【LeetCode】73. Set Matrix Zeroes(C++)
阿新 • • 發佈:2018-12-06
地址:https://leetcode.com/problems/set-matrix-zeroes/
題目:
Given a x matrix, if an element is 0, set its entire row and column to 0. Do it in-place
Example 1:
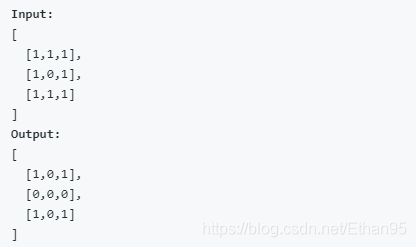
Example 2:
Follow up:
- A straight forward solution using space is probably a bad idea.
- A simple improvement uses space, but still not the best solution.
- Could you devise a constant space solution?
理解:
就是如果原陣列的某個位置是0,把其所在行列都置為0
straight forward的想法就是用一個新陣列嘍,複雜度是
。
這個題麻煩的地方是如果某個元素是0,把行列都置為0會影響後面的判斷,因此可以把需要置0的位置存成一個和所有數都不相同的數,這樣就不會混淆了。但是這樣的時間複雜度很高。
實現1:
的思路就是記錄下應該是0的行和列,最後再賦值一次。
不過這裡用unordered_set
好像很慢的樣子,可以用陣列
class Solution {
public:
void setZeroes(vector<vector<int>>& matrix) {
unordered_set<int> rs, cs;
for (int i = 0; i < matrix.size(); ++i) {
for (int j = 0; j < matrix[0].size(); ++j) {
if (matrix[i][j] == 0) {
rs.insert(i);
cs.insert(j);
}
}
}
for (auto i : rs) {
for (int j = 0; j < matrix[0].size(); ++j)
matrix[i][j] = 0;
}
for (auto j : cs) {
for (int i = 0; i < matrix.size(); ++i)
matrix[i][j] = 0;
}
}
};
實現2:
如果要求空間複雜度為
的話,可以借第一行和第一列來表示是否應該把該行或者該列置為0。對於matrix[i][j]
可以用matrix[0][j]和matrix[i][0]來表示。注意到對於matrix[0][0],兩個位置是重合的,因此需要一個額外的空間來表示。也就是matrix[0][0]表示的是第0行應該置為0,firstCol表示第0列應該置為0。
class Solution {
public:
void setZeroes(vector<vector<int>>& matrix) {
bool firstCol=false;
int r=matrix.size(),c=matrix[0].size();
for(int i=0;i<r;++i){
if(matrix[i][0]==0)
firstCol=true;
for(int j=1;j<c;++j){
if(matrix[i][j]==0){
matrix[i][0]=0;
matrix[0][j]=0;
}
}
}
for(int i=1;i<r;++i){
for(int j=1;j<c;++j){
if(matrix[i][0]==0||matrix[0][j]==0)
matrix[i][j]=0;
}
}
if(matrix[0][0]==0)
for(int j=1;j<c;++j)
matrix[0][j]=0;
if(firstCol)
for(int i=0;i<r;++i)
matrix[i][0]=0;
}
};