C++ STL迭代器原理和簡單實現
阿新 • • 發佈:2020-03-14
### 1. 迭代器簡介
為了提高C++程式設計的效率,STL(Standard Template Library)中提供了許多容器,包括vector、list、map、set等。然而有些容器(vector)可以通過下標索引的方式訪問容器裡面的資料,但是大部分的容器(list、map、set)不能使用這種方式訪問容器中的元素。**為了統一訪問不同容器時的訪問方式**,STL為每種容器在實現的時候設計了一個內嵌的**iterator類**,不同的容器有自己專屬的迭代器(專屬迭代器負責實現對應容器訪問元素的具體細節),使用迭代器來訪問容器中的資料。除此之外,**通過迭代器可以將容器和通用演算法結合在一起**,只要給予演算法不同的迭代器,就可以對不同容器執行相同的操作,例如find查詢函式(因為迭代器提供了統一的訪問方式,這是使用迭代器帶來的好處)。迭代器對一些基本操作如\*、->、++、==、!=、=進行了過載,使其具有了遍歷複雜資料結構的能力,其遍歷機制取決於所遍歷的容器,所有迭代器的使用和指標的使用非常相似。通過begin,end函式獲取容器的頭部和尾部迭代器,**end迭代器**不包含在容器之內,**當begin和end返回的迭代器相同時表示容器為空。**
> STL主要由 **容器、迭代器、演算法、函式物件、和記憶體分配器** 五大部分構成。
### 2. 迭代器的實現原理
首先,看看STL中迭代器的實現思路:
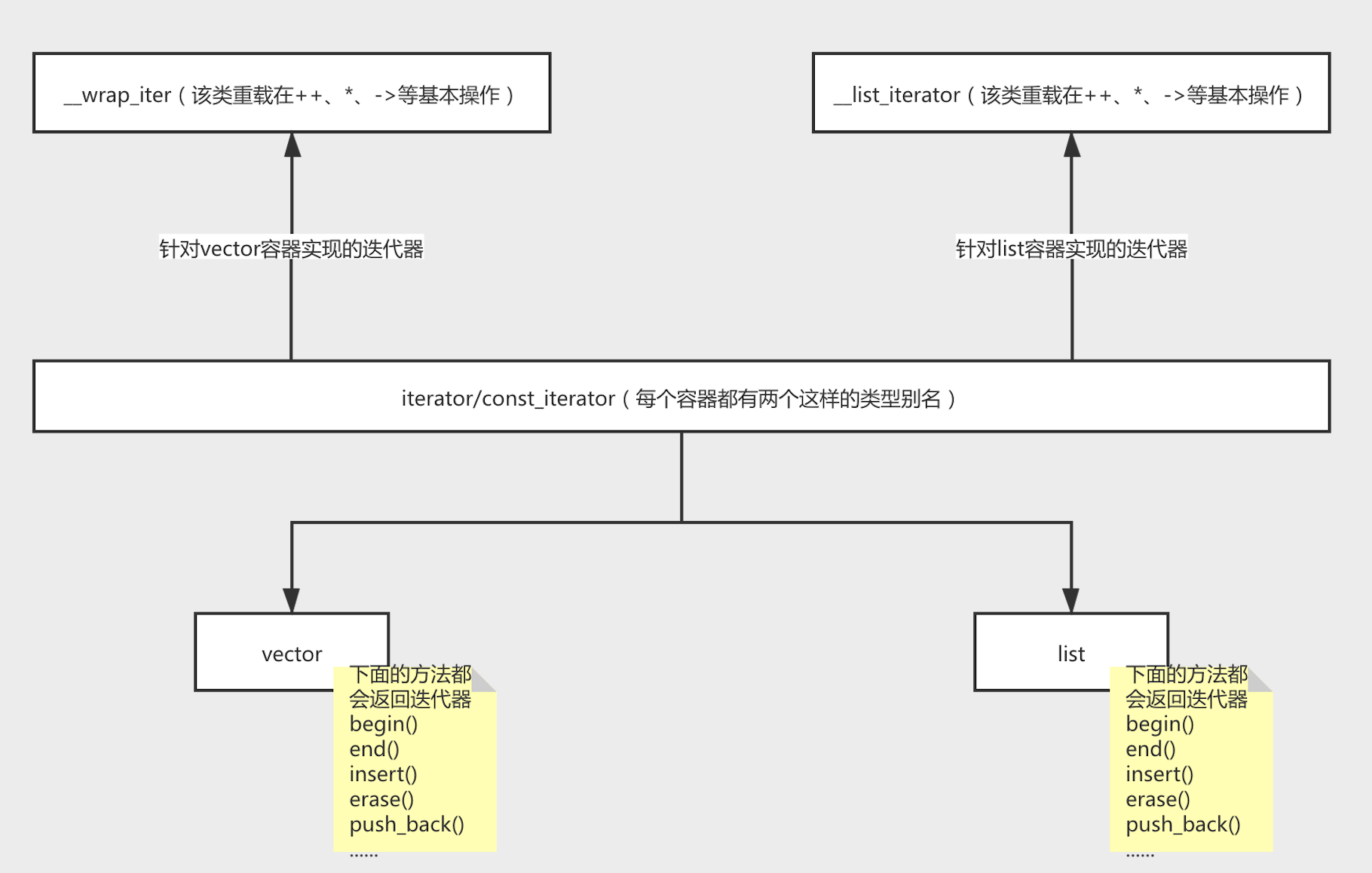
從上圖中可以看出,STL通過類型別名的方式實現了對外統一;在不同的容器中類型別名的真實迭代器型別是不一樣的,而且真實迭代器型別對於++、--、\*、->等基本操作的實現方式也是不同的。(PS:迭代器很好地詮釋了介面與實現分離的意義)
既然我們已經知道了迭代器的實現思路,現在如果讓我們自己設計一個list容器的簡單迭代器,應該如何實現呢?
1. list類需要有操作迭代器的方法
1. begin/end
2. insert/erase/emplace
2. list類有一個內部類list_iterator
1. 有一個成員變數ptr指向list容器中的某個元素
2. iterator負責過載++、--、\*、->等基本操作
3. list類定義內部類list_iterator的類型別名
以上就是實現一個list容器的簡單迭代器需要考慮的具體細節。
### 3. 迭代器的簡單實現
my_list.h(**重要部分有註釋說明**)
```C++
//
// Created by wengle on 2020-03-14.
//
#ifndef CPP_PRIMER_MY_LIST_H
#define CPP_PRIMER_MY_LIST_H
#include
template
class node {
public:
T value;
node *next;
node() : next(nullptr) {}
node(T val, node *p = nullptr) : value(val), next(p) {}
};
template
class my_list {
private:
node *head;
node *tail;
int size;
private:
class list_iterator {
private:
node *ptr; //指向list容器中的某個元素的指標
public:
list_iterator(node *p = nullptr) : ptr(p) {}
//過載++、--、*、->等基本操作
//返回引用,方便通過*it來修改物件
T &operator*() const {
return ptr->value;
}
node *operator->() const {
return ptr;
}
list_iterator &operator++() {
ptr = ptr->next;
return *this;
}
list_iterator operator++(int) {
node *tmp = ptr;
// this 是指向list_iterator的常量指標,因此*this就是list_iterator物件,前置++已經被過載過
++(*this);
return list_iterator(tmp);
}
bool operator==(const list_iterator &t) const {
return t.ptr == this->ptr;
}
bool operator!=(const list_iterator &t) const {
return t.ptr != this->ptr;
}
};
public:
typedef list_iterator iterator; //類型別名
my_list() {
head = nullptr;
tail = nullptr;
size = 0;
}
//從連結串列尾部插入元素
void push_back(const T &value) {
if (head == nullptr) {
head = new node(value);
tail = head;
} else {
tail->next = new node(value);
tail = tail->next;
}
size++;
}
//列印連結串列元素
void print(std::ostream &os = std::cout) const {
for (node *ptr = head; ptr != tail->next; ptr = ptr->next)
os << ptr->value << std::endl;
}
public:
//操作迭代器的方法
//返回連結串列頭部指標
iterator begin() const {
return list_iterator(head);
}
//返回連結串列尾部指標
iterator end() const {
return list_iterator(tail-> next);
}
//其它成員函式 insert/erase/emplace
};
#endif //CPP_PRIMER_MY_LIST_H
```
test.cpp
```C++
//
// Created by wengle on 2020-03-14.
//
#include
#include "my_list.h"
struct student {
std::string name;
int age;
student(std::string n, int a) : name(n), age(a) {}
//過載輸出操作符
friend std::ostream &operator<<(std::ostream &os, const student &stu) {
os << stu.name << " " << stu.age;
return os;
}
};
int main() {
my_list l;
l.push_back(student("bob", 1)); //臨時量作為實參傳遞給push_back方法
l.push_back(student("allen", 2));
l.push_back(student("anna", 3));
l.print();
for (my_list::iterator it = l.begin(); it != l.end(); it++) {
std::cout << *it << std::endl;
*it = student("wengle", 18);
}
return 0;
}
```
### 4. 迭代器失效
```C++
// inserting into a vector
#include
#include
int main ()
{
std::vector myvector (3,100);
std::vector::iterator it;
it = myvector.begin();
it = myvector.insert ( it , 200 );
myvector.insert (it,200,300);
//it = myvector.insert (it,200,300);
myvector.insert (it,5,500); //當程式執行到這裡時,大概率會crash
for (std::vector::iterator it2=myvector.begin(); it2