SpringBoot2.x入門:引入web模組
阿新 • • 發佈:2020-07-04
## 前提
這篇文章是《SpringBoot2.x入門》專輯的**第3篇**文章,使用的`SpringBoot`版本為`2.3.1.RELEASE`,`JDK`版本為`1.8`。
主要介紹`SpringBoot`的`web`模組引入,會相對詳細地分析不同的`Servlet`容器(如`Tomcat`、`Jetty`等)的切換,以及該模組提供的`SpringMVC`相關功能的使用。
## 依賴引入
筆者新建了一個多模組的`Maven`專案,這次的示例是子模組`ch1-web-module`。
`SpringBoot`的`web`模組實際上就是`spring-boot-starter-web`元件(下稱`web`模組),前面的文章介紹過使用`BOM`全域性管理版本,可以在(父)`POM`檔案中新增`dependencyManagement`元素:
```xml
2.3.1.RELEASE
org.springframework.boot
spring-boot-dependencies
${spring.boot.version}
import
pom
```
接來下在(子)`POM`檔案中的`dependencies`元素引入`spring-boot-starter-web`的依賴:
```xml
org.springframework.boot
spring-boot-starter-web
```
專案的子`POM`大致如下:
```xml
4.0.0
club.throwable
spring-boot-guide
1.0-SNAPSHOT
ch1-web-module
1.0-SNAPSHOT
jar
ch1-web-module
org.springframework.boot
spring-boot-starter-web
ch1-web-module
org.springframework.boot
spring-boot-maven-plugin
${spring.boot.version}
repackage
```
`spring-boot-starter-web`模組中預設使用的`Servlet`容器是嵌入式(`Embedded`)`Tomcat`,配合打包成一個`Jar`包以便可以直接使用`java -jar xxx.jar`命令啟動。
## SpringMVC的常用註解
`web`模組整合和擴充套件了`SpringMVC`的功能,移除但相容相對臃腫的`XML`配置,這裡簡單列舉幾個常用的`Spring`或者`SpringMVC`提供的註解,簡單描述各個註解的功能:
**元件註解:**
- `@Component`:標記一個類為`Spring`元件,掃描階段註冊到`IOC`容器。
- `@Repository`:標記一個類為`Repository`(倉庫)元件,它的元註解為`@Component`,一般用於`DAO`層。
- `@Service`:標記一個類為`Service`(服務)元件,它的元註解為`@Component`。
- `@Controller`:標記一個類為`Controller`(控制器)元件,它的元註解為`@Component`,一般控制器是訪問的入口,衍生註解`@RestController`,簡單理解為`@Controller`標記的控制器內所有方法都加上下面提到的`@ResponseBody`。
**引數註解:**
- `@RequestMapping`:設定對映引數,包括請求方法、請求的路徑、接收或者響應的內容型別等等,衍生註解為`GetMapping`、`PostMapping`、`PutMapping`、`DeleteMapping`、`PatchMapping`。
- `@RequestParam`:宣告一個方法引數繫結到一個請求引數。
- `@RequestBody`:宣告一個方法引數繫結到請求體,常用於內容型別為`application/json`的請求體接收。
- `@ResponseBody`:宣告一個方法返回值繫結到響應體。
- `@PathVariable`:宣告一個方法引數繫結到一個`URI`模板變數,用於提取當前請求`URI`中的部分到方法引數中。
## 編寫控制器和啟動類
在專案中編寫一個控制器`club.throwable.ch1.controller.HelloController`如下:
```java
import lombok.extern.slf4j.Slf4j;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import java.util.Optional;
@Slf4j
@Controller
@RequestMapping(path = "/ch1")
public class HelloController {
@RequestMapping(path = "/hello")
public ResponseEntity hello(@RequestParam(name = "name") String name) {
String value = String.format("[%s] say hello", name);
log.info("呼叫[/hello]介面,引數:{},響應結果:{}", name, value);
return ResponseEntity.of(Optional.of(value));
}
}
```
`HelloController`只提供了一個接收`GET`請求且請求的路徑為`/ch1/hello`的方法,它接收一個名稱為`name`的引數(引數必傳),然後返回簡單的文字:`${name} say hello`。可以使用衍生註解簡化如下:
```java
import lombok.extern.slf4j.Slf4j;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.util.Optional;
@Slf4j
@RestController
@RequestMapping(path = "/ch1")
public class HelloController {
@GetMapping(path = "/hello")
public ResponseEntity hello(@RequestParam(name = "name") String name) {
String value = String.format("[%s] say hello", name);
log.info("呼叫[/hello]介面,引數:{},響應結果:{}", name, value);
return ResponseEntity.of(Optional.of(value));
}
}
```
接著編寫一個啟動類`club.throwable.ch1.Ch1Application`,啟動類是`SpringBoot`應用程式的入口,需要提供一個`main`方法:
```java
package club.throwable.ch1;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Ch1Application {
public static void main(String[] args) {
SpringApplication.run(Ch1Application.class, args);
}
}
```
然後以`DEBUG`模式啟動一下:
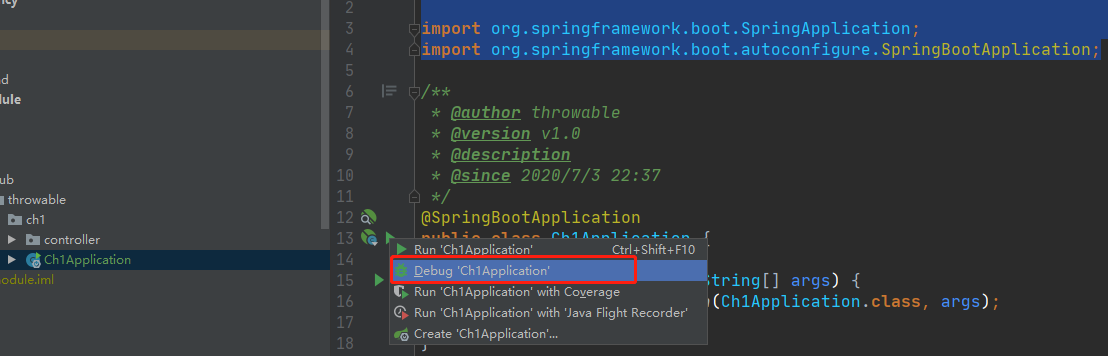
`Tomcat`預設的啟動埠是`8080`,啟動完畢後見日誌如下:
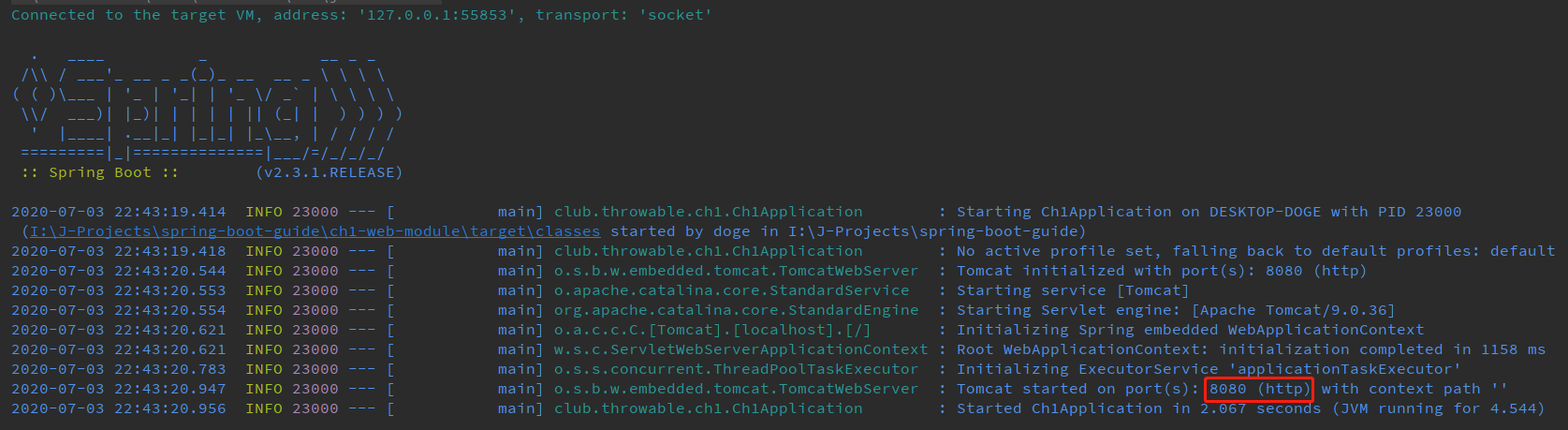
用用瀏覽器訪問`http://localhost:8080/ch1/hello?name=thrwoable`可見輸出如下:
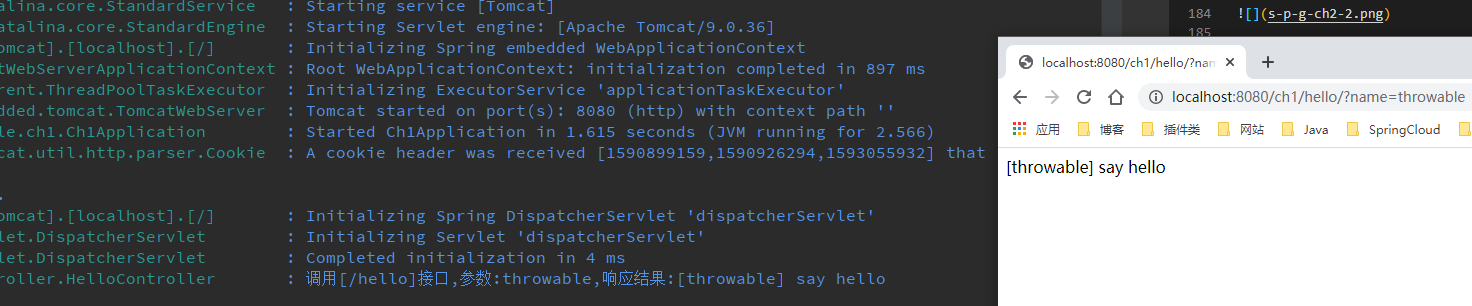
至此,一個簡單的基於`spring-boot-starter-web`開發的`web`應用已經完成。
## 切換Servlet容器
有些時候由於專案需要、運維規範或者個人喜好,並不一定強制要求使用`Tomcat`作為`Servlet`容器,常見的其他選擇有`Jetty`、`Undertow`,甚至`Netty`等。以`Jetty`和`Undertow`為例,切換為其他嵌入式`Servlet`容器需要從`spring-boot-starter-web`中排除`Tomcat`的依賴,然後引入對應的`Servlet`容器封裝好的`starter`。
**切換為`Jetty`**,修改`POM`檔案中的`dependencies`元素:
```xml
org.springframework.boot
spring-boot-starter-web
org.springframework.boot
spring-boot-starter-tomcat
org.springframework.boot
spring‐boot‐starter‐jetty
```

**切換為`Undertow`**,修改`POM`檔案中的`dependencies`元素:
```xml
org.springframework.boot
spring-boot-starter-web
org.springframework.boot
spring-boot-starter-tomcat
org.springframework.boot
spring‐boot‐starter‐undertow
```

## 小結
這篇文章主要分析瞭如何基於`SpringBoot`搭建一個入門的`web`服務,還簡單介紹了一些常用的`SpringMVC`註解的功能,最後講解如何基於`spring-boot-starter-web`切換底層的`Servlet`容器。學會搭建`MVC`應用後,就可以著手嘗試不同的請求方法或者引數,嘗試常用註解的功能。
## 程式碼倉庫
這裡給出本文搭建的`web`模組的`SpringBoot`應用的倉庫地址(持續更新):
- Github:https://github.com/zjcscut/spring-boot-guide/tree/master/ch1-web-module
(本文完 c-2-d e-a-20200703 23:09 PM)
技術公眾號《Throwable文摘》(id:throwable-doge),不定期推送筆者原創技術文章(絕不抄襲或者轉載):
![](https://public-1256189093.cos.ap-guangzhou.myqcloud.com/static/wechat-account-l