演算法--全排列、全子集、DFS\BFS問題
阿新 • • 發佈:2018-12-24
排列組合
子集合 subset(medium)
輸入一個含有不同數字的序列,輸出所有可能的集合(含空集)。要求1 集合裡元素有序排列;2 輸出結果不含有重複集合;
舉例:輸入{1,2,3], 輸出{},{1},{2},{3},{1,2},{1,3},{2,3},{1,2,3}
原題:https://leetcode.com/problems/subsets/
思路圖:
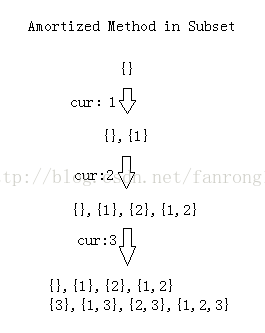
Amortized 分期償還(只是個名字而已)的思路:
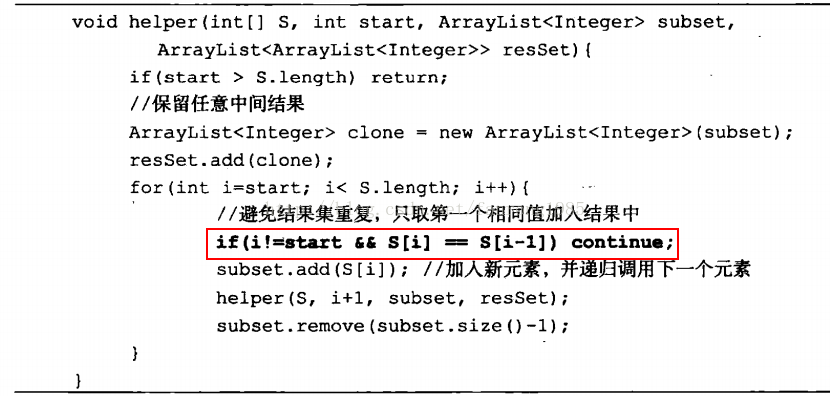
--------------------------------------------------------------------------------- 全排列permutation( Medium) 題目內容,輸入一個不含相同數字的序列,輸出所有可能的排列permutation, 需要注意順序 舉例 輸入{ 1,2,3 } 返回: {1,2,3},{1,3,2},{2,1,3},{2,3,1},{3,1,2},{3,2,1} 原題:https://leetcode.com/problems/permutations/ 思路:與全子集相似,使用遞迴方法 區別: 必須等到所有數字均在集合裡才能輸出。所以需要輔助陣列bool[] used=new bool[arr.Length]來記錄元素使用情況 問題:為什麼元素不能重複使用?為什麼架構與subset不同?
自我解答,歡迎補充:使用1,2作為輸入,確實能夠得到答案,只能說此架構設計比較巧妙。長期來看只能靠記憶
Amortized 分期償還(只是個名字而已)的思路:
遞迴程式碼(DFS):public static IList<IList<int>> Subsets(int[] nums) { IList<IList<int>> resSet = new List<IList<int>>(); Array.Sort(nums); //初始化,加入空集 resSet.Add(new List<int>()); for (int i = 0; i < nums.Length; i++) { IList<IList<int>> tmp = new List<IList<int>>(); foreach (var list in resSet) { //保留原結果 tmp.Add(list); List<int> clone = new List<int>(list); //在原有結果里加入新元素 clone.Add(nums[i]); tmp.Add(clone); } resSet = tmp; } return resSet; }
備註:變種思路,如果輸入陣列有重複值怎麼辦? 答案 1 主函式中需要先排序,為Helper函式去重打基礎; 2 如果s[i]==s[i-1],直接跳到下一步;public static IList<IList<int>> SubSetRecrisive(int[] ints) { IList<IList<int>> resSet = new List<IList<int>>(); if (ints == null || ints.Length == 0) { return resSet; } //Array.Sort(ints);//排序是為了方便有重複輸入時判斷重複; Helper(ints, 0, new List<int>(), resSet); return resSet; } public static void Helper(int[] s, int start, List<int> subset, IList<IList<int>> resSet) { //保留中間結果,不能直接新增resSet,因為這是傳址,它每次都變化; IList<int> clone = new List<int>(subset); resSet.Add(clone); for (int i = start; i < s.Length; i++) { subset.Add(s[i]);//加入新元素,並遞迴呼叫下一個元素; Helper(s,i+1,subset,resSet); subset.RemoveAt(subset.Count-1);//退回,移除subset中最後一個索引位元素 } }
--------------------------------------------------------------------------------- 全排列permutation( Medium) 題目內容,輸入一個不含相同數字的序列,輸出所有可能的排列permutation, 需要注意順序 舉例 輸入{ 1,2,3 } 返回: {1,2,3},{1,3,2},{2,1,3},{2,3,1},{3,1,2},{3,2,1} 原題:https://leetcode.com/problems/permutations/ 思路:與全子集相似,使用遞迴方法 區別: 必須等到所有數字均在集合裡才能輸出。所以需要輔助陣列bool[] used=new bool[arr.Length]來記錄元素使用情況 問題:為什麼元素不能重複使用?為什麼架構與subset不同?
public static IList<IList<int>> PermuteRecrusive(int[] ints)
{
IList<IList<int>> resSet = new List<IList<int>>();
if (ints == null || ints.Length == 0)
{
return resSet;
}
Array.Sort(ints);
PermuteHelper(ints, new List<int>(), resSet);
return resSet;
}
/// <summary>
/// 1 為什麼迴圈中不需要pos引數? 2為什麼引數不能重複使用?
/// </summary>
/// <param name="ints"></param>
/// <param name="subset"></param>
/// <param name="resSet"></param>
/// <param name="used"></param>
public static void PermuteHelper(int[] ints, List<int> subset, IList<IList<int>> resSet)
{
if (subset.Count == ints.Length)
{
resSet.Add(new List<int>(subset));
return; //為什麼需要這個?因為add後需要回到上一層遞迴;
}
for (int i = 0; i < ints.Length; i++)
{
if (subset.Contains(ints[i])) continue; //不能重複使用已有元素,為什麼?
subset.Add(ints[i]);//加入新元素,遞迴呼叫下一個元素
PermuteHelper(ints, subset, resSet);
subset.RemoveAt(subset.Count-1);//回退
}
}