SpringBoot實現本地儲存檔案上傳及提供HTTP訪問服務
阿新 • • 發佈:2020-08-11
筆者計劃為大家介紹分散式檔案系統,用於儲存應用的圖片、word、excel、pdf等檔案。在開始介紹分散式檔案系統之前,為大家介紹一下使用本機儲存來存放檔案資源。
二者的核心實現過程是一樣的:
* 上傳檔案,儲存檔案(本節是本地磁碟)
* 返回檔案HTTP訪問服務路徑給前端,進行上傳之後的效果展示
## 一、複習
服務端接收上傳的目的是提供檔案的訪問服務,那麼對於SpringBoot而言,有哪些可以提供檔案訪問的靜態資源目錄呢?
* `classpath:/META-INF/resources/` ,
* `classpath:/static/` ,
* `classpath:/public/` ,
* `classpath:/resources/`
這是之前我們為大家介紹的內容,從這裡看出這裡的靜態資源都在classpath下。那麼就出現問題:
* 應用的檔案資源不能和專案程式碼分開儲存(你見過往github上傳程式碼,還附帶專案檔案資料的麼?)
* 專案打包困難,當上傳的檔案越來越多,專案的打包jar越來越大。
* 程式碼與檔案資料不能分開儲存,就意味著檔案資料的備份將變得複雜
## 二、檔案上傳目錄自定義配置
怎麼解決上述問題?別忘記了spring boot 為我們提供了**使用`spring.resources.static-locations`配置自定義靜態檔案的位置。**
~~~
web:
upload-path: D:/data/
spring:
resources:
static-locations: classpath:/META-INF/resources/,classpath:/resources/,classpath:/static/,classpath:/public/,file:${web.upload-path}
~~~
* 配置`web.upload-path`為與專案程式碼分離的靜態資源路徑,即:檔案上傳儲存根路徑
* 配置`spring.resources.static-locations`,除了帶上Spring Boot預設的靜態資源路徑之外,加上file:${web.upload-path}指向外部的檔案資源上傳路徑。該路徑下的靜態資源可以直接對外提供HTTP訪問服務。
## 三、檔案上傳的Controller實現
詳情看程式碼註釋
~~~
@RestController
public class FileUploadController {
//繫結檔案上傳路徑到uploadPath
@Value("${web.upload-path}")
private String uploadPath;
SimpleDateFormat sdf = new SimpleDateFormat("yyyy/MM/dd/");
@PostMapping("/upload")
public String upload(MultipartFile uploadFile,
HttpServletRequest request) {
// 在 uploadPath 資料夾中通過日期對上傳的檔案歸類儲存
// 比如:/2019/06/06/cf13891e-4b95-4000-81eb-b6d70ae44930.png
String format = sdf.format(new Date());
File folder = new File(uploadPath + format);
if (!folder.isDirectory()) {
folder.mkdirs();
}
// 對上傳的檔案重新命名,避免檔案重名
String oldName = uploadFile.getOriginalFilename();
String newName = UUID.randomUUID().toString()
+ oldName.substring(oldName.lastIndexOf("."), oldName.length());
try {
// 檔案儲存
uploadFile.transferTo(new File(folder, newName));
// 返回上傳檔案的訪問路徑
String filePath = request.getScheme() + "://" + request.getServerName()
+ ":" + request.getServerPort() + format + newName;
return filePath;
} catch (IOException e) {
throw new CustomException(CustomExceptionType.SYSTEM_ERROR);
}
}
}
~~~
## 四、寫一個模擬的檔案上傳頁面,進行測試
把該upload.html檔案放到classpath:public目錄下,對外提供訪問。
~~~
~~~
訪問測試、點選“選擇檔案”,之後儲存
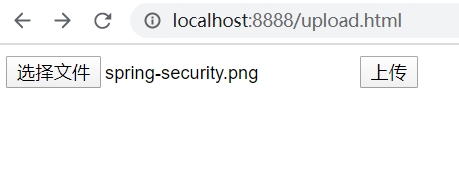
檔案被儲存到服務端的`web.upload-path`指定的資源目錄下
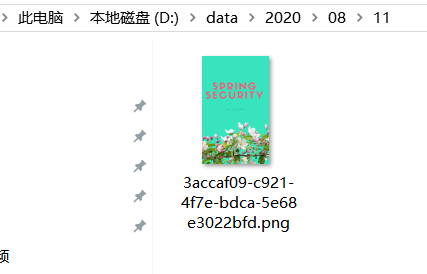
瀏覽器端響應結果如下,返回一個檔案HTTP訪問路徑:

使用該HTTP訪問路徑,在瀏覽器端訪問效果如下。證明我們的檔案已經成功上傳到服務端,以後需要訪問該圖片就通過這個HTTP URL就可以了。
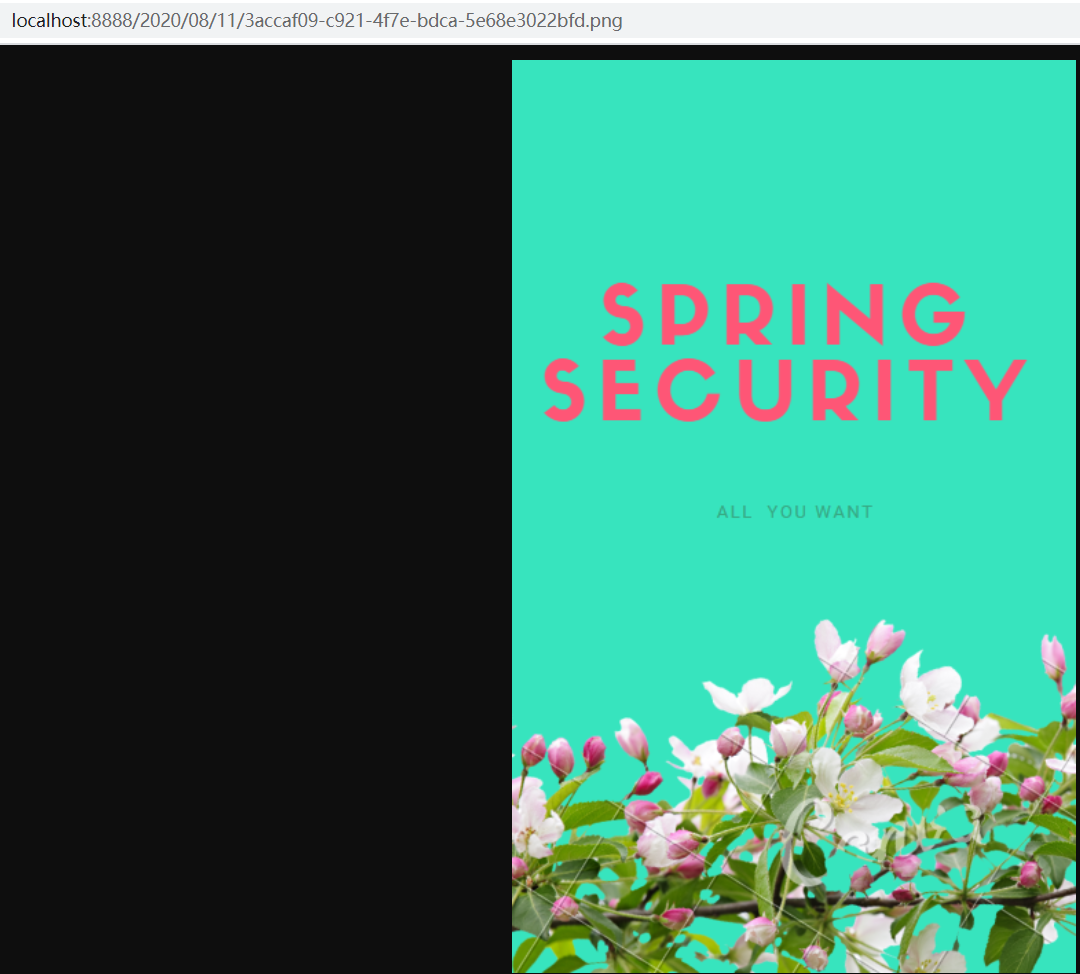
## 歡迎關注我的部落格,裡面有很多精品合集
* 本文轉載註明出處(必須帶連線,不能只轉文字):[字母哥部落格](http://www.zimug.com)。
**覺得對您有幫助的話,幫我點贊、分享!您的支援是我不竭的創作動力!** 。另外,筆者最近一段時間輸出瞭如下的精品內容,期待您的關注。
* [《手摸手教你學Spring Boot2.0》]( https://www.kancloud.cn/hanxt/springboot2/content )
* [《Spring Security-JWT-OAuth2一本通》](https://www.kancloud.cn/hanxt/springsecurity/content)
* [《實戰前後端分離RBAC許可權管理系統》](https://www.kancloud.cn/hanxt/vue-spring/content)
* [《實戰SpringCloud微服務從青銅到王者》](https://www.kancloud.cn/hanxt/springcloud/content)
* [《VUE深入淺出系列》](https://www.kancloud.cn/hanxt/vuejs2/