利用SharedPreferences做的自動登入的小Demo
阿新 • • 發佈:2018-12-31
效果圖:
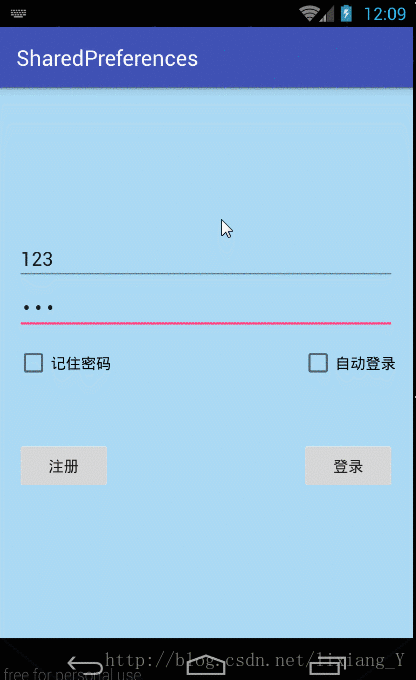
Other.java
activity_auto_login.xml
程式碼如下:
AutoLogin.java
package com.zhiyuan3g.sharedpreferences;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.text.TextUtils;
import android.view.View;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.EditText;
import android.widget.Toast;
public class AutoLogin extends AppCompatActivity implements View.OnClickListener {
private EditText editText3;
private EditText editText4;
private CheckBox checkBox;
private CheckBox checkBox2;
private Button button3;
private Button button4;
private boolean remenberNum = false;
private boolean autoLogin = false;
private SharedPreferences mSharedPreferences;
private String mEditText3;
private String mEditText4;
private SharedPreferences.Editor mEdit;
private String mPassword;
private String mUsernaem;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_auto_login);
initView();
}
private void initView() {
editText3 = (EditText) findViewById(R.id.editText3);
editText4 = (EditText) findViewById(R.id.editText4);
checkBox = (CheckBox) findViewById(R.id.checkBox);
checkBox2 = (CheckBox) findViewById(R.id.checkBox2);
button3 = (Button) findViewById(R.id.button3);
button4 = (Button) findViewById(R.id.button4);
button3.setOnClickListener(this);
button4.setOnClickListener(this);
//得到全域性的SharedPreferences物件,因為後面要用很多,所以直接定義成全域性變數
mSharedPreferences = getSharedPreferences("zhiyuan", MODE_PRIVATE);
//得到全域性的SharedPreferences的edit物件,因為後面要用很多,所以直接定義成全域性變數
mEdit = mSharedPreferences.edit();
//通過SharedPreferences物件獲取檔案中鍵名為remenberNumde、autoLogin的兩個布林值。
//這兩個值是我們儲存的是記住密碼和自動登入的兩個狀態值
boolean remenberNum1 = mSharedPreferences.getBoolean("remenberNum", false);
boolean autoLogin1 = mSharedPreferences.getBoolean("autoLogin", false);
//獲取我們以前存入的使用者名稱和密碼
mUsernaem = mSharedPreferences.getString("username", "");
mPassword = mSharedPreferences.getString("password", "");
//判斷記住密碼的狀態值是否為true,true則進入if裡面,否則進入else裡面
if (remenberNum1) {
//將我們獲取的使用者名稱和密碼資料設定給相應的EditText
editText3.setText(mUsernaem);
editText4.setText(mPassword);
//設定第一個checkBox為選中狀態(即記住密碼的checkBox),作用是還原以前記住密碼的狀態
checkBox.setChecked(true);
//再判斷自動登入的狀態值是否為true,true則進入if裡面
if (autoLogin1) {
//還原自動登入的checkBox的狀態
checkBox2.setChecked(true);
//彈吐司提示自動登入成功
Toast.makeText(getApplicationContext(), "自動登入成功", Toast.LENGTH_SHORT).show();
//通過intent意圖,跳轉到指定登入成功的頁面
Intent intent = new Intent(getApplicationContext(), Other.class);
startActivity(intent);
}
} else {
//再判斷自動登入的狀態值是否為true,true則進入if裡面
if (autoLogin1) {
//還原記住密碼的checkBox的狀態
checkBox.setChecked(true);
//還原自動登入的checkBox的狀態
checkBox2.setChecked(true);
//將我們獲取的使用者名稱和密碼資料設定給相應的EditText
editText3.setText(mUsernaem);
editText4.setText(mPassword);
//彈吐司提示自動登入成功
Toast.makeText(getApplicationContext(), "自動登入成功", Toast.LENGTH_SHORT).show();
//通過intent意圖,跳轉到指定登入成功的頁面
Intent intent = new Intent(getApplicationContext(), Other.class);
startActivity(intent);
}
}
}
/**
* function:按鈕的點選監聽
* @param v
*/
@Override
public void onClick(View v) {
//在此處獲取EditText輸入框類的文字,即使用者名稱和密碼
// 切忌不可在初始化內獲取,我們需要通過按鈕監聽實時獲得文字值
mEditText3 = editText3.getText().toString();
mEditText4 = editText4.getText().toString();
//對字串的非空判斷
if (submit()) {
switch (v.getId()) {
//當點選註冊按鈕時,進入button3的監聽處理
case R.id.button3:
//首先先把輸入文字的使用者名稱和密碼通過SharedPreferences的edit物件存入
mEdit.putString("username", mEditText3);
mEdit.putString("password", mEditText4);
//切忌,不要忘記提交,不提交無效
mEdit.commit();
//彈吐司提示註冊成功
Toast.makeText(getApplicationContext(), "恭喜,註冊成功", Toast.LENGTH_SHORT).show();
break;
//當點選登入按鈕時,進入button4的監聽處理
case R.id.button4:
//首先先把使用者名稱和密碼通過SharedPreferences物件獲取出來
mUsernaem = mSharedPreferences.getString("username", "");
mPassword = mSharedPreferences.getString("password", "");
//判斷此時輸入框EditText中的內容是否與我們獲取出來的使用者名稱和密碼相同,相同進入if裡面
if (mUsernaem.equals(mEditText3) && mPassword.equals(mEditText4)) {
//彈吐司提示登入成功
Toast.makeText(getApplicationContext(), "登入成功", Toast.LENGTH_SHORT).show();
//判斷記住密碼和自動登入的checkBox是否被勾選
if (checkBox.isChecked() && !checkBox2.isChecked()) {
//將記住密碼的狀態設定為true
remenberNum = true;
//將自動登入的狀態設定為false,以下類推,就不一一寫了
autoLogin = false;
} else if (!checkBox.isChecked() && !checkBox2.isChecked()) {
remenberNum = false;
autoLogin = false;
} else if (!checkBox.isChecked() && checkBox2.isChecked()) {
remenberNum = false;
autoLogin = true;
} else if (checkBox.isChecked() && checkBox2.isChecked()) {
remenberNum = true;
autoLogin = true;
}
//將我們剛剛得到的記住密碼和自動登入的狀態儲存下來
mEdit.putBoolean("remenberNum", remenberNum);
mEdit.putBoolean("autoLogin", autoLogin);
//同樣是需要提交的
mEdit.commit();
//通過intent意圖,跳轉到指定登入成功的頁面
startActivity(new Intent(this, Other.class));
} else {
//使用者名稱或密碼不正確時,吐司提示登入失敗
Toast.makeText(getApplicationContext(), "登入失敗", Toast.LENGTH_SHORT).show();
}
break;
}
}
}
/**
* function:對EditText輸入框非空判斷
* @return
*/
private boolean submit() {
// validate
String editText3String = editText3.getText().toString().trim();
if (TextUtils.isEmpty(editText3String)) {
Toast.makeText(this, "請輸入使用者名稱", Toast.LENGTH_SHORT).show();
return false;
}
String editText4String = editText4.getText().toString().trim();
if (TextUtils.isEmpty(editText4String)) {
Toast.makeText(this, "請輸入密碼", Toast.LENGTH_SHORT).show();
return false;
}
return true;
}
}
Other.java
package com.zhiyuan3g.sharedpreferences;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
public class Other extends AppCompatActivity implements View.OnClickListener {
private Button back_btn;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_other);
initView();
}
private void initView() {
back_btn = (Button) findViewById(R.id.back_btn);
back_btn.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.back_btn:
SharedPreferences zhiyuan = getSharedPreferences("zhiyuan", MODE_PRIVATE);
zhiyuan.edit().putBoolean("autoLogin", false).commit();
startActivity(new Intent(this,AutoLogin.class));
break;
}
}
}
activity_auto_login.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ABDAF5"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.zhiyuan3g.sharedpreferences.AutoLogin">
<EditText
android:layout_marginTop="120dp"
android:singleLine="true"
android:hint="請輸入使用者名稱"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/editText3"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:layout_alignParentRight="true"
android:layout_alignParentEnd="true"/>
<EditText
android:singleLine="true"
android:hint="請輸入密碼"
android:inputType="textPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/editText4"
android:layout_below="@+id/editText3"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:layout_alignRight="@+id/editText3"
android:layout_alignEnd="@+id/editText3"/>
<CheckBox
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="記住密碼"
android:id="@+id/checkBox"
android:layout_centerVertical="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:checked="false"/>
<CheckBox
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="自動登入"
android:id="@+id/checkBox2"
android:layout_alignTop="@+id/checkBox"
android:layout_alignRight="@+id/editText4"
android:layout_alignEnd="@+id/editText4"
android:checked="false"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="註冊"
android:id="@+id/button3"
android:layout_alignParentBottom="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:layout_marginBottom="120dp"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="登入"
android:id="@+id/button4"
android:layout_alignTop="@+id/button3"
android:layout_alignRight="@+id/checkBox2"
android:layout_alignEnd="@+id/checkBox2"/>
</RelativeLayout>
activity_other.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.zhiyuan3g.sharedpreferences.Other">
<TextView
android:layout_width="wrap_content"
android:gravity="center"
android:textSize="50dp"
android:text="登入成功"
android:layout_height="wrap_content"/>
<Button
android:layout_marginTop="100dp"
android:text="退出登入"
android:id="@+id/back_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</RelativeLayout>