javaweb匯出Excel資料與圖片
簡介
Apache POI 是用Java編寫的免費開源的跨平臺的 Java API,Apache POI提供API給Java程式對Microsoft Office格式檔案讀和寫的功能。POI為“Poor Obfuscation Implementation”的首字母縮寫,意為“可憐的模糊實現”。
Apache POI 是建立和維護操作各種符合Office Open XML(OOXML)標準和微軟的OLE 2複合文件格式(OLE2)的Java API。用它可以使用Java讀取和建立,修改MS Excel檔案.而且,還可以使用Java讀取和建立MS Word和MSPowerPoint檔案。Apache POI 提供Java操作Excel解決方案。詳情見百度百科:
apache poi百度百科
實現匯出excel兩種方式:1、JExcel 2、apache poi
區別:(1)JExcel是不支援xlsx格式,POI支援xls格式也支援xlsx格式。
(2)apache poi基於流的處理資料,適合大檔案要求記憶體更少。.
我是用的apache poi
需要的jar包
(1)poi-3.13-20150929.jar--------我的是3.13版本,官方下載地址http://poi.apache.org/download.html
(2)commons-codec-1.4.jar----上面的已包含
(3)commons-io-1.4.jar-----apache提供的IO包,很好用,官方下載地 址http://commons.apache.org/proper/commons-io/download_io.cgi
java程式碼
(1)先定義一個實體類
<span style="font-size:18px;">package com.sram.bean; import java.awt.image.BufferedImage; /** * * @類名: User * @描述: 將使用者資訊和圖片匯出到excel * @作者: chenchaoyun0 * @日期: 2016-1-28 下午10:06:23 * */ public class User { private int id; private String user_name; private String user_headSrc;//使用者頭像的路徑 private BufferedImage user_headImg;//使用者輸出頭像到excel的圖片緩衝 public User() { super(); } public User(int id, String user_name, String user_headSrc) { super(); this.id = id; this.user_name = user_name; this.user_headSrc = user_headSrc; } @Override public String toString() { return "User [id=" + id + ", user_name=" + user_name + ", user_headSrc=" + user_headSrc + ", user_headImg=" + user_headImg + "]"; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getUser_name() { return user_name; } public void setUser_name(String user_name) { this.user_name = user_name; } public String getUser_headSrc() { return user_headSrc; } public void setUser_headSrc(String user_headSrc) { this.user_headSrc = user_headSrc; } public BufferedImage getUser_headImg() { return user_headImg; } public void setUser_headImg(BufferedImage user_headImg) { this.user_headImg = user_headImg; } }</span>
(2)excel匯出的工具類
(3)設定excel的類package com.sram.util; import java.io.File; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import org.apache.poi.hssf.usermodel.HSSFCell; import org.apache.poi.hssf.usermodel.HSSFCellStyle; import org.apache.poi.hssf.usermodel.HSSFFont; import org.apache.poi.hssf.usermodel.HSSFRichTextString; import org.apache.poi.hssf.usermodel.HSSFRow; import org.apache.poi.hssf.usermodel.HSSFSheet; import org.apache.poi.hssf.usermodel.HSSFWorkbook; import org.apache.poi.hssf.util.HSSFColor; import org.apache.poi.hssf.util.Region; /** * * @類名: ExportExcel * @描述: excel工具類 * @作者: chenchaoyun0 * @日期: 2016-1-28 下午10:28:18 * */ public class ExportExcel { private HSSFWorkbook wb = null; private HSSFSheet sheet = null; /** * * <p> * 標題: * </p> * <p> * 描述: * </p> * * @param wb * @param sheet */ public ExportExcel(HSSFWorkbook wb, HSSFSheet sheet) { // super(); this.wb = wb; this.sheet = sheet; } /** * * @標題: createNormalHead * @作者: chenchaoyun0 * @描述: TODO(這裡用一句話描述這個方法的作用) * @引數: @param headString 頭部的字元 * @引數: @param colSum 報表的列數 * @返回: void 返回型別 * @丟擲: */ @SuppressWarnings("deprecation") public void createNormalHead(String headString, int colSum) { HSSFRow row = sheet.createRow(0); // 設定第一行 HSSFCell cell = row.createCell(0); // row.setHeight((short) 1000); // 定義單元格為字串型別 cell.setCellType(HSSFCell.ENCODING_UTF_16);// 中文處理 cell.setCellValue(new HSSFRichTextString(headString)); // 指定合併區域 /** * public Region(int rowFrom, short colFrom, int rowTo, short colTo) */ sheet.addMergedRegion(new Region(0, (short) 0, 0, (short) colSum)); // 定義單元格格式,新增單元格表樣式,並新增到工作簿 HSSFCellStyle cellStyle = wb.createCellStyle(); // 設定單元格水平對齊型別 cellStyle.setAlignment(HSSFCellStyle.ALIGN_CENTER); // 指定單元格居中對齊 cellStyle.setVerticalAlignment(HSSFCellStyle.VERTICAL_CENTER);// 指定單元格垂直居中對齊 cellStyle.setWrapText(true);// 指定單元格自動換行 // 設定單元格字型 HSSFFont font = wb.createFont(); font.setBoldweight(HSSFFont.BOLDWEIGHT_BOLD); font.setFontName("宋體"); font.setFontHeight((short) 600); cellStyle.setFont(font); cell.setCellStyle(cellStyle); } /** * * @標題: createNormalTwoRow * @作者: chenchaoyun0 * @描述: 建立通用報表第二行 * @引數: @param params 二行統計條件陣列 * @引數: @param colSum 需要合併到的列索引 * @返回: void 返回型別 * @丟擲: */ @SuppressWarnings("deprecation") public void createNormalTwoRow(String[] params, int colSum) { // 建立第二行 HSSFRow row1 = sheet.createRow(1); row1.setHeight((short) 400); HSSFCell cell2 = row1.createCell(0); cell2.setCellType(HSSFCell.ENCODING_UTF_16); cell2.setCellValue(new HSSFRichTextString("時間:" + params[0] + "至" + params[1])); // 指定合併區域 sheet.addMergedRegion(new Region(1, (short) 0, 1, (short) colSum)); HSSFCellStyle cellStyle = wb.createCellStyle(); cellStyle.setAlignment(HSSFCellStyle.ALIGN_CENTER); // 指定單元格居中對齊 cellStyle.setVerticalAlignment(HSSFCellStyle.VERTICAL_CENTER);// 指定單元格垂直居中對齊 cellStyle.setWrapText(true);// 指定單元格自動換行 // 設定單元格字型 HSSFFont font = wb.createFont(); font.setBoldweight(HSSFFont.BOLDWEIGHT_BOLD); font.setFontName("宋體"); font.setFontHeight((short) 250); cellStyle.setFont(font); cell2.setCellStyle(cellStyle); } /** * * @標題: createColumHeader * @作者: chenchaoyun0 * @描述: 設定報表標題 * @引數: @param columHeader 標題字串陣列 * @返回: void 返回型別 * @丟擲: */ public void createColumHeader(String[] columHeader) { // 設定列頭 在第三行 HSSFRow row2 = sheet.createRow(2); // 指定行高 row2.setHeight((short) 600); HSSFCellStyle cellStyle = wb.createCellStyle(); cellStyle.setAlignment(HSSFCellStyle.ALIGN_CENTER); // 指定單元格居中對齊 cellStyle.setVerticalAlignment(HSSFCellStyle.VERTICAL_CENTER);// 指定單元格垂直居中對齊 cellStyle.setWrapText(true);// 指定單元格自動換行 // 單元格字型 HSSFFont font = wb.createFont(); font.setBoldweight(HSSFFont.BOLDWEIGHT_BOLD); font.setFontName("宋體"); font.setFontHeight((short) 250); cellStyle.setFont(font); // 設定單元格背景色 cellStyle.setFillForegroundColor(HSSFColor.GREY_25_PERCENT.index); cellStyle.setFillPattern(HSSFCellStyle.SOLID_FOREGROUND); HSSFCell cell3 = null; for (int i = 0; i < columHeader.length; i++) { cell3 = row2.createCell(i); cell3.setCellType(HSSFCell.ENCODING_UTF_16); cell3.setCellStyle(cellStyle); cell3.setCellValue(new HSSFRichTextString(columHeader[i])); } } /** * * @標題: cteateCell * @作者: chenchaoyun0 * @描述: 建立內容單元格 * @引數: @param wb HSSFWorkbook * @引數: @param row HSSFRow * @引數: @param col short型的列索引 * @引數: @param align 對齊方式 * @引數: @param val 列 * @返回: void 返回型別 * @丟擲: */ public void cteateCell(HSSFWorkbook wb, HSSFRow row, int col, short align, String val) { HSSFCell cell = row.createCell(col); cell.setCellType(HSSFCell.ENCODING_UTF_16); cell.setCellValue(new HSSFRichTextString(val)); HSSFCellStyle cellstyle = wb.createCellStyle(); cellstyle.setAlignment(align); cell.setCellStyle(cellstyle); } /** * * @標題: createLastSumRow * @作者: chenchaoyun0 * @描述: 建立合計行 * @引數: @param colSum 需要合併到的列索引 * @引數: @param cellValue 設定檔案 * @返回: void 返回型別 * @丟擲: */ @SuppressWarnings("deprecation") public void createLastSumRow(int colSum, String[] cellValue) { HSSFCellStyle cellStyle = wb.createCellStyle(); cellStyle.setAlignment(HSSFCellStyle.ALIGN_CENTER); // 指定單元格居中對齊 cellStyle.setVerticalAlignment(HSSFCellStyle.VERTICAL_CENTER);// 指定單元格垂直居中對齊 cellStyle.setWrapText(true);// 指定單元格自動換行 // 單元格字型 HSSFFont font = wb.createFont(); font.setBoldweight(HSSFFont.BOLDWEIGHT_BOLD); font.setFontName("宋體"); font.setFontHeight((short) 250); cellStyle.setFont(font); // 獲取工作表最後一行 HSSFRow lastRow = sheet.createRow((short) (sheet.getLastRowNum() + 1)); HSSFCell sumCell = lastRow.createCell(0); sumCell.setCellValue(new HSSFRichTextString("合計")); sumCell.setCellStyle(cellStyle); // 合併 最後一行的第零列-最後一行的第一列 sheet.addMergedRegion(new Region(sheet.getLastRowNum(), (short) 0, sheet.getLastRowNum(), (short) colSum));// 指定合併區域 for (int i = 2; i < (cellValue.length + 2); i++) { // 定義最後一行的第三列 sumCell = lastRow.createCell(i); sumCell.setCellStyle(cellStyle); // 定義陣列 從0開始。 sumCell.setCellValue(new HSSFRichTextString(cellValue[i - 2])); } } /** * * @標題: outputExcel * @作者: chenchaoyun0 * @描述: 輸出excel下載 * @引數: @param fileName 檔名 * @返回: void 返回型別 * @丟擲: */ public void outputExcel(String fileName) { FileOutputStream fos = null; try { fos = new FileOutputStream(new File(fileName)); wb.write(fos); fos.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } /** * * @返回: HSSFSheet 返回型別 */ public HSSFSheet getSheet() { return sheet; } public void setSheet(HSSFSheet sheet) { this.sheet = sheet; } /** * * @返回: HSSFWorkbook 返回型別 */ public HSSFWorkbook getWb() { return wb; } /** * */ public void setWb(HSSFWorkbook wb) { this.wb = wb; } }
package com.sram.util; import java.awt.image.BufferedImage; import java.io.BufferedOutputStream; import java.io.ByteArrayOutputStream; import java.io.IOException; import java.io.OutputStream; import java.util.List; import javax.imageio.ImageIO; import javax.servlet.http.HttpServletResponse; import org.apache.poi.hssf.usermodel.HSSFCell; import org.apache.poi.hssf.usermodel.HSSFCellStyle; import org.apache.poi.hssf.usermodel.HSSFClientAnchor; import org.apache.poi.hssf.usermodel.HSSFFont; import org.apache.poi.hssf.usermodel.HSSFPatriarch; import org.apache.poi.hssf.usermodel.HSSFRichTextString; import org.apache.poi.hssf.usermodel.HSSFRow; import org.apache.poi.hssf.usermodel.HSSFSheet; import org.apache.poi.hssf.usermodel.HSSFWorkbook; import com.sram.bean.User; /** * * @類名: ExportToExcelUtil * @描述: 設定excel'的資訊 * @作者: chenchaoyun0 * @日期: 2016-1-28 下午11:19:58 * */ public class ExportToExcelUtil { public static void out(HttpServletResponse response, List<User> list) throws Exception { /** * 指定下載名 */ String fileName = "使用者資料.xls"; /** * 編碼 */ fileName = new String(fileName.getBytes("GBK"), "iso8859-1"); /** * 去除空白行 */ response.reset(); /** * 指定下載的檔名與設定相應頭 */ response.setHeader("Content-Disposition", "attachment;filename=" + fileName); /** * 下載檔案型別 */ response.setContentType("application/vnd.ms-excel"); /** * 別給我快取 */ response.setHeader("Pragma", "no-cache"); response.setHeader("Cache-Control", "no-cache"); response.setDateHeader("Expires", 0); /** * 得到輸出流,放到緩衝區 */ OutputStream output = response.getOutputStream(); BufferedOutputStream bufferedOutPut = new BufferedOutputStream(output); /** * 下面是匯出的excel格式的設定 */ /*******************我是分割線**************************************/ // 定義單元格報頭 String worksheetTitle = "這是ccy的測試"; HSSFWorkbook wb = new HSSFWorkbook(); // 建立單元格樣式 HSSFCellStyle cellStyleTitle = wb.createCellStyle(); // 指定單元格居中對齊 cellStyleTitle.setAlignment(HSSFCellStyle.ALIGN_CENTER); // 指定單元格垂直居中對齊 cellStyleTitle.setVerticalAlignment(HSSFCellStyle.VERTICAL_CENTER); // 指定當單元格內容顯示不下時自動換行 cellStyleTitle.setWrapText(true); HSSFCellStyle cellStyle = wb.createCellStyle(); // 指定單元格居中對齊 cellStyle.setAlignment(HSSFCellStyle.ALIGN_CENTER); // 指定單元格垂直居中對齊 cellStyle.setVerticalAlignment(HSSFCellStyle.VERTICAL_CENTER); // 指定當單元格內容顯示不下時自動換行 cellStyle.setWrapText(true); // 設定單元格字型 HSSFFont font = wb.createFont(); font.setBoldweight(HSSFFont.BOLDWEIGHT_BOLD); font.setFontName("宋體"); font.setFontHeight((short) 200); cellStyleTitle.setFont(font); /****************wo是分割線*****************************************/ /** * 工作表列名 */ String id = "id"; String name = "使用者名稱"; String img = "頭像"; HSSFSheet sheet = wb.createSheet(); ExportExcel exportExcel = new ExportExcel(wb, sheet); /** * 建立報表頭部 */ exportExcel.createNormalHead(worksheetTitle, 6); // 定義第一行 HSSFRow row1 = sheet.createRow(1); HSSFCell cell1 = row1.createCell(0); /** * 第一行第一列 */ cell1.setCellStyle(cellStyleTitle); cell1.setCellValue(new HSSFRichTextString(id)); /** * 第一行第二列 */ cell1 = row1.createCell(1); cell1.setCellStyle(cellStyleTitle); cell1.setCellValue(new HSSFRichTextString(name)); /** * 第一行第三列 */ cell1 = row1.createCell(2); cell1.setCellStyle(cellStyleTitle); cell1.setCellValue(new HSSFRichTextString(img)); /********************我是分割線*********************************/ /** * 定義第二行,就是我們的資料 */ HSSFRow row = sheet.createRow(2); HSSFCell cell = row.createCell(1); /** * 便利我們傳進來的user-list */ User user =null; HSSFPatriarch patriarch = sheet.createDrawingPatriarch(); for (int i = 0; i < list.size(); i++) { user = list.get(i); /** * 從i+2行開始,因為我們之前的表的標題和列的標題已經佔用了兩行 */ row = sheet.createRow(i + 2); //...id cell = row.createCell(0); cell.setCellStyle(cellStyle); cell.setCellValue(new HSSFRichTextString(user.getId() + "")); //....user_name cell = row.createCell(1); cell.setCellStyle(cellStyle); cell.setCellValue(new HSSFRichTextString("\n"+user.getUser_name()+"\n")); //...user_headImg short h =2;//定義圖片所在行 short l=2;//定義圖片所在列 cell = row.createCell(2); cell.setCellStyle(cellStyle); //得到user的圖片 BufferedImage image = user.getUser_headImg(); ByteArrayOutputStream byteArrayOut = new ByteArrayOutputStream(); ImageIO.write(image, "jpg", byteArrayOut); HSSFClientAnchor anchor = new HSSFClientAnchor(0, 0, 0, 0, l, i + h, (short) (l+1), (i+1) + h); anchor.setAnchorType(0); // 插入圖片 patriarch.createPicture(anchor, wb.addPicture( byteArrayOut.toByteArray(), HSSFWorkbook.PICTURE_TYPE_JPEG)); } try { /** * 輸出到瀏覽器 */ bufferedOutPut.flush(); wb.write(bufferedOutPut); bufferedOutPut.close();//關流 } catch (IOException e) { e.printStackTrace(); } finally { list.clear(); } } }
(4)Servlet類,注意看路徑,我這裡是模擬了資料庫資訊,而且在伺服器專案下需要有對應的圖片路徑
總結package com.sram.servlet; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; import java.util.ArrayList; import java.util.List; import javax.imageio.ImageIO; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import com.sram.bean.User; import com.sram.util.ExportToExcelUtil; /** * * @類名: OutPut2ExcelServlet * @描述: 將使用者資訊和圖片匯出到excel * @作者: chenchaoyun0 * @日期: 2016-1-28 下午10:08:57 * */ public class OutPut2ExcelServlet extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doPost(request, response); } public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html;charset=utf-8"); request.setCharacterEncoding("utf-8"); /**************我是分割線***********************/ /** * */ User user1=new User(1, "chenchaoyun0","/head/qq5.jpg"); User user2=new User(2, "呵呵噠","/head/qq1.jpg"); User user3=new User(3, "陳哈哈","/head/qq2.jpg"); User user4=new User(4, "喔喔喔","/head/qq3.jpg"); User user5=new User(5, "咳咳咳","/head/qq4.jpg"); /** * */ List<User> al=new ArrayList<User>(); al.add(user1); al.add(user2); al.add(user3); al.add(user4); al.add(user5); /** * 為使用者設定頭像 */ for (int i = 0; i < al.size(); i++) { User user = al.get(i); String realPath = this.getServletContext().getRealPath(user.getUser_headSrc());//獲取真實圖片路徑 BufferedImage img=ImageIO.read(new File(realPath)); user.setUser_headImg(img);//為每個user設定屬性 } /** * 把response給excel工具類,再輸出到瀏覽器 */ try { ExportToExcelUtil.out(response, al); } catch (Exception e) { e.printStackTrace(); } } }
(1)這個程式需要在(伺服器)tomcat下包含圖片的路徑,否則會報找不到路徑錯。我在寫二階段專案的時候是將使用者的頭像放在屬於使用者名稱下的資料夾下,但現在發現這個並不合理,因為隨著使用者更換頭像,該資料夾下的圖片會越來越多,雖說也可以更新一次頭像將之前的頭像刪掉,使用者的頭像個人覺得應該以位元組的儲存到資料庫較好些,讀取的時候在以位元組資料轉換顯示。但是在工作中是怎麼用本人還沒有接觸過。在這裡就不作演示了。
(2)另一個弊端則是實體類多了一個屬性,如果說考略到效能方面的話,假如說把使用者的BufferedImage這個屬性幹掉的話,在excel編輯使用者資訊類裡通過使用者的頭像路徑new 一個BufferedImage物件的方法去寫出圖片資訊。後者這個方法我還沒有試過,有興趣的盆友可以試試。但是如果說考慮到效能優化的話在迴圈裡new 物件或者宣告臨時變數時不合理的。有興趣的盆友可以去了解一下java的效能優化。
最後說幾句
本人第一次寫部落格,格式或許有些不對,希望大家指出,如對程式碼有什麼疑問的可聯絡我郵箱,程式有需要改進的地方請大家指出。如果大家喜歡就幫忙推薦一下~~~網友的支援和吐槽等都是我寫部落格的動力~~~
相關推薦
javaweb匯出Excel資料與圖片
簡介 Apache POI 是用Java編寫的免費開源的跨平臺的 Java API,Apache POI提供API給Java程式對Microsoft Office格式檔案讀和寫的功能。POI為“Poor Obfuscation Implementation”的首字母縮寫
匯出excel資料
<el-form-item> <el-button type="info" @click="exportOrderList"><i class="el-icon-upload2"></i>匯出excel</el-button&g
【MATLAB】匯入和匯出Excel資料
1 寫在前面 MATLAB 中有時候需要用到外部的資料進行模擬計算,或者有時需要把 MATLAB 中的資料匯出到外部表格,這就用到 Excel 與 MATLAB 之間的命令函式。 2 匯入 A=xlsread('xxx.xlsx') # xxx 為檔名稱 其中
vue匯出excel資料表格功能
前端工作量最多的就是需求,需求就是一直在變,比如當前端資料寫完之後,需要用Excel把資料下載出來。 第一步安裝依賴包,需要把程式碼下載你的專案當中 cnpm install file-saver cnpm install xlsx cnpm install script-loader
NPOI匯出excel,插入圖片
//add picture data to this workbook. byte[] bytes = System.IO.File.ReadAllBytes(@"D:\MyProject\NPOIDemo\ShapeImage\image1.jpg"); int pi
C#實現匯入匯出Excel資料的兩種方法詳解
這篇文章主要為大家詳細介紹了C#匯入匯出Excel資料的兩種方法,具有一定的參考價值,感興趣的小夥伴們可以參考一下本文為大家分享了C#匯入匯出Excel資料的具體程式碼,供大家參考,具體內容如下注:對於實體類物件最好新建一個並且繼承原有實體類,這樣可以將型別進行修改;方法一:
使用poi通過excel模板匯出excel資料
期望:用預先的xlsx的Excel表格模板參考:兩篇部落格步驟:模板+資料:相關jar報 座標:(注意jar版本 否則再tempWorkBook = new XSSFWorkbook(inputstre
動態建立gridview,匯出Excel資料
先建立一個GridControl,在建立一個GridView檢視,再傳入資料 GridControl CreateGrid(List<marketinformation> pagemodel) { GridControl grid = new
匯入EXCEL資料與自助式定義校驗關係
我們知道在企業中廣泛使用的資料採集獲取方式就是EXCEL表格填報。這主要原因是EXCEL製表和填寫使用比較簡單,並且EXCEL表格檔案的傳送與傳遞也很便利,能夠很容易地將表格下發給各級部門,並在各部門填報完成後將EXCEL檔案再次輕鬆地彙總收集上來。
C# 將圖片匯出Excel(包括 建立Excel 、檔案壓縮、遞迴刪除檔案及資料夾)
新增引用 using ICSharpCode.SharpZipLib.Zip; public void CreateDirectory(string DirectoryPath) { if (!Directory.Exist
PHP資料匯出excel表的外掛與運用
外掛下載在我的這裡有我已經上傳了,自己下載,遇到問題可以在下方留言, 1、包放在 ThinkPHP -> Library -> Vendor; 2、前端:程式碼 <div class="btn-group" style="float: left; mar
sql server 2005中表的資料與excel互相匯入匯出的方法
1、將EXECEL匯入到SQLSERVER2005資料庫方法示例: insert into dbo.tbpointconfig(pointname,punit,pmax,pmin,pvalue,generatorid,pdate,cdbh)SELECT 測點名稱,單位
匯出 excel表格(資料、echarts圖片)
/*** @Description:匯出* @author: liuc* @since: 2016年4月14日 上午10:36:39*/@RequestMapping("/exportTotalData")public void exportTotalDataList(Ht
查詢語句SqlServer與Excel資料匯入匯出
一、Excel檔案在sqlserver資料庫的本地 1、啟用Ad Hoc Distributed Queries的方法exec sp_configure 'show advanced options',1reconfigureexec sp_configure 'Ad
匯出 excel表格(資料、echarts圖片)
/*** @Description:匯出* @author: liuc* @since: 2016年4月14日 上午10:36:39*/@RequestMapping("/exportTotalData")public void exportTotalDataList(Ht
sqldeveloper中Excel資料的匯入與匯出
一:sqldeveloper中資料(Excel)的匯入 1.在資料庫中建立表(以ceshi表為例) create table ceshi(ids varchar(20),xingqi varchar(
SpringBoot圖文教程10—模板匯出|百萬資料Excel匯出|圖片匯出「easypoi」
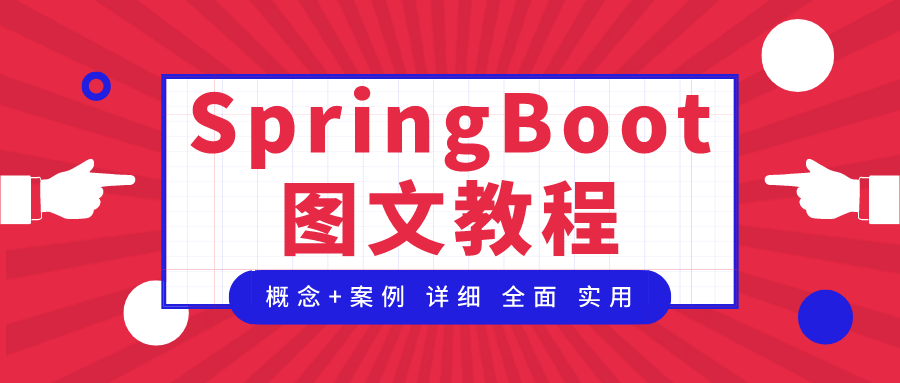 > **有天上飛的概念,就要有落地的實現** > > - 概念十遍不如程式碼一遍,朋友,希望你把文
PHP 實現大資料(30w量級)表格匯出(匯出excel) 提高效率,減少記憶體消耗,終極解決方案
使用php做專案開發的同學,一定都會有過使用php進行excel表格匯出的經歷,當匯出少量資料還好,一旦資料量級達到5w、 10w、20w甚至30以上的時候就會面臨同樣的問題: 1、匯出時間變得很慢,少則1分鐘,多則好幾分鐘,資料量一旦上來,還可能面臨導不出來的困窘(這種匯出效率正常人都會受
使用 jxl 根據下載資料模版匯出 excel 表——合併配置
使用jxl根據下載資料模版匯出excel表——合併配置: 首先根據模版檔案路徑讀取excel模版檔案,然後對excel檔案進行修改,即寫出資料到excel檔案中,再將該excel檔案儲存到目標檔案中,這裡操作excel必須是2003版本(.xls) 準備excel模版檔案
BCP SQL匯出EXCEL常見問題及解決方法;資料匯出儲存過程
一、‘xp_cmdshell’的啟用 SQL Server阻止了對元件‘xp_cmdshell’的過程‘sys.xp_cmdshell’的訪問。因為此元件已作為此服務囂安全配置的一部分而被關 閉。系統管理員可以通過使用sp_configure啟用‘xp_cmdshell’。有關啟用‘xp_cmdshell’