Android開發1、2周——GeoQuiz項目
GeoQuiz項目總結
通過學習Android基本概念與構成應用的基本組件,來開發一個叫GeoQuiz的應用。該應用的用途是測試用戶的地理知識。用戶單擊TRUE或FALSE按鈕來回答屏幕上的問題,GeoQuiz可即時反饋答案正確與否。
開發前的準備工作
想要開發一個Android應用,首先要在電腦上裝上開發軟件。在這裏推薦Android Studio,本文所有的開發都是在該平臺上進行的。
Android Studio的安裝包括:
1.Android SDK
最新版本的Android SDK。
2.Android SDK工具和平臺工具
用來測試與調試應用的一套工具。
3.Android模擬器系統鏡像
用來在不同虛擬設備上開發測試應用。
下載與安裝
可以從Android開發者網站下載Android Studio:https://developer.android.com/sdk/ 首次安裝的話,你還需要從http://www.oracle.com下載並安裝Java開發者套件(JDK7)。 如仍有安裝問題,請訪問網址https://developer.android.com/sdk/尋求幫助。下載早期版本的SDK
Android Studio自帶最新版本的SDK和系統模擬器鏡像。但若想在Android早期版本上測試應用,還需額外下載相關工具組件。可通過Android SDK管理器來配置安裝這些組件。在Android Studio中,選擇To o ls→Android→SDK Manager菜單項。如圖1-1所示。
應用開發
1.1 項目的創建
首先,打開Android Studio,創建GeoQuiz項目。如圖1.1-1所示。
圖1.1-1
此次項目的具體目錄。如圖1.1-2所示。
圖1.1-2
1.2 代碼的編寫
1.2.1 界面設計
此次項目的具體界面如下圖所示。
主界面設計(豎屏、橫屏)
CHEAT按鈕的關聯界面設計
1.2.2 源碼
在spring.xml中完成設置的代碼如下。

1 <resources> 2 <string name="app_name">GeoQuiz</string> 3 4 <string name="true_button">TRUE</string> 5 <string name="false_button">FALSE</string> 6 <string name="next_button">NEXT</string> 7 <string name="prev_button">PREV</string> 8 <string name="correct_toast">Correct!</string> 9 <string name="incorrect_toast">Incorrect!</string> 10 <string name="warning_text">Are you sure you want to do this?</string> 11 <string name="show_answer_button">Show Answer</string> 12 <string name="cheat_button">Cheat!</string> 13 <string name="judgment_toast">Cheating is wrong.</string> 14 <string name="question_oceans">The Pacific Ocean is larger than the Atlantic Ocean.</string> 15 <string name="question_mideast">The Suez Canal connects the Red Sea and the Indian Ocean.</string> 16 <string name="question_africa">The source of the Nile River is in Egypt.</string> 17 <string name="question_americas">The Amazon River is the longest river in the Americas.</string> 18 <string name="question.asia">Lake Baikal is the world\‘s oldest and deepest freshwater lake.</string> 19 </resources>

在QuizActivity.java中實現從布局到視圖的代碼如下。

1 @Override 2 protected void onCreate(Bundle savedInstanceState) { 3 super.onCreate(savedInstanceState); 4 Log.d(TAG, "onCreate(Bundle) called"); 5 setContentView(R.layout.activity_quiz); 6 7 if (savedInstanceState != null) { 8 mCurrentIndex = savedInstanceState.getInt(KEY_INDEX, 0); 9 } 10 11 mQuestionTextView = (TextView) findViewById(R.id.question_text_view); 12 13 mTrueButton = (Button) findViewById(R.id.true_button); 14 mTrueButton.setOnClickListener(new View.OnClickListener() { 15 @Override 16 public void onClick(View v){ 17 checkAnswer(true); 18 } 19 }); 20 mFalseButton = (Button) findViewById(R.id.false_button); 21 mFalseButton.setOnClickListener(new View.OnClickListener() { 22 @Override 23 public void onClick(View v){ 24 checkAnswer(false); 25 } 26 }); 27 28 mNextButton = (Button) findViewById(R.id.next_button); 29 mNextButton.setOnClickListener(new View.OnClickListener() { 30 @Override 31 public void onClick(View v) { 32 mCurrentIndex = (mCurrentIndex + 1) % mQuestionBank.length; 33 mIsCheater = false; 34 updateQuestion(); 35 } 36 }); 37 38 mPrevButton = (Button) findViewById(R.id.prev_button); 39 mPrevButton.setOnClickListener(new View.OnClickListener() { 40 @Override 41 public void onClick(View v) { 42 mCurrentIndex = (mCurrentIndex - 1) % mQuestionBank.length; 43 updateQuestion(); 44 } 45 }); 46 47 mCheatButton = (Button)findViewById(R.id.cheat_button); 48 mCheatButton.setOnClickListener(new View.OnClickListener() { 49 @Override 50 public void onClick(View v) { 51 boolean answerIsTrue = mQuestionBank[mCurrentIndex].ismAnswerTrue(); 52 Intent intent = CheatActivity.newIntent(QuizActivity.this, answerIsTrue); 53 startActivityForResult(intent, REQUEST_CODE_CHEAT); 54 } 55 }); 56 57 updateQuestion(); 58 59 }

在Question.java模型層中編寫的代碼如下。

1 public class Question { 2 private int mTextResId; 3 private boolean mAnswerTrue; 4 5 public Question(int textResId, boolean answerTrue){ 6 mTextResId = textResId; 7 mAnswerTrue = answerTrue; 8 } 9 10 public int getmTextResId() { 11 return mTextResId; 12 } 13 14 public void setmTextResId(int mTextResId) { 15 this.mTextResId = mTextResId; 16 } 17 18 public boolean ismAnswerTrue() { 19 return mAnswerTrue; 20 } 21 22 public void setmAnswerTrue(boolean mAnswerTrue) { 23 this.mAnswerTrue = mAnswerTrue; 24 } 25 }

1.3 Android與MAC設計模式
在響應用戶單擊按鈕等事件時,對象間的交互控制數據流如圖1.3-1所示。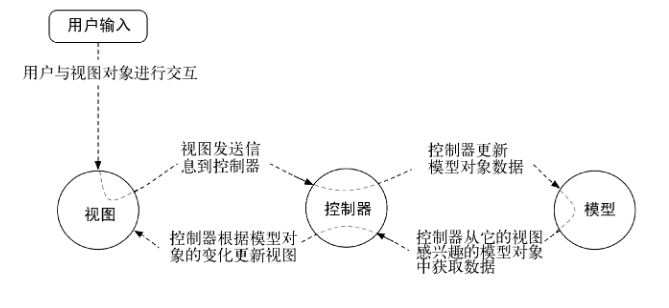
圖1.3-2
1.4 Activity的生命周期
在項目中主要使用的Activity的生命周期如圖1.4-1所示。
圖1.4-1
1.5 CHEAT按鈕的關聯界面
在activity_cheat.xml中編寫的組件代碼如下所示。

1 <?xml version="1.0" encoding="utf-8"?> 2 3 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" 4 xmlns:tools="http://schemas.android.com/tools" 5 android:layout_width="match_parent" 6 android:layout_height="match_parent" 7 android:orientation="vertical" 8 android:gravity="center" 9 tools:context="classroom.geoquiz.CheatActivity"> 10 11 <TextView 12 android:layout_width="wrap_content" 13 android:layout_height="wrap_content" 14 android:padding="24dp" 15 android:text="@string/warning_text"/> 16 17 <TextView 18 android:layout_width="wrap_content" 19 android:layout_height="wrap_content" 20 android:id="@+id/answer_text_view" 21 android:padding="24dp" 22 tools:text="Answer"/> 23 24 <Button 25 android:id="@+id/show_answer_button" 26 android:layout_width="wrap_content" 27 android:layout_height="wrap_content" 28 android:text="@string/show_answer_button"/> 29 30 </LinearLayout>

1.6 備註
以下是項目過程開發中遇到的問題。
1.6.1 Gradle文件下載速度慢
使用阿裏雲的國內鏡像倉庫地址,就可以快速的下載需要的文件。
修改項目根目錄下的文件 build.gradle 如下。
buildscript {
repositories {
maven{ url ‘http://maven.aliyun.com/nexus/content/groups/public/‘}
}
}
allprojects {
repositories {
maven{ url ‘http://maven.aliyun.com/nexus/content/groups/public/‘}
}
}
然後選擇重新構建項目就可以了。
Android開發1、2周——GeoQuiz項目