Java:對double值進行四舍五入,保留兩位小數的幾種方法
阿新 • • 發佈:2019-05-07
ner 分享圖片 什麽 text 5.6 ces 技術 git app
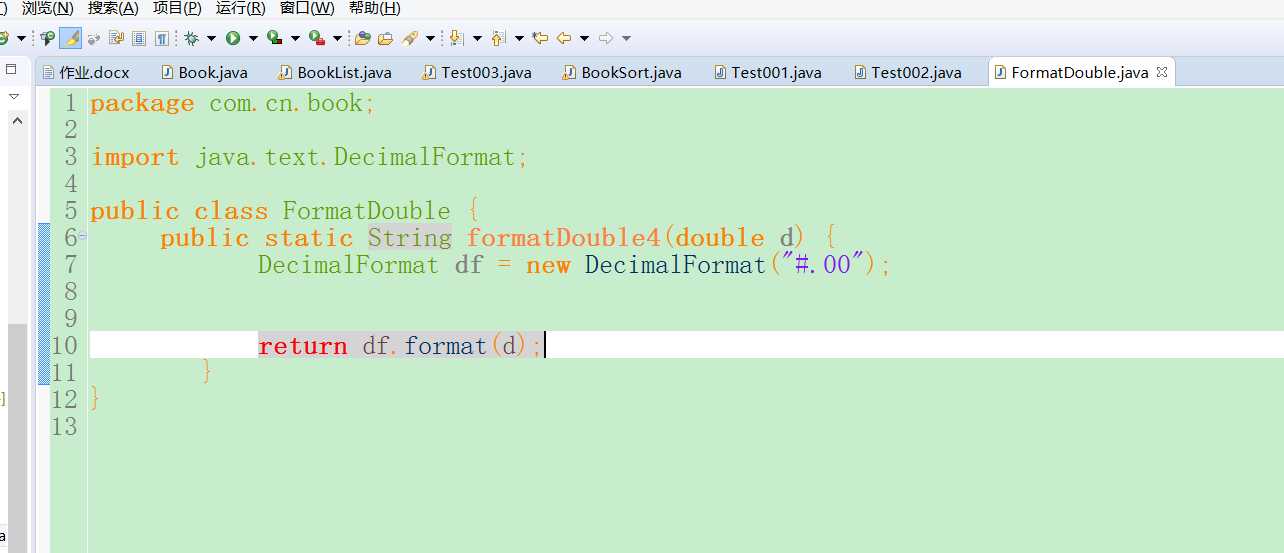
支持(0)反對(0)
回復引用
#3樓 2018-11-15 09:51 大水煮魚
BigDecimal bg = new BigDecimal(d).setScale(2, RoundingMode.UP);
這個不是傳統意義上的四舍五入,RoundingMode.HALF_UP 才是。
RoundingMode.UP: 1.111 -> 1.12,1.116 -> 1.12
RoundingMode.HALF_UP:1.111 -> 1.11,1.116 -> 1.12 支持(2)反對(0) 回復引用 #4樓 2019-04-22 17:48 古音移魂 第二種方法不是真正的四舍五入,可以用這個
轉:
1. 功能
將程序中的double值精確到小數點後兩位。可以四舍五入,也可以直接截斷。
比如:輸入12345.6789,輸出可以是12345.68也可以是12345.67。至於是否需要四舍五入,可以通過參數來決定(RoundingMode.UP/RoundingMode.DOWN等參數)。
2. 實現代碼

package com.clzhang.sample;
import java.math.BigDecimal;
import java.math.RoundingMode;
import java.text.DecimalFormat;
import java.text.NumberFormat;
public class DoubleTest {
/**
* 保留兩位小數,四舍五入的一個老土的方法
* @param d
* @return
*/
public static double formatDouble1(double d) {
return (double)Math.round(d*100)/100;
}
/**
* The BigDecimal class provides operations for arithmetic, scale manipulation, rounding, comparison, hashing, and format conversion.
* @param d
* @return
*/
public static double formatDouble2(double d) {
// 舊方法,已經不再推薦使用
// BigDecimal bg = new BigDecimal(d).setScale(2, BigDecimal.ROUND_HALF_UP);
// 新方法,如果不需要四舍五入,可以使用RoundingMode.DOWN
BigDecimal bg = new BigDecimal(d).setScale(2, RoundingMode.UP);
return bg.doubleValue();
}
/**
* NumberFormat is the abstract base class for all number formats.
* This class provides the interface for formatting and parsing numbers.
* @param d
* @return
*/
public static String formatDouble3(double d) {
NumberFormat nf = NumberFormat.getNumberInstance();
// 保留兩位小數
nf.setMaximumFractionDigits(2);
// 如果不需要四舍五入,可以使用RoundingMode.DOWN
nf.setRoundingMode(RoundingMode.UP);
return nf.format(d);
}
/**
* 這個方法挺簡單的。
* DecimalFormat is a concrete subclass of NumberFormat that formats decimal numbers.
* @param d
* @return
*/
public static String formatDouble4(double d) {
DecimalFormat df = new DecimalFormat("#.00");
return df.format(d);
}
/**
* 如果只是用於程序中的格式化數值然後輸出,那麽這個方法還是挺方便的。
* 應該是這樣使用:System.out.println(String.format("%.2f", d));
* @param d
* @return
*/
public static String formatDouble5(double d) {
return String.format("%.2f", d);
}
public static void main(String[] args) {
double d = 12345.67890;
System.out.println(formatDouble1(d));
System.out.println(formatDouble2(d));
System.out.println(formatDouble3(d));
System.out.println(formatDouble4(d));
System.out.println(formatDouble5(d));
}
}

3. 輸出
12345.68
12345.68
12,345.68
12345.68
12345.68
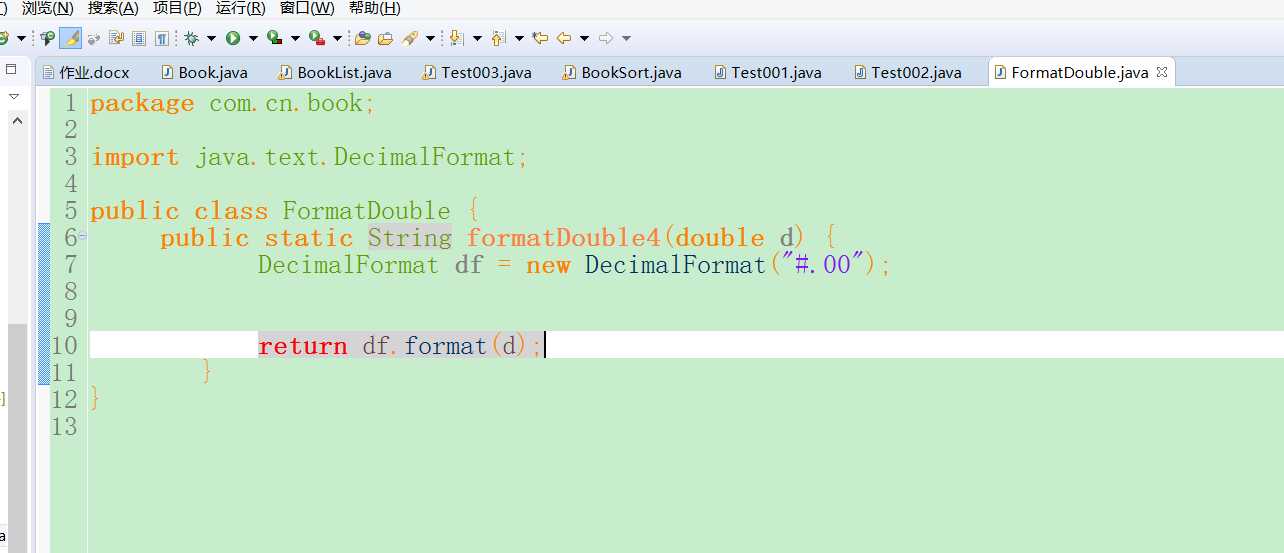
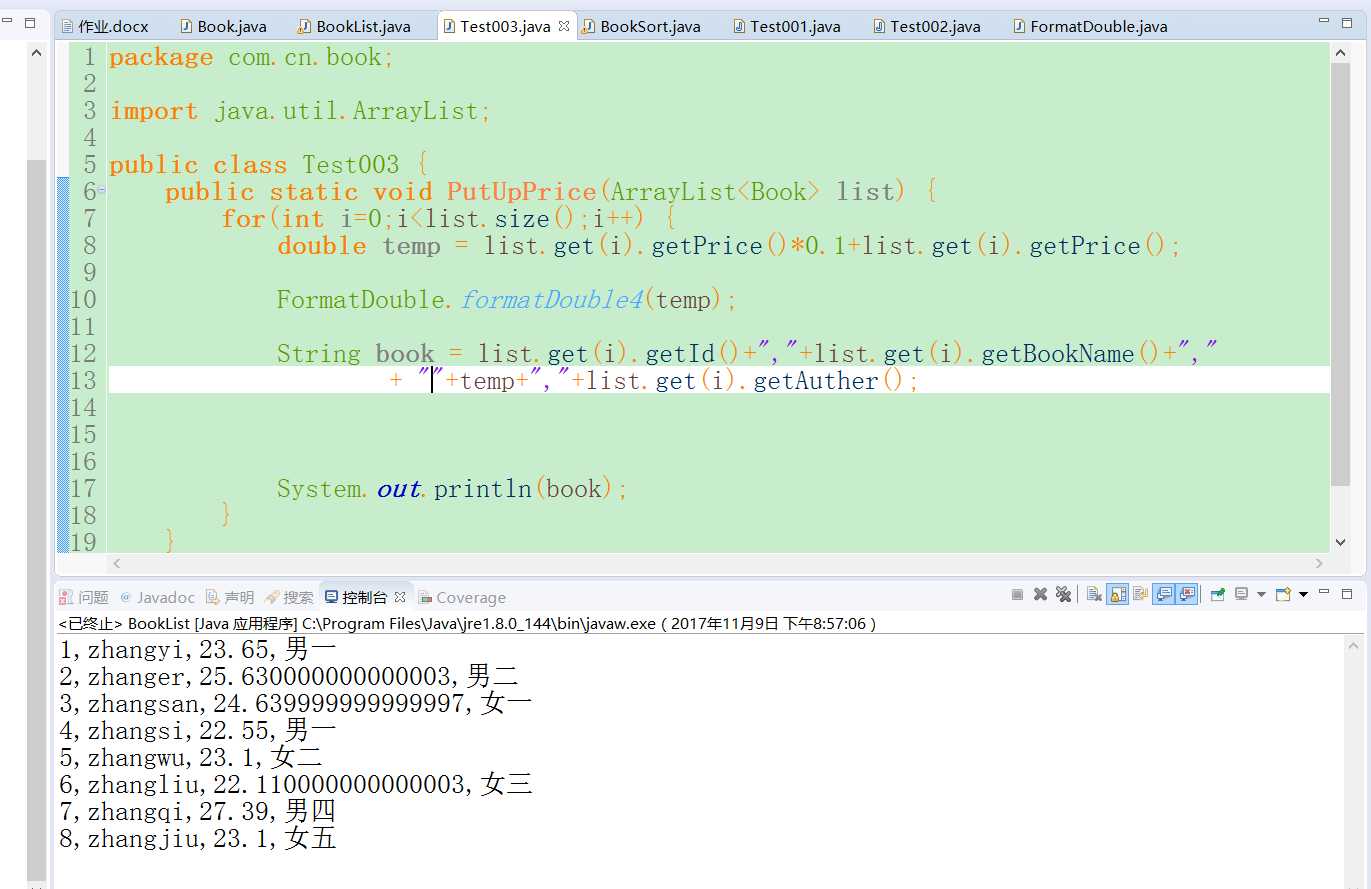
這個不是傳統意義上的四舍五入,RoundingMode.HALF_UP 才是。
RoundingMode.UP: 1.111 -> 1.12,1.116 -> 1.12
RoundingMode.HALF_UP:1.111 -> 1.11,1.116 -> 1.12 支持(2)反對(0) 回復引用 #4樓 2019-04-22 17:48 古音移魂 第二種方法不是真正的四舍五入,可以用這個
1 2 3 4 5 6 |
public static double doubleBitUp( double d, int bit) {
if (d == 0.0 )
return d;
double pow = Math.pow( 10 , bit);
return ( double )Math.round(d*pow)/pow;
}
|
Java:對double值進行四舍五入,保留兩位小數的幾種方法