A Guide to AWS Lambdas using Python triggered by an API call
A Guide to AWS Lambdas using Python, triggered by an API call

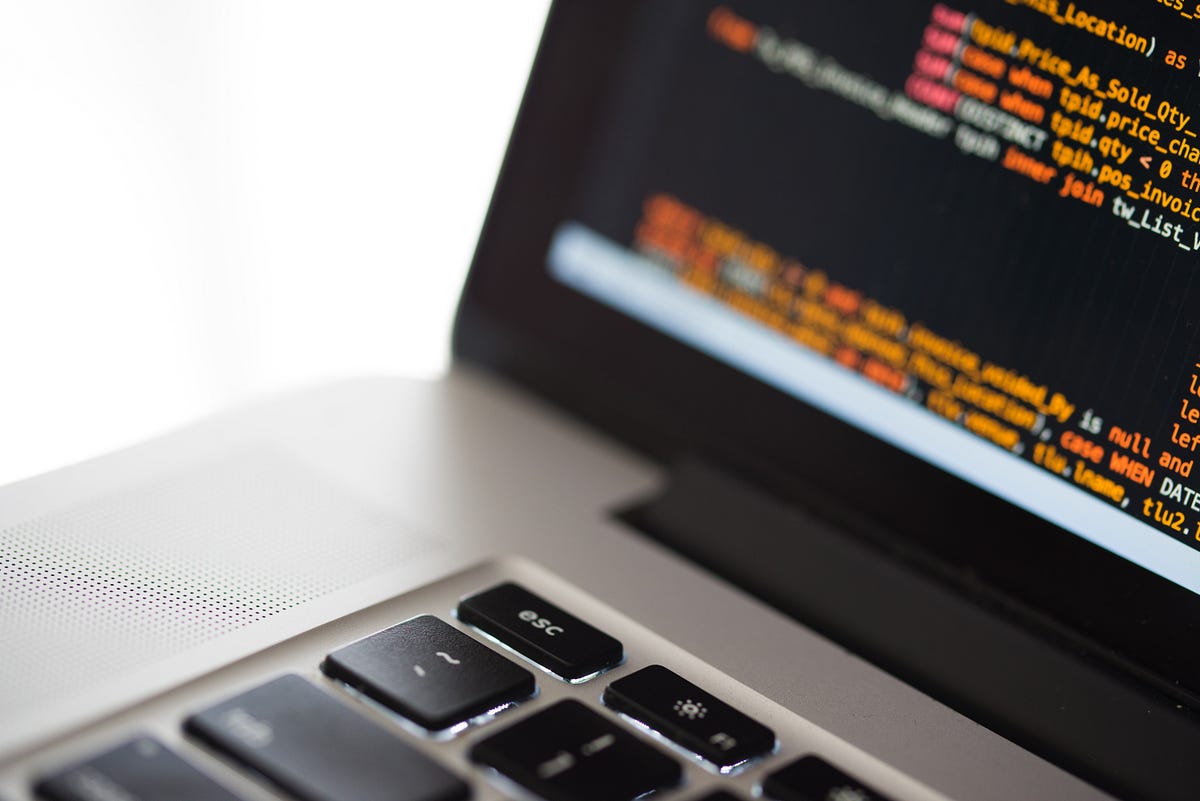
Serverless computing has been a topic of discussion off late. Serverless computing is the abstraction of servers, OS, infrastructure while retaining the core function- computing. Serverless computing has its own advantages. Developers should not have to worry about setting up entire servers before they can actually use their functions. Additionally, certain applications are better suited for computing based billing as opposed to time-based billing. Lambdas help to achieve exactly this. In very simple terms, lambdas are isolated functions that are assigned just one task. Scalability and hassle-free resource allocation are some of the other advantages of using this type of architecture. All major cloud service providers(
Following are the steps that we would follow:
- Lambda Creation
- Lambda Deployment
- Configuration of the API gateway
This guide assumes an active AWS account and some patience.
Lambda Creation
A simple lambda will look something like this:
def lambda_handler(event, context): #lambda function here ... return { 'status' : 200, 'body' : 'Lambda executed successfully!' }
This method will go in an entry point, let’s call it main
The following is taken from the official docs:
event
– AWS Lambda uses this parameter to pass in event data to the handler. This parameter is usually of the Pythondict
type. It can also belist
,str
,int
,float
, orNoneType
type.context
– AWS Lambda uses this parameter to provide runtime information to your handler. This parameter is of theLambdaContext
type.
Handling additional dependencies
Lambdas are hosted on Linux servers which already have basic packages installed. All additional dependencies will have to be explicitly put in the deployment package in a fixed format, which is described below.
In order to smoothen up this process, have a virtual environment for the particular project. To create a deployment package, create a zip with the main entry point file main.py
at the root level of the zip. Additional folders and files can be present in the zip in any format you deem fit. Make sure to not have absolute paths in your project.
It should look something like this:
lambda-test.zip/├── main.py(lambda entry point containing the lambda_handler method├── Folder1/│ ├── File1│ ├── File2│ ├── some_method.py│ └── docs├── SomeOtherFolder/│ ├── File1│ └── docs├── package1├── package2├── package3└── package4
Adding dependencies: Copy all files and folders from venv/lib/python2.7/site-packages/
to the root of the zip (packageX in the above fig.). Here venv
is the virtual environment folder for the project. Adhere to the size limits set by Amazon. Let’s call this zip package lambda-test.zip