Thymeleaf的普通表單提交與AJAX提交
阿新 • • 發佈:2019-01-10
為Java實體物件新增後臺校驗註解:
//String型別的校驗: @NotEmpty -- 不能為空 max=16 -- 最大長度為16
@NotEmpty(message = "songName不能為空")
@Size(max = 16 , message = "songName長度不能超過16")
private String songName;
@NotEmpty(message = "singer不能為空")
@Size(max = 12 , message = "singer長度不能超過12")
private String singer;
//int型別的校驗: @NotNull -- 不能為空 min=1 max=127 -- 值在1~127之間
@Range(min = 1, max = 127, message = "age的範圍在1~127")
@NotNull(message = "age不能為空")
private Integer age;
一般這些校驗都不會用到,因為更多的時候我們都是在前端進行校驗的。這裡只是進行示範。
普通表單的提交與SpringMVC沒多大區別,前端程式碼如下(insertSong.html):
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="utf-8">
<title>insert Song</title>
</head>
<body>
<br><br>
<div th:text="${hintMessage}">資訊提示!!!!</div>
<br>
<div th:errors="${song.songName}"></div>
<div th:errors="${song.singer}"></div>
<br>
<form id ="iform" th:action="@{/song/save.action}" th:method="post" th:object="${song}">
<table border="1">
<tr>
<th>歌曲名</th>
<th>演唱者</th>
<th>Action</th>
</tr>
<tr>
<!--th:field="*{songName}" 加上這個屬性時報錯-->
<td><input type="text" name="songName"/></td>
<td><input type="text" name="singer"/></td>
<td><input type="submit" value="新增"/></td>
</tr>
</table>
</form>
<hr>
</body>
</html>
後臺程式碼:
@PostMapping("/song/save.action")
public ModelAndView insertSong(@Valid Song song, BindingResult result){
//@Valid註解啟動後臺校驗,
ModelAndView modelAndView = new ModelAndView();
System.out.println("歌手名稱:"+ song.getSinger());
if(result.hasErrors()){
modelAndView.addObject("hintMessage", "出錯啦!");
}else{
String songName = song.getSongName();
Song dataSong = songService.findSongByName(songName);
if(dataSong != null){
modelAndView.addObject("hintMessage", "資料庫已有該條記錄!");
}else{
modelAndView.addObject("hintMessage", "提交成功!");
songService.insertSong(song);
}
}
modelAndView.setViewName("/success");
return modelAndView;
}
當然,要有一個方法跳轉到指定的頁面:
@GetMapping("/song/add")
public ModelAndView addSong(){
ModelAndView modelAndView = new ModelAndView();
Song song = new Song(0, null, null);
modelAndView.addObject("song", song);
modelAndView.addObject("hintMessage", "初始化成功!");
modelAndView.setViewName("/insertSong");
return modelAndView;
}
因為insertSong.html定義瞭如下程式碼:
<div th:text="${hintMessage}">資訊提示!!!!</div>
<div th:errors="${song.songName}"></div>
<div th:errors="${song.singer}"></div>
所以要進行初始化,否則會報錯:
Song song = new Song(0, null, null);
modelAndView.addObject("song", song);
modelAndView.addObject("hintMessage", "初始化成功!");
如果不要後臺校驗,就跟平常的SpringMVC程式碼一樣,只需要將url改成thymeleaf格式的就行了(th:action="@{/song/save.action}"),這裡就不進行演示了。
下面進行Thymeleaf的ajax提交的演示:
Thymeleaf中使用javaS基本格式為:
<script type="text/javascript" th:inline="javascript">
/*<![CDATA[*/
....(這裡寫js程式碼)
/*]]>*/
</script>
前端程式碼如下(ajaxTest.html):
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Thymeleaf Ajax Test</title>
<script src="../static/jquery/jquery-1.7.2.js" type="text/javascript" th:src="@{/jquery/jquery-1.7.2.js}"></script>
<script type="text/javascript" th:inline="javascript">
/*<![CDATA[*/
function ajaxSubmit(){
var songName = $("#songName").val();
var singer = $("#singer").val();
$.ajax({
url: [[@{/song/ajaxTest.action}]],
type: 'post',
dataType: 'json',
contentType: 'application/json',
data: JSON.stringify({songName : songName,singer : singer}),
async: true,
success: function(data){
if(data != null){
alert('flag : ' + data.flag + " , hintMessage : " + data.hintMessage);
}
}
});
}
/*]]>*/
</script>
</head>
<body>
<input type="text" name="songName" id="songName">
<input type="text" name="singer" id="singer">
<button onclick="ajaxSubmit()">提交</button>
</body>
</html>
要注意的地方就是ajax的url的格式:url: [[@{/song/ajaxTest.action}]],將url包含在[[url]]中。
後臺程式碼,與普通的SpringMVC基本沒有區別:
@GetMapping("/song/ajax")
public String toAjax(){
return "/ajaxTest";
}
@PostMapping("/song/ajaxTest.action")
public void ajaxTest(@RequestBody Song song, HttpServletResponse resp)throws Exception{
String songName = song.getSongName();
Song dataSong = songService.findSongByName(songName);
String flagMessage = "success";
String hintMessage="資料新增成功!!!";
if(dataSong != null){
flagMessage = "fail";
hintMessage = "資料庫已有該資料!!!";
}else{
songService.insertSong(song);
}
JSONObject jsonObject = new JSONObject();
jsonObject.put("flag",flagMessage);
jsonObject.put("hintMessage",hintMessage);
System.out.println(jsonObject.toJSONString());
resp.setContentType("text/html;charset=UTF-8");
resp.getWriter().println(jsonObject.toJSONString());
resp.getWriter().close();
}
目錄結構如下:
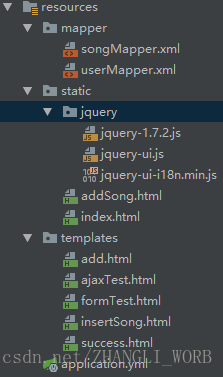
下面是幾個需要注意的地方:
1.如果希望方法返回值是String時是跳轉到指定頁面(xxx.html),Controller層應該使用@Controller註解,而不是@RestController
2.springboot預設的目錄是/resources/static,所以在使用具體的目錄時只需要以staic的下一個子目錄開始即可。如:/resources/static/jquery/jquery-1.7.2.js,在使用Thymeleaf表示路徑地址時的格式: th:src="@{/jquery/jquery-1.7.2.js}"
3.如果直接通過url就可以訪問到index.html,只需要把index.html放在static目錄下即可,即:/static/index.html
如果大家對Thymeleaf的配置不太清楚的話,可以看看我的上一篇部落格(SpringCloud與Thymeleaf整合demo),Thymeleaf的表單提交的實現基本就是這樣,希望對大家有所幫助。
同時發現了一個問題: 當我使用 th:field 這個屬性時,Thymeleaf直接拋異常,暫時也沒找到解決方法,如果有知道什麼原因的朋友求告知。最近在鬥魚上看到一個唱歌很好聽的音樂主播,鬥魚id:賣血哥,房間號:109064,各位小夥伴可以關注下!!!