【安卓筆記】自定義view之組合控制元件
阿新 • • 發佈:2019-01-31
組合控制元件即將若干個系統已有的控制元件組合到一塊形成一個組合控制元件,比如帶返回按鈕的標題欄就是一個最簡單的組合控制元件。
使用組合控制元件的好處是提高程式碼的複用性,一處定義多處使用。
下面我們將使用組合控制元件實現這樣的效果:
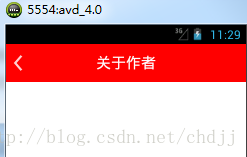
首先,我們需要自定義一個view:
首先,我們需要自定義一個view:
在這個自定義view中,我們使其載入一個我們自定義的佈局,該佈局如下:package com.example.widgets; import android.app.Activity; import android.content.Context; import android.util.AttributeSet; import android.view.LayoutInflater; import android.view.View; import android.view.View.OnClickListener; import android.widget.FrameLayout; import android.widget.ImageButton; import android.widget.TextView; import com.example.viewdemo4.R; /** * @author Rowand jj *自定義標題欄元件 */ public class TitleBar extends FrameLayout implements OnClickListener { private ImageButton ib_ret = null; private TextView tv_show = null; public TitleBar(Context context) { super(context); } public TitleBar(Context context, AttributeSet attrs) { super(context, attrs, 0); LayoutInflater inflater = LayoutInflater.from(context); inflater.inflate(R.layout.title, this);//需要指定填充的xml佈局檔案以及根檢視 ib_ret = (ImageButton) findViewById(R.id.ib_ret); tv_show = (TextView) findViewById(R.id.tv_show); ib_ret.setOnClickListener(this); } @Override public void onClick(View v) { if (this.getContext() instanceof Activity) { Activity a = (Activity) this.getContext(); a.finish(); } } public void setTitleText(CharSequence text) { this.tv_show.setText(text); } public void setLeftButListener(OnClickListener listener) { this.ib_ret.setOnClickListener(listener); } }
左側按鈕的背景是一張圖片,引用的是一個selector檔案:<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="50dp" android:background="#ff0000" > <TextView android:id="@+id/tv_show" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:textSize="20sp" android:textColor="#fff" android:text="@string/title" /> <ImageButton android:id="@+id/ib_ret" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="@drawable/title_ret_selector" android:layout_centerVertical="true" /> </RelativeLayout>
這樣,這個組合控制元件就做好了。 下面我們在activity上使用一下這個組合控制元件。 首先我們需要在佈局上指定我們定義的控制元件:<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android" > <item android:state_pressed="true" android:drawable="@drawable/navigation_previous_item"> </item> <item android:drawable="@drawable/navigation_previous_item"></item> </selector>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<com.example.widgets.TitleBar
android:id="@+id/title_bar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
>
</com.example.widgets.TitleBar>
</RelativeLayout>
就跟使用普通控制元件一樣。
下面是MainActivity:
package com.example.viewdemo4;
import android.app.Activity;
import android.os.Bundle;
import com.example.widgets.TitleBar;
public class MainActivity extends Activity
{
private TitleBar title_bar = null;
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
title_bar = (TitleBar) findViewById(R.id.title_bar);
title_bar.setTitleText("關於作者");
}
}
根據需要,我們可以修改組合控制元件顯示的文字以及左側按鈕的點選事件。