Android中主流狀態列效果實現
阿新 • • 發佈:2019-01-26
Android在早期的系統版本中,狀態列是不支援修改的,所以開啟任何應用程式會發現頂部的狀態列始終是黑條。在Android 4.4(KitKat)之後,系統的狀態列開始支援開發者定製和修改,包括顯示或隱藏,更改顏色等(嗯,一定是抄襲ios的...),又在Android 5.0(LOLLIPOP)進行了改進。這樣一來,我們就可以讓系統狀態列跟隨應用程式改變了。
下面總結了市面上幾種常見的StatusBar顯示效果。
一.狀態列完全透明
應用程式的某些頁面在狀態列完全透明,且內容區延伸到狀態列時,視覺體驗會更佳。這種顯示效果在各種應用的啟動頁中較為常見,因為啟動頁的背景是一張大圖,全屏顯示效果更好。另外在如墨跡天氣中這種效果也在多個頁面中被使用到。
以中華萬年曆的天氣頁面為例,效果如下所示:
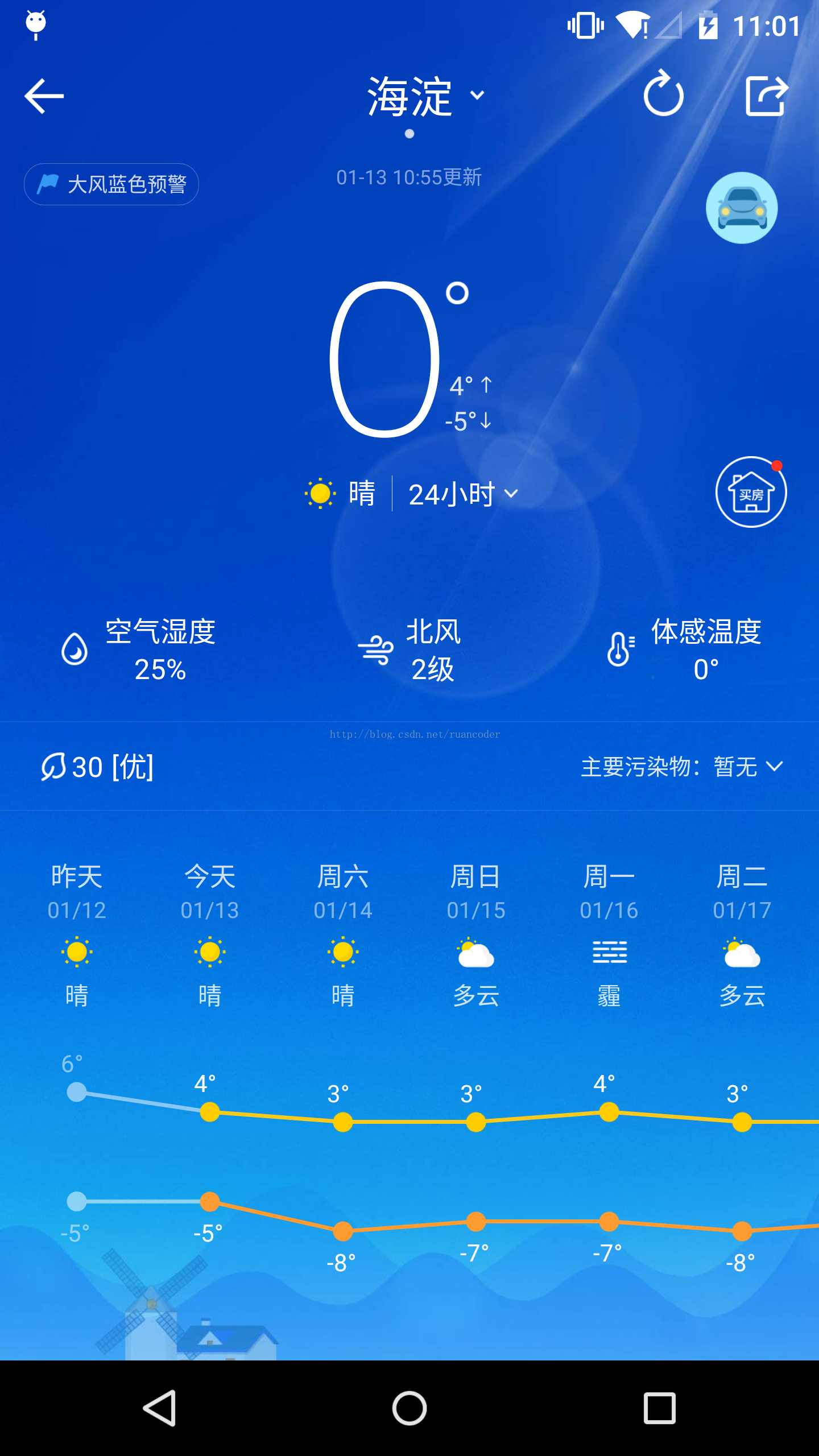
接下來進入程式碼實現。
新增如下styles.xml檔案。
values/styles.xml
values-v19/styles.xml
values-v21/styles.xml
注意,android:windowTranslucentStatus是在4.4版本引入的屬性,android:statusBarColor是在5.0版本引入的屬性,如果不新增到相應的values資料夾,會編譯不通過。
styles在工程中的結構如圖:
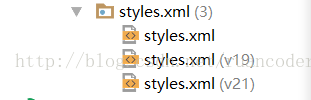
最後再將定義好的樣式新增的清單檔案中(android:theme="@style/AppTheme"),可以是<application>節點,也可以是<activity>節點,根據應用的需要來定。
上述程式碼在網上隨處可見,但是在實際測試時發現,5.0以上的裝置狀態列會顯示出透明灰色,不能達到我們想要的效果。在Activity的onCreate()方法中,呼叫setContentView()之前新增如下程式碼可解決。
到這裡就已實現我們想要的效果。另外需要注意的是,在4.4版本中,StatusBar只能實現半透明,會有一層半透明黑色覆蓋在狀態列上。
二.狀態列與標題欄顏色一致
這種效果比較常見,狀態列與標題欄顏色一致形成一個整體,如網易新聞,網易雲音樂,今日頭條,華爾街見聞等。
示例圖如下:
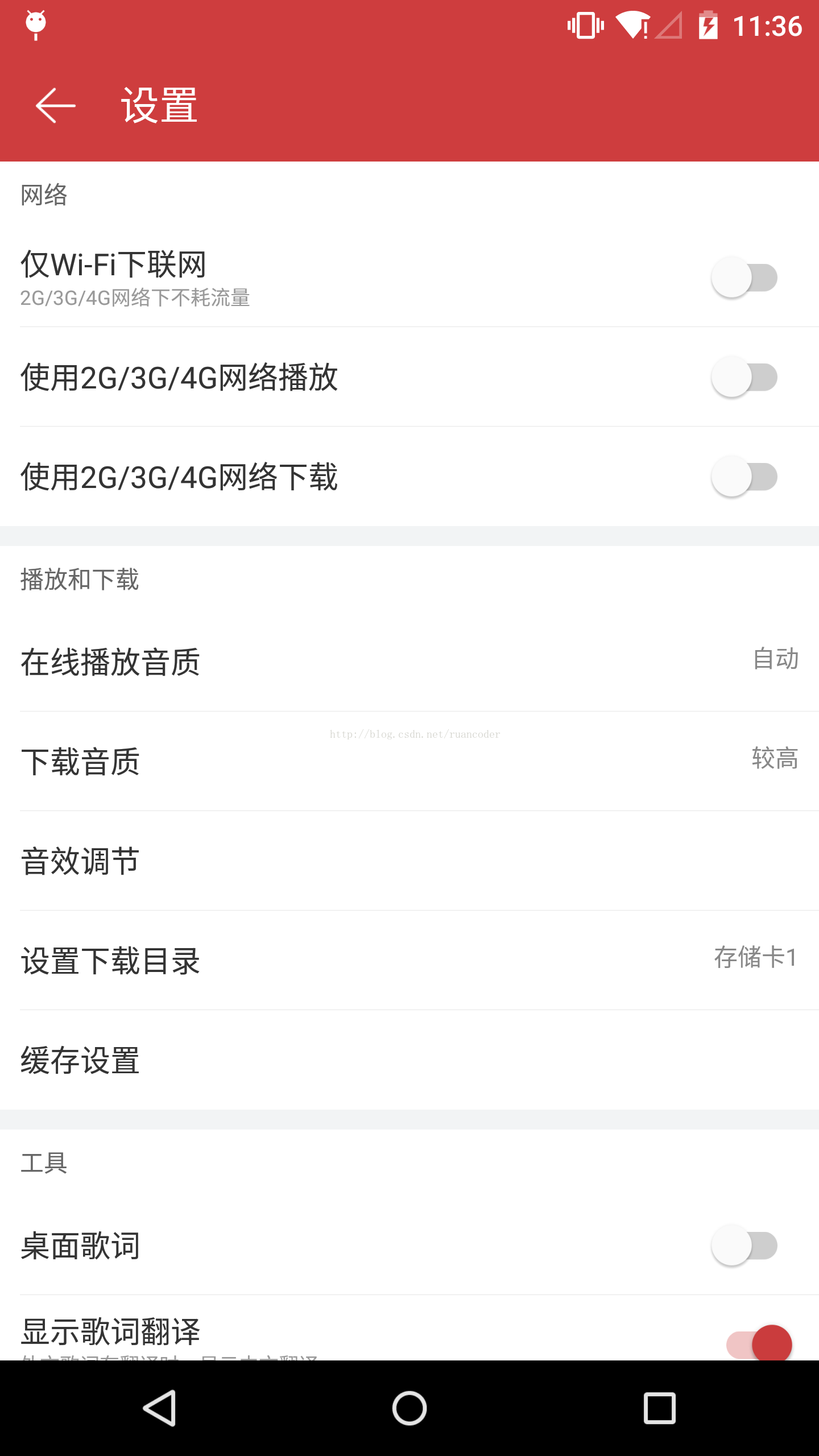
同樣是通過styles.xml檔案實現。
values/styles.xml
values-v19/styles.xml
實現上述效果關鍵在於colorPrimary和colorPrimaryDark。colorPrimary是應用程式的主色調,狀態列預設會使用colorPrimaryDark設定的顏色。這裡不用新增values-v21/styles.xml,當然,如果願意,在values-v21/styles.xml中增加<item name="android:statusBarColor">@color/colorPrimary</item>也可以,android:statusBarColor用於定義狀態列的顏色。
對colorPrimary和colorPrimaryDark的理解,可參考下圖:
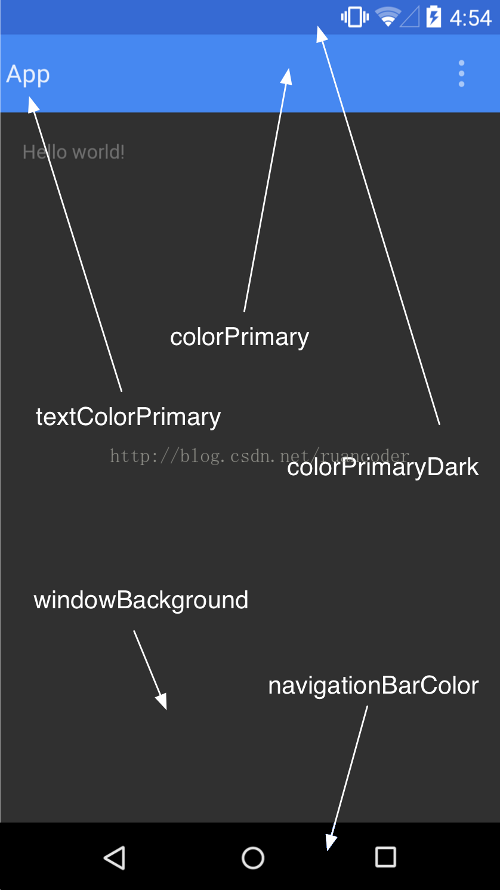
佈局檔案activity_main.xml如下。
如果使用了Toolbar作為標題欄(自己寫View也可以),一定記得在Activity中新增setSupportActionBar(toolbar)。
三.狀態列比標題欄顏色加深
示例圖如下:
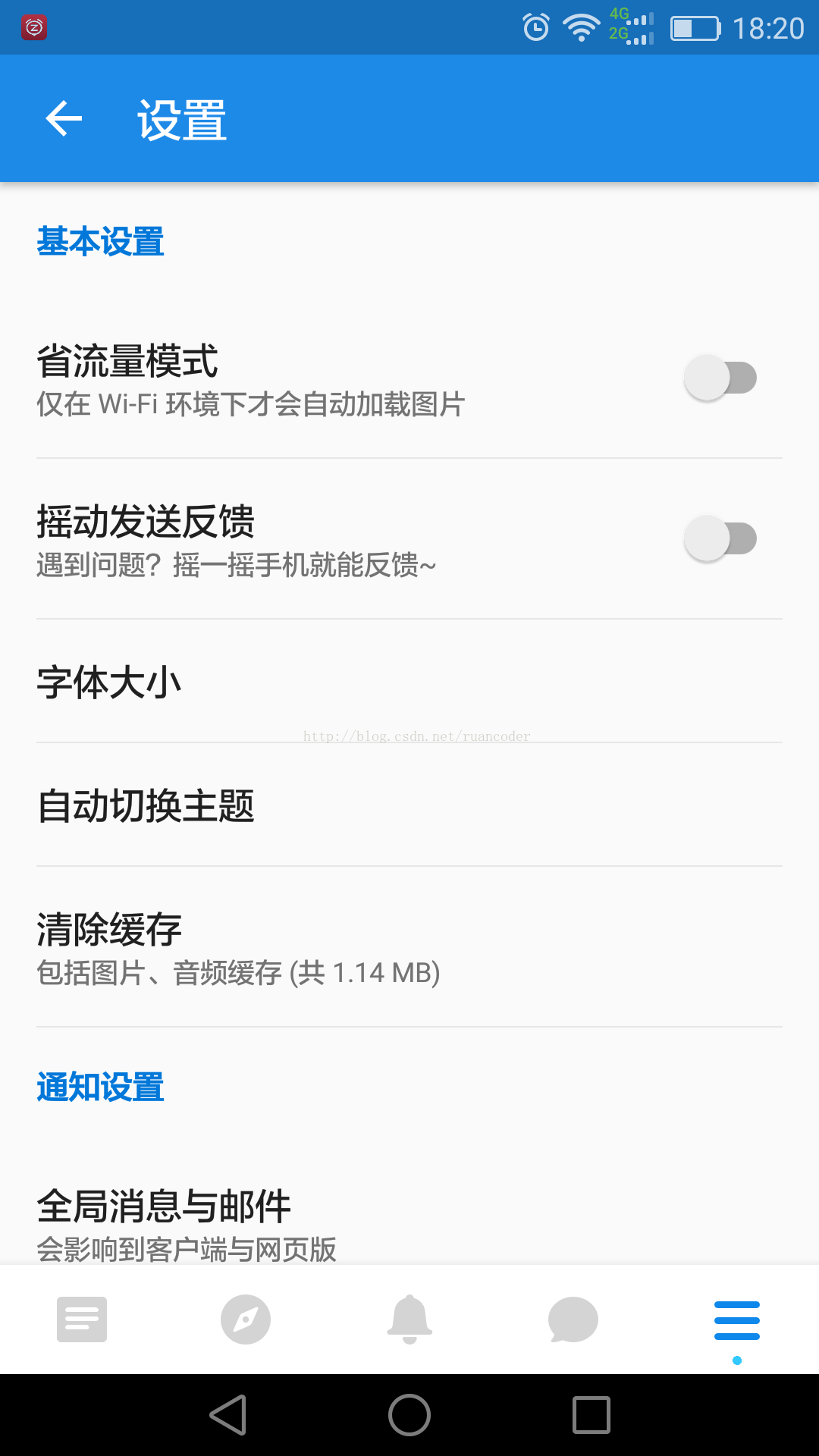
該效果只出現在Android 5.0以上版本中。當我們在Studio中建立工程時,如果選中整合AppCompat,創建出來的應用就是這種顯示效果,同時也是Material Design推薦的做法。嚴格遵循Material Design的app,如知乎、餓了麼都是這種效果。
實現方法同上述第二種情況一樣,新增styles.xml檔案即可。其中colorPrimaryDark顏色比colorPrimary深。
values/styles.xml
附官方Material Design培訓教程:
https://developer.android.google.cn/training/material/index.html
下面總結了市面上幾種常見的StatusBar顯示效果。
一.狀態列完全透明
應用程式的某些頁面在狀態列完全透明,且內容區延伸到狀態列時,視覺體驗會更佳。這種顯示效果在各種應用的啟動頁中較為常見,因為啟動頁的背景是一張大圖,全屏顯示效果更好。另外在如墨跡天氣中這種效果也在多個頁面中被使用到。
以中華萬年曆的天氣頁面為例,效果如下所示:
接下來進入程式碼實現。
新增如下styles.xml檔案。
values/styles.xml
<resources> <!-- Base application theme. --> <style name="AppBaseTheme" parent="Theme.AppCompat.Light.NoActionBar"> <!-- Customize your theme here. --> </style> <style name="AppTheme" parent="AppBaseTheme"> </style> </resources>
values-v19/styles.xml
<resources>
<style name="AppTheme" parent="AppBaseTheme">
<item name="android:windowTranslucentStatus">true</item>
</style>
</resources>
values-v21/styles.xml
<resources> <style name="AppTheme" parent="AppBaseTheme"> <item name="android:statusBarColor">@android:color/transparent</item> </style> </resources>
注意,android:windowTranslucentStatus是在4.4版本引入的屬性,android:statusBarColor是在5.0版本引入的屬性,如果不新增到相應的values資料夾,會編譯不通過。
styles在工程中的結構如圖:
最後再將定義好的樣式新增的清單檔案中(android:theme="@style/AppTheme"),可以是<application>節點,也可以是<activity>節點,根據應用的需要來定。
<application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme"> </application>
上述程式碼在網上隨處可見,但是在實際測試時發現,5.0以上的裝置狀態列會顯示出透明灰色,不能達到我們想要的效果。在Activity的onCreate()方法中,呼叫setContentView()之前新增如下程式碼可解決。
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP) {
getWindow().getDecorView().setSystemUiVisibility(View.SYSTEM_UI_FLAG_LAYOUT_STABLE | View.SYSTEM_UI_FLAG_LAYOUT_FULLSCREEN);
}
到這裡就已實現我們想要的效果。另外需要注意的是,在4.4版本中,StatusBar只能實現半透明,會有一層半透明黑色覆蓋在狀態列上。
二.狀態列與標題欄顏色一致
這種效果比較常見,狀態列與標題欄顏色一致形成一個整體,如網易新聞,網易雲音樂,今日頭條,華爾街見聞等。
示例圖如下:
同樣是通過styles.xml檔案實現。
values/styles.xml
<resources>
<!-- Base application theme. -->
<style name="AppBaseTheme" parent="Theme.AppCompat.Light.NoActionBar">
<!-- Main theme colors -->
<!-- your app branding color for the app bar -->
<item name="colorPrimary">@color/colorPrimary</item>
<!-- darker variant for the status bar and contextual app bars -->
<item name="colorPrimaryDark">@color/colorPrimary</item>
<!-- Customize your theme here. -->
</style>
<style name="AppTheme" parent="AppBaseTheme">
</style>
</resources>
values-v19/styles.xml
<resources>
<style name="AppTheme" parent="AppBaseTheme">
<item name="android:windowTranslucentStatus">true</item>
</style>
</resources>
實現上述效果關鍵在於colorPrimary和colorPrimaryDark。colorPrimary是應用程式的主色調,狀態列預設會使用colorPrimaryDark設定的顏色。這裡不用新增values-v21/styles.xml,當然,如果願意,在values-v21/styles.xml中增加<item name="android:statusBarColor">@color/colorPrimary</item>也可以,android:statusBarColor用於定義狀態列的顏色。
對colorPrimary和colorPrimaryDark的理解,可參考下圖:
佈局檔案activity_main.xml如下。
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/colorPrimary"
android:minHeight="?attr/actionBarSize">
</android.support.v7.widget.Toolbar>-->
</LinearLayout>
如果使用了Toolbar作為標題欄(自己寫View也可以),一定記得在Activity中新增setSupportActionBar(toolbar)。
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
}
}
三.狀態列比標題欄顏色加深
示例圖如下:
該效果只出現在Android 5.0以上版本中。當我們在Studio中建立工程時,如果選中整合AppCompat,創建出來的應用就是這種顯示效果,同時也是Material Design推薦的做法。嚴格遵循Material Design的app,如知乎、餓了麼都是這種效果。
實現方法同上述第二種情況一樣,新增styles.xml檔案即可。其中colorPrimaryDark顏色比colorPrimary深。
values/styles.xml
<resources>
<!-- Base application theme. -->
<style name="AppBaseTheme" parent="Theme.AppCompat.Light.NoActionBar">
<!-- Main theme colors -->
<!-- your app branding color for the app bar -->
<item name="colorPrimary">@color/colorPrimary</item>
<!-- darker variant for the status bar and contextual app bars -->
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<!-- Customize your theme here. -->
</style>
<style name="AppTheme" parent="AppBaseTheme">
</style>
</resources>
附官方Material Design培訓教程:
https://developer.android.google.cn/training/material/index.html