使用PInvoke互操作,讓C#和C++愉快的互動優勢互補
阿新 • • 發佈:2020-05-29
## 一:背景
### 1. 講故事
如果你常翻看FCL的原始碼,你會發現這裡面有不少方法藉助了C/C++的力量讓C#更快更強悍,如下所示:
``` C#
[DllImport("QCall", CharSet = CharSet.Unicode)]
[SecurityCritical]
[SuppressUnmanagedCodeSecurity]
private static extern bool InternalUseRandomizedHashing();
[DllImport("mscoree.dll", EntryPoint = "ND_RU1")]
[SuppressUnmanagedCodeSecurity]
[SecurityCritical]
public static extern byte ReadByte([In] [MarshalAs(UnmanagedType.AsAny)] object ptr, int ofs);
```
聯想到上一篇阿里簡訊netsdk也是全用C++實現,然後用C#做一層殼,兩者相互打輔助彰顯更強大的威力,還有很多做物聯網的朋友對這種.Net互操作技術太熟悉不過了,很多硬體,視訊裝置驅動都是用C/C++實現,然後用winform/WPF去做管理介面,C++還是在大學裡學過,好多年沒接觸了,為了練手這一篇用P/Invoke來將兩者相互打通。
## 二:PInvoke互操作技術
### 1. 一些前置基礎
這裡我用vs2019建立C++的Console App,修改兩個配置: 將程式匯出為dll,修改成compile方式為`Compile as C++ Code (/TP)`。
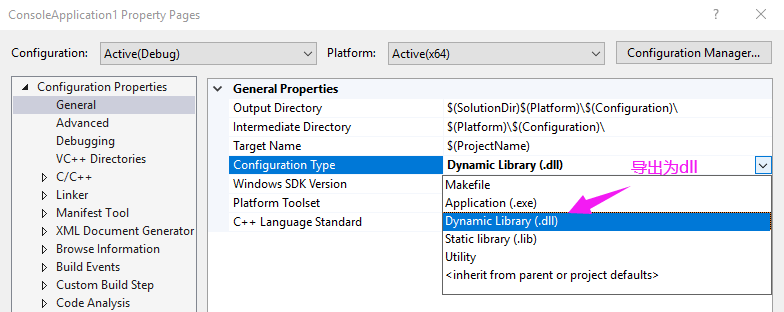
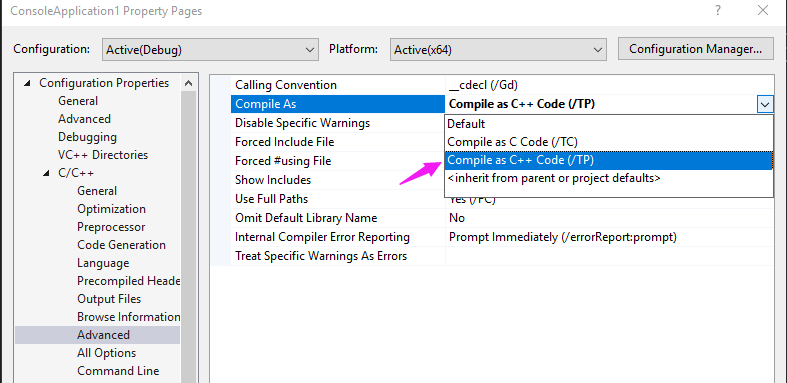
### 2. 基本型別的互操作
簡單型別是最好處理的,基本上int,long,double都是一一對應的,這裡我用C++實現了簡單的Sum操作,畫一個簡圖就是下面這樣:
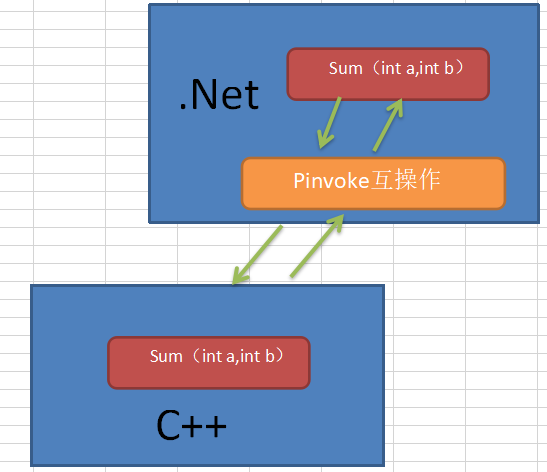
新建一個cpp檔案和一個h標頭檔案,如下程式碼。
``` C++
--- Person.cpp
extern "C"
{
_declspec(dllexport) int Sum(int a, int b);
}
--- Person.h
#include "Person.h"
#include "iostream"
using namespace std;
int Sum(int a, int b)
{
return a + b;
}
```
有一個注意的地方就是 `extern "C"`,一定要用C方式匯出,如果按照C++方式,Sum名稱會被編譯器自動修改,不信你把`extern "C"`去掉,我用ida開啟給你看一下,被修改成了 `?Sum@@YAHHH@Z`, 尷尬。
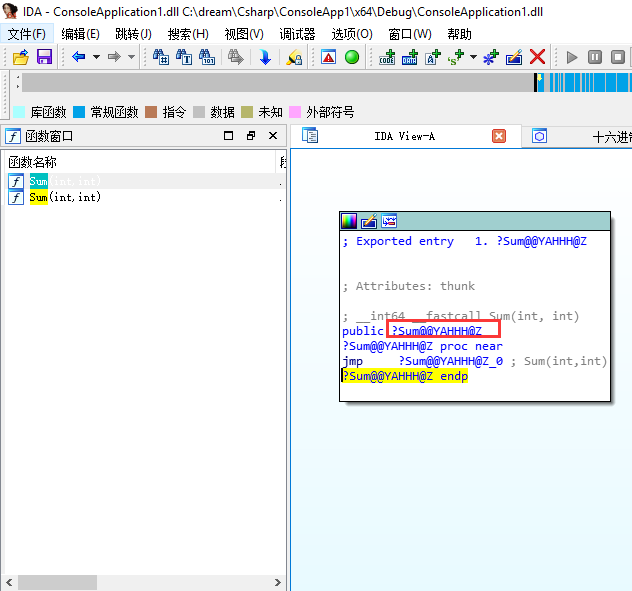
接下來把C++專案生成好的 `ConsoleApplication1.dll` copy到C#的bin目錄下,程式碼如下:
``` C#
class Program
{
[DllImport("ConsoleApplication1.dll", CallingConvention = CallingConvention.Cdecl)]
extern static int Sum(int a, int b);
static void Main(string[] args)
{
var result = Sum(10, 20);
Console.WriteLine($"10+20={result}");
Console.ReadLine();
}
}
---- output -----
10+20=30
```
### 2. 字串的互操作
我們知道託管程式碼和非託管程式碼是兩個世界,這中間涉及到了兩個世界的的型別對映,那對映關係去哪找呢? 微軟的msdn還真有一篇介紹 封送通用型別對照表: https://docs.microsoft.com/zh-cn/dotnet/standard/native-interop/type-marshaling ,大家有興趣可以看一下。

從圖中可以看到,C#中的string對應C++中的char*,所以這裡就好處理了。
``` C++
--- Person.cpp
extern "C"
{
//字串
_declspec(dllexport) int GetLength(char* chs);
}
--- Person.h
#include "Person.h"
#include "iostream"
using namespace std;
int GetLength(char* chs)
{
return strlen(chs);
}
```
然後我們看一下C#這邊怎麼寫,通常string在C++中使用asc碼,而C#中是Unicode,所以在DllImport中加一個CharSet指定即可。
``` C#
class Program
{
[DllImport("ConsoleApplication1.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi)]
extern static int GetLength([MarshalAs(UnmanagedType.LPStr)] string str);
static void Main(string[] args)
{
var str = "hello world";
Console.WriteLine($"length={GetLength(str)}");
Console.ReadLine();
}
}
---- output -----
length=11
```
### 3. 複雜型別的處理
複雜型別配置對應關係就難搞了,還容易搞錯,錯了弄不好還記憶體洩漏,怕了吧,幸好微軟提供了一個小工具`P/Invoke Interop Assistant `,它可以幫助我們自動匹配對應關係,我就演示一個封送Person類的例子。
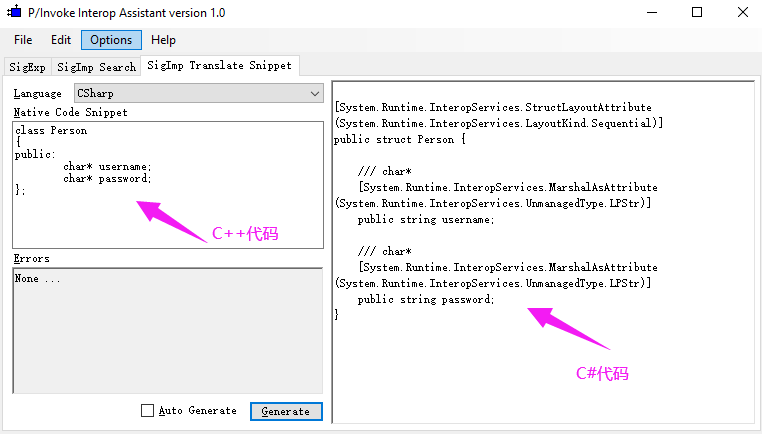
從圖中可以看到,左邊寫好 `C++`,右邊自動給你配好`C#`的對映型別,非常方便。
``` C++
--- Person.cpp
extern "C"
{
class Person
{
public:
char* username;
char* password;
};
_declspec(dllexport) char* AddPerson(Person person);
}
--- Person.h
#include "Person.h"
#include "iostream"
using namespace std;
char* AddPerson(Person person)
{
return person.username;
}
```
可以看到C++中AddPerson返回了char*,在C#中我們用IntPtr來接,然後用Marshal將指標轉換string,接下來用工具生成好的C#程式碼拷到專案中來,如下:
``` C#
[System.Runtime.InteropServices.StructLayoutAttribute(System.Runtime.InteropServices.LayoutKind.Sequential)]
public struct Person
{
/// char*
[System.Runtime.InteropServices.MarshalAsAttribute(System.Runtime.InteropServices.UnmanagedType.LPStr)]
public string username;
/// char*
[System.Runtime.InteropServices.MarshalAsAttribute(System.Runtime.InteropServices.UnmanagedType.LPStr)]
public string password;
}
class Program
{
[DllImport("ConsoleApplication1.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi)]
extern static IntPtr AddPerson(Person person);
static void Main(string[] args)
{
var person = new Person() { username = "dotnetfly", password = "123456" };
var ptr = AddPerson(person);
var str = Marshal.PtrToStringAnsi(ptr);
Console.WriteLine($"username={str}");
Console.ReadLine();
}
}
---------- output ------------
username=dotnetfly
```
### 4. 回撥函式(非同步)的處理
前面介紹的3種情況都是單向的,即C#向C++傳遞資料,有的時候也需要C++主動呼叫C#的函式,我們知道C#是用回撥函式,也就是委託包裝,具體我就不說了,很開心的是C++可以直接接你的委託,看下怎麼實現。
``` C++
--- Person.cpp
extern "C"
{
//函式指標
typedef void(_stdcall* PCALLBACK) (int result);
_declspec(dllexport) void AsyncProcess(PCALLBACK ptr);
}
--- Person.h
#include "Person.h"
#include "iostream"
using namespace std;
void AsyncProcess(PCALLBACK ptr)
{
ptr(10); //回撥C#的委託
}
```
從程式碼中看到,PCALLBACK就是我定義了函式指標,接受int引數。
``` C#
class Program
{
delegate void Callback(int a);
[DllImport("ConsoleApplication1.dll", CallingConvention = CallingConvention.Cdecl)]
extern static void AsyncProcess(Callback callback);
static void Main(string[] args)
{
AsyncProcess((i) =>
{
//這裡回撥函式哦...
Console.WriteLine($"這是回撥函式哦: {i}");
});
Console.ReadLine();
}
}
------- output -------
這是回撥函式哦: 10
```
這裡我做了一個自定義的delegate,因為我使用`Action`不接受泛型拋異常(┬_┬)。
## 四:總結
這讓我想起來前段時間用python實現的線性迴歸,為了簡便我使用了http和C#互動,這次準備用C++改寫然後PInvoke直接互動就利索了,好了,藉助C++的生態,讓 C# 如虎添翼吧~~~
---
### 如您有更多問題與我互動,掃描下方進來吧~
---
