Python開發【第四篇】:Python基礎之函數
三元運算
三元運算(三目運算),是對簡單的條件語句的縮寫。
# 書寫格式 result = 值1 if 條件 else 值2 # 如果條件成立,那麽將 “值1” 賦值給result變量,否則,將“值2”賦值給result變量
基本數據類型補充
set
set集合,是一個無序且不重復的元素集合
class set(object): """ set() -> new empty set object set(iterable) -> new set object Build an unordered collection of unique elements. """ def add(self, *args, **kwargs): # real signature unknown """ Add an element to a set,添加元素 This has no effect if the element is already present. """ pass def clear(self, *args, **kwargs): # real signature unknown """ Remove all elements from this set. 清除內容""" pass def copy(self, *args, **kwargs): # real signature unknown """ Return a shallow copy of a set. 淺拷貝 """ pass def difference(self, *args, **kwargs): # real signature unknown """ Return the difference of two or more sets as a new set. A中存在,B中不存在 (i.e. all elements that are in this set but not the others.) """ pass def difference_update(self, *args, **kwargs): # real signature unknown """ Remove all elements of another set from this set. 從當前集合中刪除和B中相同的元素""" pass def discard(self, *args, **kwargs): # real signature unknown """ Remove an element from a set if it is a member. If the element is not a member, do nothing. 移除指定元素,不存在不保錯 """ pass def intersection(self, *args, **kwargs): # real signature unknown """ Return the intersection of two sets as a new set. 交集 (i.e. all elements that are in both sets.) """ pass def intersection_update(self, *args, **kwargs): # real signature unknown """ Update a set with the intersection of itself and another. 取交集並更更新到A中 """ pass def isdisjoint(self, *args, **kwargs): # real signature unknown """ Return True if two sets have a null intersection. 如果沒有交集,返回True,否則返回False""" pass def issubset(self, *args, **kwargs): # real signature unknown """ Report whether another set contains this set. 是否是子序列""" pass def issuperset(self, *args, **kwargs): # real signature unknown """ Report whether this set contains another set. 是否是父序列""" pass def pop(self, *args, **kwargs): # real signature unknown """ Remove and return an arbitrary set element. Raises KeyError if the set is empty. 移除元素 """ pass def remove(self, *args, **kwargs): # real signature unknown """ Remove an element from a set; it must be a member. If the element is not a member, raise a KeyError. 移除指定元素,不存在保錯 """ pass def symmetric_difference(self, *args, **kwargs): # real signature unknown """ Return the symmetric difference of two sets as a new set. 對稱差集 (i.e. all elements that are in exactly one of the sets.) """ pass def symmetric_difference_update(self, *args, **kwargs): # real signature unknown """ Update a set with the symmetric difference of itself and another. 對稱差集,並更新到a中 """ pass def union(self, *args, **kwargs): # real signature unknown """ Return the union of sets as a new set. 並集 (i.e. all elements that are in either set.) """ pass def update(self, *args, **kwargs): # real signature unknown """ Update a set with the union of itself and others. 更新 """ pass
練習:尋找差異
# 數據庫中原有 old_dict = { "#1":{ ‘hostname‘:c1, ‘cpu_count‘: 2, ‘mem_capicity‘: 80 }, "#2":{ ‘hostname‘:c1, ‘cpu_count‘: 2, ‘mem_capicity‘: 80 } "#3":{ ‘hostname‘:c1, ‘cpu_count‘: 2, ‘mem_capicity‘: 80 } } # cmdb 新匯報的數據 new_dict = { "#1":{ ‘hostname‘:c1, ‘cpu_count‘: 2, ‘mem_capicity‘: 800 }, "#3":{ ‘hostname‘:c1, ‘cpu_count‘: 2, ‘mem_capicity‘: 80 } "#4":{ ‘hostname‘:c2, ‘cpu_count‘: 2, ‘mem_capicity‘: 80 } }
需要刪除:?
需要新建:?
需要更新:?
註意:無需考慮內部元素是否改變,只要原來存在,新匯報也存在,就是需要更新
深淺拷貝
一、數字和字符串
對於 數字 和 字符串 而言,賦值、淺拷貝和深拷貝無意義,因為其永遠指向同一個內存地址。
import copy # ######### 數字、字符串 ######### n1 = 123 # n1 = "i am alex age 10" print(id(n1)) # ## 賦值 ## n2 = n1 print(id(n2)) # ## 淺拷貝 ## n2 = copy.copy(n1) print(id(n2)) # ## 深拷貝 ## n3 = copy.deepcopy(n1) print(id(n3))
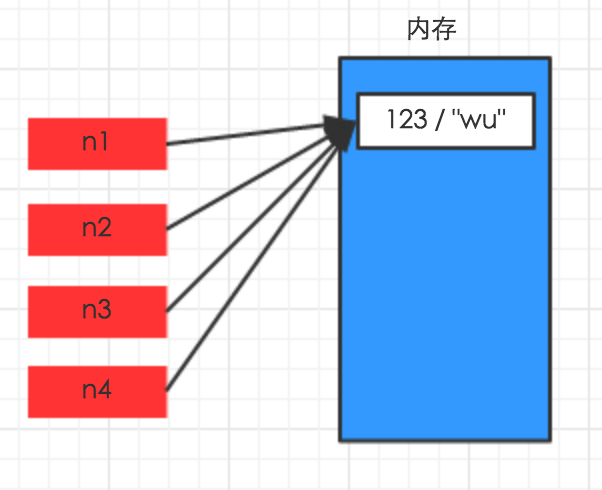
二、其他基本數據類型
對於字典、元祖、列表 而言,進行賦值、淺拷貝和深拷貝時,其內存地址的變化是不同的。
1、賦值
賦值,只是創建一個變量,該變量指向原來內存地址,如:
n1 = {"k1": "wu", "k2": 123, "k3": ["alex", 456]} n2 = n1
2、淺拷貝
淺拷貝,在內存中只額外創建第一層數據
import copy n1 = {"k1": "wu", "k2": 123, "k3": ["alex", 456]} n3 = copy.copy(n1)
3、深拷貝
深拷貝,在內存中將所有的數據重新創建一份(排除最後一層,即:python內部對字符串和數字的優化)
import copy n1 = {"k1": "wu", "k2": 123, "k3": ["alex", 456]} n4 = copy.deepcopy(n1)
函數
一、背景
在學習函數之前,一直遵循:面向過程編程,即:根據業務邏輯從上到下實現功能,其往往用一長段代碼來實現指定功能,開發過程中最常見的操作就是粘貼復制,也就是將之前實現的代碼塊復制到現需功能處,如下:
while True: if cpu利用率 > 90%: #發送郵件提醒 連接郵箱服務器 發送郵件 關閉連接 if 硬盤使用空間 > 90%: #發送郵件提醒 連接郵箱服務器 發送郵件 關閉連接 if 內存占用 > 80%: #發送郵件提醒 連接郵箱服務器 發送郵件 關閉連接
腚眼一看上述代碼,if條件語句下的內容可以被提取出來公用,如下:
def 發送郵件(內容) #發送郵件提醒 連接郵箱服務器 發送郵件 關閉連接 while True: if cpu利用率 > 90%: 發送郵件(‘CPU報警‘) if 硬盤使用空間 > 90%: 發送郵件(‘硬盤報警‘) if 內存占用 > 80%:
對於上述的兩種實現方式,第二次必然比第一次的重用性和可讀性要好,其實這就是函數式編程和面向過程編程的區別:
- 函數式:將某功能代碼封裝到函數中,日後便無需重復編寫,僅調用函數即可
- 面向對象:對函數進行分類和封裝,讓開發“更快更好更強...”
函數式編程最重要的是增強代碼的重用性和可讀性
二、定義和使用
def 函數名(參數): ... 函數體 ... 返回值
函數的定義主要有如下要點:
- def:表示函數的關鍵字
- 函數名:函數的名稱,日後根據函數名調用函數
- 函數體:函數中進行一系列的邏輯計算,如:發送郵件、計算出 [11,22,38,888,2]中的最大數等...
- 參數:為函數體提供數據
- 返回值:當函數執行完畢後,可以給調用者返回數據。
1、返回值
函數是一個功能塊,該功能到底執行成功與否,需要通過返回值來告知調用者。
以上要點中,比較重要有參數和返回值:
def 發送短信(): 發送短信的代碼... if 發送成功: return True else: return False while True: # 每次執行發送短信函數,都會將返回值自動賦值給result # 之後,可以根據result來寫日誌,或重發等操作 result = 發送短信() if result == False: 記錄日誌,短信發送失敗...
2、參數
為什麽要有參數?

def CPU報警郵件() #發送郵件提醒 連接郵箱服務器 發送郵件 關閉連接 def 硬盤報警郵件() #發送郵件提醒 連接郵箱服務器 發送郵件 關閉連接 def 內存報警郵件() #發送郵件提醒 連接郵箱服務器 發送郵件 關閉連接 while True: if cpu利用率 > 90%: CPU報警郵件() if 硬盤使用空間 > 90%: 硬盤報警郵件() if 內存占用 > 80%: 內存報警郵件()無參數實現

def 發送郵件(郵件內容) #發送郵件提醒 連接郵箱服務器 發送郵件 關閉連接 while True: if cpu利用率 > 90%: 發送郵件("CPU報警了。") if 硬盤使用空間 > 90%: 發送郵件("硬盤報警了。") if 內存占用 > 80%: 發送郵件("內存報警了。")有參數實現
函數的有三中不同的參數:
- 普通參數
- 默認參數
- 動態參數

# ######### 定義函數 ######### # name 叫做函數func的形式參數,簡稱:形參 def func(name): print name # ######### 執行函數 ######### # ‘wupeiqi‘ 叫做函數func的實際參數,簡稱:實參 func(‘wupeiqi‘)普通參數

def func(name, age = 18): print "%s:%s" %(name,age) # 指定參數 func(‘wupeiqi‘, 19) # 使用默認參數 func(‘alex‘) 註:默認參數需要放在參數列表最後默認參數

def func(*args): print args # 執行方式一 func(11,33,4,4454,5) # 執行方式二 li = [11,2,2,3,3,4,54] func(*li)動態參數

def func(**kwargs): print args # 執行方式一 func(name=‘wupeiqi‘,age=18) # 執行方式二 li = {‘name‘:‘wupeiqi‘, age:18, ‘gender‘:‘male‘} func(**li)動態參數

def func(*args, **kwargs): print args print kwargs動態參數
擴展:發送郵件實例

import smtplib from email.mime.text import MIMEText from email.utils import formataddr msg = MIMEText(‘郵件內容‘, ‘plain‘, ‘utf-8‘) msg[‘From‘] = formataddr(["武沛齊",‘[email protected]‘]) msg[‘To‘] = formataddr(["走人",‘[email protected]‘]) msg[‘Subject‘] = "主題" server = smtplib.SMTP("smtp.126.com", 25) server.login("[email protected]", "郵箱密碼") server.sendmail(‘[email protected]‘, [‘[email protected]‘,], msg.as_string()) server.quit()發送郵件實例
內置函數
註:查看詳細猛擊這裏
open函數,該函數用於文件處理
操作文件時,一般需要經歷如下步驟:
- 打開文件
- 操作文件
一、打開文件
文件句柄 = open(‘文件路徑‘, ‘模式‘)
打開文件時,需要指定文件路徑和以何等方式打開文件,打開後,即可獲取該文件句柄,日後通過此文件句柄對該文件操作。
打開文件的模式有:
- r ,只讀模式【默認】
- w,只寫模式【不可讀;不存在則創建;存在則清空內容;】
- x, 只寫模式【不可讀;不存在則創建,存在則報錯】
- a, 追加模式【可讀; 不存在則創建;存在則只追加內容;】
"+" 表示可以同時讀寫某個文件
- r+, 讀寫【可讀,可寫】
- w+,寫讀【可讀,可寫】
- x+ ,寫讀【可讀,可寫】
- a+, 寫讀【可讀,可寫】
"b"表示以字節的方式操作
- rb 或 r+b
- wb 或 w+b
- xb 或 w+b
- ab 或 a+b
註:以b方式打開時,讀取到的內容是字節類型,寫入時也需要提供字節類型
二、操作

class file(object) def close(self): # real signature unknown; restored from __doc__ 關閉文件 """ close() -> None or (perhaps) an integer. Close the file. Sets data attribute .closed to True. A closed file cannot be used for further I/O operations. close() may be called more than once without error. Some kinds of file objects (for example, opened by popen()) may return an exit status upon closing. """ def fileno(self): # real signature unknown; restored from __doc__ 文件描述符 """ fileno() -> integer "file descriptor". This is needed for lower-level file interfaces, such os.read(). """ return 0 def flush(self): # real signature unknown; restored from __doc__ 刷新文件內部緩沖區 """ flush() -> None. Flush the internal I/O buffer. """ pass def isatty(self): # real signature unknown; restored from __doc__ 判斷文件是否是同意tty設備 """ isatty() -> true or false. True if the file is connected to a tty device. """ return False def next(self): # real signature unknown; restored from __doc__ 獲取下一行數據,不存在,則報錯 """ x.next() -> the next value, or raise StopIteration """ pass def read(self, size=None): # real signature unknown; restored from __doc__ 讀取指定字節數據 """ read([size]) -> read at most size bytes, returned as a string. If the size argument is negative or omitted, read until EOF is reached. Notice that when in non-blocking mode, less data than what was requested may be returned, even if no size parameter was given. """ pass def readinto(self): # real signature unknown; restored from __doc__ 讀取到緩沖區,不要用,將被遺棄 """ readinto() -> Undocumented. Don‘t use this; it may go away. """ pass def readline(self, size=None): # real signature unknown; restored from __doc__ 僅讀取一行數據 """ readline([size]) -> next line from the file, as a string. Retain newline. A non-negative size argument limits the maximum number of bytes to return (an incomplete line may be returned then). Return an empty string at EOF. """ pass def readlines(self, size=None): # real signature unknown; restored from __doc__ 讀取所有數據,並根據換行保存值列表 """ readlines([size]) -> list of strings, each a line from the file. Call readline() repeatedly and return a list of the lines so read. The optional size argument, if given, is an approximate bound on the total number of bytes in the lines returned. """ return [] def seek(self, offset, whence=None): # real signature unknown; restored from __doc__ 指定文件中指針位置 """ seek(offset[, whence]) -> None. Move to new file position. Argument offset is a byte count. Optional argument whence defaults to (offset from start of file, offset should be >= 0); other values are 1 (move relative to current position, positive or negative), and 2 (move relative to end of file, usually negative, although many platforms allow seeking beyond the end of a file). If the file is opened in text mode, only offsets returned by tell() are legal. Use of other offsets causes undefined behavior. Note that not all file objects are seekable. """ pass def tell(self): # real signature unknown; restored from __doc__ 獲取當前指針位置 """ tell() -> current file position, an integer (may be a long integer). """ pass def truncate(self, size=None): # real signature unknown; restored from __doc__ 截斷數據,僅保留指定之前數據 """ truncate([size]) -> None. Truncate the file to at most size bytes. Size defaults to the current file position, as returned by tell(). """ pass def write(self, p_str): # real signature unknown; restored from __doc__ 寫內容 """ write(str) -> None. Write string str to file. Note that due to buffering, flush() or close() may be needed before the file on disk reflects the data written. """ pass def writelines(self, sequence_of_strings): # real signature unknown; restored from __doc__ 將一個字符串列表寫入文件 """ writelines(sequence_of_strings) -> None. Write the strings to the file. Note that newlines are not added. The sequence can be any iterable object producing strings. This is equivalent to calling write() for each string. """ pass def xreadlines(self): # real signature unknown; restored from __doc__ 可用於逐行讀取文件,非全部 """ xreadlines() -> returns self. For backward compatibility. File objects now include the performance optimizations previously implemented in the xreadlines module. """ pass2.x

class TextIOWrapper(_TextIOBase): """ Character and line based layer over a BufferedIOBase object, buffer. encoding gives the name of the encoding that the stream will be decoded or encoded with. It defaults to locale.getpreferredencoding(False). errors determines the strictness of encoding and decoding (see help(codecs.Codec) or the documentation for codecs.register) and defaults to "strict". newline controls how line endings are handled. It can be None, ‘‘, ‘\n‘, ‘\r‘, and ‘\r\n‘. It works as follows: * On input, if newline is None, universal newlines mode is enabled. Lines in the input can end in ‘\n‘, ‘\r‘, or ‘\r\n‘, and these are translated into ‘\n‘ before being returned to the caller. If it is ‘‘, universal newline mode is enabled, but line endings are returned to the caller untranslated. If it has any of the other legal values, input lines are only terminated by the given string, and the line ending is returned to the caller untranslated. * On output, if newline is None, any ‘\n‘ characters written are translated to the system default line separator, os.linesep. If newline is ‘‘ or ‘\n‘, no translation takes place. If newline is any of the other legal values, any ‘\n‘ characters written are translated to the given string. If line_buffering is True, a call to flush is implied when a call to write contains a newline character. """ def close(self, *args, **kwargs): # real signature unknown 關閉文件 pass def fileno(self, *args, **kwargs): # real signature unknown 文件描述符 pass def flush(self, *args, **kwargs): # real signature unknown 刷新文件內部緩沖區 pass def isatty(self, *args, **kwargs): # real signature unknown 判斷文件是否是同意tty設備 pass def read(self, *args, **kwargs): # real signature unknown 讀取指定字節數據 pass def readable(self, *args, **kwargs): # real signature unknown 是否可讀 pass def readline(self, *args, **kwargs): # real signature unknown 僅讀取一行數據 pass def seek(self, *args, **kwargs): # real signature unknown 指定文件中指針位置 pass def seekable(self, *args, **kwargs): # real signature unknown 指針是否可操作 pass def tell(self, *args, **kwargs): # real signature unknown 獲取指針位置 pass def truncate(self, *args, **kwargs): # real signature unknown 截斷數據,僅保留指定之前數據 pass def writable(self, *args, **kwargs): # real signature unknown 是否可寫 pass def write(self, *args, **kwargs): # real signature unknown 寫內容 pass def __getstate__(self, *args, **kwargs): # real signature unknown pass def __init__(self, *args, **kwargs): # real signature unknown pass @staticmethod # known case of __new__ def __new__(*args, **kwargs): # real signature unknown """ Create and return a new object. See help(type) for accurate signature. """ pass def __next__(self, *args, **kwargs): # real signature unknown """ Implement next(self). """ pass def __repr__(self, *args, **kwargs): # real signature unknown """ Return repr(self). """ pass buffer = property(lambda self: object(), lambda self, v: None, lambda self: None) # default closed = property(lambda self: object(), lambda self, v: None, lambda self: None) # default encoding = property(lambda self: object(), lambda self, v: None, lambda self: None) # default errors = property(lambda self: object(), lambda self, v: None, lambda self: None) # default line_buffering = property(lambda self: object(), lambda self, v: None, lambda self: None) # default name = property(lambda self: object(), lambda self, v: None, lambda self: None) # default newlines = property(lambda self: object(), lambda self, v: None, lambda self: None) # default _CHUNK_SIZE = property(lambda self: object(), lambda self, v: None, lambda self: None) # default _finalizing = property(lambda self: object(), lambda self, v: None, lambda self: None) # default3.x
三、管理上下文
為了避免打開文件後忘記關閉,可以通過管理上下文,即:
with open(‘log‘,‘r‘) as f: ...
如此方式,當with代碼塊執行完畢時,內部會自動關閉並釋放文件資源。
在Python 2.7 及以後,with又支持同時對多個文件的上下文進行管理,即:
with open(‘log1‘) as obj1, open(‘log2‘) as obj2: pass
lambda表達式
學習條件運算時,對於簡單的 if else 語句,可以使用三元運算來表示,即:
# 普通條件語句 if 1 == 1: name = ‘wupeiqi‘ else: name = ‘alex‘ # 三元運算 name = ‘wupeiqi‘ if 1 == 1 else ‘alex‘
對於簡單的函數,也存在一種簡便的表示方式,即:lambda表達式
# ###################### 普通函數 ###################### # 定義函數(普通方式) def func(arg): return arg + 1 # 執行函數 result = func(123) # ###################### lambda ###################### # 定義函數(lambda表達式) my_lambda = lambda arg : arg + 1 # 執行函數 result = my_lambda(123)
遞歸
利用函數編寫如下數列:
斐波那契數列指的是這樣一個數列 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233,377,610,987,1597,2584,4181,6765,10946,17711,28657,46368...
def func(arg1,arg2): if arg1 == 0: print arg1, arg2 arg3 = arg1 + arg2 print arg3 func(arg2, arg3) func(0,1)
練習題
1、簡述普通參數、指定參數、默認參數、動態參數的區別
2、寫函數,計算傳入字符串中【數字】、【字母】、【空格] 以及 【其他】的個數
3、寫函數,判斷用戶傳入的對象(字符串、列表、元組)長度是否大於5。
4、寫函數,檢查用戶傳入的對象(字符串、列表、元組)的每一個元素是否含有空內容。
5、寫函數,檢查傳入列表的長度,如果大於2,那麽僅保留前兩個長度的內容,並將新內容返回給調用者。
6、寫函數,檢查獲取傳入列表或元組對象的所有奇數位索引對應的元素,並將其作為新列表返回給調用者。
7、寫函數,檢查傳入字典的每一個value的長度,如果大於2,那麽僅保留前兩個長度的內容,並將新內容返回給調用者。
dic = {"k1": "v1v1", "k2": [11,22,33,44]} PS:字典中的value只能是字符串或列表
8、寫函數,利用遞歸獲取斐波那契數列中的第 10 個數,並將該值返回給調用者。
Python開發【第四篇】:Python基礎之函數