Stanford coursera Andrew Ng 機器學習課程程式設計作業(Exercise 1)Python3.x
阿新 • • 發佈:2019-02-14
Exercise 1:Linear Regression---實現一個線性迴歸
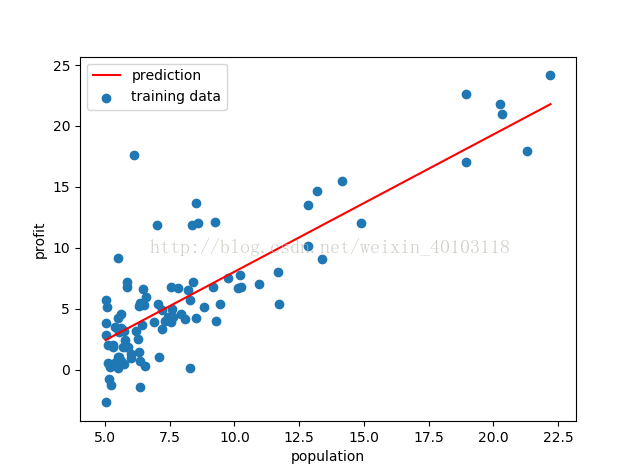
在本次練習中,需要實現一個單變數的線性迴歸。假設有一組歷史資料<城市人口,開店利潤>,現需要預測在哪個城市中開店利潤比較好?
歷史資料如下:第一列表示城市人口數,單位為萬人;第二列表示利潤,單位為10,000$
5.5277 9.1302
8.5186 13.6620
7.0032 11.8540
.....
......
程式碼
import numpy as np import pandas as pd import matplotlib.pyplot as plt # #單變數線性迴歸 # path = 'ex1data1.txt' data = pd.read_csv(path,header=None,names=['population','profit']) data.head() #檢視前5行資料(預設) ,data.head(3)則是前三行 data.describe() #得到data資料的整體概括 print(data.describe()) #新增一列 data.insert(0,'ones',1) # 就是在第一列(0) 新增名字為 ones 的一列資料,他的數值都是 1 #偏置數值x0 = 1 !!! #展示資料 data.plot(kind = 'scatter' , x = 'population' , y = 'profit' ) # 設定畫板型別,figsize是畫板大小 plt.show() def computeCost(x,y,theta): #初始化單變數線性迴歸 inner = np.power(((x*theta.T) - y),2) #power(x,2) , 就是將x數組裡面的元素都2次方 return np.sum(inner) / (2*len(x)) #初始化變數 cols = data.shape[1] x = data.iloc[:,0:cols - 1] #x是所有的行 , 去掉最後一列 y = data.iloc[:,cols - 1:cols] # y就是最後一列資料 #初始化資料 x = np.matrix(x.values) #轉化成 矩陣形式 y = np.matrix(y) theta = np.matrix(np.array([0,0])) #theta就是一個(1,2)矩陣 costFunction = computeCost(x,y,theta) print(costFunction) # # 批量梯度下降 # def gradientDescent(x,y,theta,alpha,iters): #alpha = 學習速率 ,iters = 迭代次數 temp = np.matrix(np.zeros(theta.shape)) print(temp) parameters = int(theta.ravel().shape[1]) # ravel就是合併矩陣 print(parameters) cost = np.zeros(iters) for i in range(iters): error = (x * theta.T) - y for j in range(parameters): term = np.multiply(error,x[:,j]) #multiply 對應元素乘法 temp[0,j] = theta[0,j] - ((alpha / len(x)) * np.sum(term)) theta = temp cost[i] = computeCost(x,y,theta) return theta,cost #初始化資料 alpha = 0.01 iters = 1000 gradientDescent(x,y,theta,alpha,iters) g,cost = gradientDescent(x,y,theta,alpha,iters) print(cost) print(computeCost(x,y,g)) #繪製擬合曲線 x = np.linspace(data.population.min(),data.population.max(),100) #llinspace(start,stop,num) num就是生成的樣本數 f = g[0,0] + (g[0,1] * x) #g[0,0] 代表theta0 , g[0,1] 代表theta1 fig,ax = plt.subplots() ax.plot(x,f,'r',label = 'prediction') ax.scatter(data.population,data.profit,label = 'training data') ax.legend(loc=2) ax.set_xlabel('population') ax.set_ylabel('profit') plt.show()