Spring 筆記 -04- 依賴注入例項 - 實現印表機功能(maven)
Spring 筆記 -04- 依賴注入例項 - 實現印表機功能(maven)
前面的文章是基礎,如果是剛開始學習,最好依次檢視:Spring 筆記
你可能會感覺這是把一個簡單的問題弄複雜了,
不要慌,你的錯覺沒有錯,
這裡主要是學習 Spring 的依賴注入,
很重要!
問題描述:
- 如何實現一個印表機?
分析:
- 印表機功能的實現依賴於 墨盒 和 紙張
步驟:
- (1)定義墨盒和紙張的介面標準
- (2)使用介面標準開發印表機
- (3)組裝印表機
- (4)執行印表機
一、Spring 建立專案&編碼流程
(提示:專案中檔名,建議一致)
(1)建立 Maven 專案,參考 Spring 筆記 -01- JUnit 單元測試
(2)還是一樣先展示全部目錄,避免大家不知道檔案在哪
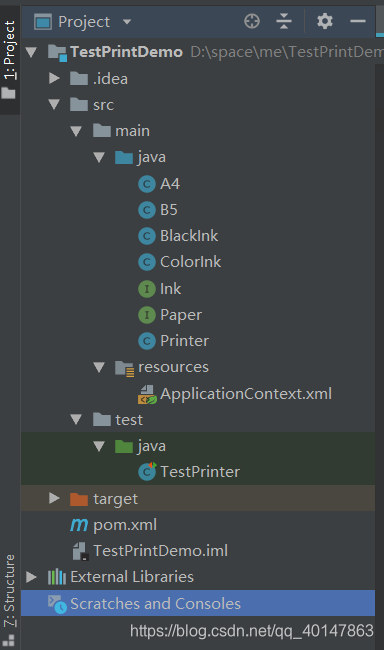
(3)配置 pom.xml 檔案,注意位置,下面有提示:
<dependencies>
<!-- https://mvnrepository.com/artifact/org.springframework/spring-context -->
<dependency>
<groupId>org.springframework< /groupId>
<artifactId>spring-context</artifactId>
<version>5.1.3.RELEASE</version>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>RELEASE</version>
<scope>test</scope>
</dependency>
</dependencies>
位置:
(4)選擇自動匯入:
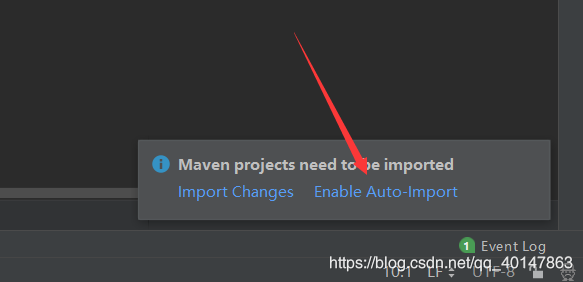
(5)新建墨盒的介面,Ink.java (首大寫的 ink)檔案,編寫程式碼:
public interface Ink {
//Ink 介面中定義了一個方法
String getColor(int r,int g,int b);
}
(6)Ink 介面的實現一:建立 BlackInk.java 檔案(表示黑白列印),原始碼:
public class BlackInk implements Ink {
//在獲取該列印狀態的顏色時,只返回黑白
//目的是學習 Spring,不必糾結
public String getColor(int r, int g, int b) {
return "黑白";
}
}
(7)Ink 介面的實現二:建立 ColorInk.java 檔案(表示彩色列印),原始碼:
public class ColorInk implements Ink{
public String getColor(int r, int g, int b) {
return "彩色";
}
}
(8)
新建 Paper.java 介面,編寫程式碼:
public interface Paper {
//Paper 介面中有一個換行常量
public final String NEWLINE = "\n";
//Paper 介面中定義了一個抽象方法
public abstract void putChar(char ch);
String getContent();
}
(9)Paper 介面的實現一:建立 A4.java 檔案(表示A4紙張),原始碼:
// A4 是對 Paper 介面的一個實現
public class A4 implements Paper {
//A4 中定義了一個,每行多少字元的屬性
private int lineChar;
//用於獲取行文字數上限值
public int getLineChar() {
return lineChar;
}
//用於設定行文字數上限值
public void setLineChar(int lineChar) {
this.lineChar = lineChar;
}
//用於設定列印的內容
public void setContent(String content) {
this.content = content;
}
//初始化內容
private String content = "";
//定義公有方法,用於將內容實現一行多少字的控制
public void putChar(char ch) {
content += String.valueOf(ch);
if (content.length() % lineChar == 0) {
content += Paper.NEWLINE;
}
}
//用於獲取列印的內容
public String getContent() {
return content;
}
}
(10)Paper 介面的實現二:建立 B5.java 檔案(表示B5紙張),這裡內容和上述 A4 內容一致,但是例項化時的每行字數是不一致的,原始碼:
// B5 是對 Paper 介面的一個實現
public class B5 implements Paper {
//A4 中定義了一個,每行多少字元的屬性
private int lineChar;
//用於獲取行文字數上限值
public int getLineChar() {
return lineChar;
}
//用於設定行文字數上限值
public void setLineChar(int lineChar) {
this.lineChar = lineChar;
}
//用於設定列印的內容
public void setContent(String content) {
this.content = content;
}
//初始化內容
private String content = "";
//定義公有方法,用於將內容實現一行多少字的控制
public void putChar(char ch) {
content += String.valueOf(ch);
if (content.length() % lineChar == 0) {
content += Paper.NEWLINE;
}
}
//用於獲取列印的內容
public String getContent() {
return content;
}
}
(11)定義執行列印功能的類,建立 Printer.java 檔案,原始碼:
//執行列印
public class Printer {
//初始化墨盒和紙張的內容為空
private Ink ink = null;
private Paper paper = null;
//用於獲取這裡的墨盒的具體值
public Ink getInk() {
return ink;
}
//用於設定墨盒的具體值
public void setInk(Ink ink) {
this.ink = ink;
}
//用於獲取這裡的紙張的具體值
public Paper getPaper() {
return paper;
}
//用於獲取這裡的墨盒的具體值
public void setPaper(Paper paper) {
this.paper = paper;
}
//開始列印啦
public void print(String str) {
//假裝給墨盒傳一個 RGB 值
System.out.println("正在使用" + ink.getColor(255, 0, 0) + "字型進行列印");
try {
for (int i = 0; i < str.length(); i++) {
//根據字數,每一個字都判斷是否換行
paper.putChar(str.charAt(i));
//Thread.sleep(100);
}
//列印內容
System.out.println(paper.getContent());
} catch (Exception e){
//處理異常
e.printStackTrace();
}
}
}
二、建立及配置 Spring 的配置檔案
(1)在 resources 目錄下建立 ApplicationContext.xml 檔案,截圖:
(2)在 ApplicationContext.xml 檔案中進行配置:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<!--Spring 相當於一個物件池,這裡存放所有例項,屬性賦值等操作,開始可能不習慣,-->
<!--但這是Spring 一大特點-->
<!--一個 a4 物件,lineChar 屬性為 20,及每 20 個字換行-->
<bean id="a4" class="A4">
<property name="lineChar" value="20"></property>
</bean>
<!--一個 b5 物件,lineChar 屬性為 15,及每 15 個字換行-->
<bean id="b5" class="B5">
<property name="lineChar" value="15"></property>
</bean>
<!--黑白墨盒-->
<bean id="blackInk" class="BlackInk"></bean>
<!--彩色墨盒-->
<bean id="colorInk" class="ColorInk"></bean>
<!--開始列印-->
<bean id="printer" class="Printer">
<!--先使用 a4 紙, 彩色印表機-->
<property name="paper" ref="a4"></property>
<property name="ink" ref="colorInk"></property>
</bean>
</beans>
三、新建測試類
(1)在 test/java 目錄下,建立 TestPrinter.java 檔案,原始碼:
//單元測試的包
import org.junit.jupiter.api.Test;
//需要先配置 context,前面在 pom.xml 檔案中的配置
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class TestPrinter {
@Test
public void testPrinter(){
//用 ApplicationContext 做測試的話,獲得 Spring 中定義的 Bean 例項(物件)
ClassPathXmlApplicationContext ac = new ClassPathXmlApplicationContext("ApplicationContext.xml");
//得到 ApplicationContext.xml 檔案中的,id 為 printer
Printer printer = (Printer) ac.getBean("printer");
//列印的內容
String printStr = "shdfhskjhgkljsfhgksjhalkjghsgsd";
//開始列印
printer.print(printStr);
}
}
四、執行測試類
- 在TestPrinter.java 檔案,右鍵執行:
更多文章連結:
支援博主
我正在參加 CSDN 2018 年部落格之星評選,希望大家能支援我,
我是【No. 001】號 肖朋偉 ,感謝大家寶貴的一票 ^_^/
投票地址:https://bss.csdn.net/m/topic/blog_star2018/index