C#學習筆記(十六):索引器和重載運算符
阿新 • • 發佈:2019-02-11
instance cit png form mage 創建 return position args
二維數組如何映射到一維數組
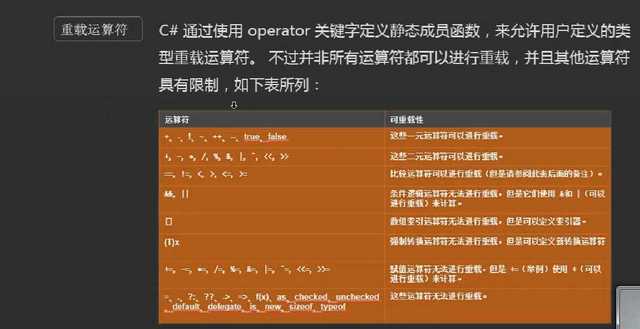
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace m1w4d2_indexes { #region 索引器-李索 //索引器可以讓我們通過不同的索引號返回對應類型的多個屬性 //索引器適用於除自動屬性以外的所有屬性特性 //索引號可以是任意類型//索引器在通過類對象來訪問和賦值 變量(類對象)[索引號] //訪問修飾 返回類型 this[索引號,索引號,索引號,索引號......] //{ get {return} set {value} } //用索引器訪問一個怪物類中的不同字段 class Monster { public Monster(int attack, int defend, int health, int speed, string name) { this.attack = attack; this.defend = defend;this.health = health; this.speed = speed; this.name = name; } int attack; int defend; int health; int speed; string name; //public string this[int indexa, int indexb] //{ // get { return ""; } // set { }//} public string this[int index] { get { string str = ""; switch (index) { case 0: str = attack.ToString(); break; case 1: str = defend.ToString(); break; case 2: str = health.ToString(); break; case 3: str = speed.ToString(); break; case 4: str = name; break; } return str; } set { switch (index) { case 0: attack = int.Parse(value); break; case 1: defend = int.Parse(value); break; case 2: health = int.Parse(value); break; case 3: speed = int.Parse(value); break; case 4: name = value; break; } } } } #endregion #region 多維度的索引號 //索引號可以任意維度 //用一個MyArray類去模擬一個二維數組 class MyArray { public MyArray(int x, int y) { //這個放元素的一維數組多長 //array = new int[10]; array = new int[x * y];//一維數組的長度 Length = new int[2]; Length[0] = x; Length[1] = y; } int[] Length; int[] array; public int GetLength(int index) { if (index > Length.Length) { throw new IndexOutOfRangeException();//拋異常 } return Length[index]; } public int this[int x, int y] { get { //我給你一個二維的下標,你如何映射到一維數組上 return array[x + y * GetLength(0)]; } set { array[x + y * GetLength(0)] = value; } } } #endregion #region 索引器-沈軍 class Unity1803 { // C# 單例模式 (單獨的實例) private static Unity1803 instance = null; public static Unity1803 Instance { get { if (instance == null) instance = new Unity1803(); return instance; } } //靜態構造函數什麽時候調用 //當我們訪問靜態成員的時候,先調用且只調用一次 //當我們創建對象的時候,先調用且只調用一次 //可以對靜態字段做初始化使用的,靜態的成員才會加載到內存中 //static Unity1803()//靜態構造函數,不能有訪問修飾符和參數 //{ // Console.WriteLine("調用靜態構造函數"); //} public int Count { private set; get; } // Student[] students = new Student[37]; // null private Unity1803() { students[0] = new Student("蔡浩", 18, 90); Count++; students[1] = new Student("江燦榮", 20, 50); Count++; students[2] = new Student("賀益民", 18, 70); Count++; } public Student this[int index] { get { return students[index]; } set { if (students[index] == null) Count++; students[index] = value; } } public Student this[string name] { get { for (int i = 0; i < Count; i++) { if (students[i].Name == name) { return students[i]; } } return null; } set { students[Count] = new Student(name, 20, 80); } } } class Student { public string Name { get; set; } public int Age { get; set; } public int CSharpScore { get; set; } public Student(string name, int age, int score) { this.Name = name; this.Age = age; this.CSharpScore = score; } public override string ToString() { return "Name:" + Name + ", Age :" + Age + ", Score :" + CSharpScore; } public static bool operator >(Student lfs, int score) { return lfs.CSharpScore > score; } public static bool operator <(Student lfs, int score) { return lfs.CSharpScore < score; } public static bool operator true(Student s) { return s.CSharpScore >= 60; } public static bool operator false(Student s) { return s.CSharpScore < 60; } // 隱式類型轉換 把字符串隱式的轉換成Student類型 public static implicit operator Student(string name) { Student s = new Student(name, 18, 75); return s; } // 顯式類型轉換 把Student類型強轉成字符串 public static explicit operator string(Student s) { return s.Name; } } #endregion class Program { static void Main(string[] args) { #region 索引器-李索 Monster monster = new Monster(10, 5, 100, 5, "哥布林"); for (int i = 0; i < 5; i++) { Console.WriteLine(monster[i]); } Console.WriteLine(); //把一個策劃文檔的數據切割成對應的字符數組 //序列化 string dataTitle = "attack,defend,health,speed,name"; string data = "100,15,500,1,蠕蟲皇後"; string[] datas = data.Split(‘,‘); for (int i = 0; i < 5; i++) { monster[i] = datas[i]; } for (int i = 0; i < 5; i++) { Console.WriteLine(monster[i]); } #endregion #region 多維度的索引號 //索引號可以任意維度 //用一個MyArray類去模擬一個二維數組 MyArray myArray = new MyArray(5, 7); for (int x = 0; x < myArray.GetLength(0); x++) { for (int y = 0; y < myArray.GetLength(1); y++) { myArray[x, y] = x * 100 + y; } } for (int y = 0; y < myArray.GetLength(1); y++) { for (int x = 0; x < myArray.GetLength(0); x++) { Console.Write(myArray[x, y] + "\t"); } Console.WriteLine(); } #endregion #region 索引器-沈軍 // 屬性 操作字段 // 索引器 對於操作集合的一種封裝 //Unity1803 unity1803 = new Unity1803(); //unity1803[2] = new Student("沈天宇", 20, 95); for (int i = 0; i < Unity1803.Instance.Count; i++) { if (Unity1803.Instance[i]) { Console.WriteLine(Unity1803.Instance[i]); // .ToString()方法 } } Unity1803.Instance[Unity1803.Instance.Count] = "丁超"; // 隱式類型轉換 string name = (string)Unity1803.Instance[0]; // 顯式類型轉換 //Console.WriteLine(unity1803["沈天宇"]); //Unity1803 _unity1803 = new Unity1803(); //Console.WriteLine(Unity1803.Count); //Unity1803.instance = new Unity1803(); // 只讀的 //Unity1803 u1 = new Unity1803(); // 不允許的 //Console.WriteLine(Unity1803.Instance[0]); // 隱式轉換 顯式轉換 int num1 = 100; byte num2 = 200; num1 = num2; // 隱式類型轉換 num2 = (byte)num1; // 顯式類型轉換 #endregion } } }
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace m1w4d2_operator { #region 練習1 //通過重載運算符,我們可以給自定類型,增加計算功能 struct Point2D { public int x, y; public Point2D(int x, int y) { this.x = x; this.y = y; } public static Point2D Up = new Point2D(0, -1); public static Point2D Down = new Point2D(0, 1); public static Point2D Left = new Point2D(-1, 0); public static Point2D Right = new Point2D(1, 0); //返回類型?,函數名(operator 運算符)?參數? //public static i = i + 1; //兩個參數,任意類型 //返回類型,任意類型 //算數運算符 是雙目運算符,參數必須是兩個,任意類型,返回類型,任意類型 public static Point2D operator +(Point2D a, Point2D b) { return new Point2D(a.x + b.x, a.y + b.y); } public static Point2D operator -(Point2D a, Point2D b) { return new Point2D(a.x - b.x, a.y - b.y); } //關系運算符 是雙目運算符,參數必須是兩個,任意類型,返回類型是bool public static bool operator ==(Point2D a, Point2D b) { return a.x == b.x && a.y == b.y; } public static bool operator !=(Point2D a, Point2D b) { return !(a == b); } public override string ToString() { return string.Format("[x:{0},y{1}]", x, y); } } #endregion #region 練習2 class Item { public static string style = "劍刀盾斧藥"; public Item(string name, int value) { this.name = name; this.value = value; } string name; int value; public override string ToString() { return string.Format("{0}:value:{1}", name, value); } } class Monster { public static Random roll = new Random(); public int attack; public string name; public int speed; public Monster(int attack, int speed, string name) { this.attack = attack; this.name = name; this.speed = speed; } public static Item operator +(Monster a, Monster b) { string name = a.name.Substring(a.name.Length / 2) + b.name.Substring(b.name.Length / 2) + Item.style[roll.Next(0, Item.style.Length)]; int value = (a.attack + b.attack) * 10 + a.speed + b.speed; if (name[name.Length - 1] == ‘屎‘) { value /= 10000; } return new Item(name, value); } public override string ToString() { return string.Format("{0}:attack:{1},speed:{2}", name, attack, speed); } } #endregion class Program { static void Main(string[] args) { #region 練習1 //老位置 Point2D oldPosition = new Point2D(5, 5); //position.x += dir.x; //新位置 是老位置 向上走一步 Console.WriteLine(oldPosition); Point2D newPosition = oldPosition + Point2D.Up; Console.WriteLine(newPosition); //請問,新位置在老位置的哪個方向 string dirStr = ""; Point2D pointDir = newPosition - oldPosition; if (pointDir == Point2D.Up) dirStr = "上"; else if (pointDir == Point2D.Down) dirStr = "下"; else if (pointDir == Point2D.Left) dirStr = "左"; else if (pointDir == Point2D.Right) dirStr = "右"; Console.WriteLine("你的前進方向是{0}", dirStr); #endregion #region 練習2 Monster a = new Monster(1000, 50, "哥布林"); Monster b = new Monster(1000, 200, "索尼克"); Console.WriteLine(a); Console.WriteLine(b); Item item = a + b; Console.WriteLine(item); #endregion } } }
C#學習筆記(十六):索引器和重載運算符