多執行緒相關 Thread、Runnable、Callable、Futrue類關係與區別
阿新 • • 發佈:2019-02-19
Java中存在Runnable、Callable、Future、FutureTask這幾個與執行緒相關的類或者介面,在Java中也是比較重要的幾個概念,我們通過下面的簡單示例來了解一下它們的作用於區別。
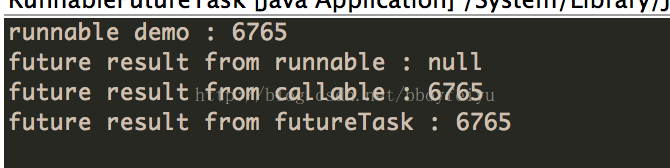
Runnable
其中Runnable應該是我們最熟悉的介面,它只有一個run()函式,用於將耗時操作寫在其中,該函式沒有返回值。然後使用某個執行緒去執行該runnable即可實現多執行緒,Thread類在呼叫start()函式後就是執行的是Runnable的run()函式。Runnable的宣告如下 :
- publicinterface Runnable {
- /**
- * When an object implementing interface <code>Runnable</code> is used
- * to create a thread, starting the thread causes the object's
- * <code>run</code> method to be called in that separately executing
- * thread.
- * <p>
- *
- * @see java.lang.Thread#run()
- */
- publicabstractvoid run();
- }
Callable
Callable與Runnable的功能大致相似,Callable中有一個call()函式,但是call()函式有返回值 ,而Runnable的run()函式不能將結果返回給客戶程式。Callable的宣告如下 :
- publicinterface Callable<V> {
- /**
- * Computes a result, or throws an exception if unable to do so.
- *
- * @return computed result
- * @throws Exception if unable to compute a result
- */
- V call() throws Exception;
- }
Future
Executor就是Runnable和Callable的排程容器,Future就是對於具體的Runnable或者Callable任務的執行結果進行
取消、查詢是否完成、獲取結果、設定結果操作。get方法會阻塞,直到任務返回結果(Future簡介)。Future宣告如下 :
- /**
- * @see FutureTask
- * @see Executor
- * @since 1.5
- * @author Doug Lea
- * @param <V> The result type returned by this Future's <tt>get</tt> method
- */
- publicinterface Future<V> {
- /**
- * Attempts to cancel execution of this task. This attempt will
- * fail if the task has already completed, has already been cancelled,
- * or could not be cancelled for some other reason. If successful,
- * and this task has not started when <tt>cancel</tt> is called,
- * this task should never run. If the task has already started,
- * then the <tt>mayInterruptIfRunning</tt> parameter determines
- * whether the thread executing this task should be interrupted in
- * an attempt to stop the task. *
- */
- boolean cancel(boolean mayInterruptIfRunning);
- /**
- * Returns <tt>true</tt> if this task was cancelled before it completed
- * normally.
- */
- boolean isCancelled();
- /**
- * Returns <tt>true</tt> if this task completed.
- *
- */
- boolean isDone();
- /**
- * Waits if necessary for the computation to complete, and then
- * retrieves its result.
- *
- * @return the computed result
- */
- V get() throws InterruptedException, ExecutionException;
- /**
- * Waits if necessary for at most the given time for the computation
- * to complete, and then retrieves its result, if available.
- *
- * @param timeout the maximum time to wait
- * @param unit the time unit of the timeout argument
- * @return the computed result
- */
- V get(long timeout, TimeUnit unit)
- throws InterruptedException, ExecutionException, TimeoutException;
- }
FutureTask
FutureTask則是一個RunnableFuture<V>,而RunnableFuture實現了Runnbale又實現了Futrue<V>這兩個介面,
- publicclass FutureTask<V> implements RunnableFuture<V>
- publicinterface RunnableFuture<V> extends Runnable, Future<V> {
- /**
- * Sets this Future to the result of its computation
- * unless it has been cancelled.
- */
- void run();
- }
另外它還可以包裝Runnable和Callable<V>, 由建構函式注入依賴。
- public FutureTask(Callable<V> callable) {
- if (callable == null)
- thrownew NullPointerException();
- this.callable = callable;
- this.state = NEW; // ensure visibility of callable
- }
- public FutureTask(Runnable runnable, V result) {
- this.callable = Executors.callable(runnable, result);
- this.state = NEW; // ensure visibility of callable
- }
- publicstatic <T> Callable<T> callable(Runnable task, T result) {
- if (task == null)
- thrownew NullPointerException();
- returnnew RunnableAdapter<T>(task, result);
- }
- /**
- * A callable that runs given task and returns given result
- */
- staticfinalclass RunnableAdapter<T> implements Callable<T> {
- final Runnable task;
- final T result;
- RunnableAdapter(Runnable task, T result) {
- this.task = task;
- this.result = result;
- }
- public T call() {
- task.run();
- return result;
- }
- }
由於FutureTask實現了Runnable,因此它既可以通過Thread包裝來直接執行,也可以提交給ExecuteService來執行。
並且還可以直接通過get()函式獲取執行結果,該函式會阻塞,直到結果返回。因此FutureTask既是Future、
Runnable,又是包裝了Callable( 如果是Runnable最終也會被轉換為Callable ), 它是這兩者的合體。
簡單示例
- package com.effective.java.concurrent.task;
- import java.util.concurrent.Callable;
- import java.util.concurrent.ExecutionException;
- import java.util.concurrent.ExecutorService;
- import java.util.concurrent.Executors;
- import java.util.concurrent.Future;
- import java.util.concurrent.FutureTask;
- /**
- *
- * @author mrsimple
- *
- */
- publicclass RunnableFutureTask {
- /**
- * ExecutorService
- */
- static ExecutorService mExecutor = Executors.newSingleThreadExecutor();
- /**
- *
- * @param args
- */
- publicstaticvoid main(String[] args) {
- runnableDemo();
- futureDemo();
- }
- /**
- * runnable, 無返回值
- */
- staticvoid runnableDemo() {
- new Thread(new Runnable() {
- @Override
- publicvoid run() {
- System.out.println("runnable demo : " + fibc(20));
- }
- }).start();
- }
- /**
- * 其中Runnable實現的是void run()方法,無返回值;Callable實現的是 V
- * call()方法,並且可以返回執行結果。其中Runnable可以提交給Thread來包裝下
- * ,直接啟動一個執行緒來執行,而Callable則一般都是提交給ExecuteService來執行。
- */
- staticvoid futureDemo() {
- try {
- /**
- * 提交runnable則沒有返回值, future沒有資料
- */
- Future<?> result = mExecutor.submit(new Runnable() {
- @Override
- publicvoid run() {
- fibc(20);
- }
- });
- System.out.println("future result from runnable : " + result.get());
- /**
- * 提交Callable, 有返回值, future中能夠獲取返回值
- */
- Future<Integer> result2 = mExecutor.submit(new Callable<Integer>() {
- @Override
- public Integer call() throws Exception {
- return fibc(20);
- }
- });
- System.out
- .println("future result from callable : " + result2.get());
- /**
- * FutureTask則是一個RunnableFuture<V>,即實現了Runnbale又實現了Futrue<V>這兩個介面,
- * 另外它還可以包裝Runnable(實際上會轉換為Callable)和Callable
- * <V>,所以一般來講是一個符合體了,它可以通過Thread包裝來直接執行,也可以提交給ExecuteService來執行
- * ,並且還可以通過v get()返回執行結果,線上程體沒有執行完成的時候,主執行緒一直阻塞等待,執行完則直接返回結果。
- */
- FutureTask<Integer> futureTask = new FutureTask<Integer>(
- new Callable<Integer>() {
- @Override
- public Integer call() throws Exception {
- return fibc(20);
- }
- });
- // 提交futureTask
- mExecutor.submit(futureTask) ;
- System.out.println("future result from futureTask : "
- + futureTask.get());
- } catch (InterruptedException e) {
- e.printStackTrace();
- } catch (ExecutionException e) {
- e.printStackTrace();
- }
- }
- /**
- * 效率底下的斐波那契數列, 耗時的操作
- *
- * @param num
- * @return
- */
- staticint fibc(int num) {
- if (num == 0) {
- return0;
- }
- if (num == 1) {
- return1;
- }
- return fibc(num - 1) + fibc(num - 2);
- }
- }
輸出結果
- 頂
- 3
- 踩
- 0