構建RESTful API(十八)
阿新 • • 發佈:2019-01-07
首先,回顧並詳細說明一下在快速入門中使用的@Controller
、@RestController
、@RequestMapping
註解。如果您對Spring MVC不熟悉並且還沒有嘗試過快速入門案例,建議先看一下快速入門的內容。
@Controller
:修飾class,用來建立處理http請求的物件@RestController
:Spring4之後加入的註解,原來在@Controller
中返回json需要@ResponseBody
來配合,如果直接用@RestController
替代@Controller
就不需要再配置@ResponseBody
,預設返回json格式。@RequestMapping
下面我們嘗試使用Spring MVC來實現一組對User物件操作的RESTful API,配合註釋詳細說明在Spring MVC中如何對映HTTP請求、如何傳參、如何編寫單元測試。
RESTful API具體設計如下:
User實體定義:
1 2 3 4 5 6 7 8 9 |
public
class
User {
private
Long id;
private
String name;
private
Integer age;
// 省略setter和getter
}
|
實現對User物件的操作介面
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
@RestController
@RequestMapping
(value=
"/users"
)
// 通過這裡配置使下面的對映都在/users下
public
class
UserController {
// 建立執行緒安全的Map
static
Map<Long, User> users = Collections.synchronizedMap(
new
HashMap<Long, User>());
@RequestMapping
(value=
"/"
, method=RequestMethod.GET)
public
List<User> getUserList() {
// 處理"/users/"的GET請求,用來獲取使用者列表
// 還可以通過@RequestParam從頁面中傳遞引數來進行查詢條件或者翻頁資訊的傳遞
List<User> r =
new
ArrayList<User>(users.values());
return
r;
}
@RequestMapping
(value=
"/"
, method=RequestMethod.POST)
public
String postUser(
@ModelAttribute
User user) {
// 處理"/users/"的POST請求,用來建立User
// 除了@ModelAttribute繫結引數之外,還可以通過@RequestParam從頁面中傳遞引數
users.put(user.getId(), user);
return
"success"
;
}
@RequestMapping
(value=
"/{id}"
, method=RequestMethod.GET)
public
User getUser(
@PathVariable
Long id) {
// 處理"/users/{id}"的GET請求,用來獲取url中id值的User資訊
// url中的id可通過@PathVariable繫結到函式的引數中
return
users.get(id);
}
@RequestMapping
(value=
"/{id}"
, method=RequestMethod.PUT)
public
String putUser(
@PathVariable
Long id,
@ModelAttribute
User user) {
// 處理"/users/{id}"的PUT請求,用來更新User資訊
User u = users.get(id);
u.setName(user.getName());
u.setAge(user.getAge());
users.put(id, u);
return
"success"
;
}
@RequestMapping
(value=
"/{id}"
, method=RequestMethod.DELETE)
public
String deleteUser(
@PathVariable
Long id) {
// 處理"/users/{id}"的DELETE請求,用來刪除User
users.remove(id);
return
"success"
;
}
}
|
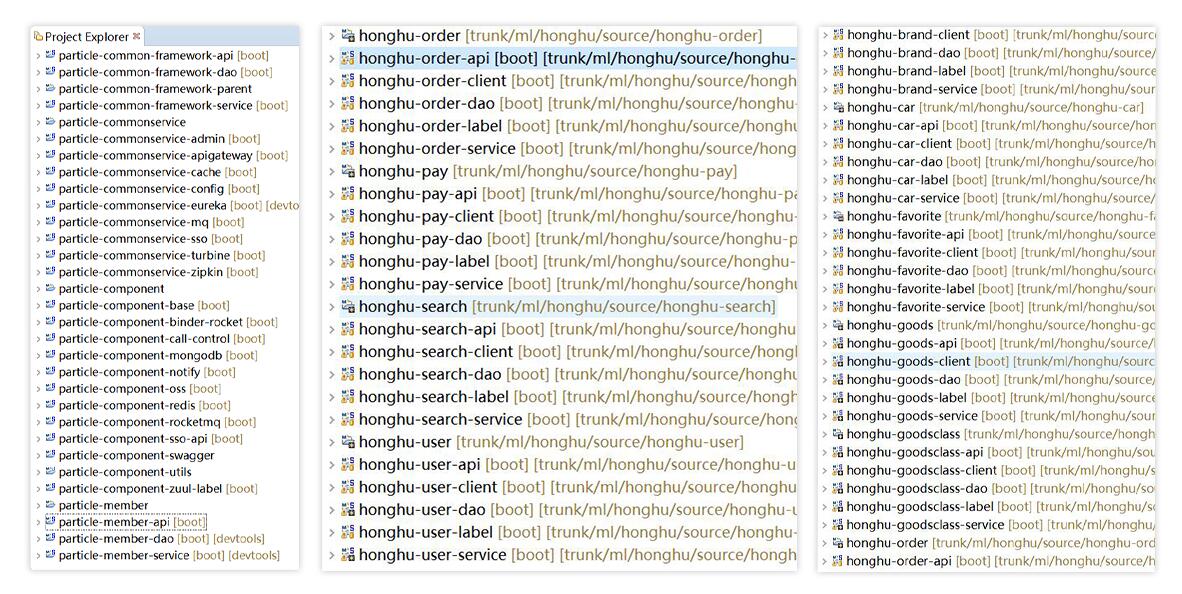