SpringBoot上傳任意文件功能的實現
阿新 • • 發佈:2017-08-01
turn span ise created recursive .get on() pro equal 一、pom文件依賴的添加
二、controller層
六、測試成功的結果
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> </dependencies>
@Controller public class FileUploadController { private final StorageService storageService; @Autowired public FileUploadController(StorageService storageService) { this.storageService = storageService; } //展示上傳過的文件 @GetMapping("/") public String listUploadedFiles(Model model) throws IOException { model.addAttribute(三、實現的service層"files", storageService.loadAll().map(path -> MvcUriComponentsBuilder.fromMethodName(FileUploadController.class, "serveFile", path.getFileName().toString()) .build().toString()) .collect(Collectors.toList())); return "uploadForm"; } //下載選定的上傳的文件 @GetMapping("/files/{filename:.+}") @ResponseBody public ResponseEntity<Resource> serveFile(@PathVariable String filename) { Resource file = storageService.loadAsResource(filename); return ResponseEntity .ok() .header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\""+file.getFilename()+"\"") .body(file); } //上傳文件 @PostMapping("/") public String handleFileUpload(@RequestParam("file") MultipartFile file, RedirectAttributes redirectAttributes) { storageService.store(file); redirectAttributes.addFlashAttribute("message", "You successfully uploaded " + file.getOriginalFilename() + "!"); return "redirect:/"; } @ExceptionHandler(StorageFileNotFoundException.class) public ResponseEntity<?> handleStorageFileNotFound(StorageFileNotFoundException exc) { return ResponseEntity.notFound().build(); } }
@Service public class FileSystemStorageService implements StorageService { private final Path rootLocation; @Autowired public FileSystemStorageService(StorageProperties properties) { this.rootLocation = Paths.get(properties.getLocation()); } @Override public void store(MultipartFile file) { try { if (file.isEmpty()) { throw new StorageException("Failed to store empty file " + file.getOriginalFilename()); } Files.copy(file.getInputStream(), this.rootLocation.resolve(file.getOriginalFilename())); } catch (IOException e) { throw new StorageException("Failed to store file " + file.getOriginalFilename(), e); } } @Override public Stream<Path> loadAll() { try { return Files.walk(this.rootLocation, 1) .filter(path -> !path.equals(this.rootLocation)) .map(path -> this.rootLocation.relativize(path)); } catch (IOException e) { throw new StorageException("Failed to read stored files", e); } } @Override public Path load(String filename) { return rootLocation.resolve(filename); } @Override public Resource loadAsResource(String filename) { try { Path file = load(filename); Resource resource = new UrlResource(file.toUri()); if(resource.exists() || resource.isReadable()) { return resource; } else { throw new StorageFileNotFoundException("Could not read file: " + filename); } } catch (MalformedURLException e) { throw new StorageFileNotFoundException("Could not read file: " + filename, e); } } @Override public void deleteAll() { FileSystemUtils.deleteRecursively(rootLocation.toFile()); } @Override public void init() { try { Files.createDirectory(rootLocation); } catch (IOException e) { throw new StorageException("Could not initialize storage", e); } } }四、在application.properties文件上配置上傳的屬性
spring.http.multipart.max-file-size=128KB
spring.http.multipart.max-request-size=128KB
五、服務啟動時的處理
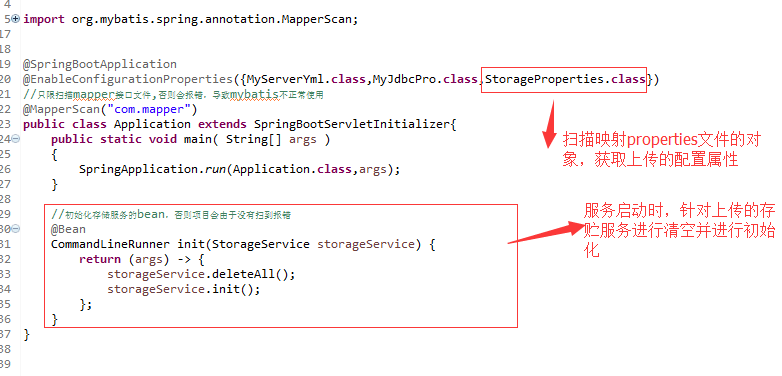
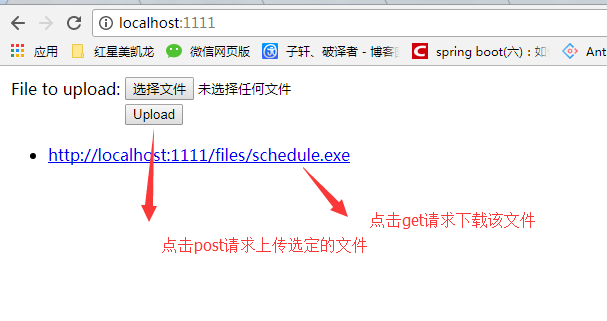
SpringBoot上傳任意文件功能的實現