用c#自己實現一個簡單的JSON解析器
阿新 • • 發佈:2020-07-20
## 一、JSON格式介紹
* JSON(JavaScript Object Notation) 是一種輕量級的資料交換格式。相對於另一種資料交換格式 XML,JSON 有著很多優點。例如易讀性更好,佔用空間更少等。在 web 應用開發領域內,得益於 JavaScript 對 JSON 提供的良好支援,JSON 要比 XML 更受開發人員青睞。所以作為開發人員,如果有興趣的話,還是應該深入瞭解一下 JSON 相關的知識。本著探究 JSON 原理的目的,我將會在這篇文章中詳細向大家介紹一個簡單的JSON解析器的解析流程和實現細節。由於 JSON 本身比較簡單,解析起來也並不複雜。所以如果大家感興趣的話,在看完本文後,不妨自己動手實現一個 JSON 解析器。好了,其他的話就不多說了,接下來讓我們移步到重點章節吧。
* [線上JOSN校驗格式化工具](http://lzltool.com/Json) 如果在解析字串的時候,拿不準這個是不是正確的JOSN,你可以在這個上面測試一下,有利於對自己程式碼的測試
## 二、解析原理介紹
- 解析物件`{}`
* 物件結構是`{"Key":[值]}`的格式,所以先解析到Key字串,將Key解析出來,然後在解析到值,因為值有可能是【`字串`、`值型別`、`布林型別`、`物件`、`陣列`、`null`】所以需要根據字首得到型別,並呼叫相應的解析方法,迴圈解析到“}”物件結尾
- 解析陣列`[]`
* 物件的結構是`[[值],[值]]`,因為值有可能是【`字串`、`值型別`、`布林型別`、`物件`、`陣列`、`null`】所以需要根據字首得到型別,並呼叫相應的解析方法,迴圈解析到`]`陣列結尾
- 解析字串
* 迴圈解析,需要判斷是否遇到轉義符`\`如果遇到,當前字元的下一個字元將是作為普通字元存入結果,如果遇到非轉義的 `"` 字元則退出字串讀取方法,並返回結果
- 解析值型別
* 迴圈拉取`[0-9]`包括`.`符號,然後呼叫轉換成double型別方法
- 解析布林型別
* 轉判斷是 `true` 還是 `false`
- 解析`null`
* 轉判斷是否為 `null`
### 解析元素流程圖
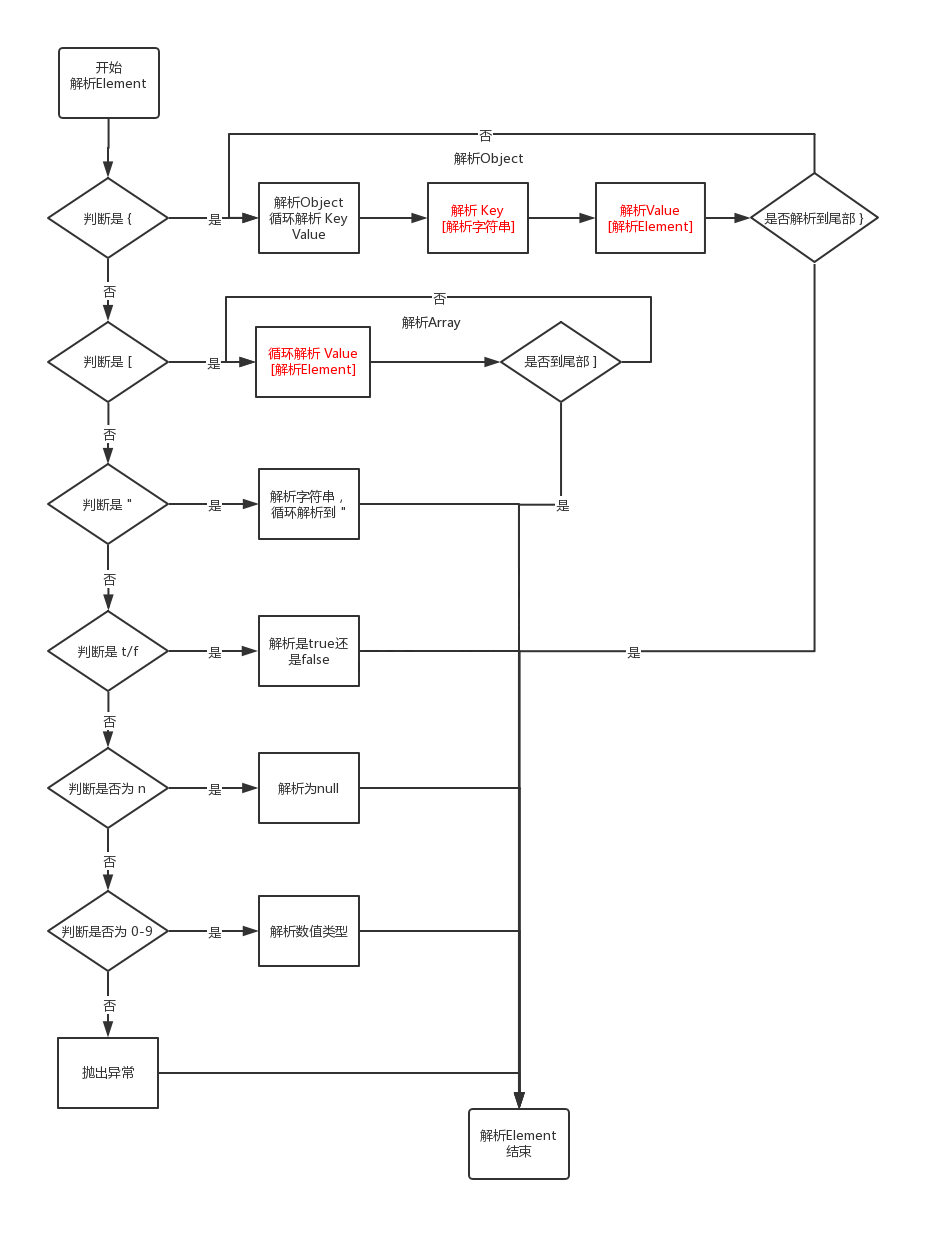
### 解析方法列表
方法名| 方法作用
:-------- | :-----
`AnalysisJson` | 解析JSON字串為C#資料結構
`AnalysisJsonObject` | 解析JSON字串為物件結構
`AnalysisJsonArray` | 解析JSON字串為陣列結構
`ReadElement` | 讀取出一個JSON結構
`ReadJsonNumber` | 讀取出一個值型別結構
`ReadJsonNull` | 讀取出一個`null`結構
`ReadJsonFalse` | 讀取出一個`false`結構
`ReadJsonTrue` | 讀取出一個`true`結構
`ReadString` | 讀取出一個字串結構
`ReadToNonBlankIndex` | 讀取到非空白字元下標位置
### 例1 解析JSON
```javascript
{"Name":"張三","Age":18}
```
- 1.解析第一個字元`{`發現是JSON物件結構,呼叫`AnalysisJsonObject`方法來解析JSON物件格式
- 2.解析物件的方法開始迴圈解析 Key-Value結構直到`}`物件尾部字元
* 先解析Key結構呼叫 `ReadString`來進行解析出Key字串從而得到`Name`這個值
* 然後解析Value因為值可能是任意結構所以呼叫`ReadElement`來解析出一個JSON結構
+ 讀取第一個字元得到`"`從而知道這個Value是一個字串,呼叫方法`ReadString`來讀取到這個Value的值`張三`
* 讀取下一個字元發現不是JSON物件的結尾字元`}`是`,`字元代表下面還存在一個Key-Value結構,繼續讀取
* 先解析Key結構呼叫 `ReadString`來進行解析出Key字串從而得到`Age`這個值
* 然後解析Value因為值可能是任意結構所以呼叫`ReadElement`來解析出一個JSON結構
+ 讀取第一個字元發現是`1`是數字,代表下面的這個結構是數值型別呼叫方法`ReadJsonNumber`來讀取數值型別
* 讀取下一個字元發現是`}`是JSON物件的結尾字元,退出JSON物件解析,返回解析的JSON物件結構例項
### 例2 解析JSON
```javascript
[{"科目":"語文","成績":99}]
```
- 1.解析第一個字元`[`發現是JSON陣列結構,呼叫方法`AnalysisJsonArray`方法來解析出JSON陣列結構
* 解析迴圈解析JSON資料結構直到遇到`]`陣列結構結尾字元
+ 因為陣列中每個元素都是可能是任意型別資料,所以呼叫`ReadElement`方法來解析值
+ 讀取值的第一個字元`{`發現是JSON物件型別呼叫`AnalysisJsonObject`方法解析JSON物件
+ 先解析Key結構呼叫 `ReadString`來進行解析出Key字串從而得到`科目`這個值
+ 然後解析Value因為值可能是任意結構所以呼叫`ReadElement`來解析出一個JSON結構
+ 讀取第一個字元得到`"`從而知道這個Value是一個字串,呼叫方法`ReadString`來讀取到這個Value的值`語文`
+ 讀取下一個字元發現不是JSON物件的結尾字元`}`是`,`字元代表下面還存在一個Key-Value結構,繼續讀取
+ 先解析Key結構呼叫 `ReadString`來進行解析出Key字串從而得到`成績`這個值
+ 然後解析Value因為值可能是任意結構所以呼叫`ReadElement`來解析出一個JSON結構
+ 讀取第一個字元發現是`9`是數字,代表下面的這個結構是數值型別呼叫方法`ReadJsonNumber`來讀取數值型別
+ 讀取下一個字元發現是`}`是JSON物件的結尾字元,退出JSON物件解析,返回解析的JSON物件結構例項
+ 讀取下一個字元發現是`]`JSON陣列的結尾,退出解析JSON陣列,返回解析的JSON陣列結構例項
## 三、程式碼實現
```csharp
///
/// JSON解析型別
///
public static class JsonConvert
{
///
/// 解析JSON
///
/// 待解析的JSON字串
///
public static JsonElement AnalysisJson(string text)
{
var index = 0;
//讀取到非空白字元
ReadToNonBlankIndex(text, ref index);
if (text[index++] == '[')
//解析陣列
return AnalysisJsonArray(text, ref index);
//解析物件
return AnalysisJsonObject(text, ref index);
}
///
/// 解析JSON物件
///
/// JSON字串
/// 開始索引位置
///
private static JsonObject AnalysisJsonObject(string text, ref int index)
{
var jsonArray = new JsonObject();
do
{
ReadToNonBlankIndex(text, ref index);
if (text[index] != '"') throw new JsonAnalysisException($"不能識別的字元“{text[index]}”!應為“\"”",index);
index++;
//讀取字串
var name = ReadString(text, ref index);
ReadToNonBlankIndex(text, ref index);
if (text[index] != ':') throw new JsonAnalysisException($"不能識別的字元“{text[index]}”!",index);
index++;
ReadToNonBlankIndex(text, ref index);
if (jsonArray.ContainsKey(name)) throw new JsonAnalysisException($"已經新增鍵值:“{name}”",index);
//讀取下一個Element
jsonArray.Add(name, ReadElement(text, ref index));
//讀取到非空白字元
ReadToNonBlankIndex(text, ref index);
} while (text[index++] != '}');
return jsonArray;
}
///
/// 解析JSON陣列
///
/// JSON字串
/// 開始索引位置
///
private static JsonArray AnalysisJsonArray(string text, ref int index)
{
var jsonArray = new JsonArray();
do
{
ReadToNonBlankIndex(text, ref index);
//讀取下一個Element
jsonArray.Add(ReadElement(text, ref index));
//讀取到非空白字元
ReadToNonBlankIndex(text, ref index);
} while (text[index++] != ']');
return jsonArray;
}
///
/// 讀取JSONElement
///
/// 字串
/// 開始下標
///
private static JsonElement ReadElement(string text, ref int index)
{
switch (text[index++])
{
case '[':
return AnalysisJsonArray(text, ref index);
case '{':
return AnalysisJsonObject(text, ref index);
case '"':
return new JsonString(ReadString(text, ref index));
case 't':
return ReadJsonTrue(text, ref index);
case 'f':
return ReadJsonFalse(text, ref index);
case 'n':
return ReadJsonNull(text, ref index);
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
case '8':
case '9':
return ReadJsonNumber(text, ref index);
default:
throw new JsonAnalysisException($"未知Element“{text[index]}”應該為【[、{{、\"、true、false、null】", index);
}
}
///
/// 讀取值型別
///
/// JSON字串
/// 開始索引
///
private static JsonNumber ReadJsonNumber(string text, ref int index)
{
var i = index;
while (i < text.Length && char.IsNumber(text[i]) || text[i] == '.') i++;
if (double.TryParse(text.Substring(index - 1, i - index + 1), out var value))
{
index = i;
return new JsonNumber(value);
}
throw new JsonAnalysisException("不能識別的數字型別!", i);
}
///
/// 讀取NULL
///
/// JSON字串
/// 開始索引
///
private static JsonNull ReadJsonNull(string text, ref int index)
{
if (text[index++] == 'u' &&
text[index++] == 'l' &&
text[index++] == 'l')
{
return new JsonNull();
}
throw new JsonAnalysisException("讀取null出錯!", index);
}
///
/// 讀取FALSE
///
/// JSON字串
/// 開始索引
///
private static JsonBoolean ReadJsonFalse(string text, ref int index)
{
if (text[index++] == 'a' &&
text[index++] == 'l' &&
text[index++] == 's' &&
text[index++] == 'e')
{
return new JsonBoolean(false);
}
throw new JsonAnalysisException("讀取布林值出錯!", index);
}
///
/// 讀取TRUE
///
/// JSON字串
/// 開始索引
///
private static JsonBoolean ReadJsonTrue(string text, ref int index)
{
if (text[index++] == 'r' &&
text[index++] == 'u' &&
text[index++] == 'e')
{
return new JsonBoolean(true);
}
throw new JsonAnalysisException("讀取布林值出錯!",index);
}
///
/// 讀取字串
///
/// JSON字串
/// 開始索引
///
private static string ReadString(string text, ref int index)
{
//是否處於轉義狀態
var value = new StringBuilder();
while (index < text.Length)
{
var c = text[index++];
if (c == '\\')
{
value.Append('\\');
if (index >= text.Length)
throw new JsonAnalysisException("未知的結尾!",index);
c = text[index++];
value.Append(c);
if (c == 'u')
{
for (int i = 0; i < 4; i++)
{
c = text[index++];
if (IsHex(c))
{
value.Append(c);
}
else
{
throw new JsonAnalysisException("不是有效的Unicode字元!",index);
}
}
}
}
else if (c == '"')
{
break;
}
else if (c == '\r' || c == '\n')
{
throw new JsonAnalysisException("傳入的JSON字串內容中不允許有換行!",index);
}
else
{
value.Append(c);
}
}
return value.ToString();
}
///
/// 判斷是否為16進位制字元
///
private static bool IsHex(char c)
{
return c >= '0' && c <= '9' || c >= 'a' && c <= 'z' || c >= 'A' && c <= 'Z';
}
///
/// 讀取到非空白字元
///
/// 字串
/// 開始下標
///
private static void ReadToNonBlankIndex(string text, ref int index)
{
while (index < text.Length && char.IsWhiteSpace(text[index])) index++;
}
}
```
### 完整DEMO程式碼下載
Github專案地址(會持續更新):[DEMO程式碼](https://github.com/liu-zhen-liang/JsonAnalysis)