Android筆記三十四.Service綜合例項二
阿新 • • 發佈:2019-02-15
綜合例項2:客戶端訪問遠端Service服務
實現:通過一個按鈕來獲取遠端Service的狀態,並顯示在兩個文字框中。
思路:假設A應用需要與B應用進行通訊,呼叫B應用中的getName()、getAuthor()方法,B應用以Service方式向A應用提供服務。所以,我們可以將A應用看成是客戶端,B應用為服務端,分別命名為AILDClient、AILDServer.
一、服務端應用程式 1.src/com.example.aildserver/song.aidl:AILD檔案 當完成aidl檔案建立後,選擇儲存,eclipse會自動在專案的gen目錄中同步生成Song.java介面檔案。介面檔案中生成一個Stub抽象類,裡面包括aidl定義的方法,還包括一些其它 輔助性的方法,如geName()、getSong()方法,我們可以通過這兩個方法實現客戶端讀寫Service服務端資料。
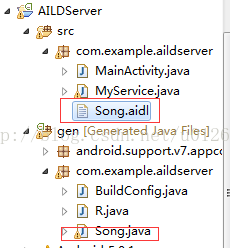
編寫Aidl檔案時,需要注意: 1.介面名和aidl檔名相同; 2.介面和方法前不用加訪問許可權修飾符public,private等,也不能用final,static;
3.Aidl預設支援的型別包話java基本型別(int、long、boolean等)和(String、List、Map、CharSequence),使用這些型別時不需要import宣告。對於List和Map中的元素型別必須是Aidl支援的型別。如果使用自定義型別作為引數或返回值,自定義型別必須實現Parcelable介面。
4.自定義型別和AIDL生成的其它介面型別在aidl描述檔案中,應該顯式import,即便在該類和定義的包在同一個包中。
5.在aidl檔案中所有非Java基本型別引數必須加上in、out、inout標記,以指明引數是輸入引數、輸出引數還是輸入輸出引數。
6.Java原始型別預設的標記為in,不能為其它標記。
2.src/com.example.aildserver/MyService.java
功能:Service子類,完成Service服務
開發核心步驟:
(1)重寫Service的onBind()方法(用於返回一個IBinder物件)、onCreate()方法、onDestroy() 方法、onUnbind()方法;
(2)定義一個Stub的子類,該內部類實現了IBinder、Song兩個介面,該子類物件將作為遠端Service的onBind()方法返回IBinder物件的代理傳給客戶端的ServiceConnection的onServiceConnected方法的第二個引數。
1.拷貝服務端.aidl檔案到客戶端 把AIDLService應用中aidl檔案所在package連同aidl檔案一起拷貝到客戶端AIDLClient應用,eclipse會自動在A應用的gen目錄中為aidl檔案同步生成Song.java介面檔案,接下來就可以在AIDLClient應用中實現與AIDLService應用通訊。 2.src/com.example.aildclient/MainActivity.java 功能:(1)啟動服務端Service服務;(2)獲取返回的IBinder代理物件,並完成與服務端程式的通訊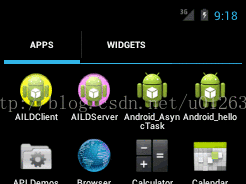
一、服務端應用程式 1.src/com.example.aildserver/song.aidl:AILD檔案 當完成aidl檔案建立後,選擇儲存,eclipse會自動在專案的gen目錄中同步生成Song.java介面檔案。介面檔案中生成一個Stub抽象類,裡面包括aidl定義的方法,還包括一些其它
- package com.example.aildserver;
- interface Song
- {
- String getName();
- String getSong();
- }
編寫Aidl檔案時,需要注意: 1.介面名和aidl檔名相同; 2.介面和方法前不用加訪問許可權修飾符public,private等,也不能用final,static;
- package com.example.aildserver;
- import com.example.aildserver.Song.Stub;
- import android.app.Service;
- import android.content.Intent;
- import android.os.Binder;
- import android.os.IBinder;
- import android.os.RemoteException;
- public class MyService extends Service {
- private String[] names = new String[] {"林俊杰","蔡依林","鄧紫棋"};
- private String[] songs = new String[] {"可惜沒如果","第三人稱","多遠都要在一起"};
- private String name,song;
- private int current=1; //當前位置
- private MyBinder binder = new MyBinder(); //例項化一個IBinder物件
- /*0.Stub內部類
- * 該內部類實現了IBinder、Song兩個介面,這個Stub類將會作為遠端Service的回撥類。*/
- public class MyBinder extends Stub
- {
- //a.客戶端回撥該方法獲取歌手名
- public String getName() throws RemoteException
- {
- return name;
- }
- //b.客戶端回撥該方法獲取歌曲
- public String getSong() throws RemoteException
- {
- return song;
- }
- }
- /*1.onBind方法
- * service用於返回一個IBinder物件給客戶端方便通訊
- */
- @Override
- public IBinder onBind(Intent arg0) {
- return binder;
- }
- /*2.onCreate方法
- * 當Service啟動後,自動呼叫該方法,用於初始化
- * */
- public void onCreate() {
- name = names[current]; //給name、song賦值
- song = songs[current];
- System.out.println("Service print:name="+name+"song="+song);
- super.onCreate();
- }
- /*3.onDestroy方法
- * 當訪問者呼叫Context.stopService方法後,呼叫該方法關閉Service服務
- * */
- public void onDestroy() {
- super.onDestroy();
- }
- /*4.onUnbind方法
- * 當訪問者調呼叫Context.unBind()方法後,呼叫該方法與Service解除繫結*/
- public boolean onUnbind(Intent intent) {
- return false;
- }
- }
- <application
- ........
- <!-- 配置service -->
- <service android:name=".MyService">
- <intent-filter>
- <action android:name="com.jiangdongguo.service"/>
- </intent-filter>
- </service>
- </application>
1.拷貝服務端.aidl檔案到客戶端 把AIDLService應用中aidl檔案所在package連同aidl檔案一起拷貝到客戶端AIDLClient應用,eclipse會自動在A應用的gen目錄中為aidl檔案同步生成Song.java介面檔案,接下來就可以在AIDLClient應用中實現與AIDLService應用通訊。 2.src/com.example.aildclient/MainActivity.java 功能:(1)啟動服務端Service服務;(2)獲取返回的IBinder代理物件,並完成與服務端程式的通訊
- package com.example.aildclient;
- import com.example.aildserver.Song;
- import android.app.Activity;
- import android.app.Service;
- import android.content.ComponentName;
- import android.content.Intent;
- import android.content.ServiceConnection;
- import android.os.Bundle;
- import android.os.IBinder;
- import android.os.RemoteException;
- import android.view.View;
- import android.view.View.OnClickListener;
- import android.widget.Button;
- import android.widget.EditText;
- public class MainActivity extends Activity {
- private Button getBtn;
- private EditText song;
- private EditText name;
- private Song binder;
- //1.建立一個ServiceConnection物件
- private ServiceConnection conn = new ServiceConnection()
- {
- public void onServiceConnected(ComponentName name, IBinder service)
- {
- binder = Song.Stub.asInterface(service); //獲取Service返回的代理IBinder物件
- }
- public void onServiceDisconnected(ComponentName name) {
- }
- };
- protected void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.main);
- getBtn=(Button)findViewById(R.id.get);
- song=(EditText)findViewById(R.id.song);
- name=(EditText)findViewById(R.id.name);
- //2.指定要啟動的Service
- Intent intent = new Intent("com.jiangdongguo.service");
- bindService(intent, conn, Service.BIND_AUTO_CREATE);
- getBtn.setOnClickListener(new OnClickListener(){
- public void onClick(View arg0)
- {
- try {
- name.setText(binder.getName());
- song.setText(binder.getSong());
- } catch (RemoteException e) {
- e.printStackTrace();
- }
- }
- });
- }
- }