POJ 2528 Mayor's posters(線段樹+離散化)
Time Limit: 1000MS | Memory Limit: 65536K |
Total Submissions: 60691 | Accepted: 17565 |
Description
The citizens of Bytetown, AB, could not stand that the candidates in the mayoral election campaign have been placing their electoral posters at all places at their whim. The city council has finally decided to build an electoral wall for placing the posters and introduce the following rules:- Every candidate can place exactly one poster on the wall.
- All posters are of the same height equal to the height of the wall; the width of a poster can be any integer number of bytes (byte is the unit of length in Bytetown).
- The wall is divided into segments and the width of each segment is one byte.
- Each poster must completely cover a contiguous number of wall segments.
They have built a wall 10000000 bytes long (such that there is enough place for all candidates). When the electoral campaign was restarted, the candidates were placing their posters on the wall and their posters differed widely in width. Moreover, the candidates started placing their posters on wall segments already occupied by other posters. Everyone in Bytetown was curious whose posters will be visible (entirely or in part) on the last day before elections.
Your task is to find the number of visible posters when all the posters are placed given the information about posters' size, their place and order of placement on the electoral wall.
Input
Output
For each input data set print the number of visible posters after all the posters are placed.The picture below illustrates the case of the sample input.
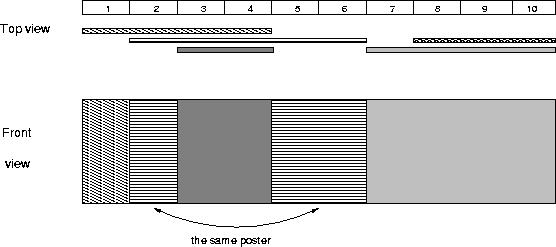
Sample Input
1 5 1 4 2 6 8 10 3 4 7 10
Sample Output
4
題目大意:
題意:在牆上貼海報,海報可以互相覆蓋,問最後可以看見幾張海報
思路:這題資料範圍很大,直接搞超時+超記憶體,需要離散化:
離散化簡單的來說就是隻取我們需要的值來用,比如說區間[1000,2000],[1990,2012]
我們用不到[-∞,999][1001,1989][1991,1999][2001,2011][2013,+∞]這些值,所以我只需要1000,1990,2000,2012就夠了,將其分別對映到0,1,2,3,在於複雜度就大大的降下來了
所以離散化要儲存所有需要用到的值,排序後,分別對映到1~n,這樣複雜度就會小很多很多
而這題的難點在於每個數字其實表示的是一個單位長度(並且一個點),這樣普通的離散化會造成許多錯誤(包括我以前的程式碼,poj這題資料奇弱)
給出下面兩個簡單的例子應該能體現普通離散化的缺陷:
1-10 1-4 5-10
1-10 1-4 6-10
為了解決這種缺陷,我們可以在排序後的陣列上加些處理,比如說[1,2,6,10]
如果相鄰數字間距大於1的話,在其中加上任意一個數字,比如加成[1,2,3,6,7,10],然後再做線段樹就好了.
線段樹功能:update:成段替換 query:簡單hash
AC程式碼:
#include <iostream>
#include <stdio.h>
#include <string.h>
#include <algorithm>
using namespace std;
const int M=112345;
int L[M],R[M];
int S[M<<2];
bool Hash[M];
int col[M<<2];
int cnt;
void pushdown(int root)
{
if(col[root]!=-1)
{
col[root<<1]=col[root<<1|1]=col[root];
col[root]=-1;
}
}
void updata(int b,int e,int c,int root,int l,int r)//b e為要查詢的區間範圍,l r為root的區間範圍
{
if(b<=l&&r<=e)
{
col[root]=c;
return;
}
pushdown(root);
int mid=(l+r)/2;
if(mid>=e)
updata(b,e,c,root<<1,l,mid);
else if(mid<b)
updata(b,e,c,root<<1|1,mid+1,r);
else
{
updata(b,mid,c,root<<1,l,mid);
updata(mid+1,e,c,root<<1|1,mid+1,r);
}
}
void query(int l,int r,int root)
{
if(col[root]!=-1)
{
if(!Hash[col[root]])cnt++;
Hash[col[root]]=true;
return;
}
if(l==r)return ;
int mid=(l+r)/2;
query(l,mid,root<<1);
query(mid+1,r,root<<1|1);
}
int get(int k,int n)//獲取離散化後的下標
{
return lower_bound(S,S+n,k)-S;
}
int main()
{
int t;
scanf("%d",&t);
while(t--)
{
int n;
scanf("%d",&n);
int k=0;
for(int i=1; i<=n; i++)
{
scanf("%d%d",&L[i],&R[i]);
S[k++]=L[i];
S[k++]=R[i];
}
sort(S,S+k);
// for(int i=0; i<k; i++)printf("%d ",S[i]);printf("\n");
int kk=1;
for(int i=1; i<k; i++)
{
if(S[i]!=S[i-1])
S[kk++]=S[i];
}
for(int i=kk-1; i>0; i--)
{
if(S[i]!=S[i-1]+1)S[kk++]=S[i-1]+1;
}
sort(S,S+kk);
//for(int i=0; i<kk; i++)printf("%d ",S[i]);printf("\n");
memset(col,-1,sizeof(col));
for(int i=1; i<=n; i++)
{
int l=get(L[i],kk);
int r=get(R[i],kk);
// printf("l=%d r=%d\n",l,r);
updata(l,r,i,1,0,kk);
}
cnt=0;
memset(Hash,false,sizeof(Hash));
query(0,kk,1);
printf("%d\n",cnt);
}
}
相關推薦
POJ 2528 Mayor's posters(線段樹+離散化)
Mayor's posters Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 60691 Accepted: 17565 Description The citizens of Byteto
POJ2528 Mayor's posters(線段樹+離散化)
Mayor’s posters Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 57835 Accepted: 16725 Description The ci
Mayor's posters(線段樹+離散化)
++ main splay d3d close tree pac pair lin 這道題最關鍵的點就在離散化吧。 假如有三張海報[1, 10] [10, 13][15, 20] 僅僅三個區間就得占用到20了。 但是離散化後就可以是[1, 2] [2, 3] [
POJ 2528 Mayor's posters (線段樹 離散化+區間更新+區間求值 )
href eof 求值 給定 一個點 一個 stream 問題 void 題目鏈接:http://poj.org/problem?id=2528 題意:塗色問題,給定n個要塗色的區間(每次用的顏色不一樣,顏色覆蓋性極強),問最後能看到多少種顏色。(貼海報問題轉換) 題解
Mayor's posters【線段樹+離散化】
題解:區間修改線段樹+離散化。cnt=unique(arr,arr+cnt)-arr 用於離散化時非常方便。程式碼:#include<stdio.h> #include<string.
poj 2528 Mayor's posters(樹狀陣列+離散化)
Mayor's posters Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 63793 Accepted: 18415 Description The citizens of Byteto
POJ2528——Mayor's posters (線段樹區間更新查詢+離散化)
The citizens of Bytetown, AB, could not stand that the candidates in the mayoral election campaign have been placing their electoral poste
HIHO #1079 : 離散化(線段樹+離散化)
#include<bits/stdc++.h> using namespace std; #define cl(a,b) memset(a,b,sizeof(a)) #define LL long long #define pb push_back #define gcd __gcd #defi
POJ 2528 Mayor's posters 線段樹(成段更新+離散化)
題意: 給出N個海報,每個海報有一個長度區間(a,b).按順序貼在牆上。 問最後可以看到幾張海報。 思路: 一想到的就是線段樹,對每個區間進行染色,最後查詢一共有多少種顏色。 第一次寫玩沒看資料大小。MLE了。。仔細一看,海報長度1QW。 然後寫了個離散化的,300MS+。
POJ 2528 Mayor's posters [ 離散化 + 線段樹 ]
題目連結: 題意概括: 依次貼上 n 張海報,每張海報會覆蓋一個區間。後貼上的海報會覆蓋前貼的海報,問最後可以看見幾張海報 這裡的區間不是拿兩端點來維護的,是直接按單位最小區間來編號。如 [3, 5] 區間是由編號為 3、4、5的區間組成的 資料範圍:
【線段樹 + 離散化 + 詳細註釋】北大 poj 2528 Mayor's posters
/* THE PROGRAM IS MADE BY PYY */ /*----------------------------------------------------------------------------// Copyright (c) 2011 panyanyany All rig
poj 2528 Mayor's posters(離散化+線段樹)
題意: 市長競選,每個市長都往牆上貼海報,海報之間彼此可以覆蓋,給出貼上順序和每個海報的起點和終點,問最後又多少海報時可見的。 剛接觸離散化,先寫一寫本人的理解: 如果原資料太大,建樹會超記憶體。因此可以先對這些數排序,然後將它們對映成排序後的序號。比如在該題中,[1,
POJ 2528 Mayor's posters (線段樹+離散化 成段替換)
題目大意: 就是在一段區間上貼海報, 後來的區間會把前面來的區間覆蓋掉, 為貼完海報後能看到幾張海報(只看到一部分也算看到) 大致思路: 就是區間替換更新, 標記一下當前區間的所有的海報是否一致, 用懶惰標記標記一下當前區間的類別 聽說資料比較水...布吉島嚴格的資料下這
POJ 2528 Mayor's posters 線段樹成段更新+離散化
題意:很多張海報貼在牆上 求可以看到幾張海報 看那兩張圖就行了 第一張俯檢視 思路:最多2W個不同的數 離散化一下 然後成段更新 a[rt] = i代表這個區間是第i張報紙 更新玩之後一次query cover[i]=1代表可以看到第i張報紙 #include <c
POJ 2528 Mayor's posters 區間染色問題
題目意思 每個案例一個數字n,接下來n行表示張貼海報的起點與終點,求有多少海報沒有被完全遮住,經典區間染色問題。本題資料量比較大,所以需要將起點和終點離散化處理。 Sample Input 1 5 1 4 2 6 8 10
poj 2528 Mayor's posters【區間離散化】
文章目錄題目連結: 題目連結: http://poj.org/problem?id=2528 題意:就是有 N 種海報,每種海報有個長度[L,R],後來的海報會覆蓋前面來的海報,問最後從最上面看,能看得到幾種海報 input: 3 3 5 6 4 5 6 8
2528 Mayor's posters(離散化+區間更新)
給你n個桌布,桌布之間高度相同,按照順序粘桌布,問能看見多少張桌布。 首先桌布的距離範圍太長,無法用陣列儲存,需要先離散化,然後進行區間更新,用陣列維護區間,每個區間陣列的值有(-1:這個區間沒桌布;0:這個區間有多個桌布;1~n:這個區間是第i個桌布)。程式碼如下: #
【POJ 2482】 Stars in Your Window(線段樹+離散化+掃描線)
d+ opera algorithm ans som lov ble word wait 【POJ 2482】 Stars in Your Window(線段樹+離散化+掃描線) Time Limit: 1000MS M
N - Picture POJ - 1177(矩形周長並 + 線段樹 + 離散化)
本程式碼採用的是橫向掃描一遍和縱向掃描一遍來得到最終的結果,核心部分就是求舉行周長並,當然也要對線段樹比較熟悉才行,畢竟矩形周長並,面積並,面積交都要用到線段樹來計算。說說求舉行周長並的過程吧,我們先計算橫向線段的長度,把所有座標的y軸座標按照升序排列,掃描線從y的最小值依次向上掃描,求出每
POJ 1151 Atlantis(線段樹離散化求面積並)(C++)
題目連結:http://poj.org/problem?id=1151 算是模板題,做這個之前要搞懂離散化。這裡,區間最好用[L,R),要不然有些區間無法計算得到。人比較懶,自己去琢磨,不寫註釋了QAQ。 #include <cstdio> #include <v