淺談快取-註解驅動的快取 Spring cache介紹
從上圖可以看出Spring Cache對快取儲存的頂層介面為Cache,快取管理的頂層介面為CacheManagerCache原始碼:package org.springframework.cache;import java.util.concurrent.Callable;/*** 定義快取通用操作的介面** @author Costin Leau* @author Juergen Hoeller* @author Stephane Nicoll* @since 3.1*/public interface Cache { /** * 返回快取的名稱 */ String getName(); /** * 返回真實的快取 */ Object getNativeCache(); /** * 返回給定key在快取中對應的value的包裝類ValueWrapper的物件 * 如何快取中不存在key的鍵值對則返回null * 如果快取中key的對應值為null,則返回一個ValueWrapper包裝類的物件 * @param key * @return * @see #get(Object, Class) */ ValueWrapper get(Object key); /** * 返回快取中key對應的value,並將其值強轉為type * @param key * @param type * @return * @throws 如果快取著找到key對應的值,但是將其轉化為指定的型別失敗將丟擲IllegalStateException * @since 4.0 * @see #get(Object) */ <T> T get(Object key, Class<T> type); /** * 如果快取中存在key對應的value則return ,如果不存在則呼叫valueLoader生成一個並將其放入快取 * @param key * @return * @throws 如果{@code valueLoader} 丟擲異常則將其包裝成ValueRetrievalException丟擲 * @since 4.3 */ <T> T get(Object key, Callable<T> valueLoader); /** *將資料對(key,value)放入快取(如果快取中存在這對資料,原來的資料會被覆蓋) * @param key * @param value */ void put(Object key, Object value); /** * 如果快取中不存在(key,value)對映對,則將其放入快取
相關推薦
淺談快取-註解驅動的快取 Spring cache介紹
在我們平常的工作當中,有好多場景需要用到快取技術,如redis,ehcache等來加速資料的訪問。作為淺談快取的第一篇筆者不想談論具體的快取技術,我更想介紹一下Spring中每次閱讀都會使我心中泛起波瀾的一個東西,那就是基於註解的快取技術。我們先看Spring參考文件中的一句
Spring cache資料(三。註釋驅動的 Spring cache 快取介紹)
概述 Spring 3.1 引入了激動人心的基於註釋(annotation)的快取(cache)技術,它本質上不是一個具體的快取實現方案(例如 EHCache 或者 OSCache),而是一個對快取使用的抽象,通過在既有程式碼中新增少量它定義的各種 annotation,即能夠達到快取
淺談圖片載入的三級快取(一)
之前被人問及過,圖片的三級快取是什麼啊,來給我講講,圖片三級快取,好高大尚的名字,聽著挺厲害,應該是很厲害的技術,當時不會啊,也就沒什麼了,沒有說出來唄,前一階端用到了BitmapUtils的圖片快取框架,索性就自己找些知識點來研究一些圖片的三級快取是什麼吧。真
淺談Oracle資料庫中的快取-Cache (IO)
Cache和Buffer是兩個不同的概念,簡單的說,Cache是加速“讀”,而buffer是緩衝“寫”,前者解決讀的問題,儲存從磁碟上讀出的資料,後者是解決寫的問題,儲存即將要寫入到磁碟上的資料。在很多情況下,這兩個名詞並沒有嚴格區分,常常把讀寫混合型別稱為buffer
快取篇(三)- Spring Cache框架
前兩篇我們講了Guava和JetCache,他們都是快取的具體實現,今天給大家分析一下Spring框架本身對這些快取具體實現的支援和融合,使用Spring Cache將大大的減少我們的Spring專案中快取使用的複雜度,提高程式碼可讀性。本文將從以下幾個方面來認識Spring Cache框架 背
淺談Linux PCI裝置驅動
轉自 http://www.uml.org.cn/embeded/201205152.asp 淺談Linux PCI裝置驅動(一) 要弄清楚Linux PCI裝置驅動,首先要明白,所謂的Linux
淺談Linux PCI裝置驅動(下)
我們在 淺談Linux PCI裝置驅動(上)中(以下簡稱 淺談(一) )介紹了PCI的配置暫存器組,而Linux PCI初始化就是使用了這些暫存器來進行的。後面我們會舉個例子來說明Linux PCI裝置驅動的主要工作內容(不是全部內容),這裡只做文字性的介紹而不會涉及具體程式碼的分析,因為要
淺談Linux PCI裝置驅動(上)
有學員建議寫寫PCI驅動,今天就找到一篇,文章很長,這基本上是全網對PCI講的比較詳細的部落格了,分成上下兩篇,這是上部分,未完待續。 要弄清楚Linux PCI裝置驅動,首先要明白,所謂的Linux PCI裝置驅動實際包括Linux PCI裝置驅動和裝置本身驅動兩部分。 不知道讀者
淺談Struts2的屬性驅動和模型驅動
一直在用Struts2實現MVC,因為相比於Struts1中大量使用request.getparameter在頁面上獲取值,struts2則提供了屬性驅動和模型驅動處理了這一問題。通過這兩個驅動,我們
使用 Spring 2.5 基於註解驅動的 Spring MVC
概述 繼 Spring 2.0 對 Spring MVC 進行重大升級後,Spring 2.5 又為 Spring MVC 引入了註解驅動功能。現在你無須讓 Controller 繼承任何介面,無需在 XML 配置檔案中定義請求和 Controller 的對映
淺談linux的LCD驅動
轉載請註明出處:http://blog.csdn.net/ruoyunliufeng/article/details/24499251 一.硬體基礎 1.硬體框圖 2.LCD控制器 瞭解硬體最直接的辦法就是看手冊,在這裡我只會簡單介紹下LCD
淺談Linux PCI裝置驅動(二)
我們在 淺談Linux PCI裝置驅動(一)中(以下簡稱 淺談(一) )介紹了PCI的配置暫存器組,而Linux PCI初始化就是使用了這些暫存器來進行的。後面我們會舉個例子來說明Linux PCI裝置驅動的主要工作內容(不是全部內容),這裡只做文字性的介紹而不會涉及具體程
淺談SpringBoot核心註解原理
SpringBoot核心註解原理 今天跟大家來探討下SpringBoot的核心註解@SpringBootApplication以及run方法,理解下springBoot為什麼不需要XML,達到零配置 首先我們先來看段程式碼 @SpringBootApplication public cla
一個快取使用的思考:Spring Cache VS Caffeine 原生 API
最近在學習本地快取發現,在 Spring 技術棧的開發中,既可以使用 Spring Cache 的註解形式操作快取,也可用各種快取方案的原生 API。那麼是否 Spring 官方提供的就是最合適的方案呢?那麼本文將通過一個案例來為你揭曉。 Spring Cache Since version 3.1, th
淺談DDD(領域驅動設計)
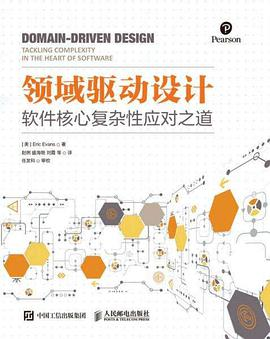 # 背景(Why) 2003 年埃裡克·埃文斯(Eric Evans)發表了《領域驅動設計》(Domain-
淺談ArcGIS GP服務 :一、框架介紹
GP全稱是Geoprocessing,可以對原有的功能進行補充,也就是說只要在桌面上實現的事情,在Server都可以實現。 首先來看一下Geoprocessing 框架,我使用的是ArcGIS10.2版本。 第一、ArcToolBox,自帶的系統的工具,只能複製和貼上,不能手工的建立。
淺談Android之Activity Decor View建立流程介紹
6 Activity DecorView建立流程介紹 上頭已經完整的介紹了Activity的啟動流程,Activity是如何繫結Window,Window的décor view是如何通過ViewRootImpl與WMS建立關聯的,也就是說,整個框架已經有了,唯一缺的就是Ac
淺談Android之Activity觸控事件傳輸機制介紹
8 Activity觸控事件傳輸機制介紹 當我們觸控式螢幕幕的時候,程式會收到對應的觸控事件,這個事件是在app端去讀取的嗎?肯定不是,如果app能讀取,那會亂套的,所以app不會有這個許可權,系統按鍵的讀取以及分發都是通過WindowManagerService來完成
淺談spring中AOP以及spring中AOP的註解方式
早就 好的 面向 XML ram ati alt 返回 增強 AOP(Aspect Oriented Programming):AOP的專業術語是"面向切面編程" 什麽是面向切面編程,我的理解就是:在不修改源代碼的情況下增強功能.好了,下面在講述aop註解方式的情況下順
淺談分散式鎖--基於快取(Redis,memcached,tair)實現篇
淺談分散式鎖--基於快取(Redis,memcached,tair)實現篇: 一、Redis分散式鎖 1、Redis實現分散式鎖的原理: 1.利用setnx命令,即只有在某個key不存在情況才能set成功該key,這樣就達到了多個程序併發去set