例項PK(Vue服務端渲染 VS Vue瀏覽器端渲染)
Vue 2.0 開始支援服務端渲染的功能,所以本文章也是基於vue 2.0以上版本。網上對於服務端渲染的資料還是比較少,最經典的莫過於Vue作者尤雨溪大神的 vue-hacker-news。本人在公司做Vue專案的時候,一直苦於產品、客戶對首屏載入要求,SEO的訴求,也想過很多解決方案,本次也是針對瀏覽器渲染不足之處,採用了服務端渲染,並且做了兩個一樣的Demo作為比較,更能直觀的對比Vue前後端的渲染。
話不多說,我們分別來看兩個Demo:(歡迎star 歡迎pull request)
兩套程式碼執行結果都是為了展示豆瓣電影的,執行效果也都是差不多,下面我們來分別簡單的闡述一下專案的機理:
一、瀏覽器端渲染豆瓣電影
首先我們用官網的腳手架搭建起來一個vue專案
npm install -g vue-cli vue init webpack doubanMovie cd doubanMovie npm install npm run dev
這樣便可以簡單地打起來一個cli框架,下面我們要做的事情就是分別配置 vue-router, vuex,然後配置我們的webpack proxyTable 讓他支援代理訪問豆瓣API。
1.配置Vue-router
我們需要三個導航頁:正在上映、即將上映、Top250;一個詳情頁,一個搜尋頁。這裡我給他們分別配置了各自的路由。在 router/index.js 下配置以下資訊:
import Vue from 'vue'
import Router from 'vue-router'
import Moving from '@/components/moving'
import Upcoming from '@/components/upcoming'
import Top250 from '@/components/top250'
import MoviesDetail from '@/components/common/moviesDetail'
import Search from '@/components/searchList'
Vue.use(Router)
/**
* 路由資訊配置
*/
export default new Router({
routes: [
{
path: '/',
name: 'Moving',
component: Moving
},
{
path: '/upcoming',
name: 'upcoming',
component: Upcoming
},
{
path: '/top250',
name: 'Top250',
component: Top250
},
{
path: '/search',
name: 'Search',
component: Search
},
{
path: '/moviesDetail',
name: 'moviesDetail',
component: MoviesDetail
}
]
})
這樣我們的路由資訊配置好了,然後每次切換路由的時候,儘量避免不要重複請求資料,所以我們還需要配置一下元件的keep-alive:在app.vue元件裡面。
<keep-alive exclude="moviesDetail"> <router-view></router-view> </keep-alive>
這樣一個基本的vue-router就配置好了。
2.引入vuex
Vuex 是一個專為 Vue.js 應用程式開發的狀態管理模式。它採用集中式儲存管理應用的所有元件的狀態,並以相應的規則保證狀態以一種可預測的方式發生變化。Vuex 也整合到 Vue 的官方除錯工具 devtools extension,提供了諸如零配置的 time-travel 除錯、狀態快照匯入匯出等高階除錯功能。
簡而言之:Vuex 相當於某種意義上設定了讀寫許可權的全域性變數,將資料儲存儲存到該“全域性變數”下,並通過一定的方法去讀寫資料。
Vuex 並不限制你的程式碼結構。但是,它規定了一些需要遵守的規則:
-
應用層級的狀態應該集中到單個 store 物件中。
-
提交 mutation 是更改狀態的唯一方法,並且這個過程是同步的。
-
非同步邏輯都應該封裝到 action 裡面。
├── index.html ├── main.js ├── api │ └── ... # 抽取出API請求 ├── components │ ├── App.vue │ └── ... └── store ├── index.js # 我們組裝模組並匯出 store 的地方 └── moving # 電影模組 ├── index.js # 模組內組裝,並匯出模組的地方 ├── actions.js # 模組基本 action ├── getters.js # 模組級別 getters ├── mutations.js # 模組級別 mutations └── types.js # 模組級別 types
所以我們開始在我們的src目錄下新建一個名為store 的資料夾 為了後期考慮 我們新建了moving 資料夾,用來組織電影,考慮到所有的action,getters,mutations,都寫在一起,檔案太混亂,所以我又給他們分別提取出來。
stroe資料夾建好,我們要開始在main.js裡面引用vuex例項:
import store from './store'
new Vue({
el: '#app',
router,
store,
template: '<App/>',
components: { App }
})
這樣,我們便可以在所有的子元件裡通過 this.$store 來使用vuex了。
3.webpack proxyTable 代理跨域
webpack 開發環境可以使用proxyTable 來代理跨域,生產環境的話可以根據各自的伺服器進行配置代理跨域就行了。在我們的專案config/index.js 檔案下可以看到有一個proxyTable的屬性,我們對其簡單的改寫
proxyTable: {
'/api': {
target: 'http://api.douban.com/v2',
changeOrigin: true,
pathRewrite: {
'^/api': ''
}
}
}
這樣當我們訪問
localhost:8080/api/movie
的時候 其實我們訪問的是
http://api.douban.com/v2/movie
這樣便達到了一種跨域請求的方案。
至此,瀏覽器端的主要配置已經介紹完了,下面我們來看看執行的結果:
為了介紹瀏覽器渲染是怎麼回事,我們執行一下npm run build 看看我們的釋出版本的檔案,到底是什麼鬼東西....
run build 後會都出一個dist目錄 ,我們可以看到裡面有個index.html,這個便是我們最終頁面將要展示的html,我們開啟,可以看到下面:
觀察好的小夥伴可以發現,我們並沒有多餘的dom元素,就只有一個div,那麼頁面要怎麼呈現呢?答案是js append,對,下面的那些js會負責innerHTML。而js是由瀏覽器解釋執行的,所以呢,我們稱之為瀏覽器渲染,這有幾個致命的缺點:
1.js放在dom結尾,如果js檔案過大,那麼必然造成頁面阻塞。使用者體驗明顯不好(這也是我我在公司反覆被產品逼問的事情)
2.不利於SEO
3.客戶端執行在老的JavaScript引擎上
對於世界上的一些地區人,可能只能用1998年產的電腦訪問網際網路的方式使用計算機。而Vue只能執行在IE9以上的瀏覽器,你可能也想為那些老式瀏覽器提供基礎內容 - 或者是在命令列中使用 Lynx的時髦的黑客
基於以上的一些問題,服務端渲染呼之欲出....
二、伺服器端渲染豆瓣電影
先看一張Vue官網的服務端渲染示意圖
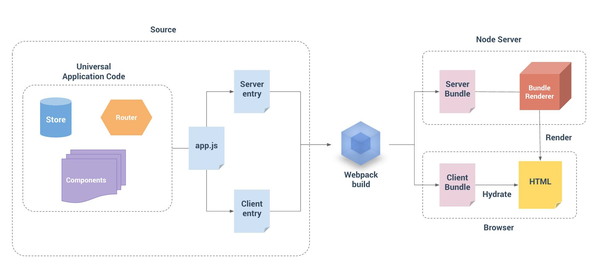
從圖上可以看出,ssr 有兩個入口檔案,client.js 和 server.js, 都包含了應用程式碼,webpack 通過兩個入口檔案分別打包成給服務端用的 server bundle 和給客戶端用的 client bundle. 當伺服器接收到了來自客戶端的請求之後,會建立一個渲染器 bundleRenderer,這個 bundleRenderer 會讀取上面生成的 server bundle 檔案,並且執行它的程式碼, 然後傳送一個生成好的 html 到瀏覽器,等到客戶端載入了 client bundle
之後,會和服務端生成的DOM 進行 Hydration(判斷這個DOM 和自己即將生成的DOM 是否相同,如果相同就將客戶端的vue例項掛載到這個DOM上, 否則會提示警告)。
具體實現:
我們需要vuex,需要router,需要伺服器,需要服務快取,需要代理跨域....不急我們慢慢來。
1.建立nodejs服務
首先我們需要一個伺服器,那麼對於nodejs,express是很好地選擇。我們來建立一個server.js
const port = process.env.PORT || 8080
app.listen(port, () => {
console.log(`server started at localhost:${port}`)
})
這裡用來啟動服務監聽 8080 埠。
然後我們開始處理所有的get請求,當請求頁面的時候,我們需要渲染頁面
app.get('*', (req, res) => {
if (!renderer) {
return res.end('waiting for compilation... refresh in a moment.')
}
const s = Date.now()
res.setHeader("Content-Type", "text/html")
res.setHeader("Server", serverInfo)
const errorHandler = err => {
if (err && err.code === 404) {
res.status(404).end('404 | Page Not Found')
} else {
// Render Error Page or Redirect
res.status(500).end('500 | Internal Server Error')
console.error(`error during render : ${req.url}`)
console.error(err)
}
}
renderer.renderToStream({ url: req.url })
.on('error', errorHandler)
.on('end', () => console.log(`whole request: ${Date.now() - s}ms`))
.pipe(res)
})
然後我們需要代理請求,這樣才能進行跨域,我們引入http-proxy-middleware模組:
const proxy = require('http-proxy-middleware');//引入代理中介軟體
/**
* proxy middleware options
* 代理跨域配置
* @type {{target: string, changeOrigin: boolean, pathRewrite: {^/api: string}}}
*/
var options = {
target: 'http://api.douban.com/v2', // target host
changeOrigin: true, // needed for virtual hosted sites
pathRewrite: {
'^/api': ''
}
};
var exampleProxy = proxy(options);
app.use('/api', exampleProxy);
這樣我們的服務端server.js便配置完成。接下來 我們需要配置服務端入口檔案,還有客戶端入口檔案,首先來配置一下客戶端檔案,新建src/entry-client.js
import 'es6-promise/auto' import { app, store, router } from './app' // prime the store with server-initialized state. // the state is determined during SSR and inlined in the page markup. if (window.__INITIAL_STATE__) { store.replaceState(window.__INITIAL_STATE__) } /** * 非同步元件 */ router.onReady(() => { // 開始掛載到dom上 app.$mount('#app') }) // service worker if (process.env.NODE_ENV === 'production' && 'serviceWorker' in navigator) { navigator.serviceWorker.register('/service-worker.js') }
客戶端入口檔案很簡單,同步服務端傳送過來的資料,然後把 vue 例項掛載到服務端渲染的 DOM 上。
再配置一下服務端入口檔案:src/entry-server.js
import { app, router, store } from './app' const isDev = process.env.NODE_ENV !== 'production' // This exported function will be called by `bundleRenderer`. // This is where we perform data-prefetching to determine the // state of our application before actually rendering it. // Since data fetching is async, this function is expected to // return a Promise that resolves to the app instance. export default context => { const s = isDev && Date.now() return new Promise((resolve, reject) => { // set router's location router.push(context.url) // wait until router has resolved possible async hooks router.onReady(() => { const matchedComponents = router.getMatchedComponents() // no matched routes if (!matchedComponents.length) { reject({ code: 404 }) } // Call preFetch hooks on components matched by the route. // A preFetch hook dispatches a store action and returns a Promise, // which is resolved when the action is complete and store state has been // updated. Promise.all(matchedComponents.map(component => { return component.preFetch && component.preFetch(store) })).then(() => { isDev && console.log(`data pre-fetch: ${Date.now() - s}ms`) // After all preFetch hooks are resolved, our store is now // filled with the state needed to render the app. // Expose the state on the render context, and let the request handler // inline the state in the HTML response. This allows the client-side // store to pick-up the server-side state without having to duplicate // the initial data fetching on the client. context.state = store.state resolve(app) }).catch(reject) }) }) }
server.js 返回一個函式,該函式接受一個從服務端傳遞過來的 context 的引數,將 vue 例項通過 promise 返回。context 一般包含 當前頁面的url,首先我們呼叫 vue-router 的 router.push(url) 切換到到對應的路由, 然後呼叫 getMatchedComponents 方法返回對應要渲染的元件, 這裡會檢查元件是否有 fetchServerData 方法,如果有就會執行它。
下面這行程式碼將服務端獲取到的資料掛載到 context 物件上,後面會把這些資料直接傳送到瀏覽器端與客戶端的vue 例項進行資料(狀態)同步。
context.state = store.state
然後我們分別配置客戶端和服務端webpack,這裡可以在我的github上fork下來參考配置,裡面每一步都有註釋,這裡不再贅述。
接著我們需要建立app.js:
import Vue from 'vue' import App from './App.vue' import store from './store' import router from './router' import { sync } from 'vuex-router-sync' import Element from 'element-ui' Vue.use(Element) // sync the router with the vuex store. // this registers `store.state.route` sync(store, router) /** * 建立vue例項 * 在這裡注入 router store 到所有的子元件 * 這樣就可以在任何地方使用 `this.$router` and `this.$store` * @type {Vue$2} */ const app = new Vue({ router, store, render: h => h(App) }) /** * 匯出 router and store. * 在這裡不需要掛載到app上。這裡和瀏覽器渲染不一樣 */ export { app, router, store }
這樣 服務端入口檔案和客戶端入口檔案便有了一個公共例項Vue, 和我們以前寫的vue例項差別不大,但是我們不會在這裡將app mount到DOM上,因為這個例項也會在服務端去執行,這裡直接將 app 暴露出去。
接下來建立路由router,建立vuex跟客戶端都差不多。詳細的可以參考我的專案...
到此,服務端渲染配置 就簡單介紹完了,下面我們啟動專案簡單的看下:
這裡跟服務端介面一樣,不一樣的是url已經不是之前的 #/而變成了請求形式 /
這樣每當瀏覽器傳送一個頁面的請求,會有伺服器渲染出一個dom字串返回,直接在瀏覽器段顯示,這樣就避免了瀏覽器端渲染的很多問題。
說起SSR,其實早在SPA (Single Page Application) 出現之前,網頁就是在服務端渲染的。伺服器接收到客戶端請求後,將資料和模板拼接成完整的頁面響應到客戶端。 客戶端直接渲染, 此時使用者希望瀏覽新的頁面,就必須重複這個過程, 重新整理頁面. 這種體驗在Web技術發展的當下是幾乎不能被接受的,於是越來越多的技術方案湧現,力求 實現無頁面重新整理或者區域性重新整理來達到優秀的互動體驗。但是SEO卻是致命的,所以一切看應用場景,這裡只為大家提供技術思路,為vue開發提供多一種可能的方案。