資料結構實驗4:C++實現迴圈佇列類
阿新 • • 發佈:2019-02-07
實驗4
4.1 實驗目的
熟練掌握佇列的順序儲存結構和鏈式儲存結構。
熟練掌握佇列的有關演算法設計,並在迴圈順序佇列和鏈佇列上實現。
根據具體給定的需求,合理設計並實現相關結構和演算法。
4.2 實驗要求
4.2.1 迴圈順序佇列的實驗要求
迴圈順序佇列結構和運算定義,演算法的實現以庫檔案方式實現,不得在測試主程式中直接實現;
實驗程式有較好可讀性,各運算和變數的命名直觀易懂,符合軟體工程要求;
程式有適當的註釋。
4.3 實驗任務
4.3.1 迴圈順序佇列實驗任務
編寫演算法實現下列問題的求解。
<1>初始化一個佇列。
<2>判斷是否隊空。
<3>判斷是否隊滿。
設佇列最大長度:MaxLen=100
第一組資料:入隊n個元素,判斷隊滿
第二組資料:用迴圈方式將1到99,99個元素入隊,判隊滿
<4>入隊
第一組資料:4,7,8,12,20,50
第二組資料:a,b,c,d,f,g
<5>出隊
<6>取隊頭元素
<7>求當前佇列中元素個數
<8>編寫演算法實現
①初始化空迴圈佇列;
②當鍵盤輸入奇數時,此奇數入隊;
③當鍵盤輸入偶數時,隊頭出隊;
④當鍵盤輸入0時,演算法退出;
⑤每當鍵盤輸入後,輸出當前佇列中的所有元素。
4.5 執行結果截圖及說明
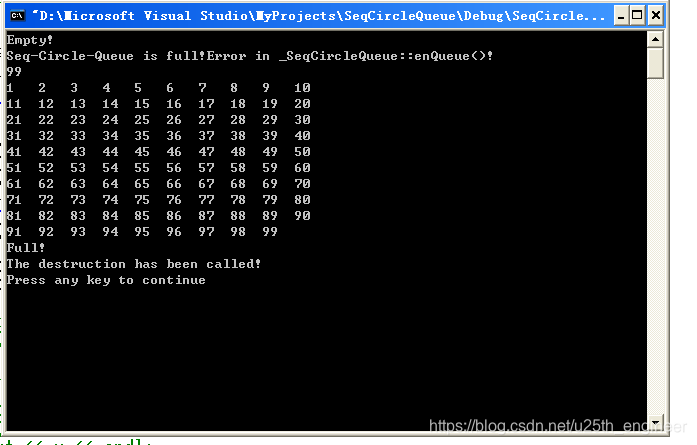
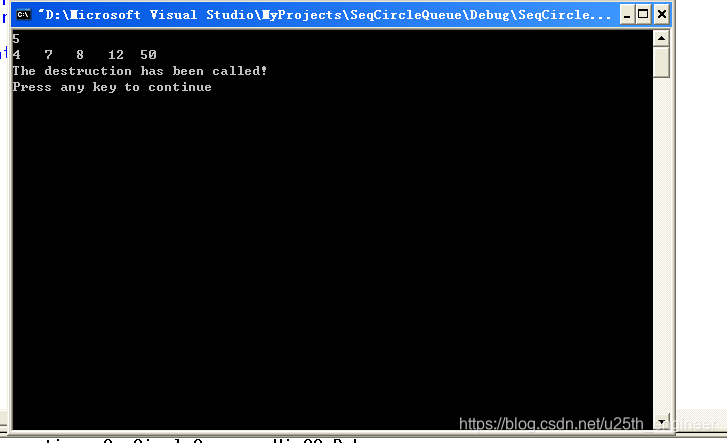
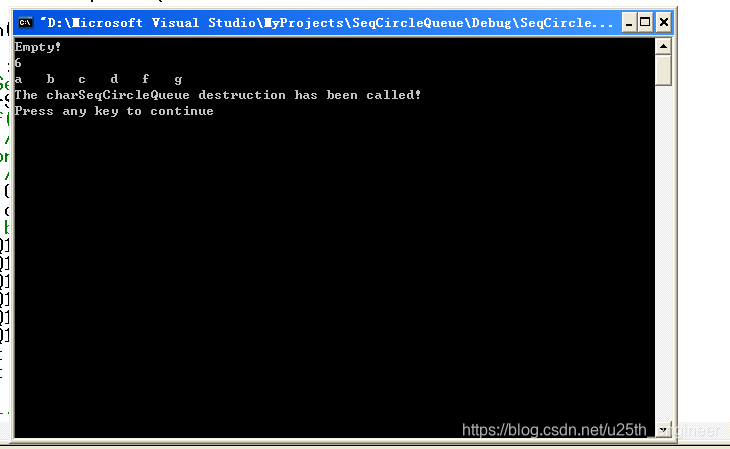
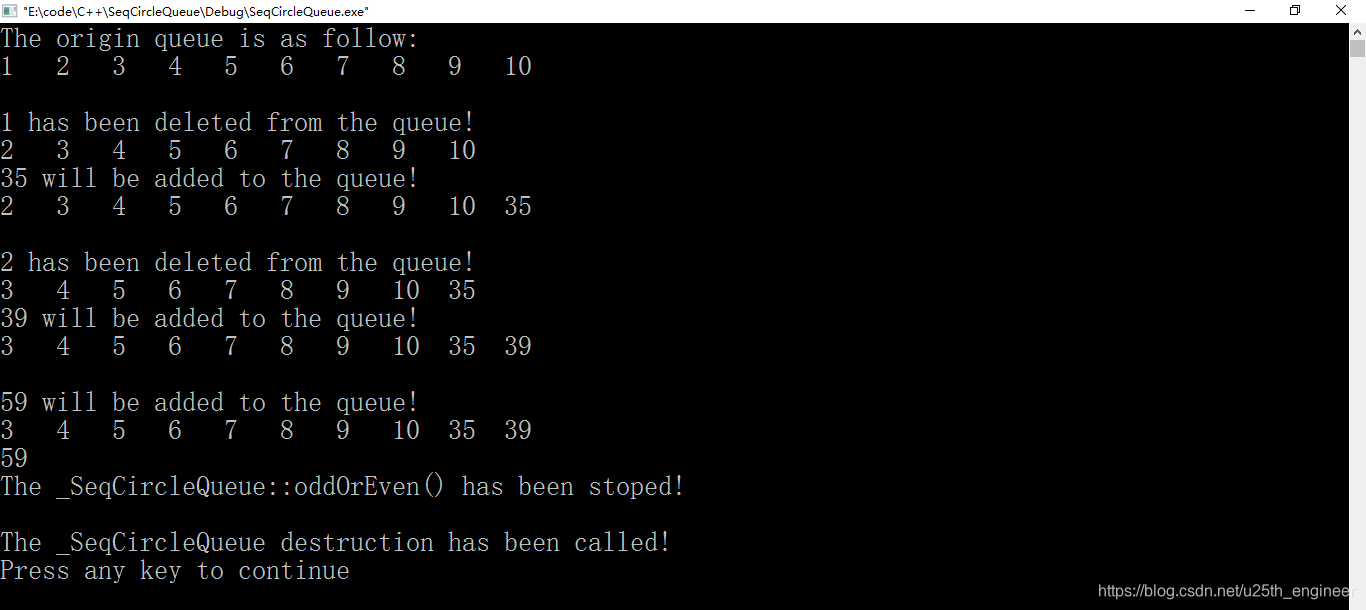
4.6 附原始碼
// stdafx.h : include file for standard system include files, // or project specific include files that are used frequently, but // are changed infrequently // #if !defined(AFX_STDAFX_H__8FA49CDF_FC99_4984_AB37_46921F7ED357__INCLUDED_) #define AFX_STDAFX_H__8FA49CDF_FC99_4984_AB37_46921F7ED357__INCLUDED_ #if _MSC_VER > 1000 #pragma once #endif // _MSC_VER > 1000 #include <stdc++.h> using namespace std; typedef int elementType; typedef char elementType1; const int maxn = 100; // TODO: reference additional headers your program requires here //{{AFX_INSERT_LOCATION}} // Microsoft Visual C++ will insert additional declarations immediately before the previous line. #endif // !defined(AFX_STDAFX_H__8FA49CDF_FC99_4984_AB37_46921F7ED357__INCLUDED_)
// _SeqCircleQueue.h: interface for the _SeqCircleQueue class.
//
//////////////////////////////////////////////////////////////////////
#if !defined(AFX__SEQCIRCLEQUEUE_H__FCBC0603_27E1_4352_833C_6BED9B418B96__INCLUDED_)
#define AFX__SEQCIRCLEQUEUE_H__FCBC0603_27E1_4352_833C_6BED9B418B96__INCLUDED_
#if _MSC_VER > 1000
#pragma once
#endif // _MSC_VER > 1000
class _SeqCircleQueue
{
public:
_SeqCircleQueue();
virtual ~_SeqCircleQueue();
bool emptySeqCircleQueue();
bool fullSeqCircleQueue();
bool enQueue( elementType value );
bool deQueue( elementType &value );
bool getFront( elementType &value );
int length();
void oddOrEven( elementType value );
friend ostream &operator<<( ostream &os, _SeqCircleQueue &scq )
{
if( ( scq._front - 1 ) % maxn == scq._rear )
return os;
int column = 0;
for( int i = scq._front; i % maxn != scq._rear; i = ( i + 1 ) % maxn )
{
os << setw(3) << setiosflags(ios::left) << scq.data[i] << " ";
column ++;
if( column % 10 == 0 )
os << endl;
}
os << endl;
}
private:
elementType data[maxn];
int _front;
int _rear;
};
#endif // !defined(AFX__SEQCIRCLEQUEUE_H__FCBC0603_27E1_4352_833C_6BED9B418B96__INCLUDED_)
// _SeqCircleQueue.cpp: implementation of the _SeqCircleQueue class.
//
//////////////////////////////////////////////////////////////////////
#include "stdafx.h"
#include "_SeqCircleQueue.h"
//////////////////////////////////////////////////////////////////////
// Construction/Destruction
//////////////////////////////////////////////////////////////////////
_SeqCircleQueue::_SeqCircleQueue()
{
_front = _rear = 0;
}
_SeqCircleQueue::~_SeqCircleQueue()
{
ios::sync_with_stdio(false);
cout << "The _SeqCircleQueue destruction has been called!" << endl;
}
bool _SeqCircleQueue::emptySeqCircleQueue()
{
return _front == _rear;
}
bool _SeqCircleQueue::fullSeqCircleQueue()
{
return ( _rear + 1 ) % maxn == _front;
}
bool _SeqCircleQueue::enQueue( elementType value )
{
if( fullSeqCircleQueue() )
{
cerr << "Seq-Circle-Queue is full!Error in _SeqCircleQueue::enQueue()!" << endl;
return false;
}
data[_rear] = value;
_rear = ( _rear + 1 ) % maxn;
return true;
}
bool _SeqCircleQueue::deQueue( elementType &value )
{
if( emptySeqCircleQueue() )
{
cerr << "Seq-Circle-Queue is empty!Error in _SeqCircleQueue::popFront()!" << endl;
return false;
}
value = data[_front];
_front = ( _front + 1 ) % maxn;
return true;
}
bool _SeqCircleQueue::getFront( elementType &value )
{
if( emptySeqCircleQueue() )
{
cerr << "Seq-Circle-Queue is empty!Error in _SeqCircleQueue::getFront()!" << endl;
return false;
}
value = data[_front];
return true;
}
int _SeqCircleQueue::length()
{
if( emptySeqCircleQueue() )
{
cerr << "Seq-Circle-Queue is empty!Error in _SeqCircleQueue::length()!" << endl;
return -1;
}
return ( _rear - _front + maxn ) % maxn;
}
void _SeqCircleQueue::oddOrEven( elementType value )
{
if( value & 1 )
{
enQueue(value);
cout << value << " will be added to the queue!" << endl;
cout << (*this);
}
else if( !( value & 1) && value != 0 )
{
elementType x;
deQueue(x);
cout << x << " has been deleted from the queue!" << endl;
cout << (*this);
}
else //if( value == 0 )
{
cout << "The _SeqCircleQueue::oddOrEven() has been stoped!" << endl;
return;
}
}
// charSeqCircleQueue.h: interface for the charSeqCircleQueue class.
//
//////////////////////////////////////////////////////////////////////
#if !defined(AFX_CHARSEQCIRCLEQUEUE_H__FBB4F8DD_2EF9_43A6_8E23_FD7E4C56908E__INCLUDED_)
#define AFX_CHARSEQCIRCLEQUEUE_H__FBB4F8DD_2EF9_43A6_8E23_FD7E4C56908E__INCLUDED_
#if _MSC_VER > 1000
#pragma once
#endif // _MSC_VER > 1000
class charSeqCircleQueue
{
public:
charSeqCircleQueue();
virtual ~charSeqCircleQueue();
bool emptyCharSeqCircleQueue();
bool fullCharSeqCircleQueue();
bool enQueue( elementType1 value );
bool deQueue( elementType1 &value );
bool getFront( elementType1 &value );
int length();
friend ostream &operator<<( ostream &os, charSeqCircleQueue &cscq )
{
if( ( cscq._front - 1 ) % maxn == cscq._rear )
return os;
int column = 0;
for( int i = cscq._front; i % maxn != cscq._rear; i = ( i + 1 ) % maxn )
{
os << setw(3) << setiosflags(ios::left) << cscq.data[i] << " ";
column ++;
if( column % 10 == 0 )
os << endl;
}
os << endl;
}
private:
elementType1 data[maxn];
int _front;
int _rear;
};
#endif // !defined(AFX_CHARSEQCIRCLEQUEUE_H__FBB4F8DD_2EF9_43A6_8E23_FD7E4C56908E__INCLUDED_)
// charSeqCircleQueue.cpp: implementation of the charSeqCircleQueue class.
//
//////////////////////////////////////////////////////////////////////
#include "stdafx.h"
#include "charSeqCircleQueue.h"
//////////////////////////////////////////////////////////////////////
// Construction/Destruction
//////////////////////////////////////////////////////////////////////
charSeqCircleQueue::charSeqCircleQueue()
{
_front = _rear = 0;
}
charSeqCircleQueue::~charSeqCircleQueue()
{
ios::sync_with_stdio(false);
cout << "The charSeqCircleQueue destruction has been called!" << endl;
}
bool charSeqCircleQueue::emptyCharSeqCircleQueue()
{
return _front == _rear;
}
bool charSeqCircleQueue::fullCharSeqCircleQueue()
{
return ( _rear + 1 ) % maxn == _front;
}
bool charSeqCircleQueue::enQueue( elementType1 value )
{
if( fullCharSeqCircleQueue() )
{
cerr << "Seq-Circle-Queue is full!Error in charSeqCircleQueue::::enQueue()!" << endl;
return false;
}
data[_rear] = value;
_rear = ( _rear + 1 ) % maxn;
return true;
}
bool charSeqCircleQueue::deQueue( elementType1 &value )
{
if( emptyCharSeqCircleQueue() )
{
cerr << "Seq-Circle-Queue is empty!Error in charSeqCircleQueue::popFront()!" << endl;
return false;
}
value = data[_front];
_front = ( _front + 1 ) % maxn;
return true;
}
bool charSeqCircleQueue::getFront( elementType1 &value )
{
if( emptyCharSeqCircleQueue() )
{
cerr << "Seq-Circle-Queue is empty!Error in charSeqCircleQueue::::getFront()!" << endl;
return false;
}
value = data[_front];
return true;
}
int charSeqCircleQueue::length()
{
if( emptyCharSeqCircleQueue() )
{
cerr << "Seq-Circle-Queue is empty!Error in charSeqCircleQueue::::length()!" << endl;
return -1;
}
return ( _rear - _front + maxn ) % maxn;
}
// SeqCircleQueue.cpp : Defines the entry point for the console application.
//
#include "stdafx.h"
#include "_SeqCircleQueue.h"
#include "charSeqCircleQueue.h"
//#include <windows.h>
int main(int argc, char* argv[])
{
ios::sync_with_stdio(false);
srand( time(NULL) );
_SeqCircleQueue SCQ1;
//charSeqCircleQueue CSCQ1;
//if( SCQ1.emptySeqCircleQueue() )
//cout << "Empty!" << endl;
for( int i = 1; i <= 10; i ++ )
SCQ1.enQueue(i);
cout << "The origin queue is as follow:" << endl << SCQ1;
for( int j = 1; j <= 5; j ++ )
{
elementType value = rand() % 100 + 2;
SCQ1.oddOrEven(value);
}
SCQ1.oddOrEven(0);
/*
if( CSCQ1.emptyCharSeqCircleQueue() )
cout << "Empty!" << endl;
//a,b,c,d,f,g
CSCQ1.enQueue('a');
CSCQ1.enQueue('b');
CSCQ1.enQueue('c');
CSCQ1.enQueue('d');
CSCQ1.enQueue('f');
CSCQ1.enQueue('g');
cout << CSCQ1.length() << endl;
cout << CSCQ1;
SCQ1.enQueue(4);//4,7,8,12,20,50
SCQ1.enQueue(7);
SCQ1.enQueue(8);
SCQ1.enQueue(12);
SCQ1.enQueue(50);
cout << SCQ1.length() << endl;
cout << SCQ1;
elementType x;
if( SCQ1.deQueue(x) )
{
cout << x << endl;
}
cout << SCQ1;
if( SCQ1.getFront(x) )
cout << x << endl;
cout << SCQ1.length() << endl;
if( SCQ1.fullSeqCircleQueue() )
cout << "Full!" << endl;
*/
cin.get();
//Sleep( 1000 * 120 );
return 0;
}