jQuery操作標簽--樣式、文本、屬性操作, 文檔處理
阿新 • • 發佈:2019-02-17
我們 lan UNC 進行 登錄 盒子模型 hide label rem
操作標簽
一、樣式操作
樣式類:
addClass(); // 添加指定的css類名 removeClass(); //移除指定的css類名 hasClass(); //判斷樣式是否存在 toggleClass(); //切換css類名,如果有就移除,如果沒有就添加
通過jQuery對象修改css樣式:
$("tagname").css({"color","red"}) //DOM操作:tag.style.color="red"
樣式操作示例1:
$("p").css("color", "red"); //將所有p標簽的字體設置為紅色
二、位置操作
offset()// 獲取匹配元素在當前窗口的相對偏移或設置元素位置 position()// 獲取匹配元素相對父元素的偏移,不能設置位置 $(window).scrollTop() //滾輪向下移動的距離 $(window).scrollLeft() //滾輪向左移動的距離
.offset()方法允許我們檢索一個元素相對於文檔(document)的當前位置。
.position()獲取相對於它最近的具有相對位置(position:relative或position:absolute)的父級元素的距離,如果找不到這樣的元素,則返回相對於瀏覽器的距離。
尺寸:
height() // 盒子模型content的大小, 就是我們設置的標簽的高度和寬度 width() innerHeight() // 內容content高度 + 兩個padding的高度 innerWidth() outerHeight() //內容高度+兩個padding的高度+兩個border的高度,不包括margin的高度,因為margin不是標簽的,是標簽和標簽之間的距離 outerWidth()
返回頂部示例代碼:
<button id="b2" class="btn btn-default c2 hide">返回頂部</button> <script src="jquery-3.2.1.min.js"></script> <script> //$("#b1").on("click", function () { // $(".c1").offset({left: 200, top:200}); //}); // $(window),window對象是還記得嗎?是不是全局的一個對象啊,整個頁面窗口對象,通過$符號包裹起來就變成了一個jQuery對象了 $(window).scroll(function () { if ($(window).scrollTop() > 100) { //當滾動條相對頂部位置的滾動距離大於100的時候才顯示那個返回頂部的按鈕,這個100你可以理解為窗口和整個頁面文檔的距離,鼠標向下滑動的距離 $("#b2").removeClass("hide"); }else { $("#b2").addClass("hide"); } }); $("#b2").on("click", function () { //jQuery綁定事件第二天我們在學,先作為了解 $(window).scrollTop(0); }) </script>
三、文本操作
HTML代碼:
html() // 取得第一個匹配元素的html內容,包含標簽內容 html(val) // 設置所有匹配元素的html內容,識別標簽,能夠表現出標簽的效果
文本值:
text()// 取得所有匹配元素的內容,只有文本內容,沒有標簽 text(val)// 設置所有匹配元素的內容,不識別標簽,將標簽作為文本插入進去
值:
val() // 取得第一個匹配元素的當前值 val(val) // 設置所有匹配元素的值 val([val1,val2]) // 設置多選的checkbox,多選select的值
自定義登陸校驗示例:
<!DOCTYPE html> <html lang="zh-CN"> <head> <meta charset="UTF-8"> <meta http-equiv="x-ua-compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>文本操作之登錄驗證</title> <style> .error { color: red; } </style> </head> <body> <form action=""> <div> <label for="input-name">用戶名</label> <input type="text" id="input-name" name="name"> <span class="error"></span> </div> <div> <label for="input-password">密碼</label> <input type="password" id="input-password" name="password"> <span class="error"></span> </div> <div> <input type="button" id="btn" value="提交"> </div> </form> <script src="https://cdn.bootcss.com/jquery/3.2.1/jquery.min.js"></script> <script> $("#btn").click(function () { var username = $("#input-name").val(); var password = $("#input-password").val(); if (username.length === 0) { $("#input-name").siblings(".error").text("用戶名不能為空"); } if (password.length === 0) { $("#input-password").siblings(".error").text("密碼不能為空"); } }) </script> </body> </html>
四、屬性操作
用與ID自帶屬性等或自定義屬性:
attr(attrName)// 返回第一個匹配元素的屬性值 attr(attrName, attrValue)// 為所有匹配元素設置一個屬性值 attr({k1: v1, k2:v2})// 為所有匹配元素設置多個屬性值 removeAttr()// 從每一個匹配的元素中刪除一個屬性
用於checkbox和radio
prop() // 獲取屬性 removeProp() // 移除屬性
註意:
在1.x及2.x版本的jQuery中使用attr對checkbox進行賦值操作時會出bug,在3.x版本的jQuery中則沒有這個問題。為了兼容性,我們在處理checkbox和radio的時候盡量使用特定的prop(),不要使用attr("checked", "checked")。
<input type="checkbox" value="1"> <input type="radio" value="2"> <script> $(":checkbox[value=‘1‘]").prop("checked", true); //設置讓其選中,設置選中或不選中的時候要註意傳的參數那個true和false不能寫成字符串形式‘true‘\‘false‘ .prop(‘checked‘,‘true‘)是不對的 $(":radio[value=‘2‘]").prop("checked", true); </script>
prop和attr的區別
prop檢索的是DOM屬性,attr檢索的是HTML屬性
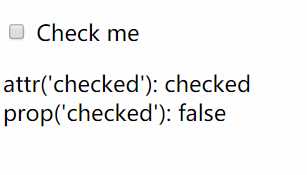
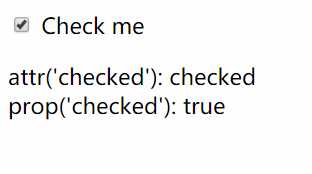
1.對於標簽上有的能看到的屬性和自定義屬性都用attr 2.對於返回布爾值的比如checkbox、radio和option的是否被選中或者設置其被選中與取消選中都用prop。
具有 true 和 false 兩個屬性的屬性,如 checked, selected 或者 disabled 使用prop(),其他的使用 attr()
示例:全選,反選,取消
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <button id="all">全選</button> <button id="reverse">反選</button> <button id="cancel">取消</button> <table border="1"> <thead> <tr> <th>#</th> <th>頭</th> <th>頭</th> </tr> </thead> <tbody> <tr> <td><input type="checkbox"></td> <td>1</td> <td>1</td> </tr> <tr> <td><input type="checkbox"></td> <td>2</td> <td>2</td> </tr> <tr> <td><input type="checkbox"></td> <td>3</td> <td>3</td> </tr> </tbody> </table> <script src="jquery.js"></script> <script> // 點擊全選按鈕 選中所有的checkbox // DOM綁定事件方法 // $("#all")[0].onclick = function(){} // jQuery綁定事件方法 $("#all").click(function () { $(":checkbox").prop(‘checked‘, true); }); // 取消 $("#cancel").on("click", function () { $(":checkbox").prop(‘checked‘, false); }); // 反選 $("#reverse").click(function () { // 1. 找到所有選中的checkbox取消選中 // $("input:checked").prop(‘checked‘, false); // // 2. 找到沒有選中的checkbox選中 // $("input:not(:checked)").prop(‘checked‘, true); //你會發現上面這麽寫,不行,為什麽呢?因為你做了第一步操作之後,再做第二步操作的時候,所有標簽就已經全部取消選中了,所以第二步就把所有標簽選中了 // 方法1. for循環所有的checkbox,挨個判斷原來選中就取消選中,原來沒選中就選中 var $checkbox = $(":checkbox"); for (var i=0;i<$checkbox.length;i++){ // 獲取原來的選中與否的狀態 var status = $($checkbox[i]).prop(‘checked‘); $($checkbox[i]).prop(‘checked‘, !status); } // 方法2. 先用變量把標簽原來的狀態保存下來 // var $unchecked = $("input:not(:checked)"); // var $checked = $("input:checked"); // // $unchecked.prop(‘checked‘, true); // $checked.prop(‘checked‘, false); }) </script> </body> </html>
五、文檔處理
添加到指定元素內部的後面
$(A).append(B)// 把B追加到A
$(A).appendTo(B)// 把A追加到B
添加到指定元素內部的前面
$(A).prepend(B)// 把B前置到A
$(A).prependTo(B)// 把A前置到B
添加到指定元素外部的後面
$(A).after(B)// 把B放到A的後面
$(A).insertAfter(B)// 把A放到B的後面
添加到指定元素外部的前面
$(A).before(B)// 把B放到A的前面
$(A).insertBefore(B)// 把A放到B的前面
移除和清空元素
remove()// 從DOM中刪除所有匹配的元素。
empty()// 刪除匹配的元素集合中所有的子節點,包括文本被全部刪除,但是匹配的元素還在
替換
replaceWith()
replaceAll()
克隆:
clone() // 參數為ture
克隆示例:
<!DOCTYPE html> <html lang="zh-CN"> <head> <meta charset="UTF-8"> <meta http-equiv="x-ua-compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>克隆</title> <style> #b1 { background-color: deeppink; padding: 5px; color: white; margin: 5px; } #b2 { background-color: dodgerblue; padding: 5px; color: white; margin: 5px; } </style> </head> <body> <button id="b1">按鈕1</button> <hr> <button id="b2">按鈕2</button> <script src="jquery-3.2.1.min.js"></script> <script> // clone方法不加參數true,克隆標簽但不克隆標簽帶的事件 $("#b1").on("click", function () { $(this).clone().insertAfter(this); }); // clone方法加參數true,克隆標簽並且克隆標簽帶的事件 $("#b2").on("click", function () { $(this).clone(true).insertAfter(this); }); </script> </body> </html>
jQuery操作標簽--樣式、文本、屬性操作, 文檔處理