firebase real-time database — our storage. When everything is successful upto this point, app sends the idea to the channel with a like a button attached to the message — We will discuss about shareIdea
in the next section.
6. Handle “Like” (up vote)


Use interactive messages, to build rich interactions like this. Crafting messages with buttons is one way to do that. Buttons can be attached to a message — when clicked, Slack will trigger a POST request to the predefined URL. This URL can be configured from app’s setting under Interactive Components
.

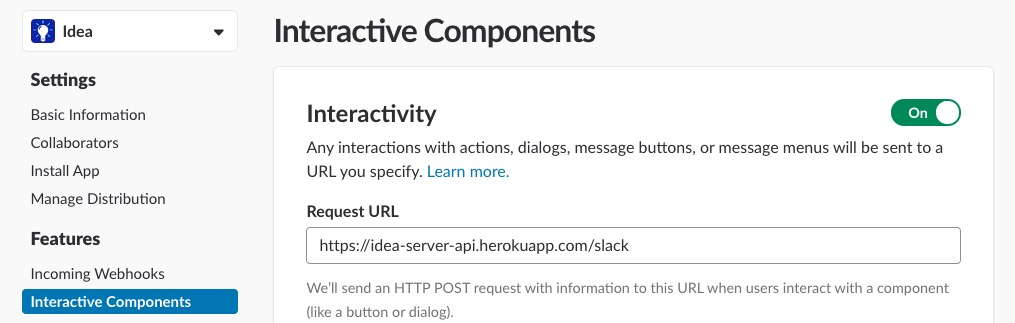
There are two stages to using buttons. First attach button to the message using actions
property under attachments
of a Slack message.
This will show a message in the channel like one shown below:


In the second stage, handle the request posted by Slack when user clicks “like” button. Add the following code to src/slack-api.js
to do that.
Few important things to note: You must identify the request using callback_id
and match it with the value used while sending the message. The app must immediately return 200 OK (line 16) to Slack to avoid a timeout message appearing in Slack channel. And finally the app must send the updated message back to response_url
(a param made available to the API through request.body.payload
). Slack will replace the old message with the new one.
Finally publish all the changes to upto this point to Heroku, by running: