swift新手進階30天一 自定義上圖片下文字的UIButton的幾種方式
阿新 • • 發佈:2018-12-30
目前很多app首頁功能區都類似工具欄上圖示加下文字的方式來自定義按鈕。當然,我們也可以用兩個控制元件實現,但是,提升不了我們的逼格。接下來就介紹幾種自定義這種上圖示下文字的按鈕的幾種方式。先上圖。
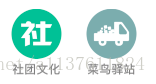
一、利用屬性文字NSAttributedString來生成圖片文字上下排列的按鈕
1.複寫NSAttributedString方法
// MARK: -使用影象和文字生成上下排列的屬性文字
extension NSAttributedString {
//將圖片屬性以及文字屬性用方法名傳入
class func imageTextInit(image: UIImage, imageW: CGFloat, imageH: CGFloat, labelTitle: String, fontSize: CGFloat, titleColor: UIColor, labelSpacing: CGFloat) -> NSAttributedString{
//1.將圖片轉換成屬性文字
let attachment = NSTextAttachment()
attachment.image = image
attachment.bounds = CGRectMake(0, 0, imageW, imageH)
let imageText = NSAttributedString(attachment: attachment)
//2.將標題轉化為屬性文字
let titleDict = [NSFontAttributeName: UIFont.systemFontOfSize(fontSize), NSForegroundColorAttributeName: titleColor]
let text = NSAttributedString(string: labelTitle, attributes: titleDict)
//3.換行文字可以讓label長度不夠時自動換行
let spaceDict = [NSFontAttributeName:UIFont.systemFontOfSize(labelSpacing)]
let lineText = NSAttributedString(string: "\n\n", attributes: spaceDict)
//4.合併屬性文字
let mutableAttr = NSMutableAttributedString(attributedString: imageText)
mutableAttr.appendAttributedString(lineText)
mutableAttr.appendAttributedString(text)
//5.將屬性文字返回
return mutableAttr.copy() as! NSAttributedString
}
}
2.寫好了屬性文字,接下來就把新增進UIButton中去
class func YWHomeListButton(imageName: String, titleName: String!) -> UIButton {
//建立按鈕型別為Custom
let btn = UIButton(type: .Custom)
//獲取我們剛得到的屬性文字,直接是NSAttributedString的子方法了
let attrStr = NSAttributedString.imageTextInit(UIImage(named: imageName)!, imageW: SCREEN_WIDTH/8*3/2, imageH: SCREEN_WIDTH/8, labelTitle: titleName, fontSize: 12, titleColor: UIColor.lightGrayColor(), labelSpacing: 4)
//設定按鈕基本屬性
btn.titleLabel?.textAlignment = .Center
btn.titleLabel?.numberOfLines = 0
btn.setAttributedTitle(attrStr, forState: .Normal)
return btn
}
3.接下來就可以用我們剛自己定義的按鈕了
let btn1 = UIButton.YWHomeListButton("homeList1", titleName: "抽獎")
let btn2 = UIButton.YWHomeListButton("homeList2", titleName: "領紅包")
let btn3 = UIButton.YWHomeListButton("homeList3", titleName: "蜂抱團")
let btn4 = UIButton.YWHomeListButton("homeList4", titleName: "一元購")
二、修改UIButton系統的titleRectForContentRect和imageRectForContentRect方法來改變文字和圖片的相對位置
1.通過計算得出我們需要的上下排列的效果
import UIKit
class YWButton: UIButton {
override init(frame: CGRect) {
super.init(frame: frame)
commonInit()
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
//設定按鈕的基本屬性
func commonInit() {
self.titleLabel?.textAlignment = .Center
self.imageView?.contentMode = .ScaleAspectFit
self.titleLabel?.font = UIFont.systemFontOfSize(12)
}
//調整系統UIButton的文字的位置
override func titleRectForContentRect(contentRect: CGRect) -> CGRect {
let titleX = 0
let titleY = contentRect.size.height * 0.35
let titleW = contentRect.size.width
let titleH = contentRect.size.height - titleY
return CGRectMake(CGFloat(titleX), titleY, titleW, titleH)
}
//調整系統UIButton的圖片的位置
override func imageRectForContentRect(contentRect: CGRect) -> CGRect {
let imageW = CGRectGetWidth(contentRect)
let imageH = contentRect.size.height * 0.4
return CGRectMake(0, 5, imageW, imageH)
}
}
2.接下來就可以直接應用我們自己的控制元件了
let basketBtn = YWButton(type: .Custom)
basketBtn.setTitleColor(themeColor, forState: .Normal)
basketBtn.setTitle("購物車", forState: .Normal)
basketBtn.setImage(UIImage(named: "YS_car_sel"), forState: .Normal)
toolBarView.addSubview(basketBtn)
let storeBtn = YWButton(type: .Custom)
storeBtn.setTitleColor(themeColor, forState: .Normal)
storeBtn.setTitle("店鋪", forState: .Normal)
storeBtn.setImage(UIImage(named: "ysstoreIcon"), forState: .Normal)
toolBarView.addSubview(storeBtn)
三、修改UIButton的EdgeInset屬性來改變文字與圖片的相對位置
extension UIButton {
func layoutButton(imageTitleSpace: CGFloat) {
//得到imageView和titleLabel的寬高
let imageWidth = self.imageView?.frame.size.width
let imageHeight = self.imageView?.frame.size.height
var labelWidth: CGFloat! = 0.0
var labelHeight: CGFloat! = 0.0
labelWidth = self.titleLabel?.intrinsicContentSize().width
labelHeight = self.titleLabel?.intrinsicContentSize().height
//初始化imageEdgeInsets和labelEdgeInsets
var imageEdgeInsets = UIEdgeInsetsZero
var labelEdgeInsets = UIEdgeInsetsZero
//重點: 修改imageEdgeInsets和labelEdgeInsets的相對位置的計算
imageEdgeInsets = UIEdgeInsetsMake(-labelHeight-imageTitleSpace/2, 0, 0, -labelWidth)
labelEdgeInsets = UIEdgeInsetsMake(0, -imageWidth!, -imageHeight!-imageTitleSpace/2, 0)
break;
self.titleEdgeInsets = labelEdgeInsets
self.imageEdgeInsets = imageEdgeInsets
}