Unity3D學習筆記(二):個體層次、絕對和局部坐標、V3平移旋轉
阿新 • • 發佈:2019-02-11
esp 返回 ESS coo his object spa cti dcom Directional Light:平行光源/方向性光源,用來模擬太陽光(角度只與旋轉角度有關,與位置無關)
Point Light:點光源,用來模擬燈泡,向四周發散光源
Spotlight:錐光源/聚光燈,用來模擬手電筒,燈塔等光源,向一個方向擴散性發散光源
Area Light:區域光,用於烘焙光照貼圖,上面這三種光源可以用於實時渲染,也可以用於烘焙光照貼圖
通過代碼控制光源旋轉、顏色、強度,可以做出24h光線變幻,日月輪轉
----Intensity光線強度,默認1,關閉0
----Shadow Type陰影類型,柔性陰影更耗資源
----Intensity Multiplier反射光
----Spot Angle錐光源的角度
燈光控制
LightType是枚舉類型
註意:當形成父子物體關系後,在檢視面板中(在代碼中不同)子物體的Transform下的屬性是相對於父物體的值。比如position就是相對於父物體坐標為坐標原點的相對坐標。
當遊戲物體沒有父物體,世界就是父物體,這個時候坐標就是相對於世界的坐標位置。
在C#代碼中所有物體都是世界坐標。
兩個遊戲物體的關聯,本質上是把兩個兩個遊戲物體Transform的關聯在一起。
建立父子關系
API:Application Programer Interface //應用程序編程接口,拿到Unity來說,有一些需要用到的功能,unity已經幫你寫好了,直接拿來用就可以得到想要的結果,不需要自己重新寫,而且也不必須知道到底是怎麽實現的。
查找遊戲物體:
GameObject.Find(string name);//通過遊戲物體的名字來查找遊戲物體的引用。但是,正常開發過程中,尤其是在頻繁的查找過程中,不建議使用Find。
添加路徑路點,“/”代表搜索路徑,加上路徑可以減少搜索次數
implicit:隱式轉換
operator重載:if(exists != null) 可以簡寫為 if(exists)
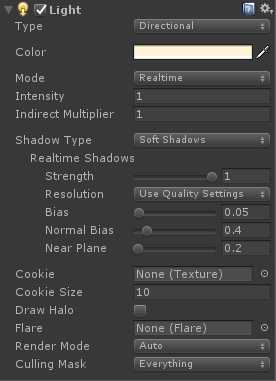
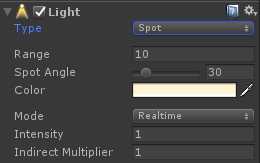
using System.Collections; using System.Collections.Generic; using UnityEngine; public class MyLight : MonoBehaviour { // Use this for initialization void Start() { Light light = GetComponent<Light>(); light.intensity = 1; //int[] array = new int[] { 1, 2, 3, 4, 5, 6 };父子物體: 在層級視圖中,將一個遊戲物體拖拽到另一個遊戲物體下面,就變成了父子物體關系。 特點:子物體動,父物體不動;父物體動,子物體跟隨移動。 作用:拼接遊戲物體。 Center:父子物體的幾何中心 Pivot:選中物體的幾何中心if (light != null) { Debug.Log(light.intensity); } } // Update is called once per frame void Update() { } }

usingGetComponent推薦使用泛型的重載 1、用代碼獲取組件 T:GetComponent<T>()//如果獲取Transform,就可以直接寫Transform,是Component類中的一個只讀的成員屬性,代表著當前這個組件對象所掛載的遊戲物體身上的Transform組件的引用。System.Collections; using System.Collections.Generic; using UnityEngine; public class Find_Parent : MonoBehaviour { public GameObject sphere; // Use this for initialization void Start() { //如何在整個場景中查找遊戲物體 //查找寫成靜態方法,是工具,不是某一個對象的方法 sphere = GameObject.Find("Find_Parent/Sphere"); Debug.Log(sphere.name); //建立父子關系,需要關聯transform gameObject.transform.parent = sphere.transform; } // Update is called once per frame void Update() { //不要在Update進行查找,GameObject.Find("Sphere"); //要建一個全局的sphere初始化 //移動父物體,子物體也會動 if (sphere) { sphere.transform.Translate(new Vector3(0, 0, 0.1f)); // //或者 // sphere.transform.Translate(Vector3.forward * 0.1f); } } }
using System.Collections; using System.Collections.Generic; using UnityEngine; public class GetComponent : MonoBehaviour { // Use this for initialization void Start () { Transform trans = GetComponent<Transform>(); //GetComponent獲取組件的方法,加()表執行 //GetComponent獲取不到組件,會返回空引用變量(空指針),在使用的時候,會報空引用異常的錯誤 Transform trans = null; Debug.Log(trans.position); if (trans != null) { Debug.Log(trans.position); } //transform transform.position = new Vector3(1, 2, 3);//不能只修改transform.position某個分量的值,需要對transform.position整體重新賦值,才會有返回值 Debug.Log(transform.position); } // Update is called once per frame void Update () { } }2、用代碼動態添加組件 gameObject可以直接在繼承自component的類中使用,代表著當前組件所掛載的遊戲物體的引用。 T:gameObject.AddComponent<T>() 當完成添加組件的任務之後,會返回這個組件的引用。
using System.Collections; using System.Collections.Generic; using UnityEngine; public class AddComponent : MonoBehaviour { // Use this for initialization void Start () { Light light = gameObject.AddComponent<Light>(); light.intensity = 1; light.type = LightType.Spot; light.color = Color.green; Destroy(light, 5.0f); } // Update is called once per frame void Update () { } }3、用代碼動態移除組件 Destroy(Object obj);
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Destroy : MonoBehaviour { // Use this for initialization void Start () { AudioSource audio = GetComponent<AudioSource>(); if (audio)//audio是傳進來的引用 { Destroy(audio); //5秒後銷毀audio引用 Destroy(audio, 5); //5秒後銷毀Lesson3腳本組件 Destroy(this, 5); } } // Update is called once per frame void Update () { } }平移:transform.Translate(Vector3 translation); transform.Translate (vector.forward) :移動的是自身坐標系的方向 transform.position += vector.forward:移動世界坐標系的方向 向量 * 標量,控制速度
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Move : MonoBehaviour { public float moveSpeed = 0.5f; // Use this for initialization void Start() { // transform.position = new Vector3(3.0f, 3.0f, 3.0f);//不要放在循環裏用,調用一次就夠了 } // Update is called once per frame void Update() { if (transform.position.z < 10.0f) { transform.Translate(Vector3.forward * moveSpeed * Time.deltaTime); } else { transform.position = new Vector3(3.0f, 3.0f, 3.0f); } //每幀z軸都移動0.1f,當前坐標加上新坐標,再返回給原坐標 transform.position += new Vector3(0, 0, 0.1f); //Translate平移 transform.Translate(new Vector3(0, 0, 0.1f)); //自定義myTranslate myTranslate(new Vector3(0, 0, 0.1f)); } void myTranslate(Vector3 translation) { transform.position += new Vector3(0, 0, 0.1f); } }平移控制
using System.Collections; using System.Collections.Generic; using UnityEngine; public class MoveControl : MonoBehaviour { private float moveSpeed = 2; // Use this for initialization void Start() { } // Update is called once per frame void Update() { if (Input.GetKey(KeyCode.W)) { this.transform.Translate(Vector3.forward * Time.deltaTime * moveSpeed); } if (Input.GetKey(KeyCode.S)) { this.transform.Translate(Vector3.back * Time.deltaTime * moveSpeed); } if (Input.GetKey(KeyCode.A)) { this.transform.Translate(Vector3.left * Time.deltaTime * moveSpeed); } if (Input.GetKey(KeyCode.D)) { this.transform.Translate(Vector3.right * Time.deltaTime * moveSpeed); } } }旋轉:transform.Rotate(Vector3 eulerAngles); transform.Rotate (vector.up) :繞著自身坐標系的方向旋轉 transform.eulerAngles += vector.up:繞著世界坐標系的方向旋轉 transform.eulerAngles = new Vector3(90,0,0);
using System.Collections; using System.Collections.Generic; using UnityEngine; public class position_eulerAngles : MonoBehaviour { // Use this for initialization void Start () { } // Update is called once per frame void Update () { transform.position += Vector3.back * 0.05f; transform.eulerAngles += Vector3.up; } }
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Rotate : MonoBehaviour { // Use this for initialization void Start() { } // Update is called once per frame void Update() { //旋轉 //歐拉角 transform.eulerAngles += new Vector3(0, 0, 0.1f); transform.eulerAngles += Vector3.up; //旋轉的API是Rotate transform.Rotate(Vector3.up); } }為什麽要繼承MonoBehaviour MonoBehaviour繼承於Behaviour
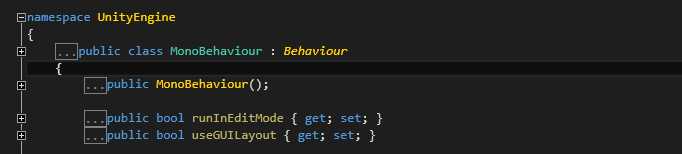
Behaviour繼承於Component,Component是所有組件的基類,enabled:組件是否激活
Object是Component的基類,組件的本質是Object類
GetComponent獲取不到組件,會返回空引用變量(空指針),在使用的時候,會報空引用異常的錯誤
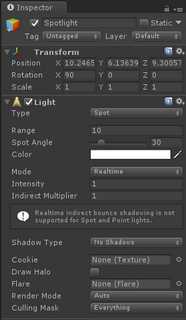
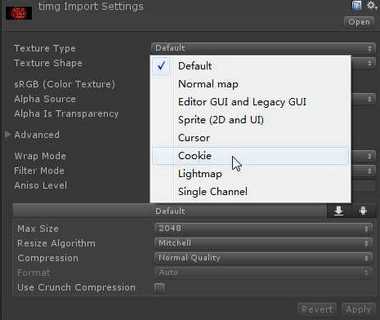
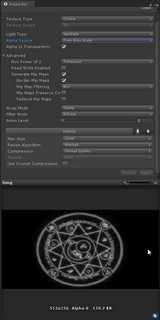
點光源,立方體貼圖(六個面可以不一樣),cookie
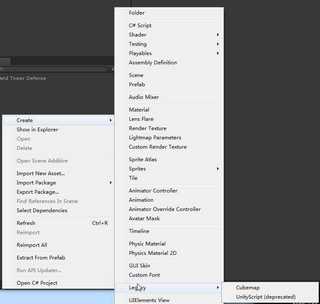
Halo光暈
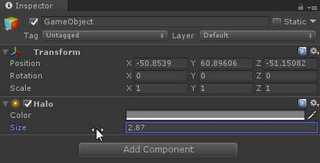
Layer:給遊戲物體添加層
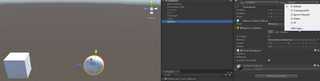
Culing Mask遮罩剔除:光源對那些層(遊戲物體)起作用

子物體從父物體拖出,會疊加上父物體的Transform,Position和Rotation做加法,Scale做乘法

F12進入定義,a是alpha通道
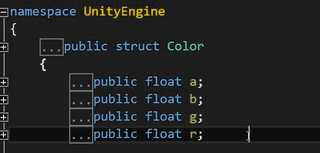
拖拽遊戲物體到腳本組件,可以自動賦值
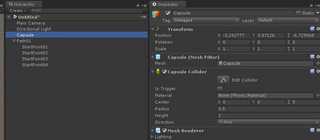
Unity3D學習筆記(二):個體層次、絕對和局部坐標、V3平移旋轉