pytest封神之路第三步 精通fixture
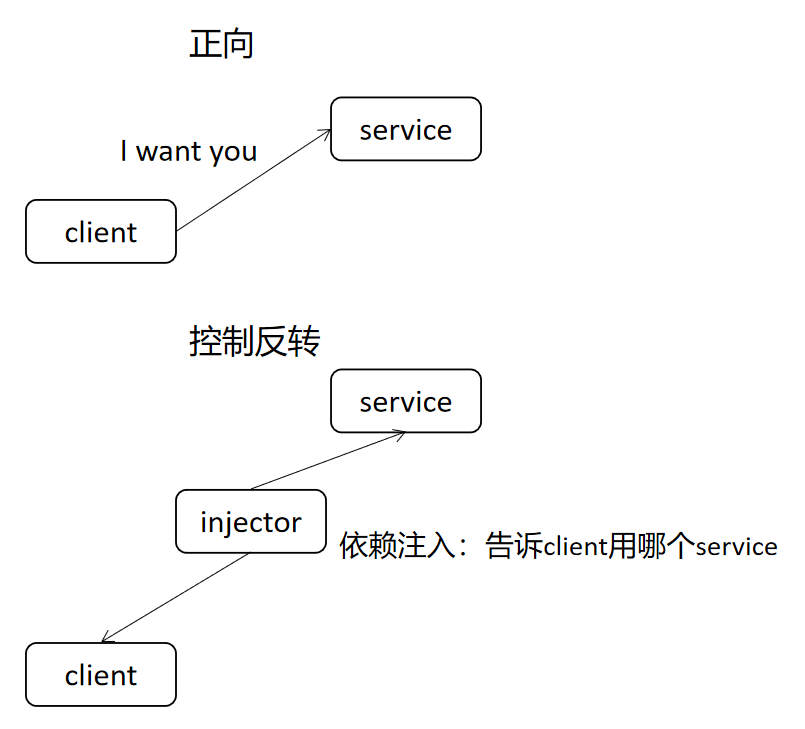
相關推薦
pytest封神之路第三步 精通fixture
首先放一句“狠話”。 如果你不會fixture,那麼你最好別說自己會pytest。 (只是為了烘托主題哈,手上的磚頭可以放下了,手動滑稽) # fixture是什麼 看看原始碼 ```python def fixture( callable_or_scope=None, *args,
pytest封神之路第四步 內建和自定義marker
可以通過命令列檢視所有marker,包括內建和自定義的 ```shell pytest --markers ``` # 內建marker 內建marker本文先講usefixtures 、filterwarnings 、skip 、skipif 、xfail這5個。引數化的marker我會寫在
pytest封神之路第五步 引數化進階
用過unittest的朋友,肯定知道可以藉助DDT實現引數化。用過JMeter的朋友,肯定知道JMeter自帶了4種引數化方式(見參考資料)。pytest同樣支援引數化,而且很簡單很實用。 # 語法 在《pytest封神之路第三步 精通fixture》和《pytest封神之路第四步 內建和自定義marke
pytest封神之路第一步 tep介紹
『 tep is a testing tool to help you write pytest more easily. Try Easy Pytest! 』 # tep前身 tep的前身是介面自動化測試框架pyface,一款面向物件設計的測試框架,我寫過一篇[部落格](https://www.cn
《帶你裝B,帶你飛》pytest成神之路2- 執行用例規則和pycharm執行的三種姿態
1. 簡介 今天北京下的雪好大好美啊!!!哎呀,忘記拍照片了,自己想象一下吧。言歸真傳,今天還是開始pytest的學習和修煉,上一篇寫完後群裡反響各式各樣的,幾家歡樂幾家愁,有的高興說自己剛好要用到了,正好一起學習,有的不開心說自己介面還沒有學完了,沒關係的學習本來就不是一件一蹴而就的事情,需要日積月累
python之路第三篇
區別 英語 utf-8 系統 存在 創建 tel run 變量名 python文件目錄操作 python中對文件、文件夾(文件操作函數)的操作需要涉及到os模塊和shutil模塊。 得到當前工作目錄,即當前Python腳本工作的目錄路
python學習之路——第三彈 (作業篇第一題)
image 操作 啟動程序 代碼 color 鎖定文件 文件 文件內容 數據 作業一:編寫登錄接口1.輸入用戶名密碼2.認證成功後顯示歡迎信息3.輸錯三次後鎖定。 所需知識點 文件基本讀寫操作,循環,列表,字典 上面的作業題是在學習完數據類型和簡單的文件操作之後布置的,
重構之路第三篇——重新組織數據
chan direction hang rate state elf with bsp 類型 本篇目錄: 1 Self Encapsulate Field(自封裝字段) 2 Replace Data Value with Object(以對象取代數據值) 3 Change
python學習之路-第三天-一個簡單的腳本
tro 說明 .py else zipfile rect dylib 環境 cef 現在有一個需求:把某個目錄下的文件備份到指定到另外一個目錄下,而且壓縮後文件為zip文件 # -*- coding:utf-8 -*- #! /usr/bin/python # Filena
Python人工智能之路 - 第三篇 : PyAudio 實現錄音 自動化交互實現問答
獲得 本地文件 一次 cor ets win 不清晰 考題 dbo Python 很強大其原因就是因為它龐大的三方庫 , 資源是非常的豐富 , 當然也不會缺少關於音頻的庫 關於音頻, PyAudio 這個庫, 可以實現開啟麥克風錄音, 可以播放音頻文件等等,此刻我們不去了解
Django之路--第三篇
creat () roo object migrate filter sta migration upd 1.ORM1.1.創建類和字段 class UserInfo(models.Model): name=models.CharField(max_length=6
ocrosoft Contest1316 - 信奧編程之路~~~~~第三關 問題 I: 尋找大富翁
EDA 第三關 using cpp print int ocr mes name http://acm.ocrosoft.com/problem.php?cid=1316&pid=8 題目描述 浙江杭州某鎮共有n個人,請找出該鎮上的前m個大富翁. 輸入
java學習之路---------第三天
今天主要就是陣列的基本概念 陣列:例子 int arr[] = new int[3]; &nb
Python之路(第三十一篇) 網路程式設計:簡單的tcp套接字通訊、粘包現象
一、簡單的tcp套接字通訊 套接字通訊的一般流程 服務端 server = socket() #建立伺服器套接字 server.bind() #把地址繫結到套接字,網路地址加埠 server.listen() #監聽連結 inf_loop:
Python之路(第三十三篇) 網路程式設計:socketserver深度解析
一、socketserver 模組介紹 socketserver是標準庫中的一個高階模組,用於網路客戶端與伺服器的實現。(version = "0.4") 在python2中寫作SocketServer,在python3中寫作socketserver。 socoketserver兩個主要的類,一個是S
Python之路(第三十四篇) 網路程式設計:驗證客戶端合法性
一、驗證客戶端合法性 如果你想在分散式系統中實現一個簡單的客戶端連結認證功能,又不像SSL那麼複雜,那麼利用hmac+加鹽的方式來實現。 客戶端驗證的總的思路是將服務端隨機產生的指定位數的位元組傳送到客戶端,兩邊同時用hmac進行加密,然後對生成的密文進行比較,相同就是合法的客戶端,不相同就是不合法
阿里P7/P8學習路線圖——技術封神之路
一、基礎篇 JVM JVM記憶體結構 堆、棧、方法區、直接記憶體、堆和棧區別 Java記憶體模型 記憶體可見性、重排序、順序一致性、volatile、鎖、final 垃圾回收 記憶體分配策略、垃圾收集器(G1)、GC演算法、GC引數、物件存活的判定 JVM引數及
黎想深度訪談騰訊頂級產品經理的進階之路——第三篇《需求》
16個月精心打磨,9位頂級產品專家研討提煉,凝聚騰訊產品經驗的八集八分鐘產品課分別從使用者、定位、需求、時機、匠心、危機、合作、商業角度出發,還原產品背後的故事,分享給你騰訊產品的心法。藝形藝意工作室創始人黎想將深度訪談騰訊頂級產品經理的進階之路,邀您一起探索一
Python之路(第三十七篇)併發程式設計:程序、multiprocess模組、建立程序方式、join()、守護程序
一、在python程式中的程序操作 之前已經瞭解了很多程序相關的理論知識,瞭解程序是什麼應該不再困難了,執行中的程式就是一個程序。所有的程序都是通過它的父程序來建立的。因此,執行起來的python程式也是一個程序,那麼也可以在程式中再建立程序。多個程序可以實現併發效果,也就是說,當程式中存在多個程序的時候
Python之路(第三十八篇) 併發程式設計:程序同步鎖/互斥鎖、訊號量、事件、佇列、生產者消費者模型
一、程序鎖(同步鎖/互斥鎖) 程序之間資料不共享,但是共享同一套檔案系統,所以訪問同一個檔案,或同一個列印終端,是沒有問題的, 而共享帶來的是競爭,競爭帶來的結果就是錯亂,如何控制,就是加鎖處理。 例子 #併發執行,效率高,但競爭同一列印終端,帶來了列印錯亂 from multiproc