《資料結構》實驗三: 棧和佇列實驗
阿新 • • 發佈:2019-02-09
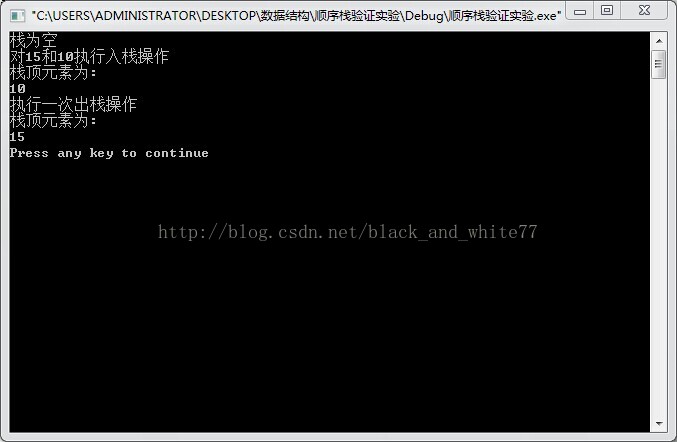
一..實驗目的
鞏固棧和佇列資料結構,學會運用棧和佇列。
1.回顧棧和佇列的邏輯結構和受限操作特點,棧和佇列的物理儲存結構和常見操作。
2.學習運用棧和佇列的知識來解決實際問題。
3.進一步鞏固程式除錯方法。
4.進一步鞏固模板程式設計。
三..實驗內容
1.自己選擇順序或鏈式儲存結構,定義一個空棧類,並定義入棧、出棧、取棧元素基本操作。然後在主程式中對給定的N個數據進行驗證,輸出各個操作結果。
2.自己選擇順序或鏈式儲存結構,定義一個空棧佇列,並定義入棧、出棧、取棧元素基本操作。然後在主程式中對給定的N個數據進行驗證,輸出各個操作結果。
3.程式設計實現一個十進位制數轉換成二進位制數。要求,要主程式中輸出一個10進度數,輸出其對應的2進位制數序列。
前兩題是必做題,第3題是選做題。
三..實驗內容
1.自己選擇順序或鏈式儲存結構,定義一個空棧類,並定義入棧、出棧、取棧元素基本操作。然後在主程式中對給定的N個數據進行驗證,輸出各個操作結果。
#ifndef SeqStack_H #define SeqStack_H const int StackSize=10; template<class DataType> class SeqStack { public: SeqStack(); ~SeqStack(){} void Push(DataType x); DataType Pop(); DataType GetTop(); int Empty(); private: DataType data[StackSize]; int top; }; #endif #include"SeqStack.h" template<class DataType> SeqStack<DataType>::SeqStack() { top=-1; } template<class DataType> void SeqStack<DataType>::Push(DataType x) { if(top==StackSize-1)throw"上溢"; top++; data[top]=x; } template<class DataType> DataType SeqStack<DataType>::Pop() { DataType x; if (top==-1)throw""; x=data[top--]; return x; } template<class DataType> DataType SeqStack<DataType>::GetTop() { if(top!=-1) return data[top]; } template<class DataType> int SeqStack<DataType>::Empty() { if(top==-1)return 1; else return 0; } #include<iostream> using namespace std; #include"SeqStack.cpp" void main() { SeqStack<int>S; if(S.Empty()) cout<<"棧為空"<<endl; else cout<<"棧非空"<<endl; cout<<"對15和10執行入棧操作"<<endl; S.Push(15); S.Push(10); cout<<"棧頂元素為:"<<endl; cout<<S.GetTop()<<endl; cout<<"執行一次出棧操作"<<endl; S.Pop(); cout<<"棧頂元素為:"<<endl; cout<<S.GetTop()<<endl; }
2.自己選擇順序或鏈式儲存結構,定義一個空棧佇列,並定義入棧、出棧、取棧元素基本操作。然後在主程式中對給定的N個數據進行驗證,輸出各個操作結果。
#ifndef LinkQueue_H #define LinkQueue_H template<class DataType> struct Node { DataType data; Node<DataType>*next; }; template<class DataType> class LinkQueue { public: LinkQueue(); ~LinkQueue(); void EnQueue(DataType x); DataType DeQueue(); DataType GetQueue(); int Empty(); private: Node<DataType>*front,*rear; }; #endif; #include"LinkQueue.h" template<class DataType> LinkQueue<DataType>::LinkQueue() { Node<DataType>*s=NULL; s=new Node<DataType>; s->next=NULL; front=rear=s; } template<class DataType> LinkQueue<DataType>::~LinkQueue() { Node<DataType>*p=NULL; while(front!=NULL) { p=front->next; delete front; front=p; } } template<class DataType> void LinkQueue<DataType>::EnQueue(DataType x) { Node<DataType>*s=NULL; s=new Node<DataType>; s->data=x; s->next=NULL; rear->next = s;rear=s; } template<class DataType> DataType LinkQueue<DataType>::DeQueue() { Node<DataType>*p=NULL; int x; if(rear==front) throw"下溢"; p=front->next; x=p->data; front->next=p->next; if(p->next==NULL)rear=front; delete p; return x; } template <class DataType> DataType LinkQueue<DataType>::GetQueue() { if(front != rear) return front ->next->data; } template<class DataType> int LinkQueue<DataType>::Empty() { if(front==rear) return 1; else return 0; } #include<iostream> using namespace std; #include"LinkQueue.cpp" void main() { LinkQueue<int>Q; if(Q.Empty()) cout<<"佇列為空"<<endl; else cout<<"佇列為空"<<endl; cout<<"元素25和75執行入隊操作:"<<endl; try { Q.EnQueue(25); Q.EnQueue(75); } catch(char*wrong) { cout<<wrong<<endl; } cout<<"檢視隊頭元素:"<<endl; cout<<Q.GetQueue()<<endl; cout<<"執行出隊操作:"<<endl; try { Q.DeQueue(); } catch(char*wrong) { cout<<wrong<<endl; } cout<<"檢視隊頭元素:"<<endl; cout<<Q.GetQueue()<<endl; }