WPF教程(十五)文字框——內聯格式
上章我們學習了文字框最核心的功能:顯示字串,在必要的時候換行。我們還用了其他顏色來凸顯文字,如果你想做的遠遠不止這些,怎麼辦?
幸好文字框支援內聯的內容。這些像控制元件一樣的結構全部繼承於內聯類,這意外著它們可以作為文字的一部分來傳遞。支援的元素包括AnchoredBlock, Bold, Hyperlink, InlineUIContainer, Italic, LineBreak, Run, Span, and Underline。接下來我們一個一個來看。
Bold, Italic and Underline
這三個是最簡單的內聯元素。從名字就可以看出是幹什麼的,例子如下:<Window x:Class="WpfTutorialSamples.Basic_controls.TextBlockInlineSample" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Title="TextBlockInlineSample" Height="100" Width="300"> <Grid> <TextBlock Margin="10" TextWrapping="Wrap"> TextBlock with <Bold>bold</Bold>, <Italic>italic</Italic> and <Underline>underlined</Underline> text. </TextBlock> </Grid> </Window>
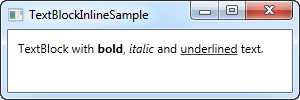
和HTML一樣,在文字兩端加上Bold標籤,就可以加粗文字。這三個元素是Span元素的子類,各自實現了具體的屬性值來達到預期的效果。如Bold標籤設定了FontWeight屬性,Italic設定了FontStyle屬性。
LineBreak
插入一個換行符。可以參看前面的例子,我們用過。
Hyperlink
超連結用於把連結新增到文字中。它的顯示樣式由系統主題決定,通常是藍色的字型帶下劃線,滑鼠懸停時變小手同時字型變紅色。通過使用NavigateUri屬性來設定你要跳轉到的URL。
<Window x:Class="WpfTutorialSamples.Basic_controls.TextBlockHyperlinkSample" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Title="TextBlockHyperlinkSample" Height="100" Width="300"> <Grid> <TextBlock Margin="10" TextWrapping="Wrap"> This text has a <Hyperlink RequestNavigate="Hyperlink_RequestNavigate" NavigateUri="https://www.google.com">link</Hyperlink> in it. </TextBlock> </Grid> </Window>
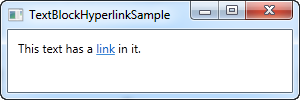
超連結也用在WPF內部的網頁,可以在網頁之間跳轉。這種情況下,你不需要具體處理RequestNavigate事件。但是,如果是從WPF程式載入外部URL,這個事件非常有用。我們訂閱RequestNavigate事件後,在載入URL時可以帶一個事件處理函式:
Runprivate void Hyperlink_RequestNavigate(object sender, System.Windows.Navigation.RequestNavigateEventArgs e) { System.Diagnostics.Process.Start(e.Uri.AbsoluteUri); }
允許你使用所有Span元素可用的屬性來設定文字的樣式。但是Span元素可能包含其他內聯元素,因此Run元素可能只包含空文字。這使得Span元素更加靈活。
Span
該元素預設情況下沒有具體的形式,允許你設定大部分具體的顯示屬性,如字型、樣式、大小、背景色和前景色等。Span元素最牛逼的自然是可以把其他內聯元素放在它的裡面,這樣就可以聯合各種各樣的文字了。接下來展示更多Span元素的使用:
<Window x:Class="WpfTutorialSamples.Basic_controls.TextBlockSpanSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="TextBlockSpanSample" Height="100" Width="300">
<Grid>
<TextBlock Margin="10" TextWrapping="Wrap">
This <Span FontWeight="Bold">is</Span> a
<Span Background="Silver" Foreground="Maroon">TextBlock</Span>
with <Span TextDecorations="Underline">several</Span>
<Span FontStyle="Italic">Span</Span> elements,
<Span Foreground="Blue">
using a <Bold>variety</Bold> of <Italic>styles</Italic>
</Span>.
</TextBlock>
</Grid>
</Window>
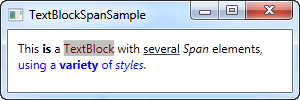
如果其他元素都非常重要,或者你只想有一個空白的地方,Span元素是最好的原則。
從後臺程式碼來格式化文字
從XAML格式文字非常容易,但是有時候,你會遇到需要在後臺程式碼裡實現。這個有點麻煩,來看例子:
<Window x:Class="WpfTutorialSamples.Basic_controls.TextBlockCodeBehindSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="TextBlockCodeBehindSample" Height="100" Width="300">
<Grid></Grid>
</Window>
using System;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Media;
namespace WpfTutorialSamples.Basic_controls
{
public partial class TextBlockCodeBehindSample : Window
{
public TextBlockCodeBehindSample()
{
InitializeComponent();
TextBlock tb = new TextBlock();
tb.TextWrapping = TextWrapping.Wrap;
tb.Margin = new Thickness(10);
tb.Inlines.Add("An example on ");
tb.Inlines.Add(new Run("the TextBlock control ") { FontWeight = FontWeights.Bold });
tb.Inlines.Add("using ");
tb.Inlines.Add(new Run("inline ") { FontStyle = FontStyles.Italic });
tb.Inlines.Add(new Run("text formatting ") { Foreground = Brushes.Blue });
tb.Inlines.Add("from ");
tb.Inlines.Add(new Run("Code-Behind") { TextDecorations = TextDecorations.Underline });
tb.Inlines.Add(".");
this.Content = tb;
}
}
}
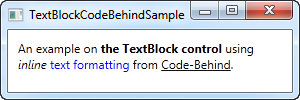
從後臺程式碼是可以實現的,在某些情況下非常必要。然而,這個例子讓我們更加意識到XAML的偉大。