Mysql 連線的使用
在前幾章節中,我們已經學會瞭如何在一張表中讀取資料,這是相對簡單的,但是在真正的應用中經常需要從多個資料表中讀取資料。
本章節我們將向大家介紹如何使用 MySQL 的 JOIN 在兩個或多個表中查詢資料。
你可以在 SELECT, UPDATE 和 DELETE 語句中使用 Mysql 的 JOIN 來聯合多表查詢。
JOIN 按照功能大致分為如下三類:
-
INNER JOIN(內連線,或等值連線):獲取兩個表中欄位匹配關系的記錄。
-
LEFT JOIN(左連線):獲取左表所有記錄,即使右表沒有對應匹配的記錄。
-
RIGHT JOIN(右連線): 與 LEFT JOIN 相反,用於獲取右表所有記錄,即使左表沒有對應匹配的記錄。
本章節使用的資料庫結構及資料下載:itread01-mysql-join-test.sql。
在命令提示符中使用 INNER JOIN
我們在itread01資料庫中有兩張表 tcount_tbl 和 itread01_tbl。兩張資料表資料如下:
例項
嘗試以下例項:
測試例項資料
mysql> use itread01;
Database changed
mysql> SELECT * FROM tcount_tbl;
+---------------+--------------+
| itread01_author | itread01_count |
+---------------+--------------+
| 入門教學 | 10 |
| itread01.COM | 20 |
| Google | 22 |
+---------------+--------------+
3 rows in set (0.01 sec)
mysql> SELECT * from itread01_tbl;
+-----------+---------------+---------------+-----------------+
| itread01_id | itread01_title | itread01_author | submission_date |
+-----------+---------------+---------------+-----------------+
| 1 | 學習 PHP | 入門教學 | 2017-04-12 |
| 2 | 學習 MySQL | 入門教學 | 2017-04-12 |
| 3 | 學習 Java | itread01.COM | 2015-05-01 |
| 4 | 學習 Python | itread01.COM | 2016-03-06 |
| 5 | 學習 C | FK | 2017-04-05 |
+-----------+---------------+---------------+-----------------+
5 rows in set (0.01 sec)
接下來我們就使用MySQL的INNER JOIN(也可以省略 INNER 使用 JOIN,效果一樣)來連線以上兩張表來讀取itread01_tbl表中所有itread01_author欄位在tcount_tbl表對應的itread01_count欄位值:
INNER JOIN
mysql> SELECT a.itread01_id, a.itread01_author, b.itread01_count FROM itread01_tbl a INNER JOIN tcount_tbl b ON a.itread01_author = b.itread01_author;
+-------------+-----------------+----------------+
| a.itread01_id | a.itread01_author | b.itread01_count |
+-------------+-----------------+----------------+
| 1 | 入門教學 | 10 |
| 2 | 入門教學 | 10 |
| 3 | itread01.COM | 20 |
| 4 | itread01.COM | 20 |
+-------------+-----------------+----------------+
4 rows in set (0.00 sec)
以上 SQL 語句等價於:
WHERE 子句
mysql> SELECT a.itread01_id, a.itread01_author, b.itread01_count FROM itread01_tbl a, tcount_tbl b WHERE a.itread01_author = b.itread01_author;
+-------------+-----------------+----------------+
| a.itread01_id | a.itread01_author | b.itread01_count |
+-------------+-----------------+----------------+
| 1 | 入門教學 | 10 |
| 2 | 入門教學 | 10 |
| 3 | itread01.COM | 20 |
| 4 | itread01.COM | 20 |
+-------------+-----------------+----------------+
4 rows in set (0.01 sec)
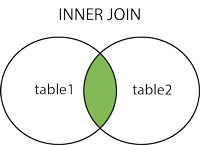
MySQL LEFT JOIN
MySQL left join 與 join 有所不同。 MySQL LEFT JOIN 會讀取左邊資料表的全部資料,即便右邊表無對應資料。
例項
嘗試以下例項,以 itread01_tbl 為左表,tcount_tbl 為右表,理解 MySQL LEFT JOIN 的應用:
LEFT JOIN
mysql> SELECT a.itread01_id, a.itread01_author, b.itread01_count FROM itread01_tbl a LEFT JOIN tcount_tbl b ON a.itread01_author = b.itread01_author;
+-------------+-----------------+----------------+
| a.itread01_id | a.itread01_author | b.itread01_count |
+-------------+-----------------+----------------+
| 1 | 入門教學 | 10 |
| 2 | 入門教學 | 10 |
| 3 | itread01.COM | 20 |
| 4 | itread01.COM | 20 |
| 5 | FK | NULL |
+-------------+-----------------+----------------+
5 rows in set (0.01 sec)
以上例項中使用了 LEFT JOIN,該語句會讀取左邊的資料表 itread01_tbl 的所有選取的欄位資料,即便在右側表 tcount_tbl中 沒有對應的 itread01_author 欄位值。
MySQL RIGHT JOIN
MySQL RIGHT JOIN 會讀取右邊資料表的全部資料,即便左邊邊表無對應資料。
例項
嘗試以下例項,以 itread01_tbl 為左表,tcount_tbl 為右表,理解MySQL RIGHT JOIN的應用:
RIGHT JOIN
mysql> SELECT a.itread01_id, a.itread01_author, b.itread01_count FROM itread01_tbl a RIGHT JOIN tcount_tbl b ON a.itread01_author = b.itread01_author;
+-------------+-----------------+----------------+
| a.itread01_id | a.itread01_author | b.itread01_count |
+-------------+-----------------+----------------+
| 1 | 入門教學 | 10 |
| 2 | 入門教學 | 10 |
| 3 | itread01.COM | 20 |
| 4 | itread01.COM | 20 |
| NULL | NULL | 22 |
+-------------+-----------------+----------------+
5 rows in set (0.01 sec)
以上例項中使用了 RIGHT JOIN,該語句會讀取右邊的資料表 tcount_tbl 的所有選取的欄位資料,即便在左側表 itread01_tbl 中沒有對應的itread01_author 欄位值。
在 PHP 指令碼中使用 JOIN
PHP 中使用 mysqli_query() 函式來執行 SQL 語句,你可以使用以上的相同的 SQL 語句作為 mysqli_query() 函式的引數。
嘗試如下例項:
MySQL ORDER BY 測試:
<?php
$dbhost = 'localhost:3306';
$dbuser = 'root';
$dbpass = '123456';
$conn = mysqli_connect($dbhost, $dbuser, $dbpass);
if(! $conn )
{
die('連線失敗: ' . mysqli_error($conn));
}
mysqli_query($conn , "set names utf8");
$sql = 'SELECT a.itread01_id, a.itread01_author, b.itread01_count FROM itread01_tbl a INNER JOIN tcount_tbl b ON a.itread01_author = b.itread01_author';
mysqli_select_db( $conn, 'itread01' );
$retval = mysqli_query( $conn, $sql );
if(! $retval )
{
die('無法讀取資料: ' . mysqli_error($conn));
}
echo '<h2>入門教學 MySQL JOIN 測試<h2>';
echo '<table border="1"><tr><td>教程 ID</td><td>作者</td><td>登陸次數</td></tr>';
while($row = mysqli_fetch_array($retval, MYSQL_ASSOC))
{
echo "<tr><td> {$row['itread01_id']}</td> ".
"<td>{$row['itread01_author']} </td> ".
"<td>{$row['itread01_count']} </td> ".
"</tr>";
}
echo '</table>';
mysqli_close($conn);
?>