Chatting it up with NestJS and Angular 6: Setting up the Project
Chatting it up with NestJS and Angular 6: Setting up the Project
This is the first article in a series covering the development of a chat application. Why a chat application? A chat application might seem simple but it provides the best opportunity to work on both front-end and back-end code as well as typical RESTful APIs and real-time communication technology. A chat application could be a great jumping off point for a number of different project types including game development.
For this first article, we will cover project setup to the point of having a running front-end and back-end application. Feel free to follow along on your own computer. If you would rather just have the code, clone or fork the companion repository here.
git clone [email protected]:patrickhousley/nestjs-angular-chat-example.gitgit checkout part-01
Tools
To get started on this project, we are going to need some tools. Most notably, we will be using the new Angular Console by the Nrwl team. You can grab a copy from their Github repository for your OS. There are also some global Node module that you may want to install, especially if you want to stick to the CLIs. Run the below command in a terminal window:
npm i -g @angular/cli @nrwl/schematics @nestjs/cli
I will try to make a point of showing the Angular Console and the CLI ways of doing things in this article. I will also be using VSCode as the IDE but you are welcome to use whatever you like.
Project Setup
Now that we have all of our tools, let’s get the project going. Open up the Angular Console and create a new project. You will need to pick a location on your computer to place the new project, type a project name, and select the correct schematic. For this article, I will be naming the project nestjs-angular-chat-example
and I will be using the @nrwl/schematics
schematics. In order to follow along, make sure you pick the right schematic. You can also generate the project using ng new nestjs-angular-chat-example [email protected]/schematics
.

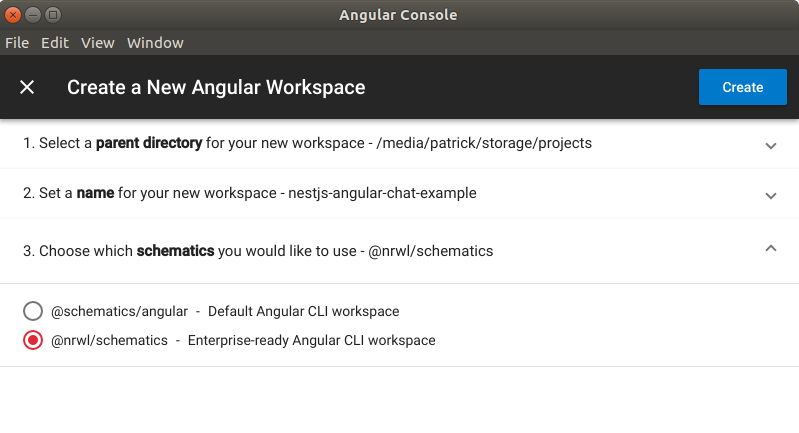
If you open the project in your IDE, it’s probably pretty blank at this point. Let’s throw in a project for our front-end Angular code. In the Angular Console, select the generate code tab and we will be generating an application. You can also generate the client application using ng generate application client --routing --style=scss
.

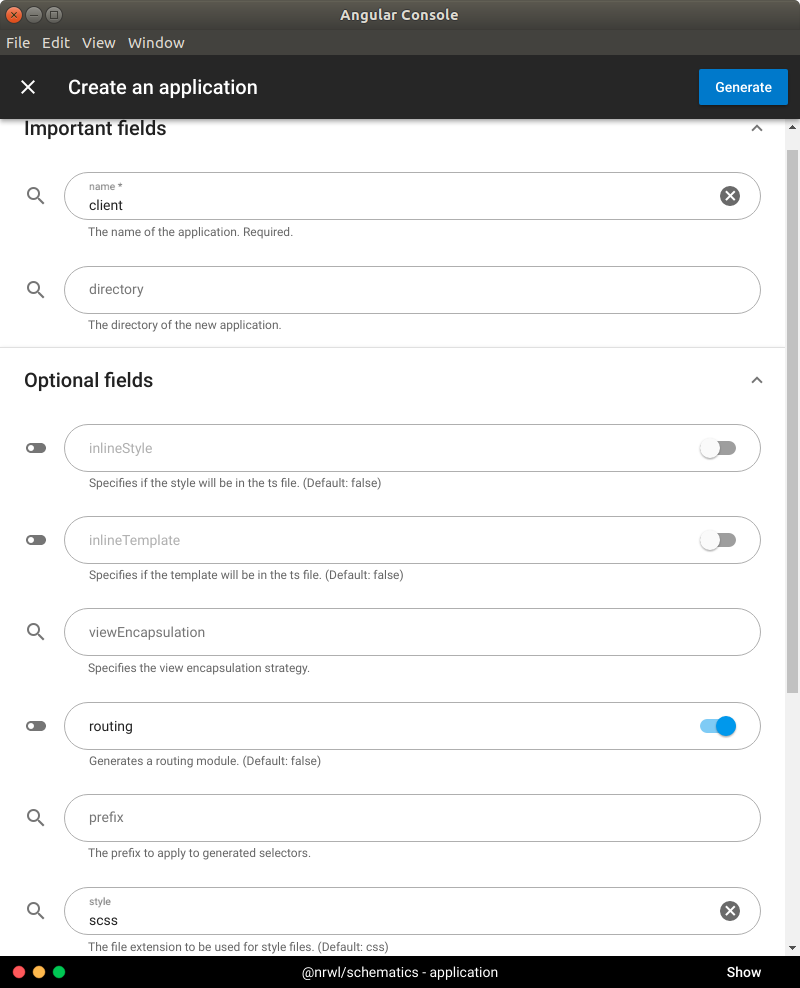
We will do the same thing to generate the server application. You can also generate the service application using ng generate application server
.

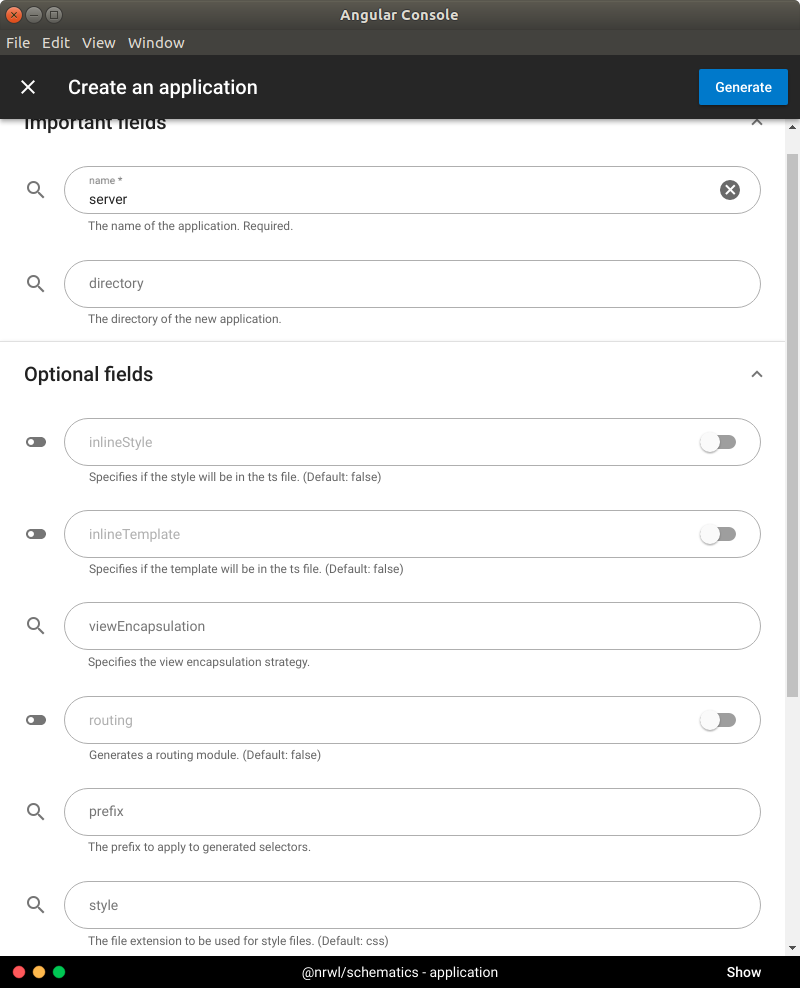
The last piece we need is a place to put any code we might share between the client and server. Typically when writing RESTful services using this setup, I will share interfaces that describe the input and output of the APIs to make the client development easier and more type safe. For this, we will generate a library simply called core
. You can also generate the library using ng generate library core
.

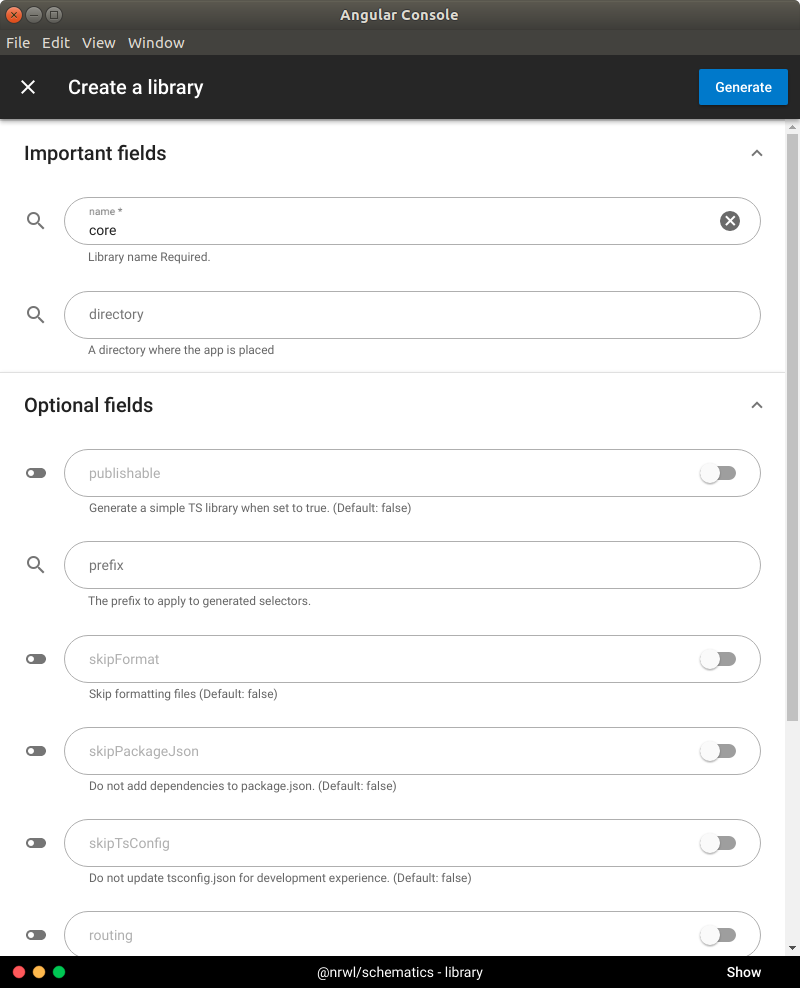
If you have followed along, you should now have a project with two applications and a shared library. Below is what the project should resemble for project structure and configuration.

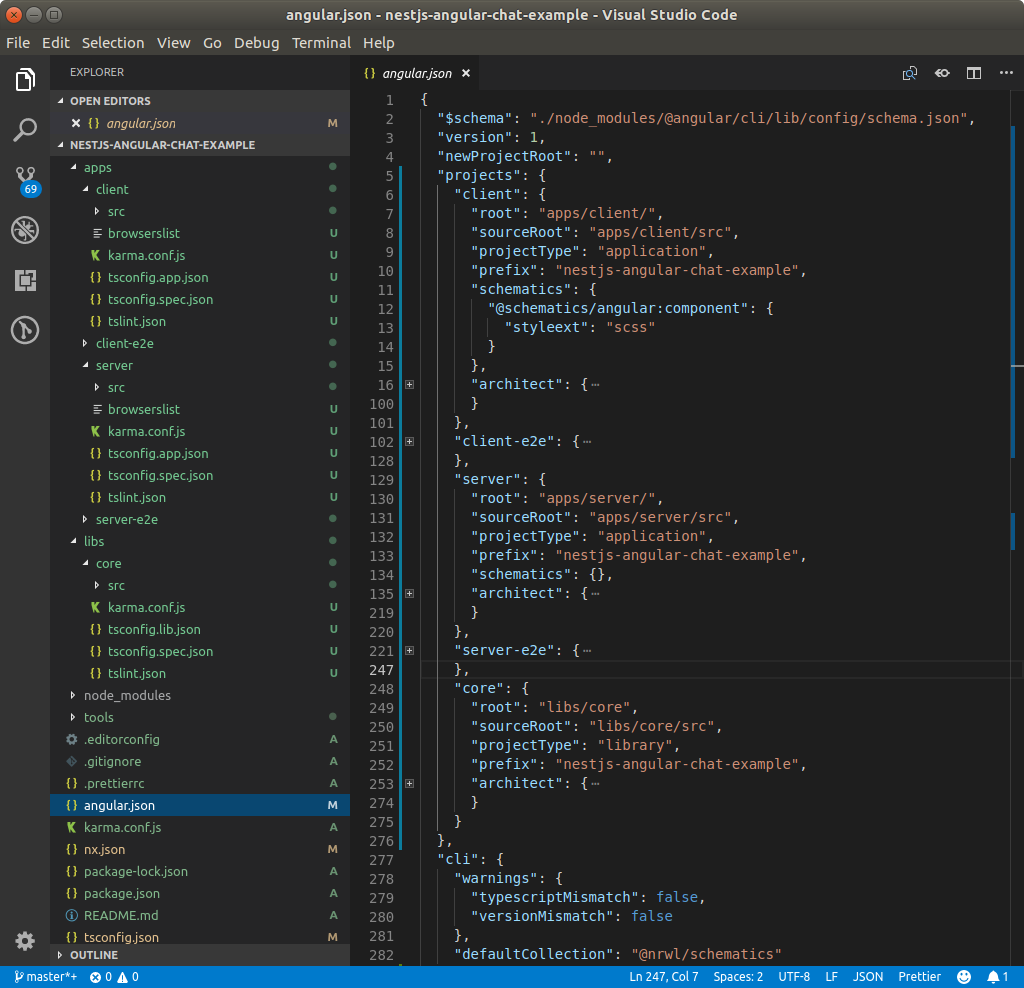
Why Nrwl Nx?
As you can see from the project structure, this is no typical Angular CLI project. If you have never used or heard about Nrwl Nx of the Angular Console, this might all look a little confusing. It’s actually pretty simple.
Nrwl Nx is a set of extensions for the Angular CLI that support working with a monorepo. Typically, using the Angular CLI, you would end up with a src directory in the root of your project and that would be where your primary app lives. But what about any shared code? Or maybe you want two app? What about an Express or Node server?
So all those things can be done while using the normal Angular CLI. The difference is, Nrwl Nx structures the project and provides some additional schematics that make working with multiple apps and shared code in the same repository easy.
Generate the Server
So you might be thinking, we already generated the server application using Nrwl Nx. Well yes but it generated it as an Angular project and we want to use NestJS. Before we generate the NestJS files, we need to cleanup our server application files.
First off, go ahead and delete the src
directory and the browserlists
and karma.conf.js
files in the apps/server
directory. Also delete the src
directory and protractor.conf.js
file in the apps/server-e2e
directory. Open up the apps/server/tsconfig.app.json
file and add the below lines to the compiler options.
"module": "commonjs","target": "es6",
Open a terminal into your project folder and change directory into the apps/server
directory. From here, we will use nest new
to generate our NestJS project.

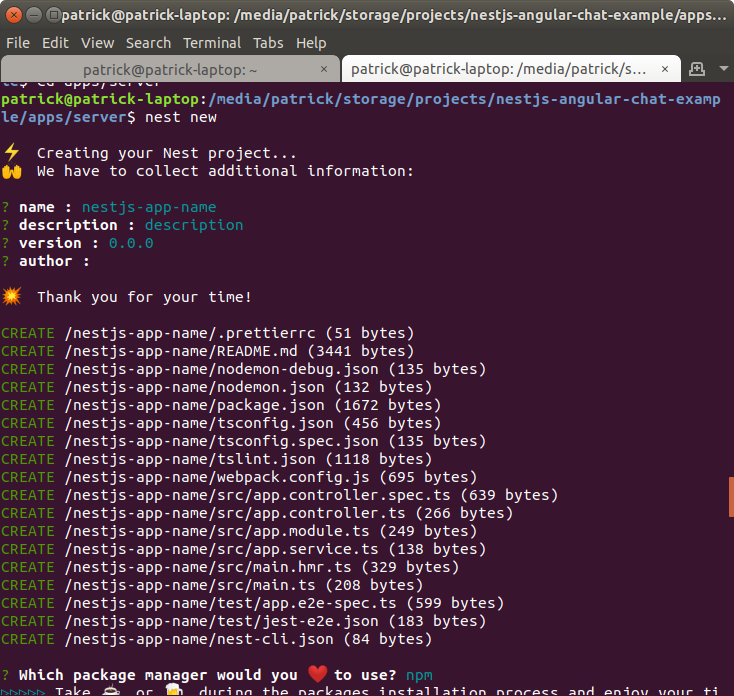
Use the default values provided for the name, description, version, and author. The CLI will generate a new folder with all the files we need but we wont be keeping them all. While you are in the terminal, go ahead and install the necessary NPM packages for NestJS.
npm i --save @nestjs/core @nestjs/common reflect-metadatanpm i -D @nestjs/testing
@types/jest @types/supertest supertest
Now that we have a generated NestJS project, we need to move the files and folders we care about out of the generated directory into our apps/server
and apps/server-e2e
directories.
cd nestjs-app-namemv src ../mkdir ../../server-e2e/srcmv test/* ../../server-e2e/src/
The angular.json
configuration must also be updated to make sure the Angular CLI knows how to build the NestJS application. Before we can do this, we will need to run npm i -D @angular-builders/custom-webpack
. Modify the server
section the of Angular CLI configuration to the below:
"server": { "root": "apps/server/", "sourceRoot": "apps/server/src", "projectType": "application", "prefix": "nestjs-angular-chat-example", "schematics": {}, "architect": { "build": { "builder": "@angular-builders/custom-webpack:server", "options": { "customWebpackConfig": { "path": "apps/server/webpack.config.js" }, "outputPath": "dist/apps/server", "main": "apps/server/src/main.ts", "tsConfig": "apps/server/tsconfig.app.json" }, "configurations": { "production": { "fileReplacements": [ { "replace": "apps/server/src/environments/environment.ts", "with": "apps/server/src/environments/environment.prod.ts" } ] } } }, ... }}
Run ng build server
followed by node dist/apps/server/main.js
and you should have a very basic NestJS server up and running. ? HURRAH ?
Conclusion
At this point, you should have a front-end and back-end application that can be ran through the console or through the Angular Console. They don’t really do much and in fact, they don’t even talk to each other. It might seem like we did a lot of work to get to this point. You might even be thinking what we have created uses on over-complicated amalgamation of CLI technologies for the simple purpose of creating a monorepo-styled proejct. You might honestly be right. In fact, there are many ways you could go about combining Angular and NestJS in a single project. Here is one example I created some time ago that simply places a server
directory in an Angular CLI generated project. And while that works and is probably fine for almost all projects, it is not, in my opinion, well structured to support future growth.
That’s where the tools the Nrwl team have been working on, like the Angular Console and Nrwl Nx, comes in. The Nrwl team have found a great way of structuring a monorepo-styled project that should support growth through the addition of new front-end and back-end apps and shared libraries.
In the remaining articles of this series, we will setup the front-end to talk to the back-end, create a “pretty” UI for users to chat with their friends, and use the NestJS websockets module to setup real-time communication. We will also touch on testing using my favorite testing framework, Jest. Stay tuned.