【資料結構週週練】022 從大到小輸出二叉排序樹中小於某個值的所有結點編號及資料
阿新 • • 發佈:2018-12-19
一、二叉排序樹
今天給大家分享的是二叉排序樹的應用,從大到小輸出二叉排序樹中小於某個值的所有結點編號及資料。
我們知道,我們做中序遍歷時,先訪問左子樹,再訪問根節點,最後訪問右子樹;通過中序遍歷會得到一個遞增的序列。該應用要求得到從大到小,一個遞減的序列,我們可以通過先訪問右子樹,再訪問根節點,最後訪問左子樹,就可以得到一個遞減的序列。
當然我們還可以使用中序遍歷,將資料存到棧中輸出,就可以逆序輸出了;或者我們可以利用頭插法建立單鏈表,然後遍歷單鏈表輸出也能滿足需求,就是程式碼量比較多啦,大家可以嘗試自己寫一下。
為了讓大家能更好的看到效果,我們支援使用者自己定義該資料。
二、示例
給定下面的二叉排序樹和一個值,從大到小輸出二叉排序樹中小於該值的所有結點編號及資料。其中圓角矩形內為結點資料,旁邊數字為結點編號,箭頭指向的結點為箭尾的孩子結點。
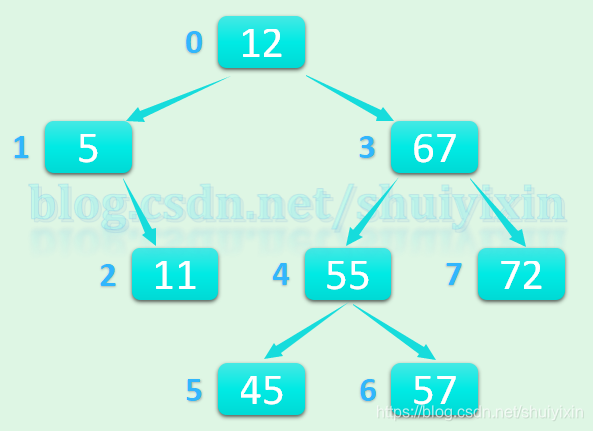
三、程式碼
#include<iostream> #include<malloc.h> using namespace std; typedef struct BiSTNode { int data; int number; struct BiSTNode *lChild, *rChild; }BiSTNode, *BiSortTree; int numData[] = { 12,5,11,67,55,45,57,72 }; int length = sizeof(numData) / sizeof(int); int number = 0; int OperationBiSortTree(BiSortTree &BST, int data) { BST = (BiSortTree)malloc(sizeof(BiSTNode)); if (!BST) { cout << "空間分配失敗(Allocate space failure.)" << endl; exit(OVERFLOW); } BST->data = data; BST->number = number++; BST->lChild = NULL; BST->rChild = NULL; return 1; } int EstablishBiSortTree(BiSortTree &BST) { BiSortTree p = BST; if (!BST) { OperationBiSortTree(BST, numData[number]); } else if (BST->data == numData[number]) { cout << "This data \" " << BST->data << " \" is existing.\n"; number++; return 0; } else if (BST->data > numData[number]) EstablishBiSortTree(BST->lChild); else EstablishBiSortTree(BST->rChild); return 1; } void VisitTree(BiSortTree BST,int data) { if (BST->rChild) VisitTree(BST->rChild,data); if (BST->data<data) { cout << "The number of the current node is " << BST->number << " ,and the data is " << BST->data << " ;\n"; } if (BST->lChild) VisitTree(BST->lChild,data); } void main() { BiSortTree BST = NULL; int data ; while (number<length) { EstablishBiSortTree(BST); } cout << "Please input a data and we will output all data which smaller than the data:"; cin >> data; VisitTree(BST, data); }