Python 中的 classmethod 和 staticmethod
阿新 • • 發佈:2018-05-19
original Go got typeerror print ini 靜態 main method 類中最常用的方法是實例方法, 即通過通過實例作為第一個參數的方法。
輸出:
Reset done for: 12
DB connection made for: 12
如果使用@staticmethod就能把相關的代碼放到對應的位置了.
下面這個圖解釋了以上代碼是怎麽運行的:
舉個例子,一個基本的實例方法就向下面這個:
class Kls(object):
def __init__(self, data):
self.data = data
def printd(self):
print(self.data)
ik1 = Kls(‘arun‘)
ik2 = Kls(‘seema‘)
ik1.printd()
ik2.printd()
這會給出如下的輸出:
arun
seema
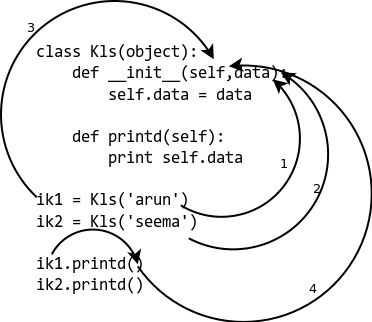
- 1,2參數傳遞給方法.
- 3 self參數指向當前實例自身.
- 4 我們不需要傳遞實例自身給方法,Python解釋器自己會做這些操作的.
如果現在我們想寫一些僅僅與類交互而不是和實例交互的方法會怎麽樣呢?
可以使用@classmethod裝飾器來創建類方法.
class Kls(object):
no_inst = 0
def __init__(self):
Kls.no_inst = Kls.no_inst + 1
@classmethod
def get_no_of_instance(cls_obj):
return cls_obj.no_inst
ik1 = Kls()
ik2 = Kls()
print ik1.get_no_of_instance()
print Kls.get_no_of_instance()
輸出:
2
2
這樣的好處是: 不管這個方式是從實例調用還是從類調用,它都用第一個參數把類傳遞過來.
@staticmethod
經常有一些跟類有關系的功能但在運行時又不需要實例和類參與的情況下需要用到靜態方法. 比如更改環境變量或者修改其他類的屬性等能用到靜態方法. 這種情況可以直接用函數解決, 但這樣同樣會擴散類內部的代碼,造成維護困難.
比如這樣:
IND = ‘ON‘
def checkind():
return (IND == ‘ON‘)
class Kls(object):
def __init__(self,data):
self.data = data
def do_reset(self):
if checkind():
print(‘Reset done for:‘, self.data)
def set_db(self):
if checkind():
self.db = ‘new db connection‘
print(‘DB connection made for:‘,self.data)
ik1 = Kls(12)
ik1.do_reset()
ik1.set_db()
輸出:
Reset done for: 12
DB connection made for: 12
如果使用@staticmethod就能把相關的代碼放到對應的位置了.
IND = ‘ON‘
class Kls(object):
def __init__(self, data):
self.data = data
@staticmethod
def checkind():
return (IND == ‘ON‘)
def do_reset(self):
if self.checkind():
print(‘Reset done for:‘, self.data)
def set_db(self):
if self.checkind():
self.db = ‘New db connection‘
print(‘DB connection made for: ‘, self.data)
ik1 = Kls(12)
ik1.do_reset()
ik1.set_db()
輸出:
Reset done for: 12
DB connection made for: 12
下面這個更全面的代碼和圖示來展示這兩種方法的不同
@staticmethod 和 @classmethod的不同
class Kls(object):
def __init__(self, data):
self.data = data
def printd(self):
print(self.data)
@staticmethod
def smethod(*arg):
print(‘Static:‘, arg)
@classmethod
def cmethod(*arg):
print(‘Class:‘, arg)
>>> ik = Kls(23)
>>> ik.printd()
23
>>> ik.smethod()
Static: ()
>>> ik.cmethod()
Class: (<class ‘__main__.Kls‘>,)
>>> Kls.printd()
TypeError: unbound method printd() must be called with Kls instance as first argument (got nothing instead)
>>> Kls.smethod()
Static: ()
>>> Kls.cmethod()
Class: (<class ‘__main__.Kls‘>,)
下面這個圖解釋了以上代碼是怎麽運行的:
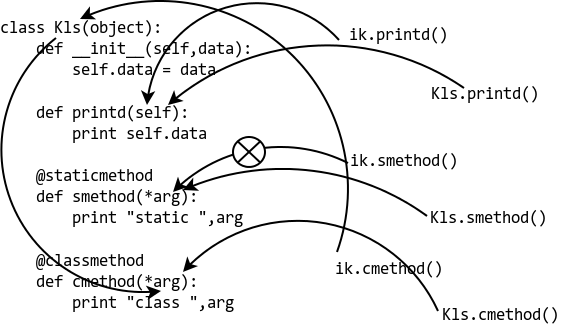
Python 中的 classmethod 和 staticmethod