What college students should learn about Git
Git is one of the most popular source control tools in the universe(?). It allows for large scale collaboration with some simple commands. The following article will share the most basic git commands, add, commit, push, and pull, to start the journey towards becoming a git expert.
I urge you to dive deeper once you’ve become comfortable with the following commands. Let’s begin!
Workspace, [Staging], Repository, and Remote

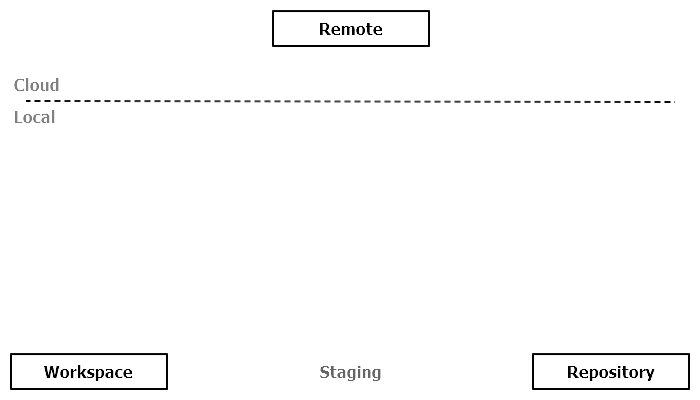
Git organizes changes into three (four?) different *locations; workspace, local repository, and remote repository. Understanding these *locations is necessary to understanding the git commands.
*Locations is not a technical term. I’m not sure what word to use to group them.
Workspace
The workspace is the local folders and files associated with the local repository. Git will recognize the changes you make here automagically.
[Local] Repository
Use git commands to save workspace changes to the local repository. It’s important to note that changing folders and files in the workspace will not change anything about the local repository. Only git commands will change the local repository.
Remote [Repository]
This is the repository that lives in the cloud. Although it is a copy of the local repository it is also separate from the local repository. Local repository changes will not show up in the remote repository until the correct git commands are executed.
Again, changing the workspace will not change the local or remote repositories. Git commands will orchestrate those changes.
[Staging]
Staging is like a lobby area between the workspace and repository. Only files that get sent to the lobby will go to the repository. So, it’s important to know that this state exists but don’t think too hard about it.
Workspace → [Local] Repository
Changes in the workspace will eventually end up in the local repository. This is called committing changes but it’s done as a two step process.
Workspace → [Staging]


1: git add .2: 3: git add [filename]4: git add [regular expression]
Line 1 will probably be the most used command because it stages all ofthe changed files in the workspace. Line 3 will stage a specific file and line 4 will stage files that match the regular expression. Start with git add .
for convenience.
Note, only staged files can be committed (saved to the local repository). It’s just how it works. So, don’t forget to stage the files you want to commit (save to the local repository).
[Staging] → [Local] Repository

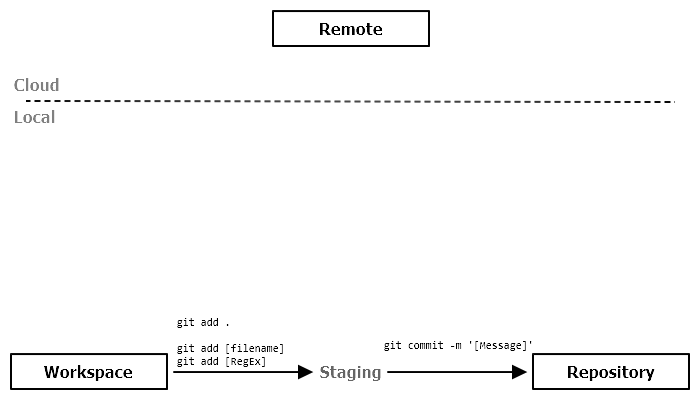
1: git commit -m '[Your Message Here]'
Line 1 will take the staged files and commit them to the local repository. The -m '[Your Message Here]'
signifies the message attached to the commit.
Do yourself a favor and write a message that will make sense five months from now. Technically it doesn’t matter what the message is but well written messages makes searching for specific changes easier.
The One Step Commit
1: git commit -a -m '[Your Message Here]'
Line 1 will do the staging and committing as one command. This is equivalent to the following:
1: git add .2: git commit -m '[Your Message Here]'
[Local] Repository → Remote [Repository]


1: git push2:3: git push origin master4: git push -u origin master
To share the local repository with the world, put them in the remote repository. To do this, push the commits from the local repository to the remote repository.
Line 3 is technically what gets executed in line 1. That means line 1 implicitly adds the origin master
to the execution. If for some reason executing line 1 doesn’t work, try line 4 and then line 1 should work. To explain further is unnecessary at this point.
Remote [Repository] → Workspace


1: git pull2: 3: git pull origin master
Source control is not only used to save information but to allow for collaborative work. Other developers will be able to fork your remote repository, make changes in their local repository, push those changes to their remote repository, and then send a pull request to the original remote repository. That probably sounds like a lot of gibberish right now so I’ll get to the point.
Other people can change your remote repository (with your permission) and you can pull those changes into your local repository and workspace. Line 1, git pull
, is how you do that.
This is how companies work. Everyone works in their own local workspace and local repository and when they’re ready to share that code with the rest of the company then they push those commits to the remote repository. At that point, it will be available to get pulled at the developers’ convenience.
Some things to consider…
Conflicts
How is it possible to collaborate on the same files without having any issues merging those changes together? Well, you’re likely to come across what are called merge conflicts. These can happen when you pull the code from the remote repository to your workspace.
Here’s an example of how this happens. Let’s say we have a “README.md” file and Amy and Frank make a change on the same line; let’s say line 5. Frank pushes those changes to the remote repository first and then Amy pulls those changes into her workspace. Amy will get a merge conflict because git won’t know whether or not to take Frank’s change over Amy’s. At this point, Amy gets to decide what line 5 will say. It can be Frank’s change, Amy’s change, both their changes, or Amy can completely rewrite line 5.
It’s important to talk with your team if you are unsure of what changes to keep when a conflict happens.
Branches
One of git’s most powerful features is how it handles branching. It’s a topic I’m not going to cover in this article but an important topic to tackle after learning the basics. It’s really the reason why so many people use git. So, even though I’m not covering it, here is what you should know.
The word master
is the default branch and is referenced implicitly in the git commands we’ve learned. We can change what branch to work in but I recommend working only in master until the basics are understood. Branching will be the next topic to tackle.
Status
1: git status
There is no UI to show the state of all these folders and files within the workspace and repositories. In order to get a sense of the state of everything we’ve talked about, use git status
. I highly recommend executing that command often.
Help
1: git help2: git help [command]
The help command gives a brief description of all the possible commands. Again, focus on the commands we’ve talked about (add, commit, push, and pull) but use git help
to reference the commands when you forget them. It’ll be easier and faster then looking up an article like this one.
Overview


Start using git and focus on the following commands. These will allow you to work effectively without diving deep into the inner workings of git.
Helpful Commands
git helpgit status
Workspace → [Local] Repository
git commit -a -m 'Your message here'
[Local] Repository → Remote [Repository]
git push
Remote [Repository] → Workspace
git pull
Keep your eyes open for a tutorial creating a GitHub repository and going through all the steps above and more with pictures.